Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceRequest.cs / 4 / DataServiceRequest.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Xml; ///non-generic placeholder for generic implementation public abstract class DataServiceRequest { ///internal constructor so that only our assembly can provide an implementation internal DataServiceRequest() { } ///Element Type public abstract Type ElementType { get; } ///Gets the URI for a the query public abstract Uri RequestUri { get; } ///Gets the URI for a the query ///a string with the URI public override string ToString() { return this.RequestUri.ToString(); } ////// get an enumerable materializes the objects the response /// ///element type /// context /// reader /// elementType ///an enumerable internal static object CreateMaterializer(DataServiceContext context, XmlReader reader, Type elementType) { Debug.Assert(null == elementType, "non-null elementType with strongly typed create delegate"); return new MaterializeAtom.MaterializeAtomT (context, reader); } /// /// get an enumerable materializes the objects the response /// ///element type /// context /// contentType /// method to get http response stream ///an enumerable internal static IEnumerableMaterialize (DataServiceContext context, string contentType, Func response) { Func create = CreateMaterializer ; return (IEnumerable )context.GetMaterializer(null, contentType, response, create); } /// /// Creates a instance of strongly typed DataServiceRequest with the given element type. /// /// element type for the DataServiceRequest. /// constructor parameter. ///returns the strongly typed DataServiceRequest instance. internal static DataServiceRequest GetInstance(Type elementType, Uri requestUri) { Type genericType = typeof(DataServiceRequest<>).MakeGenericType(elementType); return (DataServiceRequest)Activator.CreateInstance(genericType, new object[] { requestUri }); } #if !ASTORIA_LIGHT // Synchronous methods not available ////// execute uri and materialize result /// ///element type /// context /// uri to execute ///enumerable of results internal IEnumerableExecute (DataServiceContext context, Uri requestUri) { Debug.Assert(null != context, "context is null"); IEnumerable result = null; QueryAsyncResult response = null; try { DataServiceRequest serviceRequest = new DataServiceRequest (requestUri); HttpWebRequest request = context.CreateRequest(requestUri, XmlConstants.HttpMethodGet, false, null); response = new QueryAsyncResult(this, "Execute", serviceRequest, request, null, null); response.Execute(null); if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } catch (InvalidOperationException ex) { QueryOperationResponse operationResponse = response.GetResponse(result, typeof(TElement)); if (null != operationResponse) { operationResponse.Error = ex; throw new DataServiceQueryException(Strings.DataServiceException_GeneralError, ex, operationResponse); } throw; } } #endif /// enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal abstract System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse); /// /// Begins an asynchronous request to an Internet resource. /// /// source of execute (DataServiceQuery or DataServiceContext /// context /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. internal IAsyncResult BeginExecute(object source, DataServiceContext context, AsyncCallback callback, object state) { Debug.Assert(null != context, "context is null"); HttpWebRequest request = context.CreateRequest(this.RequestUri, XmlConstants.HttpMethodGet, false, null); QueryAsyncResult result = new QueryAsyncResult(source, "Execute", this, request, callback, state); result.BeginExecute(null); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Xml; ///non-generic placeholder for generic implementation public abstract class DataServiceRequest { ///internal constructor so that only our assembly can provide an implementation internal DataServiceRequest() { } ///Element Type public abstract Type ElementType { get; } ///Gets the URI for a the query public abstract Uri RequestUri { get; } ///Gets the URI for a the query ///a string with the URI public override string ToString() { return this.RequestUri.ToString(); } ////// get an enumerable materializes the objects the response /// ///element type /// context /// reader /// elementType ///an enumerable internal static object CreateMaterializer(DataServiceContext context, XmlReader reader, Type elementType) { Debug.Assert(null == elementType, "non-null elementType with strongly typed create delegate"); return new MaterializeAtom.MaterializeAtomT (context, reader); } /// /// get an enumerable materializes the objects the response /// ///element type /// context /// contentType /// method to get http response stream ///an enumerable internal static IEnumerableMaterialize (DataServiceContext context, string contentType, Func response) { Func create = CreateMaterializer ; return (IEnumerable )context.GetMaterializer(null, contentType, response, create); } /// /// Creates a instance of strongly typed DataServiceRequest with the given element type. /// /// element type for the DataServiceRequest. /// constructor parameter. ///returns the strongly typed DataServiceRequest instance. internal static DataServiceRequest GetInstance(Type elementType, Uri requestUri) { Type genericType = typeof(DataServiceRequest<>).MakeGenericType(elementType); return (DataServiceRequest)Activator.CreateInstance(genericType, new object[] { requestUri }); } #if !ASTORIA_LIGHT // Synchronous methods not available ////// execute uri and materialize result /// ///element type /// context /// uri to execute ///enumerable of results internal IEnumerableExecute (DataServiceContext context, Uri requestUri) { Debug.Assert(null != context, "context is null"); IEnumerable result = null; QueryAsyncResult response = null; try { DataServiceRequest serviceRequest = new DataServiceRequest (requestUri); HttpWebRequest request = context.CreateRequest(requestUri, XmlConstants.HttpMethodGet, false, null); response = new QueryAsyncResult(this, "Execute", serviceRequest, request, null, null); response.Execute(null); if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } catch (InvalidOperationException ex) { QueryOperationResponse operationResponse = response.GetResponse(result, typeof(TElement)); if (null != operationResponse) { operationResponse.Error = ex; throw new DataServiceQueryException(Strings.DataServiceException_GeneralError, ex, operationResponse); } throw; } } #endif /// enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal abstract System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse); /// /// Begins an asynchronous request to an Internet resource. /// /// source of execute (DataServiceQuery or DataServiceContext /// context /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. internal IAsyncResult BeginExecute(object source, DataServiceContext context, AsyncCallback callback, object state) { Debug.Assert(null != context, "context is null"); HttpWebRequest request = context.CreateRequest(this.RequestUri, XmlConstants.HttpMethodGet, false, null); QueryAsyncResult result = new QueryAsyncResult(source, "Execute", this, request, callback, state); result.BeginExecute(null); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
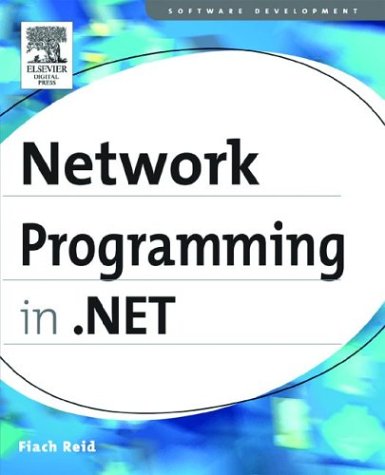
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BooleanFunctions.cs
- LoginCancelEventArgs.cs
- HttpProtocolReflector.cs
- TabPage.cs
- PolyBezierSegment.cs
- DataSourceControl.cs
- DataGridViewRow.cs
- ResourceProviderFactory.cs
- OpCopier.cs
- WrappedIUnknown.cs
- XPathPatternParser.cs
- MergePropertyDescriptor.cs
- RTTypeWrapper.cs
- SqlTypesSchemaImporter.cs
- BehaviorEditorPart.cs
- DropTarget.cs
- AssociationSet.cs
- XsdBuildProvider.cs
- DelegatingChannelListener.cs
- SmiMetaData.cs
- Border.cs
- DictationGrammar.cs
- BorderGapMaskConverter.cs
- IdentityNotMappedException.cs
- AssemblyInfo.cs
- EdmConstants.cs
- COM2Properties.cs
- SafeNativeMethodsOther.cs
- TextBlockAutomationPeer.cs
- ClientTargetSection.cs
- TraceInternal.cs
- XmlSchemaAll.cs
- PartialCachingControl.cs
- SchemaTypeEmitter.cs
- WebBrowserNavigatingEventHandler.cs
- FlowDocumentFormatter.cs
- CodeFieldReferenceExpression.cs
- InputEventArgs.cs
- DriveInfo.cs
- InstallerTypeAttribute.cs
- SelectionGlyph.cs
- XmlSchemaAll.cs
- XmlNamespaceManager.cs
- Pen.cs
- SemanticAnalyzer.cs
- CustomAssemblyResolver.cs
- ObjectViewListener.cs
- GridViewHeaderRowPresenter.cs
- MemberPath.cs
- PrinterSettings.cs
- OperandQuery.cs
- DataKeyCollection.cs
- IDQuery.cs
- TextDecoration.cs
- PersonalizablePropertyEntry.cs
- mda.cs
- BooleanKeyFrameCollection.cs
- BaseProcessor.cs
- ReachPrintTicketSerializerAsync.cs
- SimpleTypeResolver.cs
- DateTimeFormat.cs
- ReadonlyMessageFilter.cs
- DbConnectionPoolGroupProviderInfo.cs
- BamlTreeNode.cs
- safelink.cs
- _SSPISessionCache.cs
- FileUpload.cs
- ImageAttributes.cs
- ApplicationBuildProvider.cs
- ServiceOperationInvoker.cs
- SqlWebEventProvider.cs
- RelationshipConverter.cs
- PerformanceCounterCategory.cs
- SafeArrayTypeMismatchException.cs
- TransactionManagerProxy.cs
- ExpandSegmentCollection.cs
- GridViewColumnHeaderAutomationPeer.cs
- CssStyleCollection.cs
- CompositeCollection.cs
- WindowsToolbar.cs
- TextSpan.cs
- securestring.cs
- WriteableOnDemandPackagePart.cs
- ContextProperty.cs
- SettingsSavedEventArgs.cs
- OleDbWrapper.cs
- HtmlInputSubmit.cs
- Button.cs
- SQLString.cs
- ObservableDictionary.cs
- DecoderReplacementFallback.cs
- DomainUpDown.cs
- RootBrowserWindowAutomationPeer.cs
- RadialGradientBrush.cs
- DynamicActivityXamlReader.cs
- VirtualPath.cs
- HuffCodec.cs
- DefinitionUpdate.cs
- AccessorTable.cs
- CompensationParticipant.cs