Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / OneOfScalarConst.cs / 2 / OneOfScalarConst.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Linq; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; // A class that denotes the boolean expression: "scalarVar in values" // See the comments in OneOfConst for partially and fully-done objects internal class OneOfScalarConst : OneOfConst { #region Constructors // effects: Creates an expression of the form "node in scalar strings // corresponding to values". This constructor is needed for creating // discriminator type conditions internal OneOfScalarConst(JoinTreeNode node, object value, TypeUsage memberType) : this(node, new ScalarConstant(value)) { // CHANGE_[....]_IMPROVE: the memberType stuff has to be consumed } internal OneOfScalarConst(JoinTreeNode node, CellConstant value) : base(new JoinTreeSlot(node), value) { Debug.Assert(value is ScalarConstant || value.IsNull() || value.IsNotNull(), "Scalar, NULL, or NOT NULL expected"); } // effects: Creates an expression of the form "var = value" internal OneOfScalarConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), values, possibleValues) { } // effects: Creates an expression of the form "slot in domain" internal OneOfScalarConst(JoinTreeSlot slot, CellConstantDomain domain) : base(slot, domain) { } #endregion #region Methods // requires: IsFullyDone is true // effects: Fixes the range of this in accordance with range internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(IsFullyDone, "Ranges are fixed only for fully done OneOfConsts"); IEnumerable newPossibleValues = memberDomainMap.GetDomain(Slot.MemberPath); BoolLiteral newLiteral = new OneOfScalarConst(Slot, new CellConstantDomain(range, newPossibleValues)); return newLiteral.GetDomainBoolExpression(memberDomainMap); } // requires: there is at most one negated Constant internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, canSkipIsNotNull, false); } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, canSkipIsNotNull, true); } //Common code for AsCQL and TouserString methods private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, bool canSkipIsNotNull, bool userString) { Debug.Assert(Slot.MemberPath.IsScalarType(), "Expected scalar"); Set constants = new Set (Values.Values, CellConstant.EqualityComparer); // * If no negated cell constant generate the normal list // * If nullable, add AND IS NOT NULL to the list of normal values // * If has a negated cell constant, come up with a simplified // version of the constants, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL NegatedCellConstant negated = null; foreach (CellConstant constant in constants) { NegatedCellConstant tmpConstant = constant as NegatedCellConstant; if (tmpConstant != null) { Debug.Assert(negated == null, "Multiple negated constants?"); negated = tmpConstant; } } if (negated != null) { if (userString) { return negated.AsUserString(builder, blockAlias, constants, Slot.MemberPath, canSkipIsNotNull); } else { return negated.AsCql(builder, blockAlias, constants, Slot.MemberPath, canSkipIsNotNull); } } // We have only positive constants bool containsNull = constants.Contains(CellConstant.Null); constants.Remove(CellConstant.Null); //Constraint counter-example could contain undefined cellconstant. E.g for booleans (for int its optimized out due to negated constants) //we want to treat undefined as nulls if (constants.Contains(CellConstant.Undefined)) { constants.Remove(CellConstant.Undefined); containsNull = true; } if (containsNull) { if (constants.Count > 0) { builder.Append('('); } if (userString) { Slot.MemberPath.ToCompactString(builder, blockAlias); builder.Append(" is NULL"); } else { Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(" IS NULL"); } if (constants.Count > 0) { builder.Append(" OR "); } } if (constants.Count == 0) { return builder; } bool isNullable = Slot.MemberPath.IsNullable; // Generate var IN {...} or var = ... // For nullable members, add (... AND var IS NOT NULL) // so the boolean _from variables never evaluate to null // This is needed because view generation assumes 2-valued boolean logic if (isNullable && canSkipIsNotNull == false) { builder.Append("("); // enclose AND var IS NOT NULL in brackets } if (userString) { Slot.MemberPath.ToCompactString(builder, blockAlias); } else { Slot.MemberPath.AsCql(builder, blockAlias); } if (constants.Count > 1) { // Multiple values builder.Append(" IN {"); bool isFirst = true; foreach (CellConstant constant in constants) { if (isFirst == false) { builder.Append(", "); } isFirst = false; if (userString) { constant.ToCompactString(builder); } else { constant.AsCql(builder, Slot.MemberPath, blockAlias); } } builder.Append("}"); } else { // Single value builder.Append(" = "); if (userString) { constants.Single().ToCompactString(builder); } else { constants.Single().AsCql(builder, Slot.MemberPath, blockAlias); } } if (isNullable && canSkipIsNotNull == false) { // add the AND var IS NOT NULL condition builder.Append(" AND "); if (userString) { Slot.MemberPath.ToCompactString(builder, Strings.ViewGen_EntityInstanceToken); builder.Append(" is not NULL)"); // plus the closing bracket } else { Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL)"); // plus the closing bracket } } if (containsNull) { // Close the OR builder.Append(')'); } return builder; } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { Slot.ToCompactString(builder); builder.Append(" IN ("); StringUtil.ToCommaSeparatedStringSorted(builder, Values.Values); builder.Append(")"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Linq; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; // A class that denotes the boolean expression: "scalarVar in values" // See the comments in OneOfConst for partially and fully-done objects internal class OneOfScalarConst : OneOfConst { #region Constructors // effects: Creates an expression of the form "node in scalar strings // corresponding to values". This constructor is needed for creating // discriminator type conditions internal OneOfScalarConst(JoinTreeNode node, object value, TypeUsage memberType) : this(node, new ScalarConstant(value)) { // CHANGE_[....]_IMPROVE: the memberType stuff has to be consumed } internal OneOfScalarConst(JoinTreeNode node, CellConstant value) : base(new JoinTreeSlot(node), value) { Debug.Assert(value is ScalarConstant || value.IsNull() || value.IsNotNull(), "Scalar, NULL, or NOT NULL expected"); } // effects: Creates an expression of the form "var = value" internal OneOfScalarConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), values, possibleValues) { } // effects: Creates an expression of the form "slot in domain" internal OneOfScalarConst(JoinTreeSlot slot, CellConstantDomain domain) : base(slot, domain) { } #endregion #region Methods // requires: IsFullyDone is true // effects: Fixes the range of this in accordance with range internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(IsFullyDone, "Ranges are fixed only for fully done OneOfConsts"); IEnumerable newPossibleValues = memberDomainMap.GetDomain(Slot.MemberPath); BoolLiteral newLiteral = new OneOfScalarConst(Slot, new CellConstantDomain(range, newPossibleValues)); return newLiteral.GetDomainBoolExpression(memberDomainMap); } // requires: there is at most one negated Constant internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, canSkipIsNotNull, false); } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, canSkipIsNotNull, true); } //Common code for AsCQL and TouserString methods private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, bool canSkipIsNotNull, bool userString) { Debug.Assert(Slot.MemberPath.IsScalarType(), "Expected scalar"); Set constants = new Set (Values.Values, CellConstant.EqualityComparer); // * If no negated cell constant generate the normal list // * If nullable, add AND IS NOT NULL to the list of normal values // * If has a negated cell constant, come up with a simplified // version of the constants, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL NegatedCellConstant negated = null; foreach (CellConstant constant in constants) { NegatedCellConstant tmpConstant = constant as NegatedCellConstant; if (tmpConstant != null) { Debug.Assert(negated == null, "Multiple negated constants?"); negated = tmpConstant; } } if (negated != null) { if (userString) { return negated.AsUserString(builder, blockAlias, constants, Slot.MemberPath, canSkipIsNotNull); } else { return negated.AsCql(builder, blockAlias, constants, Slot.MemberPath, canSkipIsNotNull); } } // We have only positive constants bool containsNull = constants.Contains(CellConstant.Null); constants.Remove(CellConstant.Null); //Constraint counter-example could contain undefined cellconstant. E.g for booleans (for int its optimized out due to negated constants) //we want to treat undefined as nulls if (constants.Contains(CellConstant.Undefined)) { constants.Remove(CellConstant.Undefined); containsNull = true; } if (containsNull) { if (constants.Count > 0) { builder.Append('('); } if (userString) { Slot.MemberPath.ToCompactString(builder, blockAlias); builder.Append(" is NULL"); } else { Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(" IS NULL"); } if (constants.Count > 0) { builder.Append(" OR "); } } if (constants.Count == 0) { return builder; } bool isNullable = Slot.MemberPath.IsNullable; // Generate var IN {...} or var = ... // For nullable members, add (... AND var IS NOT NULL) // so the boolean _from variables never evaluate to null // This is needed because view generation assumes 2-valued boolean logic if (isNullable && canSkipIsNotNull == false) { builder.Append("("); // enclose AND var IS NOT NULL in brackets } if (userString) { Slot.MemberPath.ToCompactString(builder, blockAlias); } else { Slot.MemberPath.AsCql(builder, blockAlias); } if (constants.Count > 1) { // Multiple values builder.Append(" IN {"); bool isFirst = true; foreach (CellConstant constant in constants) { if (isFirst == false) { builder.Append(", "); } isFirst = false; if (userString) { constant.ToCompactString(builder); } else { constant.AsCql(builder, Slot.MemberPath, blockAlias); } } builder.Append("}"); } else { // Single value builder.Append(" = "); if (userString) { constants.Single().ToCompactString(builder); } else { constants.Single().AsCql(builder, Slot.MemberPath, blockAlias); } } if (isNullable && canSkipIsNotNull == false) { // add the AND var IS NOT NULL condition builder.Append(" AND "); if (userString) { Slot.MemberPath.ToCompactString(builder, Strings.ViewGen_EntityInstanceToken); builder.Append(" is not NULL)"); // plus the closing bracket } else { Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL)"); // plus the closing bracket } } if (containsNull) { // Close the OR builder.Append(')'); } return builder; } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { Slot.ToCompactString(builder); builder.Append(" IN ("); StringUtil.ToCommaSeparatedStringSorted(builder, Values.Values); builder.Append(")"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
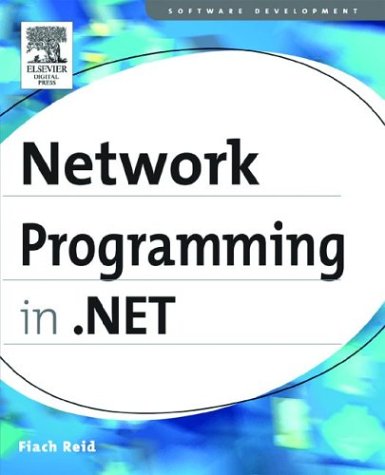
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HiddenFieldPageStatePersister.cs
- ThreadInterruptedException.cs
- RadioButtonAutomationPeer.cs
- NullNotAllowedCollection.cs
- MenuCommands.cs
- LinkedResourceCollection.cs
- Slider.cs
- InstancePersistenceContext.cs
- FtpWebResponse.cs
- FrameworkElement.cs
- PriorityChain.cs
- BackStopAuthenticationModule.cs
- TransactionWaitAsyncResult.cs
- TableStyle.cs
- DataGridViewColumnConverter.cs
- NativeMethods.cs
- CodeDomLocalizationProvider.cs
- ClientSettings.cs
- Rfc2898DeriveBytes.cs
- SocketPermission.cs
- DynamicExpression.cs
- MulticastNotSupportedException.cs
- XmlAttributeHolder.cs
- TimeEnumHelper.cs
- FileLogRecord.cs
- GlyphElement.cs
- NativeRecognizer.cs
- XsltOutput.cs
- ProfileManager.cs
- CharacterShapingProperties.cs
- SignatureToken.cs
- HttpException.cs
- AttributeQuery.cs
- InternalResources.cs
- FilterElement.cs
- FieldDescriptor.cs
- XPathNodeIterator.cs
- HostVisual.cs
- DocumentScope.cs
- metadatamappinghashervisitor.cs
- SizeF.cs
- XmlCharacterData.cs
- XamlTreeBuilder.cs
- WindowInteractionStateTracker.cs
- PriorityChain.cs
- CertificateManager.cs
- ScrollableControl.cs
- HandleCollector.cs
- ClientBuildManager.cs
- TrailingSpaceComparer.cs
- CompleteWizardStep.cs
- FileIOPermission.cs
- RandomNumberGenerator.cs
- PowerModeChangedEventArgs.cs
- HttpConfigurationContext.cs
- TextDecorationLocationValidation.cs
- DuplexClientBase.cs
- HttpModuleActionCollection.cs
- RegionIterator.cs
- ProfileServiceManager.cs
- DefaultParameterValueAttribute.cs
- EnumBuilder.cs
- XNodeNavigator.cs
- PartBasedPackageProperties.cs
- TableLayoutColumnStyleCollection.cs
- MediaCommands.cs
- WebBrowser.cs
- SiteIdentityPermission.cs
- PermissionRequestEvidence.cs
- TypeConverterValueSerializer.cs
- ContextQuery.cs
- XmlSchemaComplexContentRestriction.cs
- CodeExpressionCollection.cs
- ChangeDirector.cs
- WindowsGraphicsCacheManager.cs
- DiscreteKeyFrames.cs
- CallbackValidator.cs
- Internal.cs
- MarkupExtensionParser.cs
- SelectedDatesCollection.cs
- TextElementEnumerator.cs
- MasterPageBuildProvider.cs
- brushes.cs
- DiscardableAttribute.cs
- HttpWriter.cs
- BlockUIContainer.cs
- DbModificationCommandTree.cs
- CDSsyncETWBCLProvider.cs
- MultiPartWriter.cs
- ObjectCloneHelper.cs
- AsyncOperation.cs
- QilPatternFactory.cs
- DictionaryItemsCollection.cs
- ExtendedPropertiesHandler.cs
- Range.cs
- DateTime.cs
- RequestCachePolicy.cs
- BehaviorService.cs
- PageFunction.cs
- Currency.cs