Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Reflection / Emit / LocalBuilder.cs / 1 / LocalBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; namespace System.Reflection.Emit { [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_LocalBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public sealed class LocalBuilder : LocalVariableInfo, _LocalBuilder { #region Private Data Members private int m_localIndex; private Type m_localType; private MethodInfo m_methodBuilder; private bool m_isPinned; #endregion #region Constructor private LocalBuilder() { } internal LocalBuilder(int localIndex, Type localType, MethodInfo methodBuilder) : this(localIndex, localType, methodBuilder, false) { } internal LocalBuilder(int localIndex, Type localType, MethodInfo methodBuilder, bool isPinned) { m_isPinned = isPinned; m_localIndex = localIndex; m_localType = localType; m_methodBuilder = methodBuilder; } #endregion #region Internal Members internal int GetLocalIndex() { return m_localIndex; } internal MethodInfo GetMethodBuilder() { return m_methodBuilder; } #endregion #region LocalVariableInfo Override public override bool IsPinned { get { return m_isPinned; } } public override Type LocalType { get { return m_localType; } } public override int LocalIndex { get { return m_localIndex; } } #endregion #region Public Members public void SetLocalSymInfo(String name) { SetLocalSymInfo(name, 0, 0); } public void SetLocalSymInfo(String name, int startOffset, int endOffset) { ModuleBuilder dynMod; SignatureHelper sigHelp; int sigLength; byte[] signature; byte[] mungedSig; int index; MethodBuilder methodBuilder = m_methodBuilder as MethodBuilder; if (methodBuilder == null) // it's a light code gen entity throw new NotSupportedException(); dynMod = (ModuleBuilder) methodBuilder.Module; if (methodBuilder.IsTypeCreated()) { // cannot change method after its containing type has been created throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_TypeHasBeenCreated")); } // set the name and range of offset for the local if (dynMod.GetSymWriter() == null) { // cannot set local name if not debug module throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_NotADebugModule")); } sigHelp = SignatureHelper.GetFieldSigHelper(dynMod); sigHelp.AddArgument(m_localType); signature = sigHelp.InternalGetSignature(out sigLength); // The symbol store doesn't want the calling convention on the // front of the signature, but InternalGetSignature returns // the callinging convention. So we strip it off. This is a // bit unfortunate, since it means that we need to allocate // yet another array of bytes... mungedSig = new byte[sigLength - 1]; Array.Copy(signature, 1, mungedSig, 0, sigLength - 1); index = methodBuilder.GetILGenerator().m_ScopeTree.GetCurrentActiveScopeIndex(); if (index == -1) { // top level scope information is kept with methodBuilder methodBuilder.m_localSymInfo.AddLocalSymInfo( name, mungedSig, m_localIndex, startOffset, endOffset); } else { methodBuilder.GetILGenerator().m_ScopeTree.AddLocalSymInfoToCurrentScope( name, mungedSig, m_localIndex, startOffset, endOffset); } } #endregion void _LocalBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _LocalBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _LocalBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _LocalBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
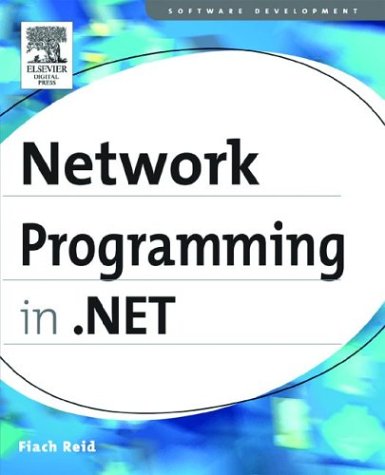
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DictionaryCustomTypeDescriptor.cs
- PeerTransportCredentialType.cs
- ContainerParaClient.cs
- CryptoProvider.cs
- _IPv6Address.cs
- AppDomainAttributes.cs
- Control.cs
- TripleDESCryptoServiceProvider.cs
- FreezableDefaultValueFactory.cs
- SafeUserTokenHandle.cs
- contentDescriptor.cs
- HwndSourceKeyboardInputSite.cs
- httpstaticobjectscollection.cs
- WebPartUserCapability.cs
- FileFormatException.cs
- VirtualPathUtility.cs
- Single.cs
- ConfigurationErrorsException.cs
- LocalizedNameDescriptionPair.cs
- LocatorPart.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- TimeoutException.cs
- ChangeDirector.cs
- TreeViewEvent.cs
- FontUnitConverter.cs
- RunWorkerCompletedEventArgs.cs
- BindingNavigator.cs
- ServicesExceptionNotHandledEventArgs.cs
- EntityDataSourceDesignerHelper.cs
- GridViewSortEventArgs.cs
- Int16KeyFrameCollection.cs
- BuildResult.cs
- Evidence.cs
- TextSelection.cs
- Span.cs
- MembershipUser.cs
- Selection.cs
- NativeWindow.cs
- DataServiceExpressionVisitor.cs
- TextSpanModifier.cs
- SystemTcpConnection.cs
- FormViewDeletedEventArgs.cs
- ToolStripDropDownMenu.cs
- ParserOptions.cs
- ReflectionServiceProvider.cs
- WebPartsPersonalization.cs
- WebPartCatalogCloseVerb.cs
- RowSpanVector.cs
- AnimationException.cs
- ObjectAssociationEndMapping.cs
- PortCache.cs
- StrongNameUtility.cs
- HybridObjectCache.cs
- ReachFixedPageSerializer.cs
- Literal.cs
- XamlPathDataSerializer.cs
- Timer.cs
- HostingPreferredMapPath.cs
- AccessKeyManager.cs
- PeerSecurityHelpers.cs
- recordstatescratchpad.cs
- DataAdapter.cs
- IgnoreSectionHandler.cs
- InvokePattern.cs
- Int32Collection.cs
- XmlWrappingReader.cs
- Ipv6Element.cs
- CounterCreationData.cs
- HtmlImage.cs
- ItemsPanelTemplate.cs
- SafeEventHandle.cs
- BehaviorEditorPart.cs
- DescendentsWalker.cs
- PerformanceCounterPermission.cs
- Interlocked.cs
- DiagnosticsConfiguration.cs
- ModelItemCollection.cs
- DataGridCell.cs
- ObjectListSelectEventArgs.cs
- SchemaDeclBase.cs
- IArgumentProvider.cs
- SHA1Managed.cs
- PasswordDeriveBytes.cs
- SendAgentStatusRequest.cs
- HeaderCollection.cs
- XmlAnyElementAttribute.cs
- TrustManager.cs
- DataTableClearEvent.cs
- HttpCookiesSection.cs
- DataGridViewButtonColumn.cs
- PropertyInfo.cs
- StylusButtonEventArgs.cs
- TextTreeRootNode.cs
- LabelLiteral.cs
- MemberNameValidator.cs
- DynamicRenderer.cs
- HttpCachePolicy.cs
- BinHexEncoding.cs
- SettingsPropertyCollection.cs
- SQLGuidStorage.cs