Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Currency.cs / 1 / Currency.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System { using System; using System.Globalization; using System.Runtime.CompilerServices; [Serializable] internal struct Currency { internal long m_value; // Constructs a Currency from a Decimal value. // public Currency(Decimal value) { m_value = Decimal.ToCurrency(value).m_value; } // Constructs a Currency from a long value without scaling. The // ignored parameter exists only to distinguish this constructor // from the constructor that takes a long. Used only in the System // package, especially in Variant. internal Currency(long value, int ignored) { m_value = value; } // Creates a Currency from an OLE Automation Currency. This method // applies no scaling to the Currency value, essentially doing a bitwise // copy. // public static Currency FromOACurrency(long cy){ return new Currency(cy, 0); } //Creates an OLE Automation Currency from a Currency instance. This // method applies no scaling to the Currency value, essentially doing // a bitwise copy. // public long ToOACurrency() { return m_value; } // Converts a Currency to a Decimal. // public static Decimal ToDecimal(Currency c) { Decimal result = new Decimal (); FCallToDecimal (ref result, c); return result; } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern void FCallToDecimal(ref Decimal result,Currency c); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
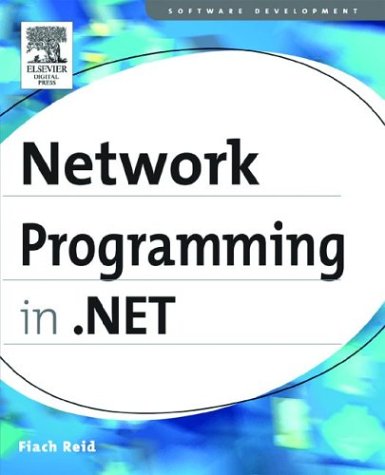
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOExtraField.cs
- DataGridHeaderBorder.cs
- ScriptManager.cs
- ParameterElement.cs
- XmlAttributeProperties.cs
- TransactionFlowElement.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- RenderData.cs
- EdmToObjectNamespaceMap.cs
- SqlTypesSchemaImporter.cs
- NotImplementedException.cs
- TextDecorations.cs
- EdmValidator.cs
- HtmlElement.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- MobileSysDescriptionAttribute.cs
- WebPartZoneCollection.cs
- DynamicValidatorEventArgs.cs
- EventRoute.cs
- TreeNodeCollection.cs
- ParseElement.cs
- KeyValuePairs.cs
- PKCS1MaskGenerationMethod.cs
- XPathNodeList.cs
- TextElementCollectionHelper.cs
- OleDbConnectionFactory.cs
- Line.cs
- BaseCodeDomTreeGenerator.cs
- MetadataArtifactLoaderComposite.cs
- SmiContext.cs
- WeakReferenceList.cs
- ButtonChrome.cs
- DataConnectionHelper.cs
- TextUtf8RawTextWriter.cs
- PeerValidationBehavior.cs
- WorkflowQueueInfo.cs
- GeneralTransform2DTo3DTo2D.cs
- LineBreakRecord.cs
- VirtualizingStackPanel.cs
- InstanceDataCollectionCollection.cs
- MeshGeometry3D.cs
- MsdtcClusterUtils.cs
- ThemeInfoAttribute.cs
- Properties.cs
- HebrewNumber.cs
- AmbientProperties.cs
- DbConnectionStringBuilder.cs
- ScrollData.cs
- DragStartedEventArgs.cs
- XmlDocumentFragment.cs
- arclist.cs
- QilPatternVisitor.cs
- CacheManager.cs
- HwndMouseInputProvider.cs
- ResetableIterator.cs
- Listen.cs
- DocumentScope.cs
- DataGridPagerStyle.cs
- XmlMembersMapping.cs
- SqlTypeConverter.cs
- EntityDataSourceColumn.cs
- CodeBinaryOperatorExpression.cs
- ValidationRuleCollection.cs
- ClientSideQueueItem.cs
- SQLRoleProvider.cs
- __Filters.cs
- CharacterShapingProperties.cs
- ThicknessAnimationUsingKeyFrames.cs
- DbReferenceCollection.cs
- EventDrivenDesigner.cs
- VirtualPath.cs
- _NegoStream.cs
- CounterSample.cs
- XmlParserContext.cs
- TreeChangeInfo.cs
- OleStrCAMarshaler.cs
- XmlDeclaration.cs
- DataContractAttribute.cs
- String.cs
- EnumConverter.cs
- DictionaryManager.cs
- UriTemplateQueryValue.cs
- DataTableMapping.cs
- CodeSnippetCompileUnit.cs
- FullTrustAssembly.cs
- PermissionToken.cs
- DesignRelationCollection.cs
- ToolStripTextBox.cs
- UInt32.cs
- EntryWrittenEventArgs.cs
- entityreference_tresulttype.cs
- AttributeQuery.cs
- KnownBoxes.cs
- CountAggregationOperator.cs
- XamlToRtfWriter.cs
- SearchExpression.cs
- LocalizableResourceBuilder.cs
- BinaryConverter.cs
- GregorianCalendarHelper.cs
- RealizationContext.cs