Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / TransformerInfoCollection.cs / 3 / TransformerInfoCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
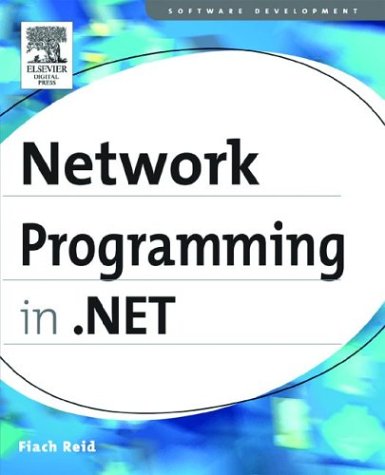
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentXmlWriter.cs
- TextRangeProviderWrapper.cs
- RunInstallerAttribute.cs
- ObjectKeyFrameCollection.cs
- ProxyWebPartConnectionCollection.cs
- TaskHelper.cs
- Variable.cs
- ModuleBuilderData.cs
- SlotInfo.cs
- ScrollData.cs
- SQLDecimal.cs
- TaskScheduler.cs
- EmissiveMaterial.cs
- WebPartDisplayMode.cs
- DbDataRecord.cs
- CorrelationTokenInvalidatedHandler.cs
- DockAndAnchorLayout.cs
- EmissiveMaterial.cs
- GreaterThan.cs
- HtmlInputHidden.cs
- IntranetCredentialPolicy.cs
- ListBoxItemWrapperAutomationPeer.cs
- WebPartConnectionsEventArgs.cs
- TextServicesCompartmentContext.cs
- Stack.cs
- HostUtils.cs
- TextParentUndoUnit.cs
- HashRepartitionStream.cs
- DataGridViewLayoutData.cs
- ImageSource.cs
- TaskExceptionHolder.cs
- XmlTypeAttribute.cs
- Formatter.cs
- RadioButton.cs
- ComponentDispatcherThread.cs
- TaiwanLunisolarCalendar.cs
- ScaleTransform3D.cs
- AutomationPeer.cs
- DesignTable.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ParameterReplacerVisitor.cs
- SecurityContextSecurityTokenAuthenticator.cs
- NameTable.cs
- TrayIconDesigner.cs
- IIS7WorkerRequest.cs
- Token.cs
- CubicEase.cs
- WizardForm.cs
- DocumentPageTextView.cs
- CodeAssignStatement.cs
- TransformConverter.cs
- RequestCachePolicyConverter.cs
- ParallelLoopState.cs
- WorkflowItemPresenter.cs
- HideDisabledControlAdapter.cs
- ReaderOutput.cs
- DashStyle.cs
- RectIndependentAnimationStorage.cs
- CodeAttachEventStatement.cs
- DBSqlParserColumnCollection.cs
- DeclarativeCatalogPart.cs
- ExceptionUtil.cs
- InternalBufferOverflowException.cs
- EntityDesignerUtils.cs
- DocumentSchemaValidator.cs
- TemplateKeyConverter.cs
- XPathNodeInfoAtom.cs
- OutputCacheSection.cs
- SqlBulkCopyColumnMappingCollection.cs
- SettingsPropertyWrongTypeException.cs
- PropertyBuilder.cs
- SchemaTableColumn.cs
- ErrorsHelper.cs
- OraclePermission.cs
- DataGridViewRowsRemovedEventArgs.cs
- ECDiffieHellmanPublicKey.cs
- ByteKeyFrameCollection.cs
- ByteStorage.cs
- WebHttpSecurityModeHelper.cs
- ImageKeyConverter.cs
- HttpServerUtilityBase.cs
- DuplicateWaitObjectException.cs
- XmlDataDocument.cs
- ProxyWebPart.cs
- DbConnectionPool.cs
- MouseActionConverter.cs
- ExpressionBuilderContext.cs
- EllipseGeometry.cs
- DataObjectPastingEventArgs.cs
- SRef.cs
- TimeSpanStorage.cs
- EdmTypeAttribute.cs
- Image.cs
- LabelLiteral.cs
- DataKeyCollection.cs
- XmlUtil.cs
- PresentationAppDomainManager.cs
- ModelItem.cs
- RadioButtonAutomationPeer.cs
- ContextMenu.cs