Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / BufferAllocator.cs / 1 / BufferAllocator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Buffer Allocators with recycling * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Collections; using System.IO; using System.Globalization; using System.Web.Util; ////////////////////////////////////////////////////////////////////////////// // Generic buffer recycling /* * Base class for allocator doing buffer recycling */ internal abstract class BufferAllocator { private int _maxFree; private int _numFree; private Stack _buffers; private static int s_ProcsFudgeFactor; static BufferAllocator() { s_ProcsFudgeFactor = SystemInfo.GetNumProcessCPUs(); if (s_ProcsFudgeFactor < 1) s_ProcsFudgeFactor = 1; if (s_ProcsFudgeFactor > 4) s_ProcsFudgeFactor = 4; } internal BufferAllocator(int maxFree) { _buffers = new Stack(); _numFree = 0; _maxFree = maxFree * s_ProcsFudgeFactor; } internal /*public*/ Object GetBuffer() { Object buffer = null; if (_numFree > 0) { lock(this) { if (_numFree > 0) { buffer = _buffers.Pop(); _numFree--; } } } if (buffer == null) buffer = AllocBuffer(); return buffer; } internal void ReuseBuffer(Object buffer) { if (_numFree < _maxFree) { lock(this) { if (_numFree < _maxFree) { _buffers.Push(buffer); _numFree++; } } } } /* * To be implemented by a derived class */ abstract protected Object AllocBuffer(); } /* * Concrete allocator class for ubyte[] buffer recycling */ internal class UbyteBufferAllocator : BufferAllocator { private int _bufferSize; internal UbyteBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new byte[_bufferSize]; } } /* * Concrete allocator class for char[] buffer recycling */ internal class CharBufferAllocator : BufferAllocator { private int _bufferSize; internal CharBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new char[_bufferSize]; } } /* * Concrete allocator class for int[] buffer recycling */ internal class IntegerArrayAllocator : BufferAllocator { private int _arraySize; internal IntegerArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new int[_arraySize]; } } /* * Concrete allocator class for IntPtr[] buffer recycling */ internal class IntPtrArrayAllocator : BufferAllocator { private int _arraySize; internal IntPtrArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new IntPtr[_arraySize]; } } }
Link Menu
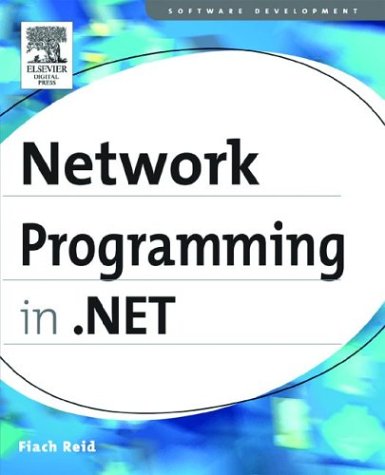
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImageFormat.cs
- IPipelineRuntime.cs
- BindingSourceDesigner.cs
- MenuItem.cs
- RectangleConverter.cs
- IntPtr.cs
- MsmqIntegrationChannelFactory.cs
- DrawingAttributesDefaultValueFactory.cs
- TextProviderWrapper.cs
- ModulesEntry.cs
- SendKeys.cs
- FindResponse.cs
- ToolStripLabel.cs
- CombinedGeometry.cs
- ObjectContextServiceProvider.cs
- EncryptedData.cs
- OptimalTextSource.cs
- JulianCalendar.cs
- ServiceObjectContainer.cs
- TrimSurroundingWhitespaceAttribute.cs
- ExtensionSimplifierMarkupObject.cs
- WebPartTracker.cs
- Resources.Designer.cs
- PageAdapter.cs
- WebCategoryAttribute.cs
- Ray3DHitTestResult.cs
- HttpHostedTransportConfiguration.cs
- SoapServerMethod.cs
- RenderData.cs
- ResourceReader.cs
- SQlBooleanStorage.cs
- HttpWrapper.cs
- DBSqlParserTableCollection.cs
- PropertyIDSet.cs
- XPathNode.cs
- FullTextState.cs
- MappingModelBuildProvider.cs
- OdbcTransaction.cs
- Shape.cs
- FilterRepeater.cs
- WebPartMenu.cs
- Sequence.cs
- QfeChecker.cs
- VisualStyleTypesAndProperties.cs
- ToolBar.cs
- TypeSemantics.cs
- ChannelTerminatedException.cs
- NativeMethods.cs
- IResourceProvider.cs
- CreateUserWizardAutoFormat.cs
- ListDictionary.cs
- SecureConversationDriver.cs
- OutputCacheProfileCollection.cs
- X509KeyIdentifierClauseType.cs
- SqlCharStream.cs
- DynamicDataRoute.cs
- EntityContainer.cs
- LoginName.cs
- VisualStyleTypesAndProperties.cs
- TransactionContextManager.cs
- HttpWrapper.cs
- DrawingBrush.cs
- TemplateField.cs
- DataRowCollection.cs
- MenuItem.cs
- securestring.cs
- ByteConverter.cs
- TriggerBase.cs
- TimersDescriptionAttribute.cs
- Propagator.ExtentPlaceholderCreator.cs
- AuthenticationConfig.cs
- DbConnectionPoolOptions.cs
- FixedSOMSemanticBox.cs
- Simplifier.cs
- Odbc32.cs
- ConfigurationSettings.cs
- ColorPalette.cs
- OleDbInfoMessageEvent.cs
- StringFunctions.cs
- AdornerDecorator.cs
- DependsOnAttribute.cs
- NamespaceInfo.cs
- TabControlEvent.cs
- IdentityReference.cs
- ObjectAnimationUsingKeyFrames.cs
- GridViewCancelEditEventArgs.cs
- TextServicesManager.cs
- StructureChangedEventArgs.cs
- TextParagraph.cs
- HtmlInputSubmit.cs
- MsmqTransportSecurityElement.cs
- BamlTreeUpdater.cs
- HtmlElement.cs
- XmlSignatureProperties.cs
- basemetadatamappingvisitor.cs
- ConfigXmlWhitespace.cs
- SQLInt16Storage.cs
- DataControlImageButton.cs
- ConstraintStruct.cs
- MultiViewDesigner.cs