Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewInsertionMark.cs / 1 / ListViewInsertionMark.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Drawing; using System.Runtime.InteropServices; using System.Diagnostics; namespace System.Windows.Forms { ////// /// public sealed class ListViewInsertionMark { private ListView listView; private int index = 0; private Color color = Color.Empty; private bool appearsAfterItem = false; internal ListViewInsertionMark(ListView listView) { this.listView = listView; } ////// Encapsulates insertion-mark information /// ////// /// Specifies whether the insertion mark appears /// after the item - otherwise it appears /// before the item (the default). /// /// public bool AppearsAfterItem { get { return appearsAfterItem; } set { if (appearsAfterItem != value) { appearsAfterItem = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Returns bounds of the insertion-mark. /// /// public Rectangle Bounds { get { NativeMethods.RECT rect = new NativeMethods.RECT(); listView.SendMessage(NativeMethods.LVM_GETINSERTMARKRECT, 0, ref rect); return Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom); } } ////// /// The color of the insertion-mark. /// /// public Color Color { get { if (color.IsEmpty) { color = SafeNativeMethods.ColorFromCOLORREF((int)listView.SendMessage(NativeMethods.LVM_GETINSERTMARKCOLOR, 0, 0)); } return color; } set { if (color != value) { color = value; if (listView.IsHandleCreated) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } ////// /// Item next to which the insertion-mark appears. /// /// public int Index { get { return index; } set { if (index != value) { index = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Performs a hit-test at the specified insertion point /// and returns the closest item. /// /// public int NearestIndex(Point pt) { NativeMethods.POINT point = new NativeMethods.POINT(); point.x = pt.X; point.y = pt.Y; NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_INSERTMARKHITTEST, point, lvInsertMark); return lvInsertMark.iItem; } internal void UpdateListView() { Debug.Assert(listView.IsHandleCreated, "ApplySavedState Precondition: List-view handle must be created"); NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); lvInsertMark.dwFlags = appearsAfterItem ? NativeMethods.LVIM_AFTER : 0; lvInsertMark.iItem = index; UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_SETINSERTMARK, 0, lvInsertMark); if (!color.IsEmpty) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
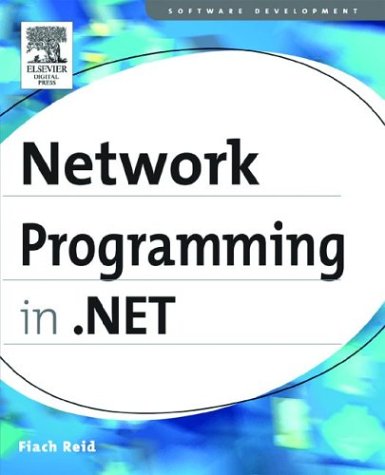
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- sitestring.cs
- HttpPostedFile.cs
- ExceptionRoutedEventArgs.cs
- SQLCharsStorage.cs
- DataGridViewCellStyleChangedEventArgs.cs
- DataBinding.cs
- GeneralTransform3DGroup.cs
- LinearKeyFrames.cs
- WebPartConnectionCollection.cs
- GroupBox.cs
- ThreadAttributes.cs
- TextRange.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- TableCell.cs
- BuildResult.cs
- CompModSwitches.cs
- TypeListConverter.cs
- ContainerFilterService.cs
- LinqDataSourceContextEventArgs.cs
- BooleanConverter.cs
- CultureData.cs
- SerializationFieldInfo.cs
- XmlSchemaValidationException.cs
- SortQuery.cs
- TreeViewCancelEvent.cs
- TableRow.cs
- AddressHeaderCollection.cs
- DragDrop.cs
- TrustSection.cs
- VectorAnimation.cs
- SymbolDocumentInfo.cs
- SchemaImporter.cs
- OleDbRowUpdatingEvent.cs
- NonSerializedAttribute.cs
- ObjectMemberMapping.cs
- Int32AnimationBase.cs
- ObjectViewFactory.cs
- MetadataCacheItem.cs
- PropertyItem.cs
- XhtmlConformanceSection.cs
- WebBrowser.cs
- SoapFault.cs
- LocalizationComments.cs
- ListManagerBindingsCollection.cs
- MexNamedPipeBindingCollectionElement.cs
- OSFeature.cs
- DataGridViewCellMouseEventArgs.cs
- SectionVisual.cs
- BeginStoryboard.cs
- ProfileSettingsCollection.cs
- CharacterMetrics.cs
- RC2.cs
- UriParserTemplates.cs
- ToolStripScrollButton.cs
- Automation.cs
- KerberosSecurityTokenAuthenticator.cs
- FaultFormatter.cs
- ReliabilityContractAttribute.cs
- OrthographicCamera.cs
- EventProviderWriter.cs
- XmlCodeExporter.cs
- BuildProvider.cs
- NameValuePermission.cs
- PropertyPathWorker.cs
- XPathBinder.cs
- MessagePropertyDescription.cs
- WebZoneDesigner.cs
- SplitterPanel.cs
- Literal.cs
- StylusButtonCollection.cs
- ManagedWndProcTracker.cs
- IndentedWriter.cs
- XmlException.cs
- DES.cs
- MouseButtonEventArgs.cs
- EventLogTraceListener.cs
- ObjectViewEntityCollectionData.cs
- TrackingQuery.cs
- iisPickupDirectory.cs
- DrawingGroup.cs
- TabControlCancelEvent.cs
- MetadataItem_Static.cs
- FixedDocumentPaginator.cs
- StandardToolWindows.cs
- AxHost.cs
- HttpServerUtilityWrapper.cs
- BasicKeyConstraint.cs
- NumberFormatter.cs
- RepeatButton.cs
- TreePrinter.cs
- EntityContainer.cs
- OracleRowUpdatingEventArgs.cs
- ToolStripItemImageRenderEventArgs.cs
- WindowsGrip.cs
- FileDialogPermission.cs
- VisualBrush.cs
- BitmapEffectCollection.cs
- DictionarySectionHandler.cs
- InvalidOperationException.cs
- CatalogZoneBase.cs