Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / BindingMAnagerBase.cs / 1 / BindingMAnagerBase.cs
namespace System.Windows.Forms { using System; using System.Windows.Forms; using System.ComponentModel; using System.Collections; using System.Diagnostics.CodeAnalysis; ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors")] // Shipped in Everett public abstract class BindingManagerBase { private BindingsCollection bindings; private bool pullingData = false; ///[To be supplied.] ////// /// protected EventHandler onCurrentChangedHandler; ///[To be supplied.] ////// /// protected EventHandler onPositionChangedHandler; // Hook BindingComplete events on all owned Binding objects, and propagate those events through our own BindingComplete event private BindingCompleteEventHandler onBindingCompleteHandler = null; // same deal about the new currentItemChanged event internal EventHandler onCurrentItemChangedHandler; // Event handler for the DataError event internal BindingManagerDataErrorEventHandler onDataErrorHandler; ///[To be supplied.] ////// /// public BindingsCollection Bindings { get { if (bindings == null) { bindings = new ListManagerBindingsCollection(this); // Hook collection change events on collection, so we can hook or unhook the BindingComplete events on individual bindings bindings.CollectionChanging += new CollectionChangeEventHandler(OnBindingsCollectionChanging); bindings.CollectionChanged += new CollectionChangeEventHandler(OnBindingsCollectionChanged); } return bindings; } } ///[To be supplied.] ////// /// internal protected void OnBindingComplete(BindingCompleteEventArgs args) { if (onBindingCompleteHandler != null) { onBindingCompleteHandler(this, args); } } ///[To be supplied.] ////// /// internal protected abstract void OnCurrentChanged(EventArgs e); ///[To be supplied.] ////// /// internal protected abstract void OnCurrentItemChanged(EventArgs e); ///[To be supplied.] ////// /// internal protected void OnDataError(Exception e) { if (onDataErrorHandler != null) { onDataErrorHandler(this, new BindingManagerDataErrorEventArgs(e)); } } ///[To be supplied.] ////// /// public abstract Object Current { get; } internal abstract void SetDataSource(Object dataSource); ///[To be supplied.] ////// /// public BindingManagerBase() { } [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not call SetDataSource // it would be a breaking change. ] internal BindingManagerBase(Object dataSource) { this.SetDataSource(dataSource); } internal abstract Type BindType{ get; } internal abstract PropertyDescriptorCollection GetItemProperties(PropertyDescriptor[] listAccessors); ///[To be supplied.] ////// /// public virtual PropertyDescriptorCollection GetItemProperties() { return GetItemProperties(null); } ///[To be supplied.] ///protected internal virtual PropertyDescriptorCollection GetItemProperties(ArrayList dataSources, ArrayList listAccessors) { IList list = null; if (this is CurrencyManager) { list = ((CurrencyManager)this).List; } if (list is ITypedList) { PropertyDescriptor[] properties = new PropertyDescriptor[listAccessors.Count]; listAccessors.CopyTo(properties, 0); return ((ITypedList)list).GetItemProperties(properties); } return this.GetItemProperties(this.BindType, 0, dataSources, listAccessors); } // listType is the type of the top list in the list.list.list.list reference // offset is how far we are in the listAccessors // listAccessors is the list of accessors (duh) // /// protected virtual PropertyDescriptorCollection GetItemProperties(Type listType, int offset, ArrayList dataSources, ArrayList listAccessors) { if (listAccessors.Count < offset) return null; if (listAccessors.Count == offset) { if (typeof(IList).IsAssignableFrom(listType)) { System.Reflection.PropertyInfo[] itemProps = listType.GetProperties(); // PropertyDescriptorCollection itemProps = TypeDescriptor.GetProperties(listType); for (int i = 0; i < itemProps.Length; i ++) { if ("Item".Equals(itemProps[i].Name) && itemProps[i].PropertyType != typeof(object)) return TypeDescriptor.GetProperties(itemProps[i].PropertyType, new Attribute[] {new BrowsableAttribute(true)}); } // return the properties on the type of the first element in the list IList list = dataSources[offset - 1] as IList; if (list != null && list.Count > 0) return TypeDescriptor.GetProperties(list[0]); } else { return TypeDescriptor.GetProperties(listType); } return null; } System.Reflection.PropertyInfo[] props = listType.GetProperties(); // PropertyDescriptorCollection props = TypeDescriptor.GetProperties(listType); if (typeof(IList).IsAssignableFrom(listType)) { PropertyDescriptorCollection itemProps = null; for (int i = 0; i < props.Length; i++) { if ("Item".Equals(props[i].Name) && props[i].PropertyType != typeof(object)) { // get all the properties that are not marked as Browsable(false) // itemProps = TypeDescriptor.GetProperties(props[i].PropertyType, new Attribute[] {new BrowsableAttribute(true)}); } } if (itemProps == null) { // use the properties on the type of the first element in the list // if offset == 0, then this means that the first dataSource did not have a strongly typed Item property. // the dataSources are added only for relatedCurrencyManagers, so in this particular case // we need to use the dataSource in the currencyManager. See ASURT 83035. IList list; if (offset == 0) list = this.DataSource as IList; else list = dataSources[offset - 1] as IList; if (list != null && list.Count > 0) { itemProps = TypeDescriptor.GetProperties(list[0]); } } if (itemProps != null) { for (int j=0; j /// /// public event BindingCompleteEventHandler BindingComplete { add { onBindingCompleteHandler += value; } remove { onBindingCompleteHandler -= value; } } ///[To be supplied.] ////// /// public event EventHandler CurrentChanged { add { onCurrentChangedHandler += value; } remove { onCurrentChangedHandler -= value; } } ///[To be supplied.] ////// /// public event EventHandler CurrentItemChanged { add { onCurrentItemChangedHandler += value; } remove { onCurrentItemChangedHandler -= value; } } ///[To be supplied.] ////// /// public event BindingManagerDataErrorEventHandler DataError { add { onDataErrorHandler += value; } remove { onDataErrorHandler -= value; } } internal abstract String GetListName(); ///[To be supplied.] ////// /// public abstract void CancelCurrentEdit(); ///[To be supplied.] ////// /// public abstract void EndCurrentEdit(); ///[To be supplied.] ////// /// public abstract void AddNew(); ///[To be supplied.] ////// /// public abstract void RemoveAt(int index); ///[To be supplied.] ////// /// public abstract int Position{get; set;} ///[To be supplied.] ////// /// public event EventHandler PositionChanged { add { this.onPositionChangedHandler += value; } remove { this.onPositionChangedHandler -= value; } } ///[To be supplied.] ////// /// protected abstract void UpdateIsBinding(); ///[To be supplied.] ////// /// protected internal abstract String GetListName(ArrayList listAccessors); ///[To be supplied.] ///public abstract void SuspendBinding(); /// public abstract void ResumeBinding(); /// /// /// protected void PullData() { bool success; PullData(out success); } ///[To be supplied.] ////// /// internal void PullData(out bool success) { success = true; pullingData = true; try { UpdateIsBinding(); int numLinks = Bindings.Count; for (int i = 0; i < numLinks; i++) { if (Bindings[i].PullData()) { success = false; } } } finally { pullingData = false; } } ///[To be supplied.] ////// /// protected void PushData() { bool success; PushData(out success); } ///[To be supplied.] ////// /// internal void PushData(out bool success) { success = true; if (pullingData) return; UpdateIsBinding(); int numLinks = Bindings.Count; for (int i = 0; i < numLinks; i++) { if (Bindings[i].PushData()) { success = false; } } } internal abstract object DataSource { get; } internal abstract bool IsBinding { get; } ///[To be supplied.] ////// /// public bool IsBindingSuspended { get { return !IsBinding; } } /// public abstract int Count { get; } // BindingComplete events on individual Bindings are propagated up through the BindingComplete event on // the owning BindingManagerBase. To do this, we have to track changes to the bindings collection, adding // or removing handlers on items in the collection as appropriate. // // For the Add and Remove cases, we hook the collection 'changed' event, and add or remove handler for // specific binding. // // For the Refresh case, we hook both the 'changing' and 'changed' events, removing handlers for all // items that were in the collection before the change, then adding handlers for whatever items are // in the collection after the change. // private void OnBindingsCollectionChanged(object sender, CollectionChangeEventArgs e) { Binding b = e.Element as Binding; switch (e.Action) { case CollectionChangeAction.Add: b.BindingComplete += new BindingCompleteEventHandler(Binding_BindingComplete); break; case CollectionChangeAction.Remove: b.BindingComplete -= new BindingCompleteEventHandler(Binding_BindingComplete); break; case CollectionChangeAction.Refresh: foreach (Binding bi in bindings) { bi.BindingComplete += new BindingCompleteEventHandler(Binding_BindingComplete); } break; } } private void OnBindingsCollectionChanging(object sender, CollectionChangeEventArgs e) { if (e.Action == CollectionChangeAction.Refresh) { foreach (Binding bi in bindings) { bi.BindingComplete -= new BindingCompleteEventHandler(Binding_BindingComplete); } } } internal void Binding_BindingComplete(object sender, BindingCompleteEventArgs args) { this.OnBindingComplete(args); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
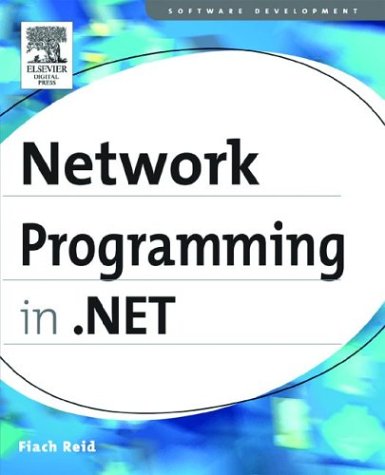
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseParaClient.cs
- HttpBrowserCapabilitiesBase.cs
- SerializerWriterEventHandlers.cs
- Matrix3DValueSerializer.cs
- nulltextcontainer.cs
- OdbcParameter.cs
- PeerMaintainer.cs
- Collection.cs
- ResizeGrip.cs
- XmlnsDefinitionAttribute.cs
- CollectionViewGroup.cs
- PictureBoxDesigner.cs
- MergePropertyDescriptor.cs
- CodeFieldReferenceExpression.cs
- SymbolMethod.cs
- ObjectItemAttributeAssemblyLoader.cs
- NamespaceEmitter.cs
- ConfigUtil.cs
- CollectionBuilder.cs
- WebPartDisplayModeEventArgs.cs
- RecognizedPhrase.cs
- PageThemeBuildProvider.cs
- WebConfigurationFileMap.cs
- TimeoutValidationAttribute.cs
- PageContentAsyncResult.cs
- ToolStripSplitStackLayout.cs
- InProcStateClientManager.cs
- XmlRootAttribute.cs
- GridToolTip.cs
- StorageComplexTypeMapping.cs
- RemotingSurrogateSelector.cs
- Highlights.cs
- Documentation.cs
- BamlRecordReader.cs
- EmbeddedObject.cs
- Perspective.cs
- SvcMapFileSerializer.cs
- XmlNamespaceDeclarationsAttribute.cs
- SrgsOneOf.cs
- PermissionRequestEvidence.cs
- CustomAttribute.cs
- ReadWriteObjectLock.cs
- OleDbDataReader.cs
- TabControlCancelEvent.cs
- ContainerCodeDomSerializer.cs
- TemplateControlParser.cs
- OleDbPropertySetGuid.cs
- MailHeaderInfo.cs
- X509SecurityToken.cs
- BaseCollection.cs
- PropertyChangingEventArgs.cs
- ColorContextHelper.cs
- Activator.cs
- DebugView.cs
- DesignerActionItem.cs
- PhysicalAddress.cs
- DbConnectionPoolIdentity.cs
- SoapReflectionImporter.cs
- CustomErrorsSection.cs
- Image.cs
- ContractMapping.cs
- FunctionParameter.cs
- NestPullup.cs
- HMACSHA1.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- WebPartDisplayModeCancelEventArgs.cs
- ScaleTransform3D.cs
- CorrelationService.cs
- RC2CryptoServiceProvider.cs
- PropVariant.cs
- SqlNotificationEventArgs.cs
- XamlReader.cs
- SystemIcons.cs
- Identifier.cs
- ParallelEnumerableWrapper.cs
- DocumentManager.cs
- DateTimeConstantAttribute.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- PrinterUnitConvert.cs
- RenderTargetBitmap.cs
- GeometryCombineModeValidation.cs
- HttpTransportSecurity.cs
- Qualifier.cs
- ListViewTableCell.cs
- ScriptingRoleServiceSection.cs
- CodeArrayCreateExpression.cs
- HierarchicalDataSourceControl.cs
- SqlBooleanMismatchVisitor.cs
- RoleService.cs
- RadioButtonBaseAdapter.cs
- typedescriptorpermission.cs
- CipherData.cs
- TraceHandlerErrorFormatter.cs
- IntellisenseTextBox.designer.cs
- ListManagerBindingsCollection.cs
- InvokePattern.cs
- securitymgrsite.cs
- WebPartManagerInternals.cs
- UdpConstants.cs
- DesignUtil.cs