Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Services / Timers / System / Timers / Timer.cs / 1 / Timer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Timers { using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Threading; using System.ComponentModel; using System.ComponentModel.Design; using System; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; ////// [ DefaultProperty("Interval"), DefaultEvent("Elapsed"), HostProtection(Synchronization=true, ExternalThreading=true) ] public class Timer : Component, ISupportInitialize { private double interval; private bool enabled; private bool initializing; private bool delayedEnable; private ElapsedEventHandler onIntervalElapsed; private bool autoReset; private ISynchronizeInvoke synchronizingObject; private bool disposed; private System.Threading.Timer timer; private TimerCallback callback; private Object cookie; ///Handles recurring events in an application. ////// public Timer() : base() { interval = 100; enabled = false; autoReset = true; initializing = false; delayedEnable = false; callback = new TimerCallback(this.MyTimerCallback); } ///Initializes a new instance of the ///class, with the properties /// set to initial values. /// public Timer(double interval) : this() { if (interval <= 0) throw new ArgumentException(SR.GetString(SR.InvalidParameter, "interval", interval)); int i = (int)Math.Ceiling(interval); if( i < 0) { throw new ArgumentException(SR.GetString(SR.InvalidParameter, "interval", interval)); } this.interval = interval; } ////// Initializes a new instance of the ///class, setting the property to the specified period. /// /// [Category("Behavior"), TimersDescription(SR.TimerAutoReset), DefaultValue(true)] public bool AutoReset { get { return this.autoReset; } set { if (DesignMode) this.autoReset = value; else if (this.autoReset != value) { this.autoReset = value; if( timer != null) { UpdateTimer(); } } } } ///Gets or sets a value indicating whether the Timer raises the Tick event each time the specified /// Interval has elapsed, /// when Enabled is set to true. ////// //[....] - The default value by design is false, don't change it. [Category("Behavior"), TimersDescription(SR.TimerEnabled), DefaultValue(false)] public bool Enabled { get { return this.enabled; } set { if (DesignMode) { this.delayedEnable = value; this.enabled = value; } else if (initializing) this.delayedEnable = value; else if (enabled != value) { if (!value) { if( timer != null) { cookie = null; timer.Dispose(); timer = null; } enabled = value; } else { enabled = value; if( timer == null) { if (disposed) { throw new ObjectDisposedException(GetType().Name); } int i = (int)Math.Ceiling(interval); cookie = new Object(); timer = new System.Threading.Timer(callback, cookie, i, autoReset? i:Timeout.Infinite); } else { UpdateTimer(); } } } } } private void UpdateTimer() { int i = (int)Math.Ceiling(interval); timer.Change(i, autoReset? i :Timeout.Infinite ); } ///Gets or sets a value indicating whether the ////// is able /// to raise events at a defined interval. /// [Category("Behavior"), TimersDescription(SR.TimerInterval), DefaultValue(100d), RecommendedAsConfigurable(true)] public double Interval { get { return this.interval; } set { if (value <= 0) throw new ArgumentException(SR.GetString(SR.TimerInvalidInterval, value, 0)); interval = value; if (timer != null) { UpdateTimer(); } } } ///Gets or /// sets the interval on which /// to raise events. ////// [Category("Behavior"), TimersDescription(SR.TimerIntervalElapsed)] public event ElapsedEventHandler Elapsed { add { onIntervalElapsed += value; } remove { onIntervalElapsed -= value; } } ///Occurs when the ///has /// elapsed. /// ////// Sets the enable property in design mode to true by default. /// ///public override ISite Site { set { base.Site = value; if (this.DesignMode) this.enabled= true; } get { return base.Site; } } /// /// [ Browsable(false), DefaultValue(null), TimersDescription(SR.TimerSynchronizingObject) ] public ISynchronizeInvoke SynchronizingObject { get { if (this.synchronizingObject == null && DesignMode) { IDesignerHost host = (IDesignerHost)GetService(typeof(IDesignerHost)); if (host != null) { object baseComponent = host.RootComponent; if (baseComponent != null && baseComponent is ISynchronizeInvoke) this.synchronizingObject = (ISynchronizeInvoke)baseComponent; } } return this.synchronizingObject; } set { this.synchronizingObject = value; } } ///Gets or sets the object used to marshal event-handler calls that are issued when /// an interval has elapsed. ////// public void BeginInit() { this.Close(); this.initializing = true; } ////// Notifies /// the object that initialization is beginning and tells it to stand by. /// ////// public void Close() { initializing = false; delayedEnable = false; enabled = false; if (timer != null ) { timer.Dispose(); timer = null; } } ///Disposes of the resources (other than memory) used by /// the ///. /// /// protected override void Dispose(bool disposing) { Close(); this.disposed = true; base.Dispose(disposing); } ////// public void EndInit() { this.initializing = false; this.Enabled = this.delayedEnable; } ////// Notifies the object that initialization is complete. /// ////// public void Start() { Enabled = true; } ///Starts the timing by setting ///to . /// public void Stop() { Enabled = false; } private void MyTimerCallback(object state) { // System.Threading.Timer will not cancel the work item queued before the timer is stopped. // We don't want to handle the callback after a timer is stopped. if( state != cookie) { return; } if (!this.autoReset) { enabled = false; } FILE_TIME filetime = new FILE_TIME(); GetSystemTimeAsFileTime(ref filetime); ElapsedEventArgs elapsedEventArgs = new ElapsedEventArgs(filetime.ftTimeLow, filetime.ftTimeHigh); try { // To avoid race between remove handler and raising the event ElapsedEventHandler intervalElapsed = this.onIntervalElapsed; if (intervalElapsed != null) { if (this.SynchronizingObject != null && this.SynchronizingObject.InvokeRequired) this.SynchronizingObject.BeginInvoke(intervalElapsed, new object[]{this, elapsedEventArgs}); else intervalElapsed(this, elapsedEventArgs); } } catch { } } [StructLayout(LayoutKind.Sequential)] internal struct FILE_TIME { internal int ftTimeLow; internal int ftTimeHigh; } [DllImport(ExternDll.Kernel32), SuppressUnmanagedCodeSecurityAttribute()] internal static extern void GetSystemTimeAsFileTime(ref FILE_TIME lpSystemTimeAsFileTime); } }/// Stops the timing by setting ///to . ///
Link Menu
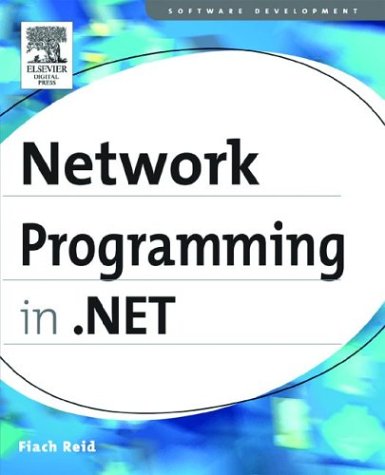
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CachedTypeface.cs
- ConnectionPoint.cs
- StyleHelper.cs
- FixUpCollection.cs
- EventLogTraceListener.cs
- CryptoApi.cs
- Signature.cs
- ProfessionalColors.cs
- LinqDataSourceView.cs
- XmlDataSourceView.cs
- SmtpFailedRecipientException.cs
- PathFigureCollection.cs
- HttpDigestClientElement.cs
- EventMap.cs
- DateTimeConstantAttribute.cs
- WebScriptServiceHostFactory.cs
- WebPartZoneBaseDesigner.cs
- DependencySource.cs
- MetadataAssemblyHelper.cs
- _OverlappedAsyncResult.cs
- SqlBulkCopyColumnMappingCollection.cs
- XPathSelectionIterator.cs
- XmlSchemaIdentityConstraint.cs
- SecurityManager.cs
- LicenseManager.cs
- ListBase.cs
- XmlSchemaSet.cs
- OrderablePartitioner.cs
- BulletDecorator.cs
- ListViewEditEventArgs.cs
- ContactManager.cs
- LabelExpression.cs
- CompilationRelaxations.cs
- DefaultExpression.cs
- PowerStatus.cs
- AssemblyAttributes.cs
- AuthenticateEventArgs.cs
- MultiAsyncResult.cs
- NavigationHelper.cs
- ConfigViewGenerator.cs
- Binding.cs
- ConfigDefinitionUpdates.cs
- Transform3DGroup.cs
- ThicknessKeyFrameCollection.cs
- AnimationTimeline.cs
- TextTreeUndoUnit.cs
- EventEntry.cs
- FileEnumerator.cs
- PathGradientBrush.cs
- ProfessionalColors.cs
- ErrorsHelper.cs
- WinInet.cs
- CatalogZone.cs
- TraceListener.cs
- SqlFunctions.cs
- ManifestSignedXml.cs
- ScriptMethodAttribute.cs
- XmlArrayItemAttributes.cs
- httpstaticobjectscollection.cs
- HttpCacheVary.cs
- X509ChainPolicy.cs
- MessageEncodingBindingElement.cs
- ObjectHandle.cs
- ICspAsymmetricAlgorithm.cs
- CompositeScriptReference.cs
- WeakEventManager.cs
- SiteMapSection.cs
- HMACSHA384.cs
- EditingCommands.cs
- WindowsSpinner.cs
- FixedPageStructure.cs
- GrammarBuilderPhrase.cs
- PageOutputQuality.cs
- DesignerWithHeader.cs
- VBCodeProvider.cs
- HelpKeywordAttribute.cs
- HwndTarget.cs
- Descriptor.cs
- DBParameter.cs
- ModelFunction.cs
- TraceSource.cs
- ListViewItemSelectionChangedEvent.cs
- ToolStripOverflow.cs
- InvalidEnumArgumentException.cs
- VerificationAttribute.cs
- NativeBuffer.cs
- TreeNodeStyleCollection.cs
- WindowsRichEditRange.cs
- DataGridViewRowConverter.cs
- RestHandler.cs
- TrackBar.cs
- XsltSettings.cs
- ZipFileInfoCollection.cs
- TextBoxRenderer.cs
- RSAOAEPKeyExchangeDeformatter.cs
- RadialGradientBrush.cs
- XmlSchemaSimpleContentRestriction.cs
- SkewTransform.cs
- Int64Animation.cs
- HtmlWindowCollection.cs