Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ToolStripAdornerWindowService.cs / 1 / ToolStripAdornerWindowService.cs
namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Security; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Design; using System.Runtime.InteropServices; using System.Windows.Forms.Design.Behavior; ////// /// Transparent Window to parent the DropDowns. /// internal sealed class ToolStripAdornerWindowService : IDisposable { private IServiceProvider serviceProvider; //standard service provider private ToolStripAdornerWindow toolStripAdornerWindow; //the transparent window all glyphs are drawn to private BehaviorService bs; private Adorner dropDownAdorner; private ArrayList dropDownCollection; private IOverlayService os; ////// This constructor is called from DocumentDesigner's Initialize method. /// internal ToolStripAdornerWindowService(IServiceProvider serviceProvider, Control windowFrame) { this.serviceProvider = serviceProvider; //create the AdornerWindow toolStripAdornerWindow = new ToolStripAdornerWindow(windowFrame); bs = (BehaviorService)serviceProvider.GetService(typeof(BehaviorService)); int indexToInsert = bs.AdornerWindowIndex; //use the adornerWindow as an overlay os = (IOverlayService)serviceProvider.GetService(typeof(IOverlayService)); if (os != null) { os.InsertOverlay(toolStripAdornerWindow, indexToInsert); } dropDownAdorner = new Adorner(); int count = bs.Adorners.Count; // Why this is NEEDED ? // To Add the Adorner at proper index in the AdornerCollection for the BehaviorService // So that the DesignerActionGlyph always stays on the Top. if (count > 1) { bs.Adorners.Insert(count - 1, dropDownAdorner); } } ////// Returns the actual Control that represents the transparent AdornerWindow. /// internal Control ToolStripAdornerWindowControl { get { return toolStripAdornerWindow; } } ////// /// Creates and returns a Graphics object for the AdornerWindow /// public Graphics ToolStripAdornerWindowGraphics { get { return toolStripAdornerWindow.CreateGraphics(); } } internal Adorner DropDownAdorner { get { return dropDownAdorner; } } ////// /// Disposes the behavior service. /// [SuppressMessage("Microsoft.Usage", "CA2213:DisposableFieldsShouldBeDisposed")] public void Dispose() { if (os != null) { os.RemoveOverlay(toolStripAdornerWindow); } toolStripAdornerWindow.Dispose(); if (bs != null) { bs.Adorners.Remove(dropDownAdorner); bs = null; } if (dropDownAdorner != null) { dropDownAdorner.Glyphs.Clear(); dropDownAdorner = null; } } ////// /// Translates a point in the AdornerWindow to screen coords. /// public Point AdornerWindowPointToScreen(Point p) { NativeMethods.POINT offset = new NativeMethods.POINT(p.X, p.Y); NativeMethods.MapWindowPoints(toolStripAdornerWindow.Handle, IntPtr.Zero, offset, 1); return new Point(offset.x, offset.y); } ////// /// Gets the location (upper-left corner) of the AdornerWindow in screen coords. /// public Point AdornerWindowToScreen() { Point origin = new Point(0, 0); return AdornerWindowPointToScreen(origin); } ////// /// Returns the location of a Control translated to AdornerWidnow coords. /// public Point ControlToAdornerWindow(Control c) { if (c.Parent == null) { return Point.Empty; } NativeMethods.POINT pt = new NativeMethods.POINT(); pt.x = c.Left; pt.y = c.Top; NativeMethods.MapWindowPoints(c.Parent.Handle, toolStripAdornerWindow.Handle, pt, 1); return new Point(pt.x, pt.y); } ////// /// Invalidates the BehaviorService's AdornerWindow. This will force a refesh of all Adorners /// and, in turn, all Glyphs. /// public void Invalidate() { toolStripAdornerWindow.InvalidateAdornerWindow(); } ////// /// Invalidates the BehaviorService's AdornerWindow. This will force a refesh of all Adorners /// and, in turn, all Glyphs. /// public void Invalidate(Rectangle rect) { toolStripAdornerWindow.InvalidateAdornerWindow(rect); } ////// /// Invalidates the BehaviorService's AdornerWindow. This will force a refesh of all Adorners /// and, in turn, all Glyphs. /// public void Invalidate(Region r) { toolStripAdornerWindow.InvalidateAdornerWindow(r); } internal ArrayList DropDowns { get { return dropDownCollection; } set { if (dropDownCollection == null) { dropDownCollection = new ArrayList(); } } } ////// ControlDesigner calls this internal method in response to a WmPaint. /// We need to know when a ControlDesigner paints - 'cause we will need /// to re-paint any glyphs above of this Control. /// internal void ProcessPaintMessage(Rectangle paintRect) { //Note, we don't call BehSvc.Invalidate because //this will just cause the messages to recurse. //Instead, invalidating this adornerWindow will //just cause a "propagatePaint" and draw the glyphs. toolStripAdornerWindow.Invalidate(paintRect); } ////// The AdornerWindow is a transparent window that resides ontop of the /// Designer's Frame. This window is used by the ToolStripAdornerWindowService to /// parent the MenuItem DropDowns. /// private class ToolStripAdornerWindow : Control { private Control designerFrame;//the designer's frame ////// Constructor that parents itself to the Designer Frame and hooks all /// necessary events. /// [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] internal ToolStripAdornerWindow(Control designerFrame) { this.designerFrame = designerFrame; this.Dock = DockStyle.Fill; this.AllowDrop = true; this.Text = "ToolStripAdornerWindow"; SetStyle(ControlStyles.Opaque,true); } ////// The key here is to set the appropriate TransparetWindow style. /// protected override CreateParams CreateParams { get { CreateParams cp = base.CreateParams; cp.Style &= ~(NativeMethods.WS_CLIPCHILDREN | NativeMethods.WS_CLIPSIBLINGS); cp.ExStyle |= 0x00000020/*WS_EX_TRANSPARENT*/; return cp; } } ////// We'll use CreateHandle as our notification for creating // our mouse hooker. /// protected override void OnHandleCreated(EventArgs e) { base.OnHandleCreated(e); } ////// Unhook and null out our mouseHook. /// protected override void OnHandleDestroyed(EventArgs e) { base.OnHandleDestroyed(e); } ////// Null out our mouseHook and unhook any events. /// protected override void Dispose(bool disposing) { if (disposing) { if (designerFrame != null) { designerFrame = null; } } base.Dispose(disposing); } ////// Returns true if the DesignerFrame is created & not being disposed. /// private bool DesignerFrameValid { get { if (designerFrame == null || designerFrame.IsDisposed || !designerFrame.IsHandleCreated) { return false; } return true; } } ////// Invalidates the transparent AdornerWindow by asking /// the Designer Frame beneath it to invalidate. Note the /// they use of the .Update() call for perf. purposes. /// internal void InvalidateAdornerWindow() { if (DesignerFrameValid) { designerFrame.Invalidate(true); designerFrame.Update(); } } ////// Invalidates the transparent AdornerWindow by asking /// the Designer Frame beneath it to invalidate. Note the /// they use of the .Update() call for perf. purposes. /// internal void InvalidateAdornerWindow(Region region) { if (DesignerFrameValid) { designerFrame.Invalidate(region, true); designerFrame.Update(); } } ////// Invalidates the transparent AdornerWindow by asking /// the Designer Frame beneath it to invalidate. Note the /// they use of the .Update() call for perf. purposes. /// internal void InvalidateAdornerWindow(Rectangle rectangle) { if (DesignerFrameValid) { designerFrame.Invalidate(rectangle, true); designerFrame.Update(); } } ////// The AdornerWindow intercepts all designer-related messages and forwards them /// to the BehaviorService for appropriate actions. Note that Paint and HitTest /// messages are correctly parsed and translated to AdornerWindow coords. /// protected override void WndProc(ref Message m) { switch(m.Msg) { case NativeMethods.WM_NCHITTEST: m.Result = (IntPtr)(NativeMethods.HTTRANSPARENT); break; default: base.WndProc(ref m); break; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
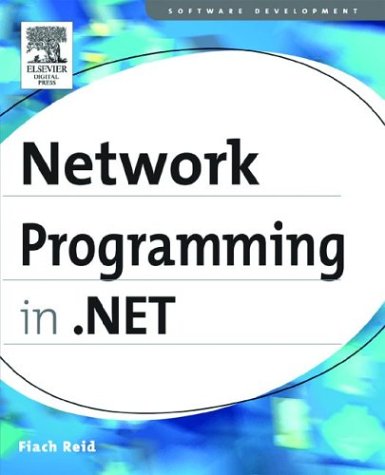
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StreamUpgradeBindingElement.cs
- LogExtent.cs
- Comparer.cs
- Container.cs
- RegexWorker.cs
- HttpGetServerProtocol.cs
- JsonReader.cs
- Application.cs
- PartialCachingAttribute.cs
- FontStretches.cs
- DataGridViewComboBoxCell.cs
- XmlTextEncoder.cs
- _ListenerResponseStream.cs
- SettingsProviderCollection.cs
- DataGridViewCheckBoxCell.cs
- MimeMultiPart.cs
- DoubleAnimationBase.cs
- AdRotator.cs
- CodeMethodReturnStatement.cs
- InstallerTypeAttribute.cs
- ActivationServices.cs
- ScriptBehaviorDescriptor.cs
- StateMachineWorkflowDesigner.cs
- ColorTransformHelper.cs
- PartialCachingAttribute.cs
- EmptyCollection.cs
- wgx_sdk_version.cs
- DataServiceRequestException.cs
- InitializationEventAttribute.cs
- DataSysAttribute.cs
- PromptStyle.cs
- SoapServerMethod.cs
- CacheModeValueSerializer.cs
- ChangePassword.cs
- TimeSpanMinutesConverter.cs
- RoleGroup.cs
- DataGridViewSortCompareEventArgs.cs
- SrgsElementFactoryCompiler.cs
- MetadataCache.cs
- StyleSelector.cs
- CaseInsensitiveOrdinalStringComparer.cs
- DocumentXPathNavigator.cs
- httpserverutility.cs
- SqlInternalConnection.cs
- ReadOnlyCollectionBase.cs
- CopyNamespacesAction.cs
- TextParagraphProperties.cs
- ListGeneralPage.cs
- ServiceEndpointCollection.cs
- TextSpan.cs
- ConfigsHelper.cs
- DataControlButton.cs
- RegexWorker.cs
- RectangleHotSpot.cs
- ColorInterpolationModeValidation.cs
- PageOrientation.cs
- DesignSurfaceServiceContainer.cs
- SimpleType.cs
- OleDbInfoMessageEvent.cs
- TableSectionStyle.cs
- XmlEncodedRawTextWriter.cs
- CryptoApi.cs
- Container.cs
- Imaging.cs
- MetafileHeader.cs
- EditorZoneBase.cs
- OleDbRowUpdatedEvent.cs
- SelectionItemPattern.cs
- MultiByteCodec.cs
- ClientSideQueueItem.cs
- Camera.cs
- RadioButton.cs
- WebPermission.cs
- TrackingServices.cs
- DataGridTablesFactory.cs
- DBSchemaRow.cs
- SqlDataSourceEnumerator.cs
- ProfilePropertyMetadata.cs
- ConfigXmlWhitespace.cs
- NumericExpr.cs
- LingerOption.cs
- RuntimeWrappedException.cs
- LambdaCompiler.Generated.cs
- NetWebProxyFinder.cs
- DbException.cs
- PseudoWebRequest.cs
- ImageListStreamer.cs
- FunctionQuery.cs
- SQLBinaryStorage.cs
- WebPartTracker.cs
- SafeFileHandle.cs
- TextEditorDragDrop.cs
- SettingsContext.cs
- QueryStringParameter.cs
- EraserBehavior.cs
- ScriptIgnoreAttribute.cs
- StyleSelector.cs
- DirectoryInfo.cs
- TableCellAutomationPeer.cs
- DesignSurface.cs