Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / StringCollectionEditor.cs / 1 / StringCollectionEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #define NEEDHELPBUTTON /* */ namespace System.Windows.Forms.Design { using System.Design; using System.ComponentModel; using System; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Windows.Forms; ////// /// The StringCollectionEditor is a collection editor that is specifically /// designed to edit collections containing strings. The collection can be /// of any type that can accept a string value; we just present a string-centric /// dialog for the user. /// internal class StringCollectionEditor : CollectionEditor { public StringCollectionEditor(Type type) : base(type) { } ////// /// Creates a new form to show the current collection. You may inherit /// from CollectionForm to provide your own form. /// protected override CollectionForm CreateCollectionForm() { return new StringCollectionForm(this); } ////// /// protected override string HelpTopic { get { return "net.ComponentModel.StringCollectionEditor"; } } ///Gets the help topic to display for the dialog help button or pressing F1. Override to /// display a different help topic. ////// /// StringCollectionForm allows visible editing of a string array. Each line in /// the edit box is an array entry. /// private class StringCollectionForm : CollectionForm { private Label instruction; private TextBox textEntry; private Button okButton; private Button cancelButton; private TableLayoutPanel okCancelTableLayoutPanel; private StringCollectionEditor editor = null; ////// /// Constructs a StringCollectionForm. /// public StringCollectionForm(CollectionEditor editor) : base(editor) { this.editor = (StringCollectionEditor) editor; InitializeComponent(); HookEvents(); } private void Edit1_keyDown(object sender, KeyEventArgs e) { if (e.KeyCode == Keys.Escape) { cancelButton.PerformClick(); e.Handled = true; } } private void StringCollectionEditor_HelpButtonClicked(object sender, CancelEventArgs e) { e.Cancel = true; editor.ShowHelp(); } private void Form_HelpRequested(object sender, HelpEventArgs e) { editor.ShowHelp(); } private void HookEvents() { this.textEntry.KeyDown += new KeyEventHandler(this.Edit1_keyDown); this.okButton.Click += new EventHandler(this.OKButton_click); this.HelpButtonClicked += new System.ComponentModel.CancelEventHandler(this.StringCollectionEditor_HelpButtonClicked); } ////// /// NOTE: The following code is required by the form /// designer. It can be modified using the form editor. Do not /// modify it using the code editor. /// private void InitializeComponent() { System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(StringCollectionEditor)); this.instruction = new System.Windows.Forms.Label(); this.textEntry = new System.Windows.Forms.TextBox(); this.okButton = new System.Windows.Forms.Button(); this.cancelButton = new System.Windows.Forms.Button(); this.okCancelTableLayoutPanel = new System.Windows.Forms.TableLayoutPanel(); this.okCancelTableLayoutPanel.SuspendLayout(); this.SuspendLayout(); // // instruction // resources.ApplyResources(this.instruction, "instruction"); this.instruction.Margin = new System.Windows.Forms.Padding(3, 1, 3, 0); this.instruction.Name = "instruction"; // // textEntry // this.textEntry.AcceptsTab = true; this.textEntry.AcceptsReturn = true; resources.ApplyResources(this.textEntry, "textEntry"); this.textEntry.Name = "textEntry"; // // okButton // resources.ApplyResources(this.okButton, "okButton"); this.okButton.DialogResult = System.Windows.Forms.DialogResult.OK; this.okButton.Margin = new System.Windows.Forms.Padding(0, 0, 3, 0); this.okButton.Name = "okButton"; // // cancelButton // resources.ApplyResources(this.cancelButton, "cancelButton"); this.cancelButton.DialogResult = System.Windows.Forms.DialogResult.Cancel; this.cancelButton.Margin = new System.Windows.Forms.Padding(3, 0, 0, 0); this.cancelButton.Name = "cancelButton"; // // okCancelTableLayoutPanel // resources.ApplyResources(this.okCancelTableLayoutPanel, "okCancelTableLayoutPanel"); this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.Controls.Add(this.okButton, 0, 0); this.okCancelTableLayoutPanel.Controls.Add(this.cancelButton, 1, 0); this.okCancelTableLayoutPanel.Name = "okCancelTableLayoutPanel"; this.okCancelTableLayoutPanel.RowStyles.Add(new System.Windows.Forms.RowStyle()); // // StringCollectionEditor // resources.ApplyResources(this, "$this"); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.Controls.Add(this.okCancelTableLayoutPanel); this.Controls.Add(this.instruction); this.Controls.Add(this.textEntry); this.HelpButton = true; this.MaximizeBox = false; this.MinimizeBox = false; this.Name = "StringCollectionEditor"; this.ShowIcon = false; this.ShowInTaskbar = false; this.okCancelTableLayoutPanel.ResumeLayout(false); this.okCancelTableLayoutPanel.PerformLayout(); this.HelpRequested += new HelpEventHandler(this.Form_HelpRequested); this.ResumeLayout(false); this.PerformLayout(); } ////// /// Commits the changes to the editor. /// private void OKButton_click(object sender, EventArgs e) { char[] delims = new char[] {'\n'}; char[] trims = new char[] {'\r'}; string[] strings = textEntry.Text.Split(delims); object[] curItems = Items; int nItems = strings.Length; for (int i = 0; i < nItems; i++) { strings[i] = strings[i].Trim(trims); } bool dirty = true; if (nItems == curItems.Length) { int i; for (i = 0; i < nItems; ++i) { if (!strings[i].Equals((string)curItems[i])) { break; } } if (i == nItems) dirty = false; } if (!dirty) { DialogResult = DialogResult.Cancel; return; } // ASURT #57372 // If the final line is blank, we don't want to create an item from it // if (strings.Length > 0 && strings[strings.Length - 1].Length == 0) { nItems--; } object[] values = new object[nItems]; for (int i = 0; i < nItems; i++) { values[i] = strings[i]; } Items = values; } //// This is called when the value property in the CollectionForm has changed. // In it you should update your user interface to reflect the current value. // // protected override void OnEditValueChanged() { object[] items = Items; string text = string.Empty; for (int i = 0; i < items.Length; i++) { if (items[i] is string) { text += (string)items[i]; if (i != items.Length - 1) { text += "\r\n"; } } } textEntry.Text = text; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
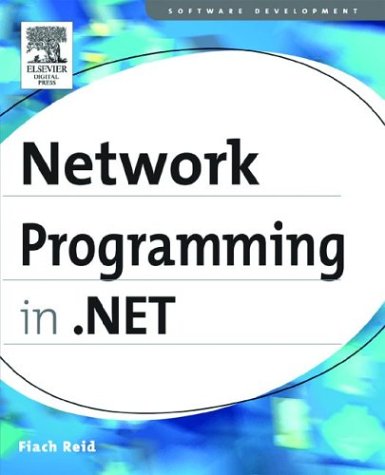
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Timer.cs
- TraceFilter.cs
- HttpApplicationFactory.cs
- DataFormats.cs
- Roles.cs
- PlainXmlSerializer.cs
- ObjectDisposedException.cs
- AddingNewEventArgs.cs
- DrawingBrush.cs
- GestureRecognitionResult.cs
- ByeOperation11AsyncResult.cs
- BamlBinaryWriter.cs
- DataObjectMethodAttribute.cs
- DataGridViewLinkColumn.cs
- ObjectPersistData.cs
- FileVersion.cs
- ReaderWriterLock.cs
- BaseTemplateCodeDomTreeGenerator.cs
- DriveInfo.cs
- Base64WriteStateInfo.cs
- KeyedHashAlgorithm.cs
- BackgroundWorker.cs
- WorkflowDefinitionDispenser.cs
- DateBoldEvent.cs
- VerbConverter.cs
- MissingSatelliteAssemblyException.cs
- CodeGen.cs
- AdCreatedEventArgs.cs
- ListViewUpdatedEventArgs.cs
- QilList.cs
- ControlValuePropertyAttribute.cs
- WindowsScrollBar.cs
- AudioDeviceOut.cs
- GeneratedContractType.cs
- WizardSideBarListControlItemEventArgs.cs
- WindowsNonControl.cs
- FastEncoderWindow.cs
- MemberAssignmentAnalysis.cs
- ProfileInfo.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- ObjectContext.cs
- ImageInfo.cs
- NumericExpr.cs
- SecurityContextSecurityToken.cs
- _NegotiateClient.cs
- Separator.cs
- AVElementHelper.cs
- XmlnsDictionary.cs
- OdbcConnection.cs
- WeakReferenceKey.cs
- GeneralTransform2DTo3DTo2D.cs
- XmlQueryContext.cs
- XmlComplianceUtil.cs
- RightsManagementEncryptionTransform.cs
- DataViewListener.cs
- ResourceExpressionBuilder.cs
- Lasso.cs
- AuthenticationService.cs
- StringBuilder.cs
- TreeViewEvent.cs
- DependencyObject.cs
- SqlDependencyListener.cs
- XmlArrayAttribute.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- SqlProcedureAttribute.cs
- PenThreadPool.cs
- XPathNavigator.cs
- PropertyEmitter.cs
- ExtractedStateEntry.cs
- PropertyDescriptor.cs
- VisualCollection.cs
- QueuePathEditor.cs
- MetabaseSettings.cs
- Command.cs
- StateMachineWorkflowInstance.cs
- AnnotationStore.cs
- _Semaphore.cs
- XPathCompiler.cs
- ButtonChrome.cs
- ObjectTypeMapping.cs
- TextHidden.cs
- OleDbConnectionFactory.cs
- FormsAuthenticationCredentials.cs
- ContractNamespaceAttribute.cs
- SHA256.cs
- ExistsInCollection.cs
- LocationUpdates.cs
- IPEndPoint.cs
- Environment.cs
- CompModSwitches.cs
- SerializerWriterEventHandlers.cs
- ProviderSettingsCollection.cs
- TraceUtils.cs
- ResolveCriteriaCD1.cs
- RenderDataDrawingContext.cs
- PointKeyFrameCollection.cs
- InfiniteTimeSpanConverter.cs
- TCEAdapterGenerator.cs
- QilPatternFactory.cs
- WithParamAction.cs