Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / StatementContext.cs / 1 / StatementContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.Collections; using System.Collections.Generic; ////// /// This object can be placed on the context stack to provide a place for statements /// to be serialized into. Normally, statements are serialized into whatever statement /// collection that is on the context stack. You can modify this behavior by creating /// a statement context and calling Populate with a collection of objects whose statements /// you would like stored in the statement table. As each object is serialized in /// SerializeToExpression it will have its contents placed in the statement table. /// saved in a table within the context. If you push this object on the stack it is your /// responsibility to integrate the statements added to it into your own collection of statements. /// public sealed class StatementContext { private ObjectStatementCollection _statements; ////// /// This is a table of statements that is offered by the statement context. /// public ObjectStatementCollection StatementCollection { get { if (_statements == null) { _statements = new ObjectStatementCollection(); } return _statements; } } } ////// /// This is a table of statements that is offered by the statement context. /// public sealed class ObjectStatementCollection : IEnumerable { private List_table; private int _version; /// /// Only creatable by the StatementContext. /// internal ObjectStatementCollection() { } ////// Adds an owner to the table. Statements can be null, in which case it /// will be demand created when fished out of the table. This will throw /// if there is already a valid collection for the owner. /// private void AddOwner(object statementOwner, CodeStatementCollection statements) { if (_table == null) { _table = new List(); } else { for (int idx = 0; idx < _table.Count; idx++) { if (object.ReferenceEquals(_table[idx].Owner, statementOwner)) { if (_table[idx].Statements != null) { throw new InvalidOperationException(); } else { if (statements != null) { _table[idx] = new TableEntry(statementOwner, statements); } return; } } } } _table.Add(new TableEntry(statementOwner, statements)); _version++; } /// /// /// Indexer. This will return the statement collection for the given owner. /// It will return null only if the owner is not in the table. /// public CodeStatementCollection this[object statementOwner] { get { if (statementOwner == null) { throw new ArgumentNullException("statementOwner"); } if (_table != null) { for (int idx = 0; idx < _table.Count; idx++) { if (object.ReferenceEquals(_table[idx].Owner, statementOwner)) { if (_table[idx].Statements == null) { _table[idx] = new TableEntry(statementOwner, new CodeStatementCollection()); } return _table[idx].Statements; } } foreach(TableEntry e in _table) { if (object.ReferenceEquals(e.Owner, statementOwner)) { return e.Statements; } } } return null; } } ////// /// Returns true if the given statement owner is in the table. /// public bool ContainsKey(object statementOwner) { if (statementOwner == null) { throw new ArgumentNullException("statementOwner"); } if (_table != null) { return (this[statementOwner] != null); } return false; } ////// /// Returns an enumerator for this table. The keys of the enumerator are statement /// owner objects and the values are instances of CodeStatementCollection. /// public IDictionaryEnumerator GetEnumerator() { return new TableEnumerator(this); } ////// /// This method populates the statement table with a collection of statement owners. /// The creator of the statement context should do this if it wishes statement tables to be used to store /// values for certain objects. /// public void Populate(ICollection statementOwners) { if (statementOwners == null) { throw new ArgumentNullException("statementOwners"); } foreach(object o in statementOwners) { Populate(o); } } ////// /// This method populates the statement table with a collection of statement owners. /// The creator of the statement context should do this if it wishes statement tables to be used to store /// values for certain objects. /// public void Populate(object owner) { if (owner == null) { throw new ArgumentNullException("owner"); } AddOwner(owner, null); } ////// /// Returns an enumerator for this table. The value is a DictionaryEntry containing /// the statement owner and the statement collection. /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } private struct TableEntry { public object Owner; public CodeStatementCollection Statements; public TableEntry(object owner, CodeStatementCollection statements) { this.Owner = owner; this.Statements = statements; } } private struct TableEnumerator : IDictionaryEnumerator { private ObjectStatementCollection _table; private int _version; int _position; public TableEnumerator(ObjectStatementCollection table) { _table = table; _version = _table._version; _position = -1; } public object Current { get { return Entry; } } public DictionaryEntry Entry { get { if (_version != _table._version) { throw new InvalidOperationException(); } if (_position < 0 || _table._table == null || _position >= _table._table.Count) { throw new InvalidOperationException(); } if (_table._table[_position].Statements == null) { _table._table[_position] = new TableEntry(_table._table[_position].Owner, new CodeStatementCollection()); } TableEntry entry = _table._table[_position]; return new DictionaryEntry(entry.Owner, entry.Statements); } } public object Key { get { return Entry.Key; } } public object Value { get { return Entry.Value; } } public bool MoveNext() { if (_table._table != null && (_position+1) < _table._table.Count) { _position++; return true; } return false; } public void Reset() { _position = -1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
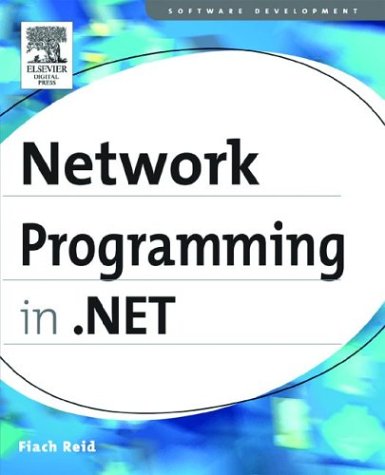
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewSelectedRowCollection.cs
- ResolveDuplex11AsyncResult.cs
- ProxyWebPartManager.cs
- CounterSet.cs
- NonPrimarySelectionGlyph.cs
- PartialList.cs
- _OSSOCK.cs
- SerializationEventsCache.cs
- EncodingTable.cs
- bindurihelper.cs
- AuthenticationSection.cs
- SessionStateItemCollection.cs
- AttributeEmitter.cs
- SiteMapDataSource.cs
- XmlText.cs
- ClipboardData.cs
- SignedPkcs7.cs
- ItemMap.cs
- activationcontext.cs
- StatusBar.cs
- GridEntryCollection.cs
- SamlAssertionKeyIdentifierClause.cs
- PartialTrustVisibleAssemblyCollection.cs
- MissingSatelliteAssemblyException.cs
- XslTransform.cs
- PropertyChangedEventArgs.cs
- ToolBarButton.cs
- ZipIOBlockManager.cs
- CodeDomExtensionMethods.cs
- AtlasWeb.Designer.cs
- Schedule.cs
- DiscoveryServiceExtension.cs
- HttpProcessUtility.cs
- CdpEqualityComparer.cs
- UnmanagedHandle.cs
- EditCommandColumn.cs
- PartialList.cs
- DoubleAnimationBase.cs
- XmlMapping.cs
- WebPartTracker.cs
- ParameterCollectionEditorForm.cs
- RelativeSource.cs
- Set.cs
- TimeZoneNotFoundException.cs
- __TransparentProxy.cs
- MiniConstructorInfo.cs
- WebSysDisplayNameAttribute.cs
- DbParameterCollectionHelper.cs
- ImageFormat.cs
- UpdateManifestForBrowserApplication.cs
- DependencyPropertyChangedEventArgs.cs
- FormCollection.cs
- IChannel.cs
- CoTaskMemSafeHandle.cs
- ScrollBar.cs
- ConfigXmlCDataSection.cs
- BatchWriter.cs
- DatatypeImplementation.cs
- GetPageCompletedEventArgs.cs
- EnumerableRowCollection.cs
- MemoryPressure.cs
- ConstructorNeedsTagAttribute.cs
- InputLanguageCollection.cs
- Style.cs
- AutoGeneratedFieldProperties.cs
- SingleConverter.cs
- RegistrySecurity.cs
- SqlDelegatedTransaction.cs
- CanExecuteRoutedEventArgs.cs
- ExtenderControl.cs
- TemplateBindingExtensionConverter.cs
- _OSSOCK.cs
- PropertyToken.cs
- HitTestWithGeometryDrawingContextWalker.cs
- SmiMetaDataProperty.cs
- AsymmetricKeyExchangeFormatter.cs
- SchemaImporterExtensionsSection.cs
- PartitionedStreamMerger.cs
- WebPart.cs
- TextViewSelectionProcessor.cs
- HttpApplicationStateWrapper.cs
- Token.cs
- StrongName.cs
- HttpProcessUtility.cs
- ValidatedControlConverter.cs
- SoapFormatterSinks.cs
- AccessDataSource.cs
- ArgumentException.cs
- CategoryAttribute.cs
- MemberDescriptor.cs
- HandlerWithFactory.cs
- AppSettingsReader.cs
- XamlToRtfParser.cs
- EmptyCollection.cs
- WindowsButton.cs
- GridView.cs
- ShapingWorkspace.cs
- CodeTypeReferenceExpression.cs
- TimersDescriptionAttribute.cs
- ExeContext.cs