Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Localizer.cs / 1 / Localizer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System.Design; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Globalization; using System.Windows.Forms; using System.Windows.Forms.Design; using Microsoft.Win32; using System.Threading; using System.Runtime.Remoting.Contexts; ////// /// [ ProvideProperty("Language", typeof(object)), ProvideProperty("LoadLanguage", typeof(object)), ProvideProperty("Localizable", typeof(object)) ] [Obsolete("This class has been deprecated. Use CodeDomLocalizationProvider instead. http://go.microsoft.com/fwlink/?linkid=14202")] public class LocalizationExtenderProvider : IExtenderProvider, IDisposable { private IServiceProvider serviceProvider; private IComponent baseComponent; private bool localizable; private bool defaultLocalizable = false; private CultureInfo language; private CultureInfo loadLanguage; private CultureInfo defaultLanguage; private const string KeyThreadDefaultLanguage = "_Thread_Default_Language"; private static object localizationLock = new Object(); ///Provides design-time localization support to enable code /// generators to provide localization features. ////// /// public LocalizationExtenderProvider(ISite serviceProvider, IComponent baseComponent) { this.serviceProvider = (IServiceProvider)serviceProvider; this.baseComponent = baseComponent; if (serviceProvider != null) { IExtenderProviderService es = (IExtenderProviderService)serviceProvider.GetService(typeof(IExtenderProviderService)); if (es != null) { es.AddExtenderProvider(this); } } language = CultureInfo.InvariantCulture; //We need to check to see if our baseComponent has its localizable value persisted into //the resource file. If so, we'll want to "inherit" this value for our baseComponent. //This enables us to create Inherited forms and inherit the localizable props from the base. System.Resources.ResourceManager resources = new System.Resources.ResourceManager(baseComponent.GetType()); if (resources != null) { System.Resources.ResourceSet rSet = resources.GetResourceSet(language, true, false); if (rSet != null) { object objLocalizable = rSet.GetObject("$this.Localizable"); if (objLocalizable is bool) { defaultLocalizable = (bool)objLocalizable; this.localizable = defaultLocalizable; } } } } private CultureInfo ThreadDefaultLanguage { get { lock(localizationLock){ if (defaultLanguage != null) { return defaultLanguage; } LocalDataStoreSlot dataSlot = Thread.GetNamedDataSlot(LocalizationExtenderProvider.KeyThreadDefaultLanguage); if (dataSlot == null) { Debug.Fail("Failed to get a data slot for ui culture"); return null; } this.defaultLanguage = (CultureInfo)Thread.GetData(dataSlot); if (this.defaultLanguage == null) { this.defaultLanguage = Application.CurrentCulture; Thread.SetData(dataSlot, this.defaultLanguage); } } return this.defaultLanguage; } } ///Initializes a new instance of the ///class using the /// specified service provider and base component. /// /// [ DesignOnly(true), Localizable(true), SRDescriptionAttribute("ParentControlDesignerLanguageDescr") ] public CultureInfo GetLanguage(object o) { return language; } ///Gets the language set for the specified object. ////// /// [ DesignOnly(true), Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public CultureInfo GetLoadLanguage(object o) { // If we never configured the load language, we're always invariant. if (loadLanguage == null) { loadLanguage = CultureInfo.InvariantCulture; } return loadLanguage; } ///Gets the language we'll use when re-loading the designer. ////// /// [ DesignOnly(true), Localizable(true), SRDescriptionAttribute("ParentControlDesignerLocalizableDescr") ] public bool GetLocalizable(object o) { return localizable; } ///Gets a value indicating whether the specified object supports design-time localization /// support. ////// /// public void SetLanguage(object o, CultureInfo language) { if(language == null) { language = CultureInfo.InvariantCulture; } if (this.language.Equals(language)) { return; } bool isInvariantCulture = (language.Equals(CultureInfo.InvariantCulture)); CultureInfo defaultUICulture = this.ThreadDefaultLanguage; this.language = language; if (!isInvariantCulture) { SetLocalizable(null,true); } if (serviceProvider != null) { IDesignerLoaderService ls = (IDesignerLoaderService)serviceProvider.GetService(typeof(IDesignerLoaderService)); IDesignerHost host = (IDesignerHost)serviceProvider.GetService(typeof(IDesignerHost)); // Only reload if we're not in the process of loading! // if (host != null) { // If we're loading, adopt the load language for later use. // if (host.Loading) { loadLanguage = language; } else { bool reloadSuccessful = false; if (ls != null) { reloadSuccessful = ls.Reload(); } if (!reloadSuccessful) { IUIService uis = (IUIService)serviceProvider.GetService(typeof(IUIService)); if (uis != null) { uis.ShowMessage(SR.GetString(SR.LocalizerManualReload)); } } } } } } ///Sets the language to use. ////// /// public void SetLocalizable(object o, bool localizable) { this.localizable = localizable; if (!localizable) { SetLanguage(null, CultureInfo.InvariantCulture); } } ///Sets a value indicating whether or not the specified object has design-time /// localization support. ////// /// public bool ShouldSerializeLanguage(object o) { return (language != null && language != CultureInfo.InvariantCulture); } ///Gets a value indicating whether the specified object should have its design-time localization support persisted. ////// [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] private bool ShouldSerializeLocalizable(object o) { return (localizable != defaultLocalizable); } ///Gets a value indicating whether the specified object should have its design-time localization support persisted. ////// [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] private void ResetLocalizable(object o) { SetLocalizable(null, defaultLocalizable); } ///Resets the localizable property to the 'defaultLocalizable' value. ////// /// public void ResetLanguage(object o) { SetLanguage(null, CultureInfo.InvariantCulture); } ///Resets the language for the specified /// object. ////// /// public void Dispose() { Dispose(true); } protected virtual void Dispose(bool disposing) { if (disposing && serviceProvider != null) { IExtenderProviderService es = (IExtenderProviderService)serviceProvider.GetService(typeof(IExtenderProviderService)); if (es != null) { es.RemoveExtenderProvider(this); } } } ///Disposes of the resources (other than memory) used by the ///. /// /// public bool CanExtend(object o) { return o.Equals(baseComponent); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Gets a value indicating whether the ///provides design-time localization information for the specified object.
Link Menu
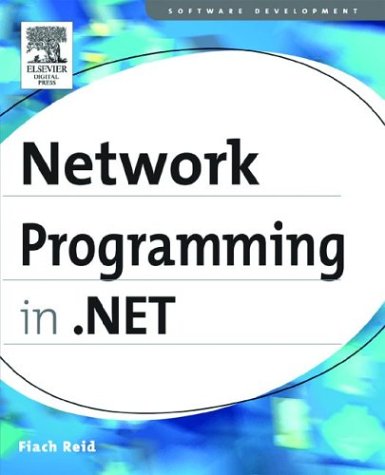
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OracleCommandBuilder.cs
- TextRangeEditTables.cs
- EncryptedHeader.cs
- DeclarationUpdate.cs
- PropVariant.cs
- FastEncoder.cs
- DataGridBoolColumn.cs
- WindowsRichEdit.cs
- InternalTypeHelper.cs
- EntityDataSourceContainerNameItem.cs
- LogicalTreeHelper.cs
- RtfNavigator.cs
- AudioFormatConverter.cs
- ProcessModelInfo.cs
- FixedSOMTableRow.cs
- WebPartConnectionsDisconnectVerb.cs
- ExtendedPropertiesHandler.cs
- HuffCodec.cs
- DictionaryEntry.cs
- ResourceAttributes.cs
- PathFigureCollection.cs
- LeaseManager.cs
- CodeExpressionCollection.cs
- Messages.cs
- WindowPattern.cs
- SecurityPolicySection.cs
- TableHeaderCell.cs
- HTMLTagNameToTypeMapper.cs
- SecurityManager.cs
- BaseTemplateBuildProvider.cs
- MediaContextNotificationWindow.cs
- HScrollBar.cs
- FindCriteriaApril2005.cs
- ExpressionVisitorHelpers.cs
- DPTypeDescriptorContext.cs
- LinqDataSourceContextEventArgs.cs
- TraceXPathNavigator.cs
- XPathArrayIterator.cs
- DataShape.cs
- InstancePersistence.cs
- DataGridViewBand.cs
- CalendarAutomationPeer.cs
- XmlNullResolver.cs
- EventSourceCreationData.cs
- BrushConverter.cs
- CultureInfo.cs
- ResolveCriteriaApril2005.cs
- GridView.cs
- SqlGatherProducedAliases.cs
- SHA512Cng.cs
- RemotingConfiguration.cs
- Collection.cs
- PhysicalFontFamily.cs
- XmlDataLoader.cs
- WindowPattern.cs
- DynamicControl.cs
- SmtpFailedRecipientException.cs
- BaseDataBoundControl.cs
- ReflectionHelper.cs
- ProviderSettingsCollection.cs
- Command.cs
- _NegotiateClient.cs
- ADMembershipProvider.cs
- HotSpotCollection.cs
- XamlParser.cs
- ISO2022Encoding.cs
- SessionEndingEventArgs.cs
- Membership.cs
- FontFamilyConverter.cs
- TabletCollection.cs
- _UriSyntax.cs
- SystemResourceHost.cs
- CustomAttributeSerializer.cs
- _FtpDataStream.cs
- AuthorizationRuleCollection.cs
- BitmapFrameEncode.cs
- PolyBezierSegment.cs
- QilDataSource.cs
- DocComment.cs
- CqlLexerHelpers.cs
- CompiledRegexRunnerFactory.cs
- Subset.cs
- Separator.cs
- DataGridViewIntLinkedList.cs
- EntityContainerEntitySet.cs
- NativeMethods.cs
- WebPartTransformerCollection.cs
- ToolboxItemAttribute.cs
- ActivityCodeDomReferenceService.cs
- RawStylusInputCustomDataList.cs
- QuaternionAnimation.cs
- WorkflowMarkupSerializerMapping.cs
- AddValidationError.cs
- BinaryObjectWriter.cs
- PermissionListSet.cs
- WebPartCatalogCloseVerb.cs
- XamlReaderConstants.cs
- BridgeDataRecord.cs
- SspiHelper.cs
- VisualBrush.cs