Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / WaveHeader.cs / 1 / WaveHeader.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // This class defines the header used to identify a waveform-audio // buffer. // // with the CLR 607 // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.Synthesis.TtsEngine; namespace System.Speech.Internal.Synthesis { ////// /// internal sealed class WaveHeader : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Initialize an instance of a byte array. /// /// ///MMSYSERR.NOERROR if successful internal WaveHeader (byte [] buffer) { _dwBufferLength = buffer.Length; _gcHandle = GCHandle.Alloc (buffer, GCHandleType.Pinned); } ////// Frees any memory allocated for the buffer. /// ~WaveHeader () { Dispose (false); } ////// TODOC /// public void Dispose () { Dispose (true); GC.SuppressFinalize (this); } ////// Frees any memory allocated for the buffer. /// private void Dispose (bool disposing) { if (disposing) { ReleaseData (); if (_gcHandleWaveHdr.IsAllocated) { _gcHandleWaveHdr.Free (); } } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void ReleaseData () { if (_gcHandle.IsAllocated) { _gcHandle.Free (); } } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal GCHandle WAVEHDR { get { if (!_gcHandleWaveHdr.IsAllocated) { _waveHdr.lpData = _gcHandle.AddrOfPinnedObject (); _waveHdr.dwBufferLength = (uint) _dwBufferLength; _waveHdr.dwBytesRecorded = 0; _waveHdr.dwUser = 0; _waveHdr.dwFlags = 0; _waveHdr.dwLoops = 0; _waveHdr.lpNext = IntPtr.Zero; _gcHandleWaveHdr = GCHandle.Alloc (_waveHdr, GCHandleType.Pinned); } return _gcHandleWaveHdr; } } internal int SizeHDR { get { return Marshal.SizeOf (_waveHdr); } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************** #region Internal Fields ////// Used by dwFlags in WaveHeader /// Set by the device driver to indicate that it is finished with the buffer /// and is returning it to the application. /// internal const int WHDR_DONE = 0x00000001; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer has been prepared with the /// waveInPrepareHeader or waveOutPrepareHeader function. /// internal const int WHDR_PREPARED = 0x00000002; ////// Used by dwFlags in WaveHeader /// This buffer is the first buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_BEGINLOOP = 0x00000004; ////// Used by dwFlags in WaveHeader /// This buffer is the last buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_ENDLOOP = 0x00000008; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer is queued for playback. /// internal const int WHDR_INQUEUE = 0x00000010; ////// Set in WaveFormat.wFormatTag to specify PCM data. /// internal const int WAVE_FORMAT_PCM = 1; #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields ////// Long pointer to the address of the waveform buffer. This buffer must /// be block-aligned according to the nBlockAlign member of the /// WaveFormat structure used to open the device. /// private GCHandle _gcHandle = new GCHandle (); private GCHandle _gcHandleWaveHdr = new GCHandle (); private WAVEHDR _waveHdr = new WAVEHDR (); ////// Specifies the length, in bytes, of the buffer. /// internal int _dwBufferLength; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // This class defines the header used to identify a waveform-audio // buffer. // // with the CLR 607 // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.Synthesis.TtsEngine; namespace System.Speech.Internal.Synthesis { ////// /// internal sealed class WaveHeader : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Initialize an instance of a byte array. /// /// ///MMSYSERR.NOERROR if successful internal WaveHeader (byte [] buffer) { _dwBufferLength = buffer.Length; _gcHandle = GCHandle.Alloc (buffer, GCHandleType.Pinned); } ////// Frees any memory allocated for the buffer. /// ~WaveHeader () { Dispose (false); } ////// TODOC /// public void Dispose () { Dispose (true); GC.SuppressFinalize (this); } ////// Frees any memory allocated for the buffer. /// private void Dispose (bool disposing) { if (disposing) { ReleaseData (); if (_gcHandleWaveHdr.IsAllocated) { _gcHandleWaveHdr.Free (); } } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void ReleaseData () { if (_gcHandle.IsAllocated) { _gcHandle.Free (); } } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal GCHandle WAVEHDR { get { if (!_gcHandleWaveHdr.IsAllocated) { _waveHdr.lpData = _gcHandle.AddrOfPinnedObject (); _waveHdr.dwBufferLength = (uint) _dwBufferLength; _waveHdr.dwBytesRecorded = 0; _waveHdr.dwUser = 0; _waveHdr.dwFlags = 0; _waveHdr.dwLoops = 0; _waveHdr.lpNext = IntPtr.Zero; _gcHandleWaveHdr = GCHandle.Alloc (_waveHdr, GCHandleType.Pinned); } return _gcHandleWaveHdr; } } internal int SizeHDR { get { return Marshal.SizeOf (_waveHdr); } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************** #region Internal Fields ////// Used by dwFlags in WaveHeader /// Set by the device driver to indicate that it is finished with the buffer /// and is returning it to the application. /// internal const int WHDR_DONE = 0x00000001; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer has been prepared with the /// waveInPrepareHeader or waveOutPrepareHeader function. /// internal const int WHDR_PREPARED = 0x00000002; ////// Used by dwFlags in WaveHeader /// This buffer is the first buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_BEGINLOOP = 0x00000004; ////// Used by dwFlags in WaveHeader /// This buffer is the last buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_ENDLOOP = 0x00000008; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer is queued for playback. /// internal const int WHDR_INQUEUE = 0x00000010; ////// Set in WaveFormat.wFormatTag to specify PCM data. /// internal const int WAVE_FORMAT_PCM = 1; #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields ////// Long pointer to the address of the waveform buffer. This buffer must /// be block-aligned according to the nBlockAlign member of the /// WaveFormat structure used to open the device. /// private GCHandle _gcHandle = new GCHandle (); private GCHandle _gcHandleWaveHdr = new GCHandle (); private WAVEHDR _waveHdr = new WAVEHDR (); ////// Specifies the length, in bytes, of the buffer. /// internal int _dwBufferLength; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
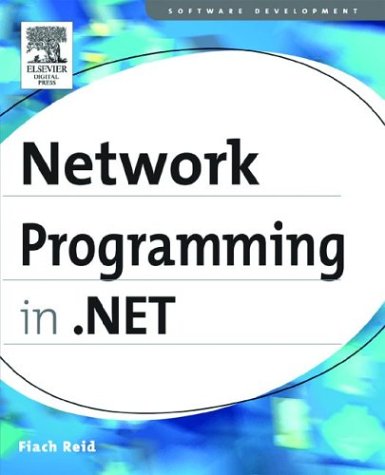
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeExporter.cs
- ConstructorNeedsTagAttribute.cs
- ClickablePoint.cs
- RegistrationContext.cs
- FullTrustAssembly.cs
- EditorPartChrome.cs
- ControlCachePolicy.cs
- OperationAbortedException.cs
- SerializationAttributes.cs
- SqlFacetAttribute.cs
- FileVersion.cs
- MsmqSecureHashAlgorithm.cs
- ListBox.cs
- TableLayoutColumnStyleCollection.cs
- RemotingAttributes.cs
- ToolStripItemDesigner.cs
- XmlBufferReader.cs
- FormatterConverter.cs
- DataGridViewRowPrePaintEventArgs.cs
- TextBox.cs
- XNameTypeConverter.cs
- DataListItemEventArgs.cs
- RemotingAttributes.cs
- Menu.cs
- UnsafeNativeMethods.cs
- EntitySqlQueryCacheEntry.cs
- NetPeerTcpBinding.cs
- ListViewItemMouseHoverEvent.cs
- PointAnimationBase.cs
- PerformanceCounterCategory.cs
- DataContractJsonSerializer.cs
- CaseExpr.cs
- UnwrappedTypesXmlSerializerManager.cs
- CopyAttributesAction.cs
- DataGridViewRowHeaderCell.cs
- StatusBarPanelClickEvent.cs
- DrawingAttributesDefaultValueFactory.cs
- XmlSchemaResource.cs
- FormViewDeleteEventArgs.cs
- CodeDefaultValueExpression.cs
- ToolstripProfessionalRenderer.cs
- CSharpCodeProvider.cs
- CatalogZone.cs
- InlineUIContainer.cs
- WebPartEditorCancelVerb.cs
- Pair.cs
- DbXmlEnabledProviderManifest.cs
- EditorBrowsableAttribute.cs
- HttpRequestBase.cs
- RegexCapture.cs
- UnsafeNativeMethods.cs
- KeyConstraint.cs
- ChoiceConverter.cs
- OperatingSystem.cs
- ButtonBaseAutomationPeer.cs
- UnionExpr.cs
- EncryptedKey.cs
- TraceProvider.cs
- Rect.cs
- JapaneseCalendar.cs
- SchemaSetCompiler.cs
- PasswordBox.cs
- Int32CAMarshaler.cs
- StoreItemCollection.Loader.cs
- DocumentsTrace.cs
- Figure.cs
- RepeaterItemCollection.cs
- Clause.cs
- X509IssuerSerialKeyIdentifierClause.cs
- ConfigurationElementCollection.cs
- ServicePointManager.cs
- ConfigurationSectionGroup.cs
- FixedHighlight.cs
- HttpHeaderCollection.cs
- PanelDesigner.cs
- FontDifferentiator.cs
- HttpListenerContext.cs
- WorkflowServiceBehavior.cs
- ExpressionReplacer.cs
- CultureSpecificStringDictionary.cs
- CompositeDispatchFormatter.cs
- DataBindingCollection.cs
- GifBitmapEncoder.cs
- Connector.cs
- RawStylusInput.cs
- PropertyMetadata.cs
- HtmlToClrEventProxy.cs
- NamespaceInfo.cs
- SessionStateContainer.cs
- UnsafeNativeMethods.cs
- BrowserTree.cs
- ToolStripStatusLabel.cs
- WebPartCollection.cs
- ObjectViewListener.cs
- StringFunctions.cs
- DTCTransactionManager.cs
- cache.cs
- Rfc2898DeriveBytes.cs
- FileRecordSequence.cs
- AdRotator.cs