Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / SiblingIterators.cs / 1 / SiblingIterators.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Diagnostics; using System.Collections; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Iterate over all following-sibling content nodes. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct FollowingSiblingIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent; ////// Initialize the FollowingSiblingIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.filter = filter; } ////// Position the iterator on the next following-sibling node. Return true if such a node exists and /// set Current property. Otherwise, return false (Current property is undefined). /// public bool MoveNext() { return this.filter.MoveToFollowingSibling(this.navCurrent); } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } ////// Iterate over child following-sibling nodes. This is a simple variation on the ContentMergeIterator, so use containment /// to reuse its code (can't use inheritance with structures). /// [EditorBrowsable(EditorBrowsableState.Never)] public struct FollowingSiblingMergeIterator { private ContentMergeIterator wrapped; ////// Initialize the FollowingSiblingMergeIterator. /// public void Create(XmlNavigatorFilter filter) { this.wrapped.Create(filter); } ////// Position this iterator to the next content or sibling node. Return IteratorResult.NoMoreNodes if there are /// no more content or sibling nodes. Return IteratorResult.NeedInputNode if the next input node needs to be /// fetched first. Return IteratorResult.HaveCurrent if the Current property is set to the next node in the /// iteration. /// public IteratorResult MoveNext(XPathNavigator navigator) { return this.wrapped.MoveNext(navigator, false); } ////// Return the current result navigator. This is only defined after MoveNext() has returned IteratorResult.HaveCurrent. /// public XPathNavigator Current { get { return this.wrapped.Current; } } } ////// Iterate over all preceding nodes according to XPath preceding axis rules, returning nodes in reverse /// document order. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct PrecedingSiblingIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent; ////// Initialize the PrecedingSiblingIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.filter = filter; } ////// Return true if the Current property is set to the next Preceding node in reverse document order. /// public bool MoveNext() { return this.filter.MoveToPreviousSibling(this.navCurrent); } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } ////// Iterate over all preceding-sibling content nodes in document order. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct PrecedingSiblingDocOrderIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent, navEnd; private bool needFirst, useCompPos; ////// Initialize the PrecedingSiblingDocOrderIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.filter = filter; this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.navEnd = XmlQueryRuntime.SyncToNavigator(this.navEnd, context); this.needFirst = true; // If the context node will be filtered out, then use ComparePosition to // determine when the context node has been passed by. Otherwise, IsSamePosition // is sufficient to determine when the context node has been reached. this.useCompPos = this.filter.IsFiltered(context); } ////// Position the iterator on the next preceding-sibling node. Return true if such a node exists and /// set Current property. Otherwise, return false (Current property is undefined). /// public bool MoveNext() { if (this.needFirst) { // Get first matching preceding-sibling node if (!this.navCurrent.MoveToParent()) return false; if (!this.filter.MoveToContent(this.navCurrent)) return false; this.needFirst = false; } else { // Get next matching preceding-sibling node if (!this.filter.MoveToFollowingSibling(this.navCurrent)) return false; } // Accept matching sibling only if it precedes navEnd in document order if (this.useCompPos) return (this.navCurrent.ComparePosition(this.navEnd) == XmlNodeOrder.Before); if (this.navCurrent.IsSamePosition(this.navEnd)) { // Found the original context node, so iteration is complete. If MoveNext // is called again, use ComparePosition so that false will continue to be // returned. this.useCompPos = true; return false; } return true; } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Diagnostics; using System.Collections; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Iterate over all following-sibling content nodes. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct FollowingSiblingIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent; ////// Initialize the FollowingSiblingIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.filter = filter; } ////// Position the iterator on the next following-sibling node. Return true if such a node exists and /// set Current property. Otherwise, return false (Current property is undefined). /// public bool MoveNext() { return this.filter.MoveToFollowingSibling(this.navCurrent); } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } ////// Iterate over child following-sibling nodes. This is a simple variation on the ContentMergeIterator, so use containment /// to reuse its code (can't use inheritance with structures). /// [EditorBrowsable(EditorBrowsableState.Never)] public struct FollowingSiblingMergeIterator { private ContentMergeIterator wrapped; ////// Initialize the FollowingSiblingMergeIterator. /// public void Create(XmlNavigatorFilter filter) { this.wrapped.Create(filter); } ////// Position this iterator to the next content or sibling node. Return IteratorResult.NoMoreNodes if there are /// no more content or sibling nodes. Return IteratorResult.NeedInputNode if the next input node needs to be /// fetched first. Return IteratorResult.HaveCurrent if the Current property is set to the next node in the /// iteration. /// public IteratorResult MoveNext(XPathNavigator navigator) { return this.wrapped.MoveNext(navigator, false); } ////// Return the current result navigator. This is only defined after MoveNext() has returned IteratorResult.HaveCurrent. /// public XPathNavigator Current { get { return this.wrapped.Current; } } } ////// Iterate over all preceding nodes according to XPath preceding axis rules, returning nodes in reverse /// document order. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct PrecedingSiblingIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent; ////// Initialize the PrecedingSiblingIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.filter = filter; } ////// Return true if the Current property is set to the next Preceding node in reverse document order. /// public bool MoveNext() { return this.filter.MoveToPreviousSibling(this.navCurrent); } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } ////// Iterate over all preceding-sibling content nodes in document order. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct PrecedingSiblingDocOrderIterator { private XmlNavigatorFilter filter; private XPathNavigator navCurrent, navEnd; private bool needFirst, useCompPos; ////// Initialize the PrecedingSiblingDocOrderIterator. /// public void Create(XPathNavigator context, XmlNavigatorFilter filter) { this.filter = filter; this.navCurrent = XmlQueryRuntime.SyncToNavigator(this.navCurrent, context); this.navEnd = XmlQueryRuntime.SyncToNavigator(this.navEnd, context); this.needFirst = true; // If the context node will be filtered out, then use ComparePosition to // determine when the context node has been passed by. Otherwise, IsSamePosition // is sufficient to determine when the context node has been reached. this.useCompPos = this.filter.IsFiltered(context); } ////// Position the iterator on the next preceding-sibling node. Return true if such a node exists and /// set Current property. Otherwise, return false (Current property is undefined). /// public bool MoveNext() { if (this.needFirst) { // Get first matching preceding-sibling node if (!this.navCurrent.MoveToParent()) return false; if (!this.filter.MoveToContent(this.navCurrent)) return false; this.needFirst = false; } else { // Get next matching preceding-sibling node if (!this.filter.MoveToFollowingSibling(this.navCurrent)) return false; } // Accept matching sibling only if it precedes navEnd in document order if (this.useCompPos) return (this.navCurrent.ComparePosition(this.navEnd) == XmlNodeOrder.Before); if (this.navCurrent.IsSamePosition(this.navEnd)) { // Found the original context node, so iteration is complete. If MoveNext // is called again, use ComparePosition so that false will continue to be // returned. this.useCompPos = true; return false; } return true; } ////// Return the current result navigator. This is only defined after MoveNext() has returned true. /// public XPathNavigator Current { get { return this.navCurrent; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
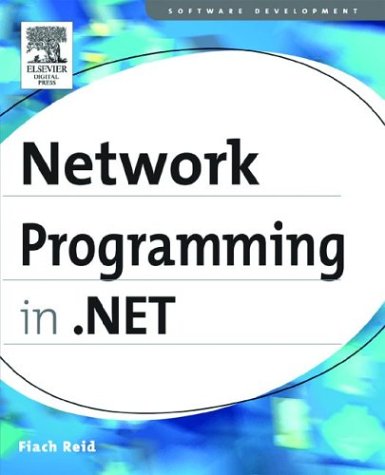
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FieldAccessException.cs
- Msec.cs
- RadioButton.cs
- ExtendLockAsyncResult.cs
- QilGeneratorEnv.cs
- SQLMoney.cs
- WindowProviderWrapper.cs
- DesignColumn.cs
- CodeSubDirectory.cs
- InputMethod.cs
- NotSupportedException.cs
- BufferBuilder.cs
- CacheMode.cs
- MDIClient.cs
- DataGridLinkButton.cs
- FileReader.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- XmlSerializerAssemblyAttribute.cs
- OdbcError.cs
- PageAsyncTask.cs
- RtfControlWordInfo.cs
- ModifierKeysValueSerializer.cs
- BaseCodePageEncoding.cs
- FileRecordSequence.cs
- ImageMapEventArgs.cs
- InputScopeConverter.cs
- EventData.cs
- EFTableProvider.cs
- ValueOfAction.cs
- MachineSettingsSection.cs
- CacheOutputQuery.cs
- NavigationProgressEventArgs.cs
- GroupedContextMenuStrip.cs
- dtdvalidator.cs
- Pair.cs
- DataPagerCommandEventArgs.cs
- WebChannelFactory.cs
- Method.cs
- SetterBase.cs
- XmlMapping.cs
- SecurityContext.cs
- VScrollProperties.cs
- updateconfighost.cs
- ObjectSpanRewriter.cs
- GC.cs
- WebMessageBodyStyleHelper.cs
- FrameworkTemplate.cs
- Pen.cs
- LocalizedNameDescriptionPair.cs
- IProvider.cs
- Translator.cs
- AuthenticateEventArgs.cs
- AdornedElementPlaceholder.cs
- precedingsibling.cs
- DbModificationCommandTree.cs
- MailWriter.cs
- WizardForm.cs
- Deflater.cs
- Figure.cs
- COM2AboutBoxPropertyDescriptor.cs
- TextRangeEditLists.cs
- DirectoryNotFoundException.cs
- AmbientProperties.cs
- ToolStripOverflow.cs
- AuthenticateEventArgs.cs
- RectConverter.cs
- StickyNote.cs
- WebPartTracker.cs
- OpenFileDialog.cs
- TreeNodeCollection.cs
- RowBinding.cs
- ComAdminWrapper.cs
- PropertyIDSet.cs
- SqlInfoMessageEvent.cs
- MemberRelationshipService.cs
- CodeStatementCollection.cs
- OleDbStruct.cs
- ValueTypeFixupInfo.cs
- LoadedOrUnloadedOperation.cs
- Facet.cs
- WebPermission.cs
- SqlTrackingWorkflowInstance.cs
- XmlSubtreeReader.cs
- SafeRightsManagementEnvironmentHandle.cs
- ConnectionsZoneDesigner.cs
- XsltException.cs
- ListViewItem.cs
- EntityDataSourceWrapperCollection.cs
- DataServiceOperationContext.cs
- Int32.cs
- ManagementInstaller.cs
- log.cs
- HebrewNumber.cs
- Helpers.cs
- PublisherIdentityPermission.cs
- PropVariant.cs
- VisualCollection.cs
- NativeMethods.cs
- WindowsFormsSectionHandler.cs
- ProtocolsConfigurationEntry.cs