Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Configuration / System / Configuration / ExceptionUtil.cs / 1 / ExceptionUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration.Internal; static internal class ExceptionUtil { static internal ArgumentException ParameterInvalid(string parameter) { return new ArgumentException(SR.GetString(SR.Parameter_Invalid, parameter), parameter); } static internal ArgumentException ParameterNullOrEmpty(string parameter) { return new ArgumentException(SR.GetString(SR.Parameter_NullOrEmpty, parameter), parameter); } static internal ArgumentException PropertyInvalid(string property) { return new ArgumentException(SR.GetString(SR.Property_Invalid, property), property); } static internal ArgumentException PropertyNullOrEmpty(string property) { return new ArgumentException(SR.GetString(SR.Property_NullOrEmpty, property), property); } static internal InvalidOperationException UnexpectedError(string methodName) { return new InvalidOperationException(SR.GetString(SR.Unexpected_Error, methodName)); } static internal string NoExceptionInformation { get { return SR.GetString(SR.No_exception_information_available); } } static internal ConfigurationErrorsException WrapAsConfigException(string outerMessage, Exception e, IConfigErrorInfo errorInfo) { if (errorInfo != null) { return WrapAsConfigException(outerMessage, e, errorInfo.Filename, errorInfo.LineNumber); } else { return WrapAsConfigException(outerMessage, e, null, 0); } } static internal ConfigurationErrorsException WrapAsConfigException(string outerMessage, Exception e, string filename, int line) { // // Preserve ConfigurationErrorsException // ConfigurationErrorsException ce = e as ConfigurationErrorsException; if (ce != null) { return ce; } // // Promote deprecated ConfigurationException to ConfigurationErrorsException // ConfigurationException deprecatedException = e as ConfigurationException; if (deprecatedException != null) { return new ConfigurationErrorsException(deprecatedException); } // // For XML exceptions, preserve the text of the exception in the outer message. // XmlException xe = e as XmlException; if (xe != null) { if (xe.LineNumber != 0) { line = xe.LineNumber; } return new ConfigurationErrorsException(xe.Message, xe, filename, line); } // // Wrap other exceptions in an inner exception, and give as much info as possible // if (e != null) { return new ConfigurationErrorsException( SR.GetString(SR.Wrapped_exception_message, outerMessage, e.Message), e, filename, line); } // // If there is no exception, create a new exception with no further information. // return new ConfigurationErrorsException( SR.GetString(SR.Wrapped_exception_message, outerMessage, ExceptionUtil.NoExceptionInformation), filename, line); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration.Internal; static internal class ExceptionUtil { static internal ArgumentException ParameterInvalid(string parameter) { return new ArgumentException(SR.GetString(SR.Parameter_Invalid, parameter), parameter); } static internal ArgumentException ParameterNullOrEmpty(string parameter) { return new ArgumentException(SR.GetString(SR.Parameter_NullOrEmpty, parameter), parameter); } static internal ArgumentException PropertyInvalid(string property) { return new ArgumentException(SR.GetString(SR.Property_Invalid, property), property); } static internal ArgumentException PropertyNullOrEmpty(string property) { return new ArgumentException(SR.GetString(SR.Property_NullOrEmpty, property), property); } static internal InvalidOperationException UnexpectedError(string methodName) { return new InvalidOperationException(SR.GetString(SR.Unexpected_Error, methodName)); } static internal string NoExceptionInformation { get { return SR.GetString(SR.No_exception_information_available); } } static internal ConfigurationErrorsException WrapAsConfigException(string outerMessage, Exception e, IConfigErrorInfo errorInfo) { if (errorInfo != null) { return WrapAsConfigException(outerMessage, e, errorInfo.Filename, errorInfo.LineNumber); } else { return WrapAsConfigException(outerMessage, e, null, 0); } } static internal ConfigurationErrorsException WrapAsConfigException(string outerMessage, Exception e, string filename, int line) { // // Preserve ConfigurationErrorsException // ConfigurationErrorsException ce = e as ConfigurationErrorsException; if (ce != null) { return ce; } // // Promote deprecated ConfigurationException to ConfigurationErrorsException // ConfigurationException deprecatedException = e as ConfigurationException; if (deprecatedException != null) { return new ConfigurationErrorsException(deprecatedException); } // // For XML exceptions, preserve the text of the exception in the outer message. // XmlException xe = e as XmlException; if (xe != null) { if (xe.LineNumber != 0) { line = xe.LineNumber; } return new ConfigurationErrorsException(xe.Message, xe, filename, line); } // // Wrap other exceptions in an inner exception, and give as much info as possible // if (e != null) { return new ConfigurationErrorsException( SR.GetString(SR.Wrapped_exception_message, outerMessage, e.Message), e, filename, line); } // // If there is no exception, create a new exception with no further information. // return new ConfigurationErrorsException( SR.GetString(SR.Wrapped_exception_message, outerMessage, ExceptionUtil.NoExceptionInformation), filename, line); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
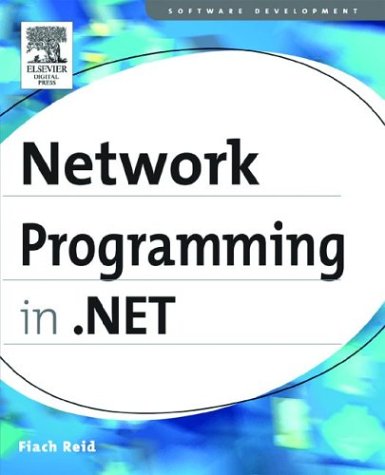
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeferredElementTreeState.cs
- cookieexception.cs
- ButtonFlatAdapter.cs
- CounterCreationData.cs
- RadioButtonStandardAdapter.cs
- ServiceDescriptionImporter.cs
- MediaTimeline.cs
- DragCompletedEventArgs.cs
- AspNetPartialTrustHelpers.cs
- NetworkInformationException.cs
- AutoCompleteStringCollection.cs
- DataSourceControl.cs
- Material.cs
- PeerMaintainer.cs
- ApplicationGesture.cs
- ComplexObject.cs
- QilCloneVisitor.cs
- EmbossBitmapEffect.cs
- IgnorePropertiesAttribute.cs
- ProcessHostServerConfig.cs
- BufferModeSettings.cs
- Validator.cs
- NoResizeSelectionBorderGlyph.cs
- FontConverter.cs
- InfiniteTimeSpanConverter.cs
- SystemWebExtensionsSectionGroup.cs
- SqlConnectionString.cs
- _ListenerAsyncResult.cs
- TreeViewTemplateSelector.cs
- ToolStripDropDownClosingEventArgs.cs
- ActivityWithResultValueSerializer.cs
- AppSecurityManager.cs
- WeakRefEnumerator.cs
- Columns.cs
- Base64Encoder.cs
- DelegateBodyWriter.cs
- Camera.cs
- ReferenceEqualityComparer.cs
- UnsafeNativeMethodsMilCoreApi.cs
- ContainerSelectorBehavior.cs
- WindowsNonControl.cs
- ConstraintStruct.cs
- IntAverageAggregationOperator.cs
- CustomLineCap.cs
- TransformerConfigurationWizardBase.cs
- DiffuseMaterial.cs
- TransportReplyChannelAcceptor.cs
- DataPagerCommandEventArgs.cs
- DirectionalLight.cs
- TrackingProfileDeserializationException.cs
- Tuple.cs
- ErrorWebPart.cs
- CapabilitiesUse.cs
- GifBitmapDecoder.cs
- RegexWorker.cs
- DataGridGeneralPage.cs
- FigureParaClient.cs
- EntityFrameworkVersions.cs
- UserUseLicenseDictionaryLoader.cs
- HttpRequestBase.cs
- HandlerBase.cs
- WindowsTab.cs
- Utils.cs
- XsltQilFactory.cs
- EndpointDiscoveryMetadata11.cs
- HyperLinkField.cs
- TextRunCache.cs
- CompressedStack.cs
- SqlExpander.cs
- PeerToPeerException.cs
- MemberPath.cs
- DrawingContextWalker.cs
- BezierSegment.cs
- XmlReflectionImporter.cs
- EngineSiteSapi.cs
- DataGridViewUtilities.cs
- COM2ExtendedTypeConverter.cs
- InputProcessorProfiles.cs
- XPathItem.cs
- GlyphElement.cs
- TextFragmentEngine.cs
- OleDbConnectionInternal.cs
- OperationCanceledException.cs
- ProgressBar.cs
- DeferredTextReference.cs
- WindowsScrollBar.cs
- LayoutTable.cs
- SqlDataSourceView.cs
- CommandSet.cs
- XmlParserContext.cs
- ObjectItemCachedAssemblyLoader.cs
- ParallelEnumerableWrapper.cs
- DataGridViewLinkCell.cs
- DialogWindow.cs
- LambdaCompiler.Unary.cs
- SimpleMailWebEventProvider.cs
- SymbolMethod.cs
- Int64Animation.cs
- XmlChildNodes.cs
- MsmqIntegrationElement.cs