Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Runtime / CompilerServices / AssemblySettingAttributes.cs / 1 / AssemblySettingAttributes.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //////////////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////////////// namespace System.Runtime.CompilerServices { using System; using System.Runtime.InteropServices; /* NGenHint is not supported in Whidbey [Serializable] public enum NGenHint { Default = 0x0000, // No preference specified Eager = 0x0001, // NGen at install time Lazy = 0x0002, // NGen after install time Never = 0x0003, // Assembly should not be ngened } */ [Serializable] public enum LoadHint { Default = 0x0000, // No preference specified Always = 0x0001, // Dependency is always loaded Sometimes = 0x0002, // Dependency is sometimes loaded //Never = 0x0003, // Dependency is never loaded } [Serializable, AttributeUsage(AttributeTargets.Assembly)] public sealed class DefaultDependencyAttribute : Attribute { private LoadHint loadHint; public DefaultDependencyAttribute ( LoadHint loadHintArgument ) { loadHint = loadHintArgument; } public LoadHint LoadHint { get { return loadHint; } } } [Serializable, AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)] public sealed class DependencyAttribute : Attribute { private String dependentAssembly; private LoadHint loadHint; public DependencyAttribute ( String dependentAssemblyArgument, LoadHint loadHintArgument ) { dependentAssembly = dependentAssemblyArgument; loadHint = loadHintArgument; } public String DependentAssembly { get { return dependentAssembly; } } public LoadHint LoadHint { get { return loadHint; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //////////////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////////////// namespace System.Runtime.CompilerServices { using System; using System.Runtime.InteropServices; /* NGenHint is not supported in Whidbey [Serializable] public enum NGenHint { Default = 0x0000, // No preference specified Eager = 0x0001, // NGen at install time Lazy = 0x0002, // NGen after install time Never = 0x0003, // Assembly should not be ngened } */ [Serializable] public enum LoadHint { Default = 0x0000, // No preference specified Always = 0x0001, // Dependency is always loaded Sometimes = 0x0002, // Dependency is sometimes loaded //Never = 0x0003, // Dependency is never loaded } [Serializable, AttributeUsage(AttributeTargets.Assembly)] public sealed class DefaultDependencyAttribute : Attribute { private LoadHint loadHint; public DefaultDependencyAttribute ( LoadHint loadHintArgument ) { loadHint = loadHintArgument; } public LoadHint LoadHint { get { return loadHint; } } } [Serializable, AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)] public sealed class DependencyAttribute : Attribute { private String dependentAssembly; private LoadHint loadHint; public DependencyAttribute ( String dependentAssemblyArgument, LoadHint loadHintArgument ) { dependentAssembly = dependentAssemblyArgument; loadHint = loadHintArgument; } public String DependentAssembly { get { return dependentAssembly; } } public LoadHint LoadHint { get { return loadHint; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
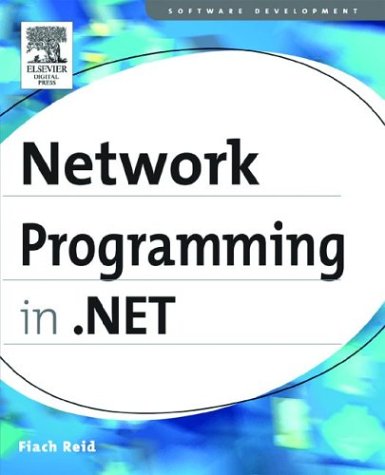
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FlowDocumentView.cs
- EntityDescriptor.cs
- Transactions.cs
- XmlEventCache.cs
- SessionStateUtil.cs
- EdmComplexTypeAttribute.cs
- DBSqlParserTableCollection.cs
- TableLayoutPanelCellPosition.cs
- HtmlShim.cs
- ListView.cs
- _HeaderInfoTable.cs
- ClientUtils.cs
- UInt16.cs
- ErrorRuntimeConfig.cs
- SettingsAttributes.cs
- XmlLangPropertyAttribute.cs
- CommandID.cs
- SettingsPropertyValueCollection.cs
- Line.cs
- Rotation3DAnimationBase.cs
- ChineseLunisolarCalendar.cs
- MetadataItem_Static.cs
- ConnectionPoolManager.cs
- x509utils.cs
- PathSegment.cs
- safemediahandle.cs
- RotationValidation.cs
- DependencyPropertyChangedEventArgs.cs
- BamlReader.cs
- UnmanagedBitmapWrapper.cs
- SiteMapNode.cs
- PropertyItemInternal.cs
- AnyReturnReader.cs
- AppDomainManager.cs
- Shape.cs
- UnsafeNativeMethods.cs
- CanonicalFormWriter.cs
- CultureTableRecord.cs
- FormatterConverter.cs
- MessageBox.cs
- XsdBuilder.cs
- Point3D.cs
- StackBuilderSink.cs
- SerialPort.cs
- XPathNavigator.cs
- UiaCoreApi.cs
- EntitySqlException.cs
- OrderedHashRepartitionEnumerator.cs
- AncestorChangedEventArgs.cs
- JsonReader.cs
- CommentAction.cs
- CounterCreationData.cs
- IChannel.cs
- unitconverter.cs
- TableItemPatternIdentifiers.cs
- ZoneLinkButton.cs
- TextServicesDisplayAttributePropertyRanges.cs
- QueryUtil.cs
- MouseGestureValueSerializer.cs
- SmiMetaDataProperty.cs
- TreeView.cs
- SelectedCellsChangedEventArgs.cs
- PrintPageEvent.cs
- MailHeaderInfo.cs
- DecoderBestFitFallback.cs
- ConnectionManagementSection.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- PositiveTimeSpanValidator.cs
- TypeElement.cs
- ViewGenResults.cs
- ButtonFlatAdapter.cs
- OneWayChannelFactory.cs
- ClientTarget.cs
- SHA1.cs
- ForwardPositionQuery.cs
- BuildResult.cs
- VerificationException.cs
- WorkItem.cs
- KeyedHashAlgorithm.cs
- XmlAttributeOverrides.cs
- HtmlAnchor.cs
- Module.cs
- DataGridItemCollection.cs
- SqlFacetAttribute.cs
- FormClosedEvent.cs
- UnmanagedMemoryStream.cs
- PersonalizationProviderHelper.cs
- SpellerHighlightLayer.cs
- ServiceRoute.cs
- DetailsViewRowCollection.cs
- ParserContext.cs
- XmlnsDictionary.cs
- XmlSchemaValidationException.cs
- List.cs
- RubberbandSelector.cs
- AdjustableArrowCap.cs
- PolyLineSegment.cs
- CanExecuteRoutedEventArgs.cs
- XslTransform.cs
- ZipArchive.cs