Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationTypes / System / Windows / Automation / WindowPatternIdentifiers.cs / 1 / WindowPatternIdentifiers.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Automation Identifiers for Window Pattern // // History: // 04/15/2005 : MKarr Added // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { // Disable warning for obsolete types. These are scheduled to be removed in M8.2 so // only need the warning to come out for components outside of APT. #pragma warning disable 0618 ////// following the Office and HTML definition of WindowState. /// [ComVisible(true)] [Guid("fdc8f176-aed2-477a-8c89-ea04cc5f278d")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum WindowVisualState #else public enum WindowVisualState #endif { ///window is normal Normal, ///window is maximized Maximized, ///window is minimized Minimized } ////// The current state of the window for user interaction /// [ComVisible(true)] [Guid("65101cc7-7904-408e-87a7-8c6dbd83a18b")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum WindowInteractionState #else public enum WindowInteractionState #endif { ////// window is running. This does not guarantee that the window ready for user interaction, /// nor does it guarantee the windows is not "not responding". /// Running, ///window is closing Closing, ///window is ready for the user to interact with it ReadyForUserInteraction, ///window is block by a modal window. BlockedByModalWindow, ///window is not responding NotResponding } ///wrapper class for Window pattern #if (INTERNAL_COMPILE) internal static class WindowPatternIdentifiers #else public static class WindowPatternIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Returns the Window pattern identifier public static readonly AutomationPattern Pattern = AutomationPattern.Register(AutomationIdentifierGuids.Window_Pattern, "WindowPatternIdentifiers.Pattern"); ///Property ID: CanMaximize - public static readonly AutomationProperty CanMaximizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_CanMaximize_Property, "WindowPatternIdentifiers.CanMaximizeProperty"); ///Property ID: CanMinimize - public static readonly AutomationProperty CanMinimizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_CanMinimize_Property, "WindowPatternIdentifiers.CanMinimizeProperty"); ///Property ID: IsModal - Is this is a modal window public static readonly AutomationProperty IsModalProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_IsModal_Property, "WindowPatternIdentifiers.IsModalProperty"); ///Property ID: WindowVisualState - Is the Window Maximized, Minimized, or Normal (aka restored) public static readonly AutomationProperty WindowVisualStateProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_VisualState_Property, "WindowPatternIdentifiers.WindowVisualStateProperty"); ///Property ID: WindowInteractionState - Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. public static readonly AutomationProperty WindowInteractionStateProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_InteractionState_Property, "WindowPatternIdentifiers.WindowInteractionStateProperty"); ///Property ID: - This window is always on top public static readonly AutomationProperty IsTopmostProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_IsTopmost_Property, "WindowPatternIdentifiers.IsTopmostProperty"); ///Event ID: WindowOpened - Immediately after opening the window - ApplicationWindows or Window Status is not guarantee to be: ReadyForUserInteraction public static readonly AutomationEvent WindowOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.Window_Opened_Event, "WindowPatternIdentifiers.WindowOpenedProperty"); ///Event ID: WindowClosed - Immediately after closing the window public static readonly AutomationEvent WindowClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.Window_Closed_Event, "WindowPatternIdentifiers.WindowClosedProperty"); #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Automation Identifiers for Window Pattern // // History: // 04/15/2005 : MKarr Added // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { // Disable warning for obsolete types. These are scheduled to be removed in M8.2 so // only need the warning to come out for components outside of APT. #pragma warning disable 0618 ////// following the Office and HTML definition of WindowState. /// [ComVisible(true)] [Guid("fdc8f176-aed2-477a-8c89-ea04cc5f278d")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum WindowVisualState #else public enum WindowVisualState #endif { ///window is normal Normal, ///window is maximized Maximized, ///window is minimized Minimized } ////// The current state of the window for user interaction /// [ComVisible(true)] [Guid("65101cc7-7904-408e-87a7-8c6dbd83a18b")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum WindowInteractionState #else public enum WindowInteractionState #endif { ////// window is running. This does not guarantee that the window ready for user interaction, /// nor does it guarantee the windows is not "not responding". /// Running, ///window is closing Closing, ///window is ready for the user to interact with it ReadyForUserInteraction, ///window is block by a modal window. BlockedByModalWindow, ///window is not responding NotResponding } ///wrapper class for Window pattern #if (INTERNAL_COMPILE) internal static class WindowPatternIdentifiers #else public static class WindowPatternIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Returns the Window pattern identifier public static readonly AutomationPattern Pattern = AutomationPattern.Register(AutomationIdentifierGuids.Window_Pattern, "WindowPatternIdentifiers.Pattern"); ///Property ID: CanMaximize - public static readonly AutomationProperty CanMaximizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_CanMaximize_Property, "WindowPatternIdentifiers.CanMaximizeProperty"); ///Property ID: CanMinimize - public static readonly AutomationProperty CanMinimizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_CanMinimize_Property, "WindowPatternIdentifiers.CanMinimizeProperty"); ///Property ID: IsModal - Is this is a modal window public static readonly AutomationProperty IsModalProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_IsModal_Property, "WindowPatternIdentifiers.IsModalProperty"); ///Property ID: WindowVisualState - Is the Window Maximized, Minimized, or Normal (aka restored) public static readonly AutomationProperty WindowVisualStateProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_VisualState_Property, "WindowPatternIdentifiers.WindowVisualStateProperty"); ///Property ID: WindowInteractionState - Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. public static readonly AutomationProperty WindowInteractionStateProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_InteractionState_Property, "WindowPatternIdentifiers.WindowInteractionStateProperty"); ///Property ID: - This window is always on top public static readonly AutomationProperty IsTopmostProperty = AutomationProperty.Register(AutomationIdentifierGuids.Window_IsTopmost_Property, "WindowPatternIdentifiers.IsTopmostProperty"); ///Event ID: WindowOpened - Immediately after opening the window - ApplicationWindows or Window Status is not guarantee to be: ReadyForUserInteraction public static readonly AutomationEvent WindowOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.Window_Opened_Event, "WindowPatternIdentifiers.WindowOpenedProperty"); ///Event ID: WindowClosed - Immediately after closing the window public static readonly AutomationEvent WindowClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.Window_Closed_Event, "WindowPatternIdentifiers.WindowClosedProperty"); #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
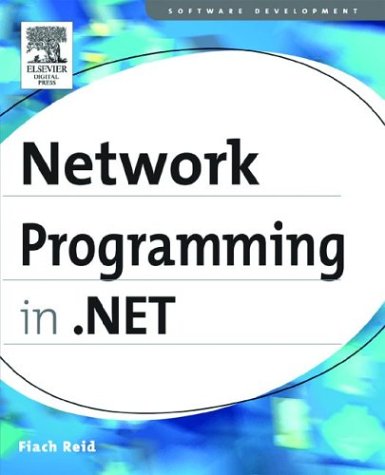
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SizeIndependentAnimationStorage.cs
- LineUtil.cs
- AttributeUsageAttribute.cs
- PersistenceMetadataNamespace.cs
- WindowHideOrCloseTracker.cs
- RestClientProxyHandler.cs
- XmlDownloadManager.cs
- ApplicationInterop.cs
- Point4DValueSerializer.cs
- _LocalDataStore.cs
- DeleteBookmarkScope.cs
- EntryWrittenEventArgs.cs
- SearchForVirtualItemEventArgs.cs
- StrokeDescriptor.cs
- ToolStripGrip.cs
- DbProviderFactory.cs
- InkPresenter.cs
- TemplateColumn.cs
- _emptywebproxy.cs
- SecurityPermission.cs
- VectorCollectionValueSerializer.cs
- WebMessageEncodingBindingElement.cs
- RenderDataDrawingContext.cs
- AdjustableArrowCap.cs
- EntityContainerEmitter.cs
- DataGridViewRowHeaderCell.cs
- GenerateTemporaryTargetAssembly.cs
- StickyNoteAnnotations.cs
- RayMeshGeometry3DHitTestResult.cs
- FontFaceLayoutInfo.cs
- TrackingDataItem.cs
- InvokeWebServiceDesigner.cs
- UnmanagedMemoryStreamWrapper.cs
- FlowLayoutPanel.cs
- DataChangedEventManager.cs
- XPathNodeHelper.cs
- PageTheme.cs
- ObfuscateAssemblyAttribute.cs
- MasterPageParser.cs
- FileDialog.cs
- TextEditorLists.cs
- StringSorter.cs
- PKCS1MaskGenerationMethod.cs
- ErrorTableItemStyle.cs
- mansign.cs
- StorageSetMapping.cs
- InfoCardRSACryptoProvider.cs
- versioninfo.cs
- SafeUserTokenHandle.cs
- RtType.cs
- Site.cs
- ExtensionQuery.cs
- Cloud.cs
- Effect.cs
- SpanIndex.cs
- Int64KeyFrameCollection.cs
- RewritingPass.cs
- RightNameExpirationInfoPair.cs
- SpecialNameAttribute.cs
- ResourceIDHelper.cs
- FastEncoder.cs
- TextChange.cs
- remotingproxy.cs
- oledbconnectionstring.cs
- Msmq4SubqueuePoisonHandler.cs
- querybuilder.cs
- GlyphRunDrawing.cs
- ToolStripDropDownButton.cs
- Site.cs
- VolatileEnlistmentMultiplexing.cs
- Image.cs
- XmlParserContext.cs
- AddingNewEventArgs.cs
- KnownTypes.cs
- LongValidatorAttribute.cs
- ActivityTrace.cs
- RealProxy.cs
- ColorInterpolationModeValidation.cs
- EncoderParameters.cs
- SQLStringStorage.cs
- FlowDecision.cs
- TemplatedAdorner.cs
- NegatedConstant.cs
- ObjectSecurity.cs
- EditorAttribute.cs
- ApplicationManager.cs
- ErrorWebPart.cs
- XmlSchemaNotation.cs
- IgnoreSectionHandler.cs
- ComMethodElementCollection.cs
- SamlAssertionKeyIdentifierClause.cs
- DataColumnCollection.cs
- PeerNameRecord.cs
- ConfigXmlAttribute.cs
- _ConnectOverlappedAsyncResult.cs
- SerialPinChanges.cs
- XmlSortKeyAccumulator.cs
- HwndSourceParameters.cs
- ClientScriptManagerWrapper.cs
- Object.cs