Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / TextEditorParagraphs.cs / 1 / TextEditorParagraphs.cs
//---------------------------------------------------------------------------- // // File: TextEditorParagraphs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A component of TextEditor supporting paragraph formating commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorParagraphs { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool acceptsRichContent, bool registerEventListeners) { CanExecuteRoutedEventHandler onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); if (acceptsRichContent) { // Editing Commands: Paragraph Editing // ----------------------------------- CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignLeft , new ExecutedRoutedEventHandler(OnAlignLeft) , onQueryStatusNYI, SRID.KeyAlignLeft, SRID.KeyAlignLeftDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignCenter , new ExecutedRoutedEventHandler(OnAlignCenter) , onQueryStatusNYI, SRID.KeyAlignCenter, SRID.KeyAlignCenterDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignRight , new ExecutedRoutedEventHandler(OnAlignRight) , onQueryStatusNYI, SRID.KeyAlignRight, SRID.KeyAlignRightDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignJustify , new ExecutedRoutedEventHandler(OnAlignJustify) , onQueryStatusNYI, SRID.KeyAlignJustify, SRID.KeyAlignJustifyDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplySingleSpace , new ExecutedRoutedEventHandler(OnApplySingleSpace) , onQueryStatusNYI, SRID.KeyApplySingleSpace, SRID.KeyApplySingleSpaceDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyOneAndAHalfSpace , new ExecutedRoutedEventHandler(OnApplyOneAndAHalfSpace) , onQueryStatusNYI, SRID.KeyApplyOneAndAHalfSpace, SRID.KeyApplyOneAndAHalfSpaceDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyDoubleSpace , new ExecutedRoutedEventHandler(OnApplyDoubleSpace) , onQueryStatusNYI, SRID.KeyApplyDoubleSpace, SRID.KeyApplyDoubleSpaceDisplayString ); } CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyParagraphFlowDirectionLTR, new ExecutedRoutedEventHandler(OnApplyParagraphFlowDirectionLTR), onQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyParagraphFlowDirectionRTL, new ExecutedRoutedEventHandler(OnApplyParagraphFlowDirectionRTL), onQueryStatusNYI); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// AlignLeft command event handler. /// private static void OnAlignLeft(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Left, /*applyToParagraphs*/true); } ////// AlignCenter command event handler. /// private static void OnAlignCenter(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Center, /*applyToParagraphs*/true); } ////// AlignRight command event handler. /// private static void OnAlignRight(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Right, /*applyToParagraphs*/true); } ////// AlignJustify command event handler. /// private static void OnAlignJustify(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Justify, /*applyToParagraphs*/true); } private static void OnApplySingleSpace(object sender, ExecutedRoutedEventArgs e) { // } private static void OnApplyOneAndAHalfSpace(object sender, ExecutedRoutedEventArgs e) { // } private static void OnApplyDoubleSpace(object sender, ExecutedRoutedEventArgs e) { // } ////// OnApplyParagraphFlowDirectionLTR command event handler. /// private static void OnApplyParagraphFlowDirectionLTR(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); TextEditorCharacters._OnApplyProperty(This, FrameworkElement.FlowDirectionProperty, FlowDirection.LeftToRight, /*applyToParagraphs*/true); } ////// OnApplyParagraphFlowDirectionRTL command event handler. /// private static void OnApplyParagraphFlowDirectionRTL(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); TextEditorCharacters._OnApplyProperty(This, FrameworkElement.FlowDirectionProperty, FlowDirection.RightToLeft, /*applyToParagraphs*/true); } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object sender, CanExecuteRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } e.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextEditorParagraphs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A component of TextEditor supporting paragraph formating commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorParagraphs { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool acceptsRichContent, bool registerEventListeners) { CanExecuteRoutedEventHandler onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); if (acceptsRichContent) { // Editing Commands: Paragraph Editing // ----------------------------------- CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignLeft , new ExecutedRoutedEventHandler(OnAlignLeft) , onQueryStatusNYI, SRID.KeyAlignLeft, SRID.KeyAlignLeftDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignCenter , new ExecutedRoutedEventHandler(OnAlignCenter) , onQueryStatusNYI, SRID.KeyAlignCenter, SRID.KeyAlignCenterDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignRight , new ExecutedRoutedEventHandler(OnAlignRight) , onQueryStatusNYI, SRID.KeyAlignRight, SRID.KeyAlignRightDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.AlignJustify , new ExecutedRoutedEventHandler(OnAlignJustify) , onQueryStatusNYI, SRID.KeyAlignJustify, SRID.KeyAlignJustifyDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplySingleSpace , new ExecutedRoutedEventHandler(OnApplySingleSpace) , onQueryStatusNYI, SRID.KeyApplySingleSpace, SRID.KeyApplySingleSpaceDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyOneAndAHalfSpace , new ExecutedRoutedEventHandler(OnApplyOneAndAHalfSpace) , onQueryStatusNYI, SRID.KeyApplyOneAndAHalfSpace, SRID.KeyApplyOneAndAHalfSpaceDisplayString ); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyDoubleSpace , new ExecutedRoutedEventHandler(OnApplyDoubleSpace) , onQueryStatusNYI, SRID.KeyApplyDoubleSpace, SRID.KeyApplyDoubleSpaceDisplayString ); } CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyParagraphFlowDirectionLTR, new ExecutedRoutedEventHandler(OnApplyParagraphFlowDirectionLTR), onQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.ApplyParagraphFlowDirectionRTL, new ExecutedRoutedEventHandler(OnApplyParagraphFlowDirectionRTL), onQueryStatusNYI); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// AlignLeft command event handler. /// private static void OnAlignLeft(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Left, /*applyToParagraphs*/true); } ////// AlignCenter command event handler. /// private static void OnAlignCenter(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Center, /*applyToParagraphs*/true); } ////// AlignRight command event handler. /// private static void OnAlignRight(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Right, /*applyToParagraphs*/true); } ////// AlignJustify command event handler. /// private static void OnAlignJustify(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } TextEditorCharacters._OnApplyProperty(This, Block.TextAlignmentProperty, TextAlignment.Justify, /*applyToParagraphs*/true); } private static void OnApplySingleSpace(object sender, ExecutedRoutedEventArgs e) { // } private static void OnApplyOneAndAHalfSpace(object sender, ExecutedRoutedEventArgs e) { // } private static void OnApplyDoubleSpace(object sender, ExecutedRoutedEventArgs e) { // } ////// OnApplyParagraphFlowDirectionLTR command event handler. /// private static void OnApplyParagraphFlowDirectionLTR(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); TextEditorCharacters._OnApplyProperty(This, FrameworkElement.FlowDirectionProperty, FlowDirection.LeftToRight, /*applyToParagraphs*/true); } ////// OnApplyParagraphFlowDirectionRTL command event handler. /// private static void OnApplyParagraphFlowDirectionRTL(object sender, ExecutedRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); TextEditorCharacters._OnApplyProperty(This, FrameworkElement.FlowDirectionProperty, FlowDirection.RightToLeft, /*applyToParagraphs*/true); } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object sender, CanExecuteRoutedEventArgs e) { TextEditor This = TextEditor._GetTextEditor(sender); if (This == null) { return; } e.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
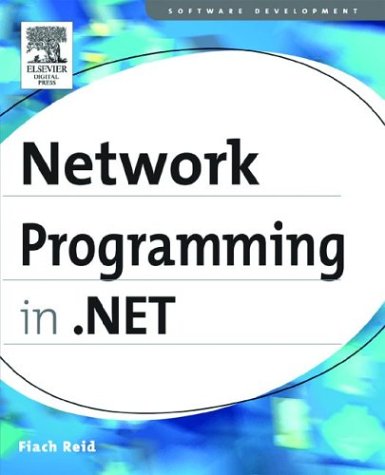
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImportRequest.cs
- xsdvalidator.cs
- AuthorizationContext.cs
- ServiceNotStartedException.cs
- brushes.cs
- CustomError.cs
- EncodingDataItem.cs
- DataGridViewRowPrePaintEventArgs.cs
- autovalidator.cs
- ModuleElement.cs
- SiteIdentityPermission.cs
- CriticalHandle.cs
- FileLoadException.cs
- PathFigure.cs
- SamlSecurityToken.cs
- UIElementParagraph.cs
- DetailsViewPagerRow.cs
- MenuItem.cs
- TreeViewCancelEvent.cs
- AppDomainShutdownMonitor.cs
- LocatorBase.cs
- Converter.cs
- TraceListener.cs
- ScopedKnownTypes.cs
- WebResponse.cs
- X509CertificateChain.cs
- GenericXmlSecurityToken.cs
- ConfigurationLockCollection.cs
- TriggerCollection.cs
- ConfigXmlCDataSection.cs
- COAUTHINFO.cs
- ServicesExceptionNotHandledEventArgs.cs
- Tablet.cs
- ResourceReferenceExpressionConverter.cs
- ArgumentsParser.cs
- DnsPermission.cs
- NonClientArea.cs
- WebColorConverter.cs
- SqlGenerator.cs
- CrossContextChannel.cs
- TextTreeTextElementNode.cs
- SqlCommandSet.cs
- HyperLinkColumn.cs
- ReferencedType.cs
- DataGridPagingPage.cs
- cookiecontainer.cs
- DbProviderFactory.cs
- ObjectStateFormatter.cs
- MonikerHelper.cs
- RootCodeDomSerializer.cs
- LifetimeServices.cs
- MemberRelationshipService.cs
- LoadWorkflowByKeyAsyncResult.cs
- GregorianCalendarHelper.cs
- PageBuildProvider.cs
- Events.cs
- MessagePartSpecification.cs
- OleTxTransactionInfo.cs
- DataPagerField.cs
- BaseParser.cs
- RecommendedAsConfigurableAttribute.cs
- EmptyEnumerator.cs
- LogPolicy.cs
- HttpsHostedTransportConfiguration.cs
- MultiDataTrigger.cs
- mil_sdk_version.cs
- LexicalChunk.cs
- LassoSelectionBehavior.cs
- CssTextWriter.cs
- DataGridViewDataErrorEventArgs.cs
- QuotedPrintableStream.cs
- AppearanceEditorPart.cs
- SharedConnectionInfo.cs
- ParameterBuilder.cs
- VisualStyleInformation.cs
- BinaryNode.cs
- ExternalException.cs
- BuiltInPermissionSets.cs
- XmlObjectSerializerWriteContext.cs
- Brush.cs
- BinaryNode.cs
- RegexCaptureCollection.cs
- InternalsVisibleToAttribute.cs
- SqlStatistics.cs
- HtmlProps.cs
- ExpressionHelper.cs
- InvalidBodyAccessException.cs
- DesignerForm.cs
- ItemCollection.cs
- FlowDocumentPage.cs
- PolicyManager.cs
- BooleanAnimationUsingKeyFrames.cs
- ChtmlCommandAdapter.cs
- LicenseManager.cs
- ZipIOExtraFieldPaddingElement.cs
- FormatControl.cs
- ContainerParagraph.cs
- AssemblyBuilder.cs
- SystemIPInterfaceProperties.cs
- RangeExpression.cs