Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / DbXmlEnabledProviderManifest.cs / 1 / DbXmlEnabledProviderManifest.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Xml; using System.Data.EntityModel.SchemaObjectModel; using System.Data.EntityModel; using System.Data.Entity; using System.Data.Metadata.Edm; namespace System.Data.Common { ////// A specialization of the ProviderManifest that accepts an XmlReader /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbXmlEnabledProviderManifest : DbProviderManifest { private string _namespaceName; private System.Collections.ObjectModel.ReadOnlyCollection_primitiveTypes; private Dictionary > _facetDescriptions = new Dictionary >(); private System.Collections.ObjectModel.ReadOnlyCollection _functions; private Dictionary _storeTypeNameToEdmPrimitiveType = new Dictionary (); private Dictionary _storeTypeNameToStorePrimitiveType = new Dictionary (); protected DbXmlEnabledProviderManifest(XmlReader reader) { if (reader == null) { throw EntityUtil.ProviderIncompatible(Strings.IncorrectProviderManifest, new ArgumentNullException("reader")); } Load(reader); } #region Protected Properties For Fields public override string NamespaceName { get { return this._namespaceName; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] protected Dictionary StoreTypeNameToEdmPrimitiveType { get { return this._storeTypeNameToEdmPrimitiveType; } } protected Dictionary StoreTypeNameToStorePrimitiveType { get { return this._storeTypeNameToStorePrimitiveType; } } #endregion /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for. ///The FacetDescriptions for the type given. public override System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType type) { Debug.Assert(type is PrimitiveType, "DbXmlEnabledProviderManifest.GetFacetDescriptions(): Argument is not a PrimitiveType"); return GetReadOnlyCollection(type as PrimitiveType, _facetDescriptions, Helper.EmptyFacetDescriptionEnumerable); } public override System.Collections.ObjectModel.ReadOnlyCollection GetStoreTypes() { return _primitiveTypes; } /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public override System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions() { return _functions; } private void Load(XmlReader reader) { Schema schema; IList errors = SchemaManager.LoadProviderManifest(reader, reader.BaseURI.Length > 0 ? reader.BaseURI : null, true /*checkForSystemNamespace*/, out schema); if (errors.Count != 0) { throw EntityUtil.ProviderIncompatible(Strings.IncorrectProviderManifest + Helper.CombineErrorMessage(errors)); } _namespaceName = schema.Namespace; List listOfPrimitiveTypes = new List (); foreach (System.Data.EntityModel.SchemaObjectModel.SchemaType schemaType in schema.SchemaTypes) { TypeElement typeElement = schemaType as TypeElement; if (typeElement != null) { PrimitiveType type = typeElement.PrimitiveType; type.ProviderManifest = this; type.DataSpace = DataSpace.SSpace; type.SetReadOnly(); listOfPrimitiveTypes.Add(type); _storeTypeNameToStorePrimitiveType.Add(type.Name.ToLowerInvariant(), type); _storeTypeNameToEdmPrimitiveType.Add(type.Name.ToLowerInvariant(), EdmProviderManifest.Instance.GetPrimitiveType(type.PrimitiveTypeKind)); System.Collections.ObjectModel.ReadOnlyCollection descriptions; if (EnumerableToReadOnlyCollection(typeElement.FacetDescriptions, out descriptions)) { _facetDescriptions.Add(type, descriptions); } } } this._primitiveTypes = Array.AsReadOnly(listOfPrimitiveTypes.ToArray()); // load the functions ItemCollection collection = new EmptyItemCollection(); IEnumerable items = Converter.ConvertSchema(schema, this, collection); if (!EnumerableToReadOnlyCollection (items, out this._functions)) { this._functions = Helper.EmptyEdmFunctionReadOnlyCollection; } //SetReadOnly on all the Functions foreach (EdmFunction function in this._functions) { function.SetReadOnly(); } } private static System.Collections.ObjectModel.ReadOnlyCollection GetReadOnlyCollection (PrimitiveType type, Dictionary > typeDictionary, System.Collections.ObjectModel.ReadOnlyCollection useIfEmpty) { System.Collections.ObjectModel.ReadOnlyCollection collection; if (typeDictionary.TryGetValue(type, out collection)) { return collection; } else { return useIfEmpty; } } private static bool EnumerableToReadOnlyCollection (IEnumerable enumerable, out System.Collections.ObjectModel.ReadOnlyCollection collection) where Target : BaseType { List list = new List (); foreach (BaseType item in enumerable) { if (typeof(Target) == typeof(BaseType) || item is Target) { list.Add((Target)item); } } if (list.Count != 0) { collection = list.AsReadOnly(); return true; } collection = null; return false; } private class EmptyItemCollection : ItemCollection { public EmptyItemCollection() : base(DataSpace.SSpace) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Xml; using System.Data.EntityModel.SchemaObjectModel; using System.Data.EntityModel; using System.Data.Entity; using System.Data.Metadata.Edm; namespace System.Data.Common { ////// A specialization of the ProviderManifest that accepts an XmlReader /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbXmlEnabledProviderManifest : DbProviderManifest { private string _namespaceName; private System.Collections.ObjectModel.ReadOnlyCollection_primitiveTypes; private Dictionary > _facetDescriptions = new Dictionary >(); private System.Collections.ObjectModel.ReadOnlyCollection _functions; private Dictionary _storeTypeNameToEdmPrimitiveType = new Dictionary (); private Dictionary _storeTypeNameToStorePrimitiveType = new Dictionary (); protected DbXmlEnabledProviderManifest(XmlReader reader) { if (reader == null) { throw EntityUtil.ProviderIncompatible(Strings.IncorrectProviderManifest, new ArgumentNullException("reader")); } Load(reader); } #region Protected Properties For Fields public override string NamespaceName { get { return this._namespaceName; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] protected Dictionary StoreTypeNameToEdmPrimitiveType { get { return this._storeTypeNameToEdmPrimitiveType; } } protected Dictionary StoreTypeNameToStorePrimitiveType { get { return this._storeTypeNameToStorePrimitiveType; } } #endregion /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for. ///The FacetDescriptions for the type given. public override System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType type) { Debug.Assert(type is PrimitiveType, "DbXmlEnabledProviderManifest.GetFacetDescriptions(): Argument is not a PrimitiveType"); return GetReadOnlyCollection(type as PrimitiveType, _facetDescriptions, Helper.EmptyFacetDescriptionEnumerable); } public override System.Collections.ObjectModel.ReadOnlyCollection GetStoreTypes() { return _primitiveTypes; } /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public override System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions() { return _functions; } private void Load(XmlReader reader) { Schema schema; IList errors = SchemaManager.LoadProviderManifest(reader, reader.BaseURI.Length > 0 ? reader.BaseURI : null, true /*checkForSystemNamespace*/, out schema); if (errors.Count != 0) { throw EntityUtil.ProviderIncompatible(Strings.IncorrectProviderManifest + Helper.CombineErrorMessage(errors)); } _namespaceName = schema.Namespace; List listOfPrimitiveTypes = new List (); foreach (System.Data.EntityModel.SchemaObjectModel.SchemaType schemaType in schema.SchemaTypes) { TypeElement typeElement = schemaType as TypeElement; if (typeElement != null) { PrimitiveType type = typeElement.PrimitiveType; type.ProviderManifest = this; type.DataSpace = DataSpace.SSpace; type.SetReadOnly(); listOfPrimitiveTypes.Add(type); _storeTypeNameToStorePrimitiveType.Add(type.Name.ToLowerInvariant(), type); _storeTypeNameToEdmPrimitiveType.Add(type.Name.ToLowerInvariant(), EdmProviderManifest.Instance.GetPrimitiveType(type.PrimitiveTypeKind)); System.Collections.ObjectModel.ReadOnlyCollection descriptions; if (EnumerableToReadOnlyCollection(typeElement.FacetDescriptions, out descriptions)) { _facetDescriptions.Add(type, descriptions); } } } this._primitiveTypes = Array.AsReadOnly(listOfPrimitiveTypes.ToArray()); // load the functions ItemCollection collection = new EmptyItemCollection(); IEnumerable items = Converter.ConvertSchema(schema, this, collection); if (!EnumerableToReadOnlyCollection (items, out this._functions)) { this._functions = Helper.EmptyEdmFunctionReadOnlyCollection; } //SetReadOnly on all the Functions foreach (EdmFunction function in this._functions) { function.SetReadOnly(); } } private static System.Collections.ObjectModel.ReadOnlyCollection GetReadOnlyCollection (PrimitiveType type, Dictionary > typeDictionary, System.Collections.ObjectModel.ReadOnlyCollection useIfEmpty) { System.Collections.ObjectModel.ReadOnlyCollection collection; if (typeDictionary.TryGetValue(type, out collection)) { return collection; } else { return useIfEmpty; } } private static bool EnumerableToReadOnlyCollection (IEnumerable enumerable, out System.Collections.ObjectModel.ReadOnlyCollection collection) where Target : BaseType { List list = new List (); foreach (BaseType item in enumerable) { if (typeof(Target) == typeof(BaseType) || item is Target) { list.Add((Target)item); } } if (list.Count != 0) { collection = list.AsReadOnly(); return true; } collection = null; return false; } private class EmptyItemCollection : ItemCollection { public EmptyItemCollection() : base(DataSpace.SSpace) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
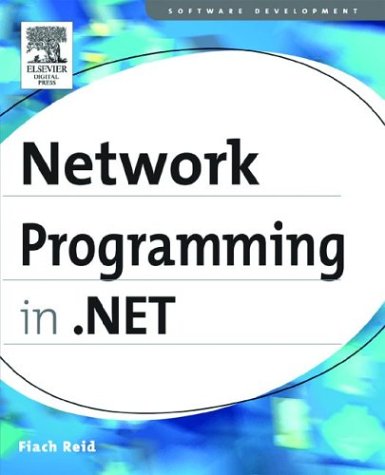
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormViewDeletedEventArgs.cs
- FixedSOMLineCollection.cs
- SimpleExpression.cs
- Queue.cs
- ResourceProviderFactory.cs
- HostVisual.cs
- SqlDependencyListener.cs
- EventLogPermissionAttribute.cs
- RequestQueryProcessor.cs
- LinearQuaternionKeyFrame.cs
- GregorianCalendarHelper.cs
- CompositeFontFamily.cs
- ProfileServiceManager.cs
- TdsEnums.cs
- Authorization.cs
- CompiledELinqQueryState.cs
- PeerToPeerException.cs
- PrintControllerWithStatusDialog.cs
- UdpDiscoveryEndpointElement.cs
- TemplateManager.cs
- ReachUIElementCollectionSerializer.cs
- HtmlContainerControl.cs
- FileNotFoundException.cs
- CompilerScopeManager.cs
- StopRoutingHandler.cs
- NetworkCredential.cs
- CapabilitiesUse.cs
- EntityClientCacheEntry.cs
- GeometryConverter.cs
- KeyGestureValueSerializer.cs
- SimpleRecyclingCache.cs
- Perspective.cs
- TreeNodeClickEventArgs.cs
- UnmanagedBitmapWrapper.cs
- ObjectContext.cs
- Canonicalizers.cs
- CharacterBuffer.cs
- RawTextInputReport.cs
- ProcessHostServerConfig.cs
- SrgsRulesCollection.cs
- SqlAliaser.cs
- LocalFileSettingsProvider.cs
- ThaiBuddhistCalendar.cs
- UshortList2.cs
- assemblycache.cs
- SQLCharsStorage.cs
- _UriSyntax.cs
- InteropExecutor.cs
- DbConnectionStringBuilder.cs
- HostingEnvironmentWrapper.cs
- BoolExpression.cs
- GeometryDrawing.cs
- CompiledRegexRunnerFactory.cs
- WebBrowserDesigner.cs
- DecoderNLS.cs
- ListComponentEditor.cs
- TextOutput.cs
- PageHandlerFactory.cs
- State.cs
- wgx_render.cs
- FixedHyperLink.cs
- DocumentScope.cs
- RtfToken.cs
- AnimationException.cs
- IntSecurity.cs
- ConcurrentDictionary.cs
- HwndMouseInputProvider.cs
- CapacityStreamGeometryContext.cs
- IxmlLineInfo.cs
- DataViewSettingCollection.cs
- MemoryMappedView.cs
- CodeCatchClauseCollection.cs
- XmlCharType.cs
- sqlinternaltransaction.cs
- State.cs
- SqlTransaction.cs
- ExpressionList.cs
- FixedSOMTable.cs
- XPathMessageContext.cs
- FontSizeConverter.cs
- ProxyWebPartManager.cs
- Queue.cs
- RemoteCryptoRsaServiceProvider.cs
- LambdaCompiler.cs
- ConfigurationSectionGroup.cs
- QilLoop.cs
- ValidatedMobileControlConverter.cs
- GCHandleCookieTable.cs
- SettingsBase.cs
- HttpWriter.cs
- ClientClassGenerator.cs
- InheritanceAttribute.cs
- ExceptionRoutedEventArgs.cs
- XmlWriterTraceListener.cs
- ConfigurationManager.cs
- SqlDataSourceConfigureFilterForm.cs
- Policy.cs
- Encoder.cs
- DataGridPageChangedEventArgs.cs
- SingleAnimationUsingKeyFrames.cs