Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DisplayInformation.cs / 1 / DisplayInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System.Security; using System.Security.Permissions; internal class DisplayInformation { private static bool highContrast; //whether we are under hight contrast mode private static bool lowRes; //whether we are under low resolution mode private static bool isTerminalServerSession; //whether this application is run on a terminal server (remote desktop) private static bool highContrastSettingValid; //indicates whether the high contrast setting is correct private static bool lowResSettingValid; //indicates whether the low resolution setting is correct private static bool terminalSettingValid; //indicates whether the terminal server setting is correct private static short bitsPerPixel = 0; private static bool dropShadowSettingValid; private static bool dropShadowEnabled; private static bool menuAccessKeysUnderlinedValid; private static bool menuAccessKeysUnderlined; static DisplayInformation() { SystemEvents.UserPreferenceChanging += new UserPreferenceChangingEventHandler(UserPreferenceChanging); SystemEvents.DisplaySettingsChanging += new EventHandler(DisplaySettingsChanging); } public static short BitsPerPixel { get { if (bitsPerPixel == 0) { // we used to iterate through all screens, but // for some reason unused screens can temparily appear // in the AllScreens collection - we would honor the display // setting of an unused screen. // According to EnumDisplayMonitors, a primary screen check should be sufficient bitsPerPixel = (short)Screen.PrimaryScreen.BitsPerPixel; } return bitsPerPixel; } } //////tests to see if the monitor is in low resolution mode (8-bit color depth or less). /// public static bool LowResolution { get { if (lowResSettingValid && !lowRes) { return lowRes; } // dont cache if we're in low resolution. lowRes = BitsPerPixel <= 8; lowResSettingValid = true; return lowRes; } } //////tests to see if we are under high contrast mode /// public static bool HighContrast { get { if (highContrastSettingValid) { return highContrast; } highContrast = SystemInformation.HighContrast; highContrastSettingValid = true; return highContrast; } } public static bool IsDropShadowEnabled { get { if (dropShadowSettingValid) { return dropShadowEnabled; } dropShadowEnabled = SystemInformation.IsDropShadowEnabled; dropShadowSettingValid = true; return dropShadowEnabled; } } //////test to see if we are under terminal server mode /// public static bool TerminalServer { get { if (terminalSettingValid) { return isTerminalServerSession; } isTerminalServerSession = SystemInformation.TerminalServerSession; terminalSettingValid = true; return isTerminalServerSession; } } // return if mnemonic underlines should always be there regardless of ALT public static bool MenuAccessKeysUnderlined { get { if (menuAccessKeysUnderlinedValid) { return menuAccessKeysUnderlined; } menuAccessKeysUnderlined = SystemInformation.MenuAccessKeysUnderlined; menuAccessKeysUnderlinedValid = true; return menuAccessKeysUnderlined; } } //////event handler for change in display setting /// private static void DisplaySettingsChanging(object obj, EventArgs ea) { highContrastSettingValid = false; lowResSettingValid = false; terminalSettingValid = false; dropShadowSettingValid = false; menuAccessKeysUnderlinedValid = false; } //////event handler for change in user preference /// private static void UserPreferenceChanging(object obj, UserPreferenceChangingEventArgs e) { highContrastSettingValid = false; lowResSettingValid = false; terminalSettingValid = false; dropShadowSettingValid = false; bitsPerPixel = 0; if (e.Category == UserPreferenceCategory.General) { menuAccessKeysUnderlinedValid =false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System.Security; using System.Security.Permissions; internal class DisplayInformation { private static bool highContrast; //whether we are under hight contrast mode private static bool lowRes; //whether we are under low resolution mode private static bool isTerminalServerSession; //whether this application is run on a terminal server (remote desktop) private static bool highContrastSettingValid; //indicates whether the high contrast setting is correct private static bool lowResSettingValid; //indicates whether the low resolution setting is correct private static bool terminalSettingValid; //indicates whether the terminal server setting is correct private static short bitsPerPixel = 0; private static bool dropShadowSettingValid; private static bool dropShadowEnabled; private static bool menuAccessKeysUnderlinedValid; private static bool menuAccessKeysUnderlined; static DisplayInformation() { SystemEvents.UserPreferenceChanging += new UserPreferenceChangingEventHandler(UserPreferenceChanging); SystemEvents.DisplaySettingsChanging += new EventHandler(DisplaySettingsChanging); } public static short BitsPerPixel { get { if (bitsPerPixel == 0) { // we used to iterate through all screens, but // for some reason unused screens can temparily appear // in the AllScreens collection - we would honor the display // setting of an unused screen. // According to EnumDisplayMonitors, a primary screen check should be sufficient bitsPerPixel = (short)Screen.PrimaryScreen.BitsPerPixel; } return bitsPerPixel; } } //////tests to see if the monitor is in low resolution mode (8-bit color depth or less). /// public static bool LowResolution { get { if (lowResSettingValid && !lowRes) { return lowRes; } // dont cache if we're in low resolution. lowRes = BitsPerPixel <= 8; lowResSettingValid = true; return lowRes; } } //////tests to see if we are under high contrast mode /// public static bool HighContrast { get { if (highContrastSettingValid) { return highContrast; } highContrast = SystemInformation.HighContrast; highContrastSettingValid = true; return highContrast; } } public static bool IsDropShadowEnabled { get { if (dropShadowSettingValid) { return dropShadowEnabled; } dropShadowEnabled = SystemInformation.IsDropShadowEnabled; dropShadowSettingValid = true; return dropShadowEnabled; } } //////test to see if we are under terminal server mode /// public static bool TerminalServer { get { if (terminalSettingValid) { return isTerminalServerSession; } isTerminalServerSession = SystemInformation.TerminalServerSession; terminalSettingValid = true; return isTerminalServerSession; } } // return if mnemonic underlines should always be there regardless of ALT public static bool MenuAccessKeysUnderlined { get { if (menuAccessKeysUnderlinedValid) { return menuAccessKeysUnderlined; } menuAccessKeysUnderlined = SystemInformation.MenuAccessKeysUnderlined; menuAccessKeysUnderlinedValid = true; return menuAccessKeysUnderlined; } } //////event handler for change in display setting /// private static void DisplaySettingsChanging(object obj, EventArgs ea) { highContrastSettingValid = false; lowResSettingValid = false; terminalSettingValid = false; dropShadowSettingValid = false; menuAccessKeysUnderlinedValid = false; } //////event handler for change in user preference /// private static void UserPreferenceChanging(object obj, UserPreferenceChangingEventArgs e) { highContrastSettingValid = false; lowResSettingValid = false; terminalSettingValid = false; dropShadowSettingValid = false; bitsPerPixel = 0; if (e.Category == UserPreferenceCategory.General) { menuAccessKeysUnderlinedValid =false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
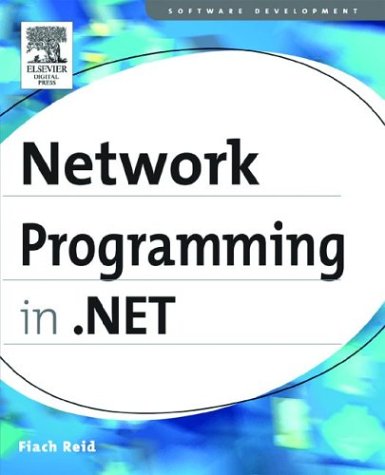
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartExportVerb.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- OracleSqlParser.cs
- WorkflowMarkupSerializationManager.cs
- TextElementCollection.cs
- SqlDataSourceSelectingEventArgs.cs
- PointLightBase.cs
- TabletCollection.cs
- BulletDecorator.cs
- PermissionAttributes.cs
- SiblingIterators.cs
- StructuralType.cs
- SqlDataSourceQueryEditorForm.cs
- ObservableDictionary.cs
- SemanticValue.cs
- WCFModelStrings.Designer.cs
- GroupBox.cs
- DataBindingsDialog.cs
- DataTemplateKey.cs
- OraclePermission.cs
- DrawingAttributes.cs
- OneOf.cs
- GridLength.cs
- DBNull.cs
- ColumnMapVisitor.cs
- TrackingDataItem.cs
- PingOptions.cs
- SqlRowUpdatedEvent.cs
- DataColumnMapping.cs
- OnOperation.cs
- ConnectionManagementSection.cs
- HttpApplicationFactory.cs
- PropertyToken.cs
- TraceContextRecord.cs
- ObjectDataSourceEventArgs.cs
- ObjectSecurity.cs
- FormsAuthenticationUser.cs
- SafeRegistryKey.cs
- WebPartConnectVerb.cs
- CodePageUtils.cs
- FlowDocumentPageViewerAutomationPeer.cs
- ItemChangedEventArgs.cs
- XmlQueryContext.cs
- DurableInstanceContextProvider.cs
- CodeVariableReferenceExpression.cs
- ZoneLinkButton.cs
- HttpRuntime.cs
- Label.cs
- X509Certificate2Collection.cs
- CodeTypeReferenceExpression.cs
- SctClaimSerializer.cs
- Brush.cs
- HtmlLink.cs
- UniformGrid.cs
- ClockController.cs
- WebPartAuthorizationEventArgs.cs
- TimeStampChecker.cs
- ServiceDescriptionSerializer.cs
- NumberFunctions.cs
- NativeBuffer.cs
- ExpressionQuoter.cs
- CommandField.cs
- ThreadAbortException.cs
- DomNameTable.cs
- XmlSerializationGeneratedCode.cs
- ZipIOModeEnforcingStream.cs
- FormView.cs
- LayoutEngine.cs
- PersianCalendar.cs
- KoreanCalendar.cs
- NGCUIElementCollectionSerializerAsync.cs
- ResourceDescriptionAttribute.cs
- Zone.cs
- UncommonField.cs
- DictionaryGlobals.cs
- HandlerBase.cs
- ListViewTableCell.cs
- DeviceSpecificDialogCachedState.cs
- DataPagerFieldCollection.cs
- SafeCryptContextHandle.cs
- XamlRtfConverter.cs
- DetailsViewPagerRow.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- PageBreakRecord.cs
- HMAC.cs
- InsufficientMemoryException.cs
- ProfileGroupSettings.cs
- OdbcFactory.cs
- OpenTypeLayout.cs
- BooleanExpr.cs
- ToolBarButton.cs
- SecurityTokenReferenceStyle.cs
- ScrollProviderWrapper.cs
- StructuralType.cs
- ResourceReferenceExpressionConverter.cs
- TransformDescriptor.cs
- SafeReversePInvokeHandle.cs
- RegexMatchCollection.cs
- DictionaryGlobals.cs
- Matrix3D.cs