Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / WindowsGrip.cs / 1 / WindowsGrip.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Button Proxy // // History: // 07/01/2003 : preid Created //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsGrip: ProxyFragment { // ----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors public WindowsGrip (IntPtr hwnd, ProxyHwnd parent, int item) : base( hwnd, parent, item) { _sType = ST.Get(STID.LocalizedControlTypeGrip); _sAutomationId = "Window.Grip"; // This string is a non-localizable string } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface ////// Gets the bounding rectangle for this element /// internal override Rect BoundingRectangle { get { if (IsGripPresent(_hwnd, false)) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (Misc.GetClientRectInScreenCoordinates(_hwnd, ref client)) { NativeMethods.SIZE sizeGrip = GetGripSize(_hwnd, false); if (Misc.IsLayoutRTL(_hwnd)) { return new Rect(client.left - sizeGrip.cx, client.bottom, sizeGrip.cx, sizeGrip.cy); } else { return new Rect(client.right, client.bottom, sizeGrip.cx, sizeGrip.cy); } } } return Rect.Empty; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods static internal bool IsGripPresent(IntPtr hwnd, bool onStatusBar) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (!Misc.GetClientRectInScreenCoordinates(hwnd, ref client)) { return false; } // According to the documentation of GetClientRect, the left and top members are zero. So if // they are negitive the control must be minimized, therefore the grip is not present. if (client.left < 0 && client.top < 0 ) { return false; } NativeMethods.SIZE sizeGrip = GetGripSize(hwnd, onStatusBar); if (!onStatusBar) { // When not on a status bar the grip should be out side of the client area. sizeGrip.cx *= -1; sizeGrip.cy *= -1; } if (Misc.IsLayoutRTL(hwnd)) { int x = client.left + (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMLEFT; } else { int x = client.right - (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMRIGHT; } } internal static NativeMethods.SIZE GetGripSize(IntPtr hwnd, bool onStatusBar) { using (ThemePart themePart = new ThemePart(hwnd, onStatusBar ? "STATUS" : "SCROLLBAR")) { return themePart.Size(onStatusBar ? (int)ThemePart.STATUSPARTS.SP_GRIPPER : (int)ThemePart.SCROLLBARPARTS.SBP_SIZEBOX, 0); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Button Proxy // // History: // 07/01/2003 : preid Created //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsGrip: ProxyFragment { // ----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors public WindowsGrip (IntPtr hwnd, ProxyHwnd parent, int item) : base( hwnd, parent, item) { _sType = ST.Get(STID.LocalizedControlTypeGrip); _sAutomationId = "Window.Grip"; // This string is a non-localizable string } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface ////// Gets the bounding rectangle for this element /// internal override Rect BoundingRectangle { get { if (IsGripPresent(_hwnd, false)) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (Misc.GetClientRectInScreenCoordinates(_hwnd, ref client)) { NativeMethods.SIZE sizeGrip = GetGripSize(_hwnd, false); if (Misc.IsLayoutRTL(_hwnd)) { return new Rect(client.left - sizeGrip.cx, client.bottom, sizeGrip.cx, sizeGrip.cy); } else { return new Rect(client.right, client.bottom, sizeGrip.cx, sizeGrip.cy); } } } return Rect.Empty; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods static internal bool IsGripPresent(IntPtr hwnd, bool onStatusBar) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (!Misc.GetClientRectInScreenCoordinates(hwnd, ref client)) { return false; } // According to the documentation of GetClientRect, the left and top members are zero. So if // they are negitive the control must be minimized, therefore the grip is not present. if (client.left < 0 && client.top < 0 ) { return false; } NativeMethods.SIZE sizeGrip = GetGripSize(hwnd, onStatusBar); if (!onStatusBar) { // When not on a status bar the grip should be out side of the client area. sizeGrip.cx *= -1; sizeGrip.cy *= -1; } if (Misc.IsLayoutRTL(hwnd)) { int x = client.left + (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMLEFT; } else { int x = client.right - (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMRIGHT; } } internal static NativeMethods.SIZE GetGripSize(IntPtr hwnd, bool onStatusBar) { using (ThemePart themePart = new ThemePart(hwnd, onStatusBar ? "STATUS" : "SCROLLBAR")) { return themePart.Size(onStatusBar ? (int)ThemePart.STATUSPARTS.SP_GRIPPER : (int)ThemePart.SCROLLBARPARTS.SBP_SIZEBOX, 0); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
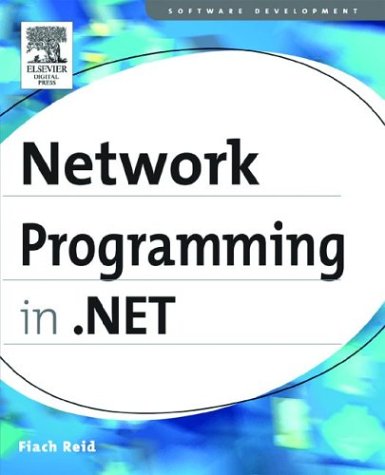
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StickyNote.cs
- FieldBuilder.cs
- BrushMappingModeValidation.cs
- XamlTreeBuilder.cs
- SecurityContext.cs
- XmlDownloadManager.cs
- ConfigurationStrings.cs
- DBConcurrencyException.cs
- XsdDataContractExporter.cs
- RIPEMD160Managed.cs
- SchemaElementLookUpTableEnumerator.cs
- SelectionEditingBehavior.cs
- SignatureToken.cs
- TimelineClockCollection.cs
- WebPartConnectionsDisconnectVerb.cs
- ImageField.cs
- JsonReaderDelegator.cs
- SweepDirectionValidation.cs
- CqlIdentifiers.cs
- CodeObject.cs
- ApplicationDirectory.cs
- StylusCaptureWithinProperty.cs
- CustomAttribute.cs
- Brush.cs
- AxisAngleRotation3D.cs
- ContentPresenter.cs
- ToolStripPanelCell.cs
- DataGridViewLinkCell.cs
- TransactedBatchingElement.cs
- HttpCachePolicy.cs
- DescendantBaseQuery.cs
- OptimalTextSource.cs
- Codec.cs
- DecimalKeyFrameCollection.cs
- SelectionRange.cs
- SpeakCompletedEventArgs.cs
- LayoutEvent.cs
- SHA384Managed.cs
- NodeFunctions.cs
- WriteFileContext.cs
- SqlDataReaderSmi.cs
- ContainerVisual.cs
- webeventbuffer.cs
- AlignmentXValidation.cs
- SplitterCancelEvent.cs
- InitializerFacet.cs
- QueryCacheEntry.cs
- TimeoutException.cs
- HtmlInputRadioButton.cs
- EDesignUtil.cs
- ContextDataSourceView.cs
- DoWhileDesigner.xaml.cs
- ToolBarButton.cs
- SHA512CryptoServiceProvider.cs
- Material.cs
- VerifyHashRequest.cs
- TranslateTransform3D.cs
- ImageFormatConverter.cs
- FormViewDeletedEventArgs.cs
- DataRecordInfo.cs
- XmlWriterTraceListener.cs
- CodeGenHelper.cs
- TaskExceptionHolder.cs
- AxisAngleRotation3D.cs
- MimeTextImporter.cs
- Header.cs
- TextShapeableCharacters.cs
- GeometryHitTestParameters.cs
- LogExtent.cs
- HandlerFactoryCache.cs
- FormsAuthenticationUser.cs
- XmlIgnoreAttribute.cs
- DispatchChannelSink.cs
- UtilityExtension.cs
- RegularExpressionValidator.cs
- SQLBinaryStorage.cs
- TrackingProvider.cs
- JumpTask.cs
- KnowledgeBase.cs
- DynamicRendererThreadManager.cs
- MorphHelpers.cs
- SR.cs
- XmlSchemaAnnotation.cs
- BasicKeyConstraint.cs
- WithStatement.cs
- IHttpResponseInternal.cs
- XomlCompilerHelpers.cs
- WindowsStartMenu.cs
- ViewManager.cs
- GZipUtils.cs
- WebConfigurationHostFileChange.cs
- QuotaExceededException.cs
- CryptoProvider.cs
- HttpConfigurationContext.cs
- ResourceCollectionInfo.cs
- XmlDataSourceView.cs
- EditableTreeList.cs
- DataSourceCache.cs
- QueryOperatorEnumerator.cs
- PageParser.cs