Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Data / XmlNamespaceMapping.cs / 1 / XmlNamespaceMapping.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMapping object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // ISupportInitialize namespace System.Windows.Data { ////// XmlNamespaceMapping Class /// used for declaring Xml Namespace Mappings /// public class XmlNamespaceMapping : ISupportInitialize { ////// Constructor for XmlNamespaceMapping /// public XmlNamespaceMapping() { } ////// Constructor for XmlNamespaceMapping /// public XmlNamespaceMapping(string prefix, Uri uri) { _prefix = prefix; _uri = uri; } ////// The prefix to be used for this Namespace /// public string Prefix { get { return _prefix; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "Prefix", this.GetType().Name)); if (_prefix != null && _prefix != value) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "Prefix", this.GetType().Name)); _prefix = value; } } ////// The Uri to be used for this Namespace, /// can be declared as an attribute or as the /// TextContent of the XmlNamespaceMapping markup tag /// public Uri Uri { get { return _uri; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "Uri", this.GetType().Name)); if (_uri != null && _uri != value) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "Uri", this.GetType().Name)); _uri = value; } } ////// Equality comparison by value /// public override bool Equals(object obj) { return (this == (obj as XmlNamespaceMapping)); // call the == operator override } ////// Equality comparison by value /// public static bool operator == (XmlNamespaceMapping mappingA, XmlNamespaceMapping mappingB) { // cannot just compare with (mappingX == null), it'll cause recursion and stack overflow! if (object.ReferenceEquals(mappingA, null)) return object.ReferenceEquals(mappingB, null); if (object.ReferenceEquals(mappingB, null)) return false; #pragma warning disable 1634, 1691 // presharp false positive for null-checking on mappings #pragma warning suppress 56506 return ((mappingA.Prefix == mappingB.Prefix) && (mappingA.Uri == mappingB.Uri)) ; #pragma warning restore 1634, 1691 } ////// Inequality comparison by value /// public static bool operator != (XmlNamespaceMapping mappingA, XmlNamespaceMapping mappingB) { return !(mappingA == mappingB); } ////// Hash function for this type /// public override int GetHashCode() { // note that the hash code can change, but only during intialization // (_prefix and _uri can only be changed once, from null to // non-null, and only during [Begin/End]Init). Technically this is // still a violation of the "constant during lifetime" rule, however // in practice this is acceptable. It is very unlikely that someone // will put an XmlNamespaceMapping into a hashtable before it is initialized. int hash = 0; if (_prefix != null) hash = _prefix.GetHashCode(); if (_uri != null) return unchecked(hash + _uri.GetHashCode()); else return hash; } #region ISupportInitialize ///Begin Initialization void ISupportInitialize.BeginInit() { _initializing = true; } ///End Initialization, verify that internal state is consistent void ISupportInitialize.EndInit() { if (_prefix == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "Prefix", this.GetType().Name)); } if (_uri == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "Uri", this.GetType().Name)); } _initializing = false; } #endregion ISupportInitialize private string _prefix; private Uri _uri; private bool _initializing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMapping object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // ISupportInitialize namespace System.Windows.Data { ////// XmlNamespaceMapping Class /// used for declaring Xml Namespace Mappings /// public class XmlNamespaceMapping : ISupportInitialize { ////// Constructor for XmlNamespaceMapping /// public XmlNamespaceMapping() { } ////// Constructor for XmlNamespaceMapping /// public XmlNamespaceMapping(string prefix, Uri uri) { _prefix = prefix; _uri = uri; } ////// The prefix to be used for this Namespace /// public string Prefix { get { return _prefix; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "Prefix", this.GetType().Name)); if (_prefix != null && _prefix != value) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "Prefix", this.GetType().Name)); _prefix = value; } } ////// The Uri to be used for this Namespace, /// can be declared as an attribute or as the /// TextContent of the XmlNamespaceMapping markup tag /// public Uri Uri { get { return _uri; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "Uri", this.GetType().Name)); if (_uri != null && _uri != value) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "Uri", this.GetType().Name)); _uri = value; } } ////// Equality comparison by value /// public override bool Equals(object obj) { return (this == (obj as XmlNamespaceMapping)); // call the == operator override } ////// Equality comparison by value /// public static bool operator == (XmlNamespaceMapping mappingA, XmlNamespaceMapping mappingB) { // cannot just compare with (mappingX == null), it'll cause recursion and stack overflow! if (object.ReferenceEquals(mappingA, null)) return object.ReferenceEquals(mappingB, null); if (object.ReferenceEquals(mappingB, null)) return false; #pragma warning disable 1634, 1691 // presharp false positive for null-checking on mappings #pragma warning suppress 56506 return ((mappingA.Prefix == mappingB.Prefix) && (mappingA.Uri == mappingB.Uri)) ; #pragma warning restore 1634, 1691 } ////// Inequality comparison by value /// public static bool operator != (XmlNamespaceMapping mappingA, XmlNamespaceMapping mappingB) { return !(mappingA == mappingB); } ////// Hash function for this type /// public override int GetHashCode() { // note that the hash code can change, but only during intialization // (_prefix and _uri can only be changed once, from null to // non-null, and only during [Begin/End]Init). Technically this is // still a violation of the "constant during lifetime" rule, however // in practice this is acceptable. It is very unlikely that someone // will put an XmlNamespaceMapping into a hashtable before it is initialized. int hash = 0; if (_prefix != null) hash = _prefix.GetHashCode(); if (_uri != null) return unchecked(hash + _uri.GetHashCode()); else return hash; } #region ISupportInitialize ///Begin Initialization void ISupportInitialize.BeginInit() { _initializing = true; } ///End Initialization, verify that internal state is consistent void ISupportInitialize.EndInit() { if (_prefix == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "Prefix", this.GetType().Name)); } if (_uri == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "Uri", this.GetType().Name)); } _initializing = false; } #endregion ISupportInitialize private string _prefix; private Uri _uri; private bool _initializing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
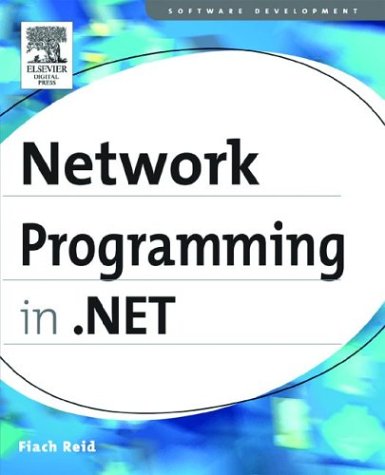
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Literal.cs
- ToolStripScrollButton.cs
- SqlAliaser.cs
- HashStream.cs
- ProfileSettings.cs
- StringResourceManager.cs
- HttpClientCertificate.cs
- Int64AnimationBase.cs
- PrintDialogException.cs
- LinqDataSourceDeleteEventArgs.cs
- TypeConverterValueSerializer.cs
- Span.cs
- DataListItem.cs
- FrugalList.cs
- SettingsPropertyNotFoundException.cs
- CodeTypeParameterCollection.cs
- Site.cs
- XmlCDATASection.cs
- DictionaryContent.cs
- CFStream.cs
- RefreshPropertiesAttribute.cs
- TimeoutException.cs
- CodeExporter.cs
- IndexOutOfRangeException.cs
- InstanceStore.cs
- LogicalExpr.cs
- HtmlEmptyTagControlBuilder.cs
- MailDefinition.cs
- EntityDataSourceColumn.cs
- CollectionViewSource.cs
- Condition.cs
- BezierSegment.cs
- TraceHandler.cs
- safelinkcollection.cs
- RouteParser.cs
- ToolStripLocationCancelEventArgs.cs
- ConfigXmlSignificantWhitespace.cs
- ServicesUtilities.cs
- TableChangeProcessor.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- PagesSection.cs
- XhtmlBasicTextBoxAdapter.cs
- DbDeleteCommandTree.cs
- EntityDataSourceViewSchema.cs
- IisTraceWebEventProvider.cs
- _RequestCacheProtocol.cs
- LogExtentCollection.cs
- WindowsClaimSet.cs
- TextLineResult.cs
- ClientUtils.cs
- TraceInternal.cs
- HttpDebugHandler.cs
- CriticalExceptions.cs
- DataGridTextBox.cs
- ISO2022Encoding.cs
- DbParameterHelper.cs
- SelectedDatesCollection.cs
- ObjectParameter.cs
- webproxy.cs
- TreeNodeMouseHoverEvent.cs
- RelationshipEndCollection.cs
- BitmapEffectInputData.cs
- DataPagerCommandEventArgs.cs
- XhtmlTextWriter.cs
- WasHttpHandlersInstallComponent.cs
- HttpInputStream.cs
- ProxyWebPartManager.cs
- DeobfuscatingStream.cs
- ComponentSerializationService.cs
- ListParaClient.cs
- MailDefinition.cs
- FixedFlowMap.cs
- SweepDirectionValidation.cs
- TraceSwitch.cs
- UnsafeNativeMethods.cs
- Command.cs
- AttachedPropertyBrowsableAttribute.cs
- IPAddressCollection.cs
- RequestCachePolicy.cs
- TagNameToTypeMapper.cs
- Membership.cs
- TdsRecordBufferSetter.cs
- DataGridViewRowPrePaintEventArgs.cs
- SiteOfOriginContainer.cs
- AutoSizeToolBoxItem.cs
- CharKeyFrameCollection.cs
- BrowserCapabilitiesFactory.cs
- MsmqIntegrationElement.cs
- PrintingPermissionAttribute.cs
- StorageFunctionMapping.cs
- XmlSchemaSimpleTypeUnion.cs
- SchemaImporterExtensionElementCollection.cs
- WmpBitmapEncoder.cs
- ReadOnlyHierarchicalDataSourceView.cs
- SBCSCodePageEncoding.cs
- SafePointer.cs
- RestHandlerFactory.cs
- UntypedNullExpression.cs
- DataBindEngine.cs
- QueueProcessor.cs