Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / documents / ContentHostHelper.cs / 1 / ContentHostHelper.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentHostHelper.cs // // Description: The ContentHostHelper class contains static methods that are // useful for performing common tasks with IContentHost. // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // Listusing System.Windows; // IContentHost using System.Windows.Controls; // TextBlock using System.Windows.Controls.Primitives; // DocumentPageView using System.Windows.Documents; // FlowDocument using System.Windows.Media; // Visual using MS.Internal.PtsHost; // FlowDocumentPage namespace MS.Internal.Documents { /// /// Static helper functions for dealing with IContentHost. /// internal static class ContentHostHelper { //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Given a ContentElement searches for associated IContentHost, if one exists. /// /// Content element ///Associated IContentHost with ContentElement. internal static IContentHost FindContentHost(ContentElement contentElement) { IContentHost ich = null; DependencyObject parent; TextContainer textContainer; if (contentElement == null) { return null; } // If the ContentElement is a TextElement, retrieve IContentHost form the owner // of TextContainer. if (contentElement is TextElement) { textContainer = ((TextElement)contentElement).TextContainer; parent = textContainer.Parent; if (parent is IContentHost) // TextBlock { ich = (IContentHost)parent; } else if (parent is FlowDocument) // Viewers { ich = GetICHFromFlowDocument((TextElement)contentElement, (FlowDocument)parent); } else if (textContainer.TextView != null && textContainer.TextView.RenderScope is IContentHost) { // TextBlock hosted in ControlTemplate ich = (IContentHost)textContainer.TextView.RenderScope; } } // else; cannot retrive IContentHost return ich; } #endregion Internal Methods //-------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Given a ContentElement within FlowDocument searches for associated IContentHost. /// /// Content element /// FlowDocument hosting ContentElement. ///Associated IContentHost with ContentElement. private static IContentHost GetICHFromFlowDocument(TextElement contentElement, FlowDocument flowDocument) { IContentHost ich = null; ListpageViews; ITextView textView = flowDocument.StructuralCache.TextContainer.TextView; if (textView != null) { // If FlowDocument is hosted by FlowDocumentScrollViewer, the RenderScope // is FlowDocumentView object which hosts PageVisual representing the content. // This PageVisual is also IContentHost for the entire content of DocumentPage. if (textView.RenderScope is FlowDocumentView) // FlowDocumentScrollViewer { if (VisualTreeHelper.GetChildrenCount(textView.RenderScope) > 0) { ich = VisualTreeHelper.GetChild(textView.RenderScope, 0) as IContentHost; } } // Our best guess is that FlowDocument is hosted by DocumentViewerBase. // In this case search the style for all DocumentPageViews. // Having collection of DocumentPageViews, find for the one which hosts TextElement. else if (textView.RenderScope is FrameworkElement) { pageViews = new List (); FindDocumentPageViews(textView.RenderScope, pageViews); for (int i = 0; i < pageViews.Count; i++) { if (pageViews[i].DocumentPage is FlowDocumentPage) { textView = (ITextView)((IServiceProvider)pageViews[i].DocumentPage).GetService(typeof(ITextView)); if (textView != null && textView.IsValid) { // Check if the page contains ContentElement. Check Start and End // position, which will give desired results in most of the cases. // Having hyperlink spanning more than 2 pages is not very common, // and this code will not work with it correctly. if (textView.Contains(contentElement.ContentStart) || textView.Contains(contentElement.ContentEnd)) { ich = pageViews[i].DocumentPage.Visual as IContentHost; } } } } } } return ich; } /// /// Does deep Visual tree walk to retrieve all DocumentPageViews. /// It stops recursing down into visual tree in following situations: /// a) Visual is UIElement and it is not part of Contol Template, /// b) Visual is DocumentPageView. /// /// FrameworkElement that is part of Control Template. /// Collection of DocumentPageViews; found elements are appended here. ///Whether collection of DocumentPageViews has been updated. private static void FindDocumentPageViews(Visual root, ListpageViews) { Invariant.Assert(root != null); Invariant.Assert(pageViews != null); if (root is DocumentPageView) { pageViews.Add((DocumentPageView)root); } else { FrameworkElement fe; // Do deep tree walk to retrieve all DocumentPageViews. // It stops recursing down into visual tree in following situations: // a) Visual is UIElement and it is not part of Contol Template, // b) Visual is DocumentPageView. // Add to collection any DocumentPageViews found in the Control Template. int count = root.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual child = root.InternalGetVisualChild(i); fe = child as FrameworkElement; if (fe != null) { if (fe.TemplatedParent != null) { if (fe is DocumentPageView) { pageViews.Add(fe as DocumentPageView); } else { FindDocumentPageViews(fe, pageViews); } } } else { FindDocumentPageViews(child, pageViews); } } } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentHostHelper.cs // // Description: The ContentHostHelper class contains static methods that are // useful for performing common tasks with IContentHost. // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // List using System.Windows; // IContentHost using System.Windows.Controls; // TextBlock using System.Windows.Controls.Primitives; // DocumentPageView using System.Windows.Documents; // FlowDocument using System.Windows.Media; // Visual using MS.Internal.PtsHost; // FlowDocumentPage namespace MS.Internal.Documents { /// /// Static helper functions for dealing with IContentHost. /// internal static class ContentHostHelper { //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Given a ContentElement searches for associated IContentHost, if one exists. /// /// Content element ///Associated IContentHost with ContentElement. internal static IContentHost FindContentHost(ContentElement contentElement) { IContentHost ich = null; DependencyObject parent; TextContainer textContainer; if (contentElement == null) { return null; } // If the ContentElement is a TextElement, retrieve IContentHost form the owner // of TextContainer. if (contentElement is TextElement) { textContainer = ((TextElement)contentElement).TextContainer; parent = textContainer.Parent; if (parent is IContentHost) // TextBlock { ich = (IContentHost)parent; } else if (parent is FlowDocument) // Viewers { ich = GetICHFromFlowDocument((TextElement)contentElement, (FlowDocument)parent); } else if (textContainer.TextView != null && textContainer.TextView.RenderScope is IContentHost) { // TextBlock hosted in ControlTemplate ich = (IContentHost)textContainer.TextView.RenderScope; } } // else; cannot retrive IContentHost return ich; } #endregion Internal Methods //-------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Given a ContentElement within FlowDocument searches for associated IContentHost. /// /// Content element /// FlowDocument hosting ContentElement. ///Associated IContentHost with ContentElement. private static IContentHost GetICHFromFlowDocument(TextElement contentElement, FlowDocument flowDocument) { IContentHost ich = null; ListpageViews; ITextView textView = flowDocument.StructuralCache.TextContainer.TextView; if (textView != null) { // If FlowDocument is hosted by FlowDocumentScrollViewer, the RenderScope // is FlowDocumentView object which hosts PageVisual representing the content. // This PageVisual is also IContentHost for the entire content of DocumentPage. if (textView.RenderScope is FlowDocumentView) // FlowDocumentScrollViewer { if (VisualTreeHelper.GetChildrenCount(textView.RenderScope) > 0) { ich = VisualTreeHelper.GetChild(textView.RenderScope, 0) as IContentHost; } } // Our best guess is that FlowDocument is hosted by DocumentViewerBase. // In this case search the style for all DocumentPageViews. // Having collection of DocumentPageViews, find for the one which hosts TextElement. else if (textView.RenderScope is FrameworkElement) { pageViews = new List (); FindDocumentPageViews(textView.RenderScope, pageViews); for (int i = 0; i < pageViews.Count; i++) { if (pageViews[i].DocumentPage is FlowDocumentPage) { textView = (ITextView)((IServiceProvider)pageViews[i].DocumentPage).GetService(typeof(ITextView)); if (textView != null && textView.IsValid) { // Check if the page contains ContentElement. Check Start and End // position, which will give desired results in most of the cases. // Having hyperlink spanning more than 2 pages is not very common, // and this code will not work with it correctly. if (textView.Contains(contentElement.ContentStart) || textView.Contains(contentElement.ContentEnd)) { ich = pageViews[i].DocumentPage.Visual as IContentHost; } } } } } } return ich; } /// /// Does deep Visual tree walk to retrieve all DocumentPageViews. /// It stops recursing down into visual tree in following situations: /// a) Visual is UIElement and it is not part of Contol Template, /// b) Visual is DocumentPageView. /// /// FrameworkElement that is part of Control Template. /// Collection of DocumentPageViews; found elements are appended here. ///Whether collection of DocumentPageViews has been updated. private static void FindDocumentPageViews(Visual root, ListpageViews) { Invariant.Assert(root != null); Invariant.Assert(pageViews != null); if (root is DocumentPageView) { pageViews.Add((DocumentPageView)root); } else { FrameworkElement fe; // Do deep tree walk to retrieve all DocumentPageViews. // It stops recursing down into visual tree in following situations: // a) Visual is UIElement and it is not part of Contol Template, // b) Visual is DocumentPageView. // Add to collection any DocumentPageViews found in the Control Template. int count = root.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual child = root.InternalGetVisualChild(i); fe = child as FrameworkElement; if (fe != null) { if (fe.TemplatedParent != null) { if (fe is DocumentPageView) { pageViews.Add(fe as DocumentPageView); } else { FindDocumentPageViews(fe, pageViews); } } } else { FindDocumentPageViews(child, pageViews); } } } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
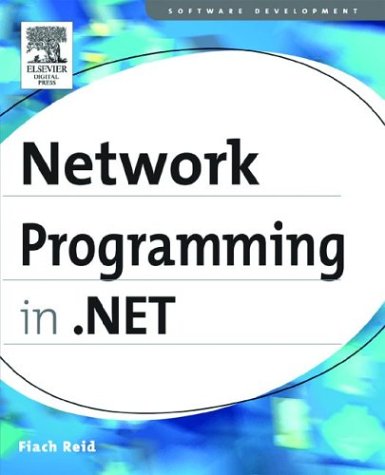
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripCustomTypeDescriptor.cs
- ToolStripHighContrastRenderer.cs
- XmlWrappingWriter.cs
- NonParentingControl.cs
- ScrollableControl.cs
- HostingEnvironment.cs
- Int32Animation.cs
- BuildProviderCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- Attachment.cs
- safemediahandle.cs
- Latin1Encoding.cs
- LZCodec.cs
- LiteralTextParser.cs
- StrokeCollection2.cs
- TypeDelegator.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- RotateTransform3D.cs
- AtomMaterializerLog.cs
- MessageAction.cs
- Point4D.cs
- CqlErrorHelper.cs
- Stack.cs
- ReferenceService.cs
- ParameterEditorUserControl.cs
- QueryContinueDragEventArgs.cs
- ScriptManager.cs
- DecimalAnimation.cs
- Types.cs
- NetStream.cs
- SqlNode.cs
- Quad.cs
- RedistVersionInfo.cs
- Rect3DValueSerializer.cs
- NonParentingControl.cs
- CodeArrayCreateExpression.cs
- XhtmlBasicCalendarAdapter.cs
- Regex.cs
- MatrixAnimationUsingPath.cs
- DataListItemEventArgs.cs
- AuthenticationModulesSection.cs
- EDesignUtil.cs
- TcpConnectionPoolSettings.cs
- RunWorkerCompletedEventArgs.cs
- XmlSchemaSimpleContent.cs
- ResizeGrip.cs
- Propagator.Evaluator.cs
- MultipartContentParser.cs
- TimeoutException.cs
- XmlSyndicationContent.cs
- LockCookie.cs
- ProtocolReflector.cs
- DesignerProperties.cs
- ChangeBlockUndoRecord.cs
- SynchronizedChannelCollection.cs
- BorderGapMaskConverter.cs
- EventItfInfo.cs
- CodeIterationStatement.cs
- EditorZone.cs
- SystemWebCachingSectionGroup.cs
- DataGridTable.cs
- FlowLayoutSettings.cs
- TextChange.cs
- BitmapEffectGeneralTransform.cs
- CellNormalizer.cs
- BufferedConnection.cs
- AsyncDataRequest.cs
- CacheAxisQuery.cs
- SQLDateTime.cs
- RectangleF.cs
- UIElement3DAutomationPeer.cs
- StructuralObject.cs
- WeakReference.cs
- ReadonlyMessageFilter.cs
- XmlSchemaComplexType.cs
- JavaScriptString.cs
- PageCodeDomTreeGenerator.cs
- EventLogPermissionAttribute.cs
- AudioException.cs
- QfeChecker.cs
- ViewService.cs
- DBParameter.cs
- XmlJsonWriter.cs
- XmlSchemaInferenceException.cs
- ResourcesGenerator.cs
- ProfileGroupSettings.cs
- Int32AnimationBase.cs
- Visual3D.cs
- ResourceContainer.cs
- pingexception.cs
- TypeConverterValueSerializer.cs
- CombinedGeometry.cs
- XmlHierarchyData.cs
- EmbossBitmapEffect.cs
- BoundField.cs
- ISessionStateStore.cs
- BitmapPalette.cs
- DefaultValueConverter.cs
- UIPermission.cs
- InstanceData.cs