Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPointPropertyInfoDefaults.cs / 1 / StylusPointPropertyInfoDefaults.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; namespace System.Windows.Input { internal static class StylusPointPropertyInfoDefaults { ////// X /// internal static readonly StylusPointPropertyInfo X = new StylusPointPropertyInfo(StylusPointProperties.X, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Y /// internal static readonly StylusPointPropertyInfo Y = new StylusPointPropertyInfo(StylusPointProperties.Y, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Z /// internal static readonly StylusPointPropertyInfo Z = new StylusPointPropertyInfo(StylusPointProperties.Z, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Width /// internal static readonly StylusPointPropertyInfo Width = new StylusPointPropertyInfo(StylusPointProperties.Width, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Height /// internal static readonly StylusPointPropertyInfo Height = new StylusPointPropertyInfo(StylusPointProperties.Height, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// SystemTouch /// internal static readonly StylusPointPropertyInfo SystemTouch = new StylusPointPropertyInfo(StylusPointProperties.SystemTouch, 0, 1, StylusPointPropertyUnit.None, 1.0f); ////// PacketStatus internal static readonly StylusPointPropertyInfo PacketStatus = new StylusPointPropertyInfo(StylusPointProperties.PacketStatus, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// SerialNumber internal static readonly StylusPointPropertyInfo SerialNumber = new StylusPointPropertyInfo(StylusPointProperties.SerialNumber, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// NormalPressure /// internal static readonly StylusPointPropertyInfo NormalPressure = new StylusPointPropertyInfo(StylusPointProperties.NormalPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// TangentPressure /// internal static readonly StylusPointPropertyInfo TangentPressure = new StylusPointPropertyInfo(StylusPointProperties.TangentPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// ButtonPressure /// internal static readonly StylusPointPropertyInfo ButtonPressure = new StylusPointPropertyInfo(StylusPointProperties.ButtonPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// XTiltOrientation /// internal static readonly StylusPointPropertyInfo XTiltOrientation = new StylusPointPropertyInfo(StylusPointProperties.XTiltOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// YTiltOrientation /// internal static readonly StylusPointPropertyInfo YTiltOrientation = new StylusPointPropertyInfo(StylusPointProperties.YTiltOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// AzimuthOrientation /// internal static readonly StylusPointPropertyInfo AzimuthOrientation = new StylusPointPropertyInfo(StylusPointProperties.AzimuthOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// AltitudeOrientation /// internal static readonly StylusPointPropertyInfo AltitudeOrientation = new StylusPointPropertyInfo(StylusPointProperties.AltitudeOrientation, -900, 900, StylusPointPropertyUnit.Degrees, 10.0f); ////// TwistOrientation /// internal static readonly StylusPointPropertyInfo TwistOrientation = new StylusPointPropertyInfo(StylusPointProperties.TwistOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// PitchRotation internal static readonly StylusPointPropertyInfo PitchRotation = new StylusPointPropertyInfo(StylusPointProperties.PitchRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// RollRotation internal static readonly StylusPointPropertyInfo RollRotation = new StylusPointPropertyInfo(StylusPointProperties.RollRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// YawRotation internal static readonly StylusPointPropertyInfo YawRotation = new StylusPointPropertyInfo(StylusPointProperties.YawRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// TipButton internal static readonly StylusPointPropertyInfo TipButton = new StylusPointPropertyInfo(StylusPointProperties.TipButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// BarrelButton internal static readonly StylusPointPropertyInfo BarrelButton = new StylusPointPropertyInfo(StylusPointProperties.BarrelButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// SecondaryTipButton internal static readonly StylusPointPropertyInfo SecondaryTipButton = new StylusPointPropertyInfo(StylusPointProperties.SecondaryTipButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// Default Value /// internal static readonly StylusPointPropertyInfo DefaultValue = new StylusPointPropertyInfo(new StylusPointProperty(Guid.NewGuid(), false), Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0F); ////// DefaultButton internal static readonly StylusPointPropertyInfo DefaultButton = new StylusPointPropertyInfo(new StylusPointProperty(Guid.NewGuid(), true), 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// For a given StylusPointProperty, return the default property info /// /// stylusPointProperty ///internal static StylusPointPropertyInfo GetStylusPointPropertyInfoDefault(StylusPointProperty stylusPointProperty) { if (stylusPointProperty.Id == StylusPointPropertyIds.X) { return StylusPointPropertyInfoDefaults.X; } if (stylusPointProperty.Id == StylusPointPropertyIds.Y) { return StylusPointPropertyInfoDefaults.Y; } if (stylusPointProperty.Id == StylusPointPropertyIds.Z) { return StylusPointPropertyInfoDefaults.Z; } if (stylusPointProperty.Id == StylusPointPropertyIds.Width) { return StylusPointPropertyInfoDefaults.Width; } if (stylusPointProperty.Id == StylusPointPropertyIds.Height) { return StylusPointPropertyInfoDefaults.Height; } if (stylusPointProperty.Id == StylusPointPropertyIds.SystemTouch) { return StylusPointPropertyInfoDefaults.SystemTouch; } if (stylusPointProperty.Id == StylusPointPropertyIds.PacketStatus) { return StylusPointPropertyInfoDefaults.PacketStatus; } if (stylusPointProperty.Id == StylusPointPropertyIds.SerialNumber) { return StylusPointPropertyInfoDefaults.SerialNumber; } if (stylusPointProperty.Id == StylusPointPropertyIds.NormalPressure) { return StylusPointPropertyInfoDefaults.NormalPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.TangentPressure) { return StylusPointPropertyInfoDefaults.TangentPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.ButtonPressure) { return StylusPointPropertyInfoDefaults.ButtonPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.XTiltOrientation) { return StylusPointPropertyInfoDefaults.XTiltOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.YTiltOrientation) { return StylusPointPropertyInfoDefaults.YTiltOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.AzimuthOrientation) { return StylusPointPropertyInfoDefaults.AzimuthOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.AltitudeOrientation) { return StylusPointPropertyInfoDefaults.AltitudeOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.TwistOrientation) { return StylusPointPropertyInfoDefaults.TwistOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.PitchRotation) { return StylusPointPropertyInfoDefaults.PitchRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.RollRotation) { return StylusPointPropertyInfoDefaults.RollRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.YawRotation) { return StylusPointPropertyInfoDefaults.YawRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.TipButton) { return StylusPointPropertyInfoDefaults.TipButton; } if (stylusPointProperty.Id == StylusPointPropertyIds.BarrelButton) { return StylusPointPropertyInfoDefaults.BarrelButton; } if (stylusPointProperty.Id == StylusPointPropertyIds.SecondaryTipButton) { return StylusPointPropertyInfoDefaults.SecondaryTipButton; } // // return a default // if (stylusPointProperty.IsButton) { return StylusPointPropertyInfoDefaults.DefaultButton; } return StylusPointPropertyInfoDefaults.DefaultValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; namespace System.Windows.Input { internal static class StylusPointPropertyInfoDefaults { ////// X /// internal static readonly StylusPointPropertyInfo X = new StylusPointPropertyInfo(StylusPointProperties.X, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Y /// internal static readonly StylusPointPropertyInfo Y = new StylusPointPropertyInfo(StylusPointProperties.Y, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Z /// internal static readonly StylusPointPropertyInfo Z = new StylusPointPropertyInfo(StylusPointProperties.Z, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Width /// internal static readonly StylusPointPropertyInfo Width = new StylusPointPropertyInfo(StylusPointProperties.Width, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// Height /// internal static readonly StylusPointPropertyInfo Height = new StylusPointPropertyInfo(StylusPointProperties.Height, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.Centimeters, 1000.0f); ////// SystemTouch /// internal static readonly StylusPointPropertyInfo SystemTouch = new StylusPointPropertyInfo(StylusPointProperties.SystemTouch, 0, 1, StylusPointPropertyUnit.None, 1.0f); ////// PacketStatus internal static readonly StylusPointPropertyInfo PacketStatus = new StylusPointPropertyInfo(StylusPointProperties.PacketStatus, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// SerialNumber internal static readonly StylusPointPropertyInfo SerialNumber = new StylusPointPropertyInfo(StylusPointProperties.SerialNumber, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// NormalPressure /// internal static readonly StylusPointPropertyInfo NormalPressure = new StylusPointPropertyInfo(StylusPointProperties.NormalPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// TangentPressure /// internal static readonly StylusPointPropertyInfo TangentPressure = new StylusPointPropertyInfo(StylusPointProperties.TangentPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// ButtonPressure /// internal static readonly StylusPointPropertyInfo ButtonPressure = new StylusPointPropertyInfo(StylusPointProperties.ButtonPressure, 0, 1023, StylusPointPropertyUnit.None, 1.0f); ////// XTiltOrientation /// internal static readonly StylusPointPropertyInfo XTiltOrientation = new StylusPointPropertyInfo(StylusPointProperties.XTiltOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// YTiltOrientation /// internal static readonly StylusPointPropertyInfo YTiltOrientation = new StylusPointPropertyInfo(StylusPointProperties.YTiltOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// AzimuthOrientation /// internal static readonly StylusPointPropertyInfo AzimuthOrientation = new StylusPointPropertyInfo(StylusPointProperties.AzimuthOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// AltitudeOrientation /// internal static readonly StylusPointPropertyInfo AltitudeOrientation = new StylusPointPropertyInfo(StylusPointProperties.AltitudeOrientation, -900, 900, StylusPointPropertyUnit.Degrees, 10.0f); ////// TwistOrientation /// internal static readonly StylusPointPropertyInfo TwistOrientation = new StylusPointPropertyInfo(StylusPointProperties.TwistOrientation, 0, 3600, StylusPointPropertyUnit.Degrees, 10.0f); ////// PitchRotation internal static readonly StylusPointPropertyInfo PitchRotation = new StylusPointPropertyInfo(StylusPointProperties.PitchRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// RollRotation internal static readonly StylusPointPropertyInfo RollRotation = new StylusPointPropertyInfo(StylusPointProperties.RollRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// YawRotation internal static readonly StylusPointPropertyInfo YawRotation = new StylusPointPropertyInfo(StylusPointProperties.YawRotation, Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0f); /// /// TipButton internal static readonly StylusPointPropertyInfo TipButton = new StylusPointPropertyInfo(StylusPointProperties.TipButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// BarrelButton internal static readonly StylusPointPropertyInfo BarrelButton = new StylusPointPropertyInfo(StylusPointProperties.BarrelButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// SecondaryTipButton internal static readonly StylusPointPropertyInfo SecondaryTipButton = new StylusPointPropertyInfo(StylusPointProperties.SecondaryTipButton, 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// Default Value /// internal static readonly StylusPointPropertyInfo DefaultValue = new StylusPointPropertyInfo(new StylusPointProperty(Guid.NewGuid(), false), Int32.MinValue, Int32.MaxValue, StylusPointPropertyUnit.None, 1.0F); ////// DefaultButton internal static readonly StylusPointPropertyInfo DefaultButton = new StylusPointPropertyInfo(new StylusPointProperty(Guid.NewGuid(), true), 0, 1, StylusPointPropertyUnit.None, 1.0f); /// /// For a given StylusPointProperty, return the default property info /// /// stylusPointProperty ///internal static StylusPointPropertyInfo GetStylusPointPropertyInfoDefault(StylusPointProperty stylusPointProperty) { if (stylusPointProperty.Id == StylusPointPropertyIds.X) { return StylusPointPropertyInfoDefaults.X; } if (stylusPointProperty.Id == StylusPointPropertyIds.Y) { return StylusPointPropertyInfoDefaults.Y; } if (stylusPointProperty.Id == StylusPointPropertyIds.Z) { return StylusPointPropertyInfoDefaults.Z; } if (stylusPointProperty.Id == StylusPointPropertyIds.Width) { return StylusPointPropertyInfoDefaults.Width; } if (stylusPointProperty.Id == StylusPointPropertyIds.Height) { return StylusPointPropertyInfoDefaults.Height; } if (stylusPointProperty.Id == StylusPointPropertyIds.SystemTouch) { return StylusPointPropertyInfoDefaults.SystemTouch; } if (stylusPointProperty.Id == StylusPointPropertyIds.PacketStatus) { return StylusPointPropertyInfoDefaults.PacketStatus; } if (stylusPointProperty.Id == StylusPointPropertyIds.SerialNumber) { return StylusPointPropertyInfoDefaults.SerialNumber; } if (stylusPointProperty.Id == StylusPointPropertyIds.NormalPressure) { return StylusPointPropertyInfoDefaults.NormalPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.TangentPressure) { return StylusPointPropertyInfoDefaults.TangentPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.ButtonPressure) { return StylusPointPropertyInfoDefaults.ButtonPressure; } if (stylusPointProperty.Id == StylusPointPropertyIds.XTiltOrientation) { return StylusPointPropertyInfoDefaults.XTiltOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.YTiltOrientation) { return StylusPointPropertyInfoDefaults.YTiltOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.AzimuthOrientation) { return StylusPointPropertyInfoDefaults.AzimuthOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.AltitudeOrientation) { return StylusPointPropertyInfoDefaults.AltitudeOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.TwistOrientation) { return StylusPointPropertyInfoDefaults.TwistOrientation; } if (stylusPointProperty.Id == StylusPointPropertyIds.PitchRotation) { return StylusPointPropertyInfoDefaults.PitchRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.RollRotation) { return StylusPointPropertyInfoDefaults.RollRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.YawRotation) { return StylusPointPropertyInfoDefaults.YawRotation; } if (stylusPointProperty.Id == StylusPointPropertyIds.TipButton) { return StylusPointPropertyInfoDefaults.TipButton; } if (stylusPointProperty.Id == StylusPointPropertyIds.BarrelButton) { return StylusPointPropertyInfoDefaults.BarrelButton; } if (stylusPointProperty.Id == StylusPointPropertyIds.SecondaryTipButton) { return StylusPointPropertyInfoDefaults.SecondaryTipButton; } // // return a default // if (stylusPointProperty.IsButton) { return StylusPointPropertyInfoDefaults.DefaultButton; } return StylusPointPropertyInfoDefaults.DefaultValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
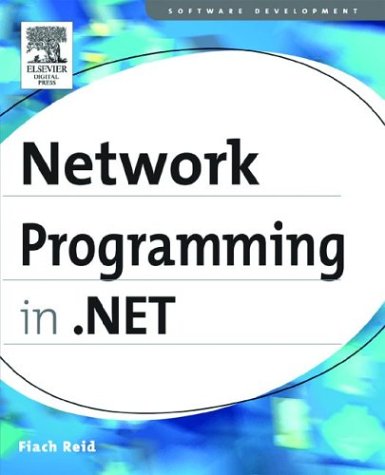
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BidOverLoads.cs
- SqlServer2KCompatibilityCheck.cs
- ControlBuilder.cs
- DataFormat.cs
- Renderer.cs
- WebPartCatalogCloseVerb.cs
- ServicePerformanceCounters.cs
- SQLByteStorage.cs
- StackOverflowException.cs
- JsonEnumDataContract.cs
- XsdBuildProvider.cs
- FixedHyperLink.cs
- IdentityElement.cs
- PrinterResolution.cs
- ReferenceList.cs
- LocalizabilityAttribute.cs
- System.Data_BID.cs
- SkipQueryOptionExpression.cs
- BamlCollectionHolder.cs
- TextCharacters.cs
- PtsHelper.cs
- TextParagraphView.cs
- StringWriter.cs
- ReflectionTypeLoadException.cs
- ResourceManagerWrapper.cs
- DocumentApplicationJournalEntry.cs
- CompiledIdentityConstraint.cs
- CreateUserWizard.cs
- ValidatingReaderNodeData.cs
- RadioButtonStandardAdapter.cs
- QuotedStringFormatReader.cs
- PiiTraceSource.cs
- PropertyDescriptorCollection.cs
- FixedTextView.cs
- GestureRecognizer.cs
- CaretElement.cs
- HttpHeaderCollection.cs
- HostingEnvironment.cs
- LinqDataSourceDeleteEventArgs.cs
- WsiProfilesElement.cs
- StringResourceManager.cs
- ProfileBuildProvider.cs
- XmlMembersMapping.cs
- QueryTaskGroupState.cs
- RayHitTestParameters.cs
- RelationshipWrapper.cs
- NameValueFileSectionHandler.cs
- HitTestResult.cs
- AuthenticationManager.cs
- WebMessageFormatHelper.cs
- ResourceExpressionEditorSheet.cs
- configsystem.cs
- BamlResourceDeserializer.cs
- SelectionRangeConverter.cs
- WindowsFont.cs
- SafeHandle.cs
- Executor.cs
- WaitForChangedResult.cs
- SpeechSynthesizer.cs
- Native.cs
- X509Utils.cs
- __ComObject.cs
- ValidationResult.cs
- BinaryMethodMessage.cs
- ContactManager.cs
- NamedPermissionSet.cs
- EmptyEnumerator.cs
- ReadWriteObjectLock.cs
- DataGridViewRowPrePaintEventArgs.cs
- ControlParameter.cs
- ScriptResourceHandler.cs
- JavaScriptSerializer.cs
- BaseDataList.cs
- ToolStripItemEventArgs.cs
- MdImport.cs
- SerializerDescriptor.cs
- ClientScriptManager.cs
- _ListenerAsyncResult.cs
- FullTextLine.cs
- EntryPointNotFoundException.cs
- PropertyChangedEventArgs.cs
- PartitionResolver.cs
- AttachedPropertyMethodSelector.cs
- StringComparer.cs
- HandledMouseEvent.cs
- Timeline.cs
- VScrollBar.cs
- DataServiceKeyAttribute.cs
- BevelBitmapEffect.cs
- DrawingContextWalker.cs
- AssemblyBuilder.cs
- DataGridViewCellCollection.cs
- ContextProperty.cs
- X509ScopedServiceCertificateElement.cs
- Vector3DKeyFrameCollection.cs
- StringBuilder.cs
- PublishLicense.cs
- RegexCaptureCollection.cs
- SplayTreeNode.cs
- InputLanguageCollection.cs