Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / Panel.cs / 1 / Panel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Windows.Forms.Layout; ////// /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultProperty("BorderStyle"), DefaultEvent("Paint"), Docking(DockingBehavior.Ask), Designer("System.Windows.Forms.Design.PanelDesigner, " + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionPanel) ] public class Panel : ScrollableControl { private BorderStyle borderStyle = System.Windows.Forms.BorderStyle.None; ////// Represents a ////// control. /// /// public Panel() : base() { // this class overrides GetPreferredSizeCore, let Control automatically cache the result SetState2(STATE2_USEPREFERREDSIZECACHE, true); TabStop = false; SetStyle(ControlStyles.Selectable | ControlStyles.AllPaintingInWmPaint, false); SetStyle(ControlStyles.SupportsTransparentBackColor, true); } ///Initializes a new instance of the ///class. /// /// [Browsable(true), EditorBrowsable(EditorBrowsableState.Always), DesignerSerializationVisibility(DesignerSerializationVisibility.Visible)] public override bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ///Override to re-expose AutoSize. ///[SRCategory(SR.CatPropertyChanged), SRDescription(SR.ControlOnAutoSizeChangedDescr)] [Browsable(true), EditorBrowsable(EditorBrowsableState.Always)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// Allows the control to optionally shrink when AutoSize is true. /// [ SRDescription(SR.ControlAutoSizeModeDescr), SRCategory(SR.CatLayout), Browsable(true), DefaultValue(AutoSizeMode.GrowOnly), Localizable(true) ] public virtual AutoSizeMode AutoSizeMode { get { return GetAutoSizeMode(); } set { if (!ClientUtils.IsEnumValid(value, (int)value, (int)AutoSizeMode.GrowAndShrink, (int)AutoSizeMode.GrowOnly)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(AutoSizeMode)); } if (GetAutoSizeMode() != value) { SetAutoSizeMode(value); if(ParentInternal != null) { // DefaultLayout does not keep anchor information until it needs to. When // AutoSize became a common property, we could no longer blindly call into // DefaultLayout, so now we do a special InitLayout just for DefaultLayout. if(ParentInternal.LayoutEngine == DefaultLayout.Instance) { ParentInternal.LayoutEngine.InitLayout(this, BoundsSpecified.Size); } LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.AutoSize); } } } } ////// /// [ SRCategory(SR.CatAppearance), DefaultValue(BorderStyle.None), DispId(NativeMethods.ActiveX.DISPID_BORDERSTYLE), SRDescription(SR.PanelBorderStyleDescr) ] public BorderStyle BorderStyle { get { return borderStyle; } set { if (borderStyle != value) { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)BorderStyle.None, (int)BorderStyle.Fixed3D)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(BorderStyle)); } borderStyle = value; UpdateStyles(); } } } ///Indicates the /// border style for the control. ////// /// /// Returns the parameters needed to create the handle. Inheriting classes /// can override this to provide extra functionality. They should not, /// however, forget to call base.getCreateParams() first to get the struct /// filled up with the basic info. /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.ExStyle |= NativeMethods.WS_EX_CONTROLPARENT; cp.ExStyle &= (~NativeMethods.WS_EX_CLIENTEDGE); cp.Style &= (~NativeMethods.WS_BORDER); switch (borderStyle) { case BorderStyle.Fixed3D: cp.ExStyle |= NativeMethods.WS_EX_CLIENTEDGE; break; case BorderStyle.FixedSingle: cp.Style |= NativeMethods.WS_BORDER; break; } return cp; } } ////// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Size DefaultSize { get { return new Size(200, 100); } } internal override Size GetPreferredSizeCore(Size proposedSize) { // Translating 0,0 from ClientSize to actual Size tells us how much space // is required for the borders. Size borderSize = SizeFromClientSize(Size.Empty); Size totalPadding = borderSize + Padding.Size; return LayoutEngine.GetPreferredSize(this, proposedSize - totalPadding) + totalPadding; } ////// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event KeyEventHandler KeyUp { add { base.KeyUp += value; } remove { base.KeyUp -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event KeyEventHandler KeyDown { add { base.KeyDown += value; } remove { base.KeyDown -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event KeyPressEventHandler KeyPress { add { base.KeyPress += value; } remove { base.KeyPress -= value; } } /// /// /// [DefaultValue(false)] new public bool TabStop { get { return base.TabStop; } set { base.TabStop = value; } } ////// /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), Bindable(false)] public override string Text { get { return base.Text; } set { base.Text = value; } } ////// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler TextChanged { add { base.TextChanged += value; } remove { base.TextChanged -= value; } } /// /// /// /// protected override void OnResize(EventArgs eventargs) { if (DesignMode && borderStyle == BorderStyle.None) { Invalidate(); } base.OnResize(eventargs); } internal override void PrintToMetaFileRecursive(HandleRef hDC, IntPtr lParam, Rectangle bounds) { base.PrintToMetaFileRecursive(hDC, lParam, bounds); using (WindowsFormsUtils.DCMapping mapping = new WindowsFormsUtils.DCMapping(hDC, bounds)) { using(Graphics g = Graphics.FromHdcInternal(hDC.Handle)) { ControlPaint.PrintBorder(g, new Rectangle(Point.Empty, Size), BorderStyle, Border3DStyle.Sunken); } } } ///Fires the event indicating that the panel has been resized. /// Inheriting controls should use this in favour of actually listening to /// the event, but should not forget to call base.onResize() to /// ensure that the event is still fired for external listeners. ////// /// ///private static string StringFromBorderStyle(BorderStyle value) { Type borderStyleType = typeof(BorderStyle); return (ClientUtils.IsEnumValid(value, (int)value, (int)BorderStyle.None, (int)BorderStyle.Fixed3D)) ? (borderStyleType.ToString() + "." + value.ToString()) : "[Invalid BorderStyle]"; } /// /// /// Returns a string representation for this control. /// ///public override string ToString() { string s = base.ToString(); return s + ", BorderStyle: " + StringFromBorderStyle(borderStyle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
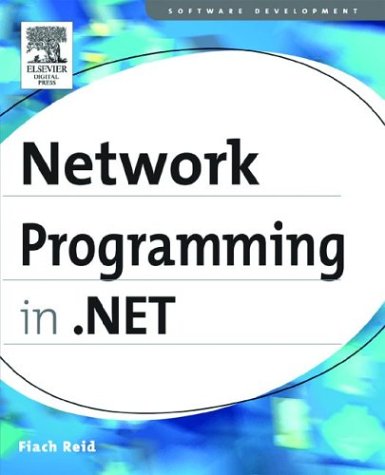
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- mediaclock.cs
- DispatcherObject.cs
- FlowDocumentFormatter.cs
- KnownBoxes.cs
- XmlArrayAttribute.cs
- x509store.cs
- CustomExpression.cs
- CreateUserWizard.cs
- CookieProtection.cs
- ClientTarget.cs
- TemplateApplicationHelper.cs
- DoubleLink.cs
- PriorityChain.cs
- _ShellExpression.cs
- Int64AnimationUsingKeyFrames.cs
- GPRECT.cs
- TypedTableBaseExtensions.cs
- RtfControlWordInfo.cs
- BuildResultCache.cs
- RowCache.cs
- Icon.cs
- TableStyle.cs
- Frame.cs
- DynamicDataManager.cs
- InvalidDataContractException.cs
- GatewayDefinition.cs
- OneOfConst.cs
- NamespaceInfo.cs
- Comparer.cs
- VirtualDirectoryMappingCollection.cs
- X509ChainElement.cs
- RegistrySecurity.cs
- CroppedBitmap.cs
- PartitionResolver.cs
- WeakRefEnumerator.cs
- IPipelineRuntime.cs
- ModuleBuilderData.cs
- DoubleConverter.cs
- EntityViewContainer.cs
- NativeObjectSecurity.cs
- FixedSchema.cs
- InternalsVisibleToAttribute.cs
- altserialization.cs
- InstanceView.cs
- BasicAsyncResult.cs
- SettingsAttributeDictionary.cs
- MimeXmlReflector.cs
- CommentEmitter.cs
- QilInvoke.cs
- regiisutil.cs
- ellipse.cs
- DataSetFieldSchema.cs
- SectionVisual.cs
- MLangCodePageEncoding.cs
- TextAutomationPeer.cs
- DataPagerFieldItem.cs
- CalendarDay.cs
- SimpleApplicationHost.cs
- IUnknownConstantAttribute.cs
- XmlNodeWriter.cs
- DataStreams.cs
- ApplicationManager.cs
- AsymmetricKeyExchangeFormatter.cs
- ExpressionLexer.cs
- DbConnectionPoolGroup.cs
- UnmanagedHandle.cs
- ManualResetEvent.cs
- Quaternion.cs
- ReadOnlyDataSourceView.cs
- LocalValueEnumerator.cs
- EntityCommand.cs
- SatelliteContractVersionAttribute.cs
- ObjectTypeMapping.cs
- LayoutEditorPart.cs
- ErrorFormatterPage.cs
- Attribute.cs
- EntityContainerEmitter.cs
- GenericAuthenticationEventArgs.cs
- NotifyParentPropertyAttribute.cs
- TextEditorParagraphs.cs
- SharedStatics.cs
- SettingsPropertyCollection.cs
- TextElementCollectionHelper.cs
- SymmetricCryptoHandle.cs
- SignedXml.cs
- VariableQuery.cs
- UpdateCommand.cs
- FontFamilyValueSerializer.cs
- StaticResourceExtension.cs
- PipelineModuleStepContainer.cs
- TextContainerChangeEventArgs.cs
- CodeTypeParameterCollection.cs
- XslCompiledTransform.cs
- CompositeDesignerAccessibleObject.cs
- ActivityWithResultConverter.cs
- XmlBinaryWriter.cs
- WebPartMenuStyle.cs
- ObjectSecurity.cs
- ConfigurationLockCollection.cs
- webeventbuffer.cs