Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DrawListViewItemEventArgs.cs / 1 / DrawListViewItemEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView items. /// public class DrawListViewItemEventArgs : EventArgs { private readonly Graphics graphics; private readonly ListViewItem item; private readonly Rectangle bounds; private readonly int itemIndex; private readonly ListViewItemStates state; private bool drawDefault; ////// /// Creates a new DrawListViewItemEventArgs with the given parameters. /// public DrawListViewItemEventArgs(Graphics graphics, ListViewItem item, Rectangle bounds, int itemIndex, ListViewItemStates state) { this.graphics = graphics; this.item = item; this.bounds = bounds; this.itemIndex = itemIndex; this.state = state; this.drawDefault = false; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The item to be painted. /// public ListViewItem Item { get { return item; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The index of the item that should be painted. /// public int ItemIndex { get { return itemIndex; } } ////// /// Miscellaneous state information. /// public ListViewItemStates State { get { return state; } } ////// /// Draws the item's background. /// public void DrawBackground() { Brush backBrush = new SolidBrush(item.BackColor); Graphics.FillRectangle(backBrush, bounds); backBrush.Dispose(); } ////// /// Draws a focus rectangle in the given bounds, if the item is focused. In Details View, if FullRowSelect is /// true, the rectangle is drawn around the whole item, else around the first sub-item's text area. /// public void DrawFocusRectangle() { if ((state & ListViewItemStates.Focused) == ListViewItemStates.Focused) { Rectangle focusBounds = bounds; ControlPaint.DrawFocusRectangle(graphics, UpdateBounds(focusBounds, false /*drawText*/), item.ForeColor, item.BackColor); } } ////// /// Draws the item's text (overloaded) - useful only when View != View.Details /// public void DrawText() { DrawText(TextFormatFlags.Left); } ////// /// Draws the item's text (overloaded) - useful only when View != View.Details - takes a TextFormatFlags argument. /// public void DrawText(TextFormatFlags flags) { TextRenderer.DrawText(graphics, item.Text, item.Font, UpdateBounds(bounds, true /*drawText*/), item.ForeColor, flags); } private Rectangle UpdateBounds(Rectangle originalBounds, bool drawText) { Rectangle resultBounds = originalBounds; if (item.ListView.View == View.Details) { // Note: this logic will compute the bounds so they align w/ the system drawn bounds only // for the default font. if (!item.ListView.FullRowSelect && item.SubItems.Count > 0) { ListViewItem.ListViewSubItem subItem = item.SubItems[0]; Size textSize = TextRenderer.MeasureText(subItem.Text, subItem.Font); resultBounds = new Rectangle(originalBounds.X, originalBounds.Y, textSize.Width, textSize.Height); // Add some padding so we paint like the system control paints. resultBounds.X += 4; resultBounds.Width ++; } else { resultBounds.X +=4; resultBounds.Width -= 4; } if (drawText) { resultBounds.X --; } } return resultBounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
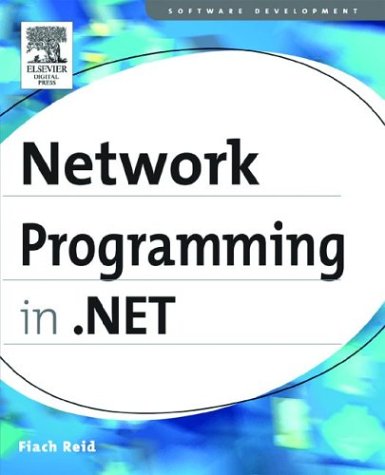
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Color.cs
- ReflectEventDescriptor.cs
- FigureHelper.cs
- TreeNodeCollection.cs
- PropertyInfoSet.cs
- TabletDevice.cs
- PageClientProxyGenerator.cs
- NamespaceDecl.cs
- HtmlEncodedRawTextWriter.cs
- JsonQNameDataContract.cs
- DataGridViewColumnStateChangedEventArgs.cs
- DataListCommandEventArgs.cs
- CodeChecksumPragma.cs
- CodeParameterDeclarationExpressionCollection.cs
- FormsAuthenticationEventArgs.cs
- BlockUIContainer.cs
- ExtensionSimplifierMarkupObject.cs
- ReadOnlyCollection.cs
- EntityProviderFactory.cs
- BasicBrowserDialog.cs
- SpeakCompletedEventArgs.cs
- SQLStringStorage.cs
- GcHandle.cs
- HttpServerUtilityBase.cs
- Converter.cs
- XmlIncludeAttribute.cs
- BitmapEffectState.cs
- SqlTriggerContext.cs
- CommentEmitter.cs
- GraphicsContainer.cs
- EdmProperty.cs
- DataGridViewColumnCollectionDialog.cs
- Calendar.cs
- TripleDESCryptoServiceProvider.cs
- Point.cs
- UrlMappingsSection.cs
- ThreadTrace.cs
- ObjectViewFactory.cs
- UntrustedRecipientException.cs
- ControlBuilder.cs
- ReaderOutput.cs
- MemberPath.cs
- Path.cs
- KeyManager.cs
- ButtonChrome.cs
- SimpleWorkerRequest.cs
- ShaderEffect.cs
- UniqueIdentifierService.cs
- FormViewUpdatedEventArgs.cs
- SystemIPGlobalStatistics.cs
- Classification.cs
- ToolStripContainerActionList.cs
- SettingsSection.cs
- Point3DIndependentAnimationStorage.cs
- ClientData.cs
- EnumerableValidator.cs
- ReaderWriterLock.cs
- WebPartUtil.cs
- ProvidePropertyAttribute.cs
- ElasticEase.cs
- StyleCollection.cs
- LocalBuilder.cs
- ScrollProperties.cs
- SiteMapDataSourceView.cs
- BindingList.cs
- TextContainer.cs
- SizeConverter.cs
- ExpressionBuilderContext.cs
- HelpEvent.cs
- SqlInfoMessageEvent.cs
- LinqDataSourceValidationException.cs
- WebConfigManager.cs
- basecomparevalidator.cs
- AnalyzedTree.cs
- TreeNodeConverter.cs
- GridViewDeletedEventArgs.cs
- httpstaticobjectscollection.cs
- HtmlElementErrorEventArgs.cs
- GACMembershipCondition.cs
- ShaderEffect.cs
- SafeNativeMethods.cs
- WpfGeneratedKnownProperties.cs
- DBSqlParserTable.cs
- MemberRelationshipService.cs
- ColumnResizeUndoUnit.cs
- TextAutomationPeer.cs
- SafeNativeMethods.cs
- PropertyMapper.cs
- ToolStripScrollButton.cs
- XmlArrayAttribute.cs
- NullEntityWrapper.cs
- RepeaterItemCollection.cs
- UnsafeNativeMethods.cs
- FormViewPagerRow.cs
- ButtonField.cs
- WindowsSlider.cs
- ProcessModuleDesigner.cs
- XDRSchema.cs
- WindowHideOrCloseTracker.cs
- path.cs