Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2ColorConverter.cs / 1 / COM2ColorConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; ////// /// This class maps an OLE_COLOR to a managed Color editor. /// internal class Com2ColorConverter : Com2DataTypeToManagedDataTypeConverter{ ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType{ get{ return typeof(Color); } } ////// /// Converts the native value into a managed value /// public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd){ object baseValue = nativeValue; int intVal = 0; // get the integer value out of the native... // if (nativeValue is UInt32){ intVal = (int)(UInt32)nativeValue; } else if (nativeValue is Int32){ intVal = (int)nativeValue; } return ColorTranslator.FromOle(intVal); } ////// /// Converts the managed value into a native value /// public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet){ // don't cancel the set cancelSet = false; // we default to black. // if (managedValue == null){ managedValue = Color.Black; } if (managedValue is Color){ return ColorTranslator.ToOle(((Color)managedValue)); } Debug.Fail("Don't know how to set type:" + managedValue.GetType().Name); return 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
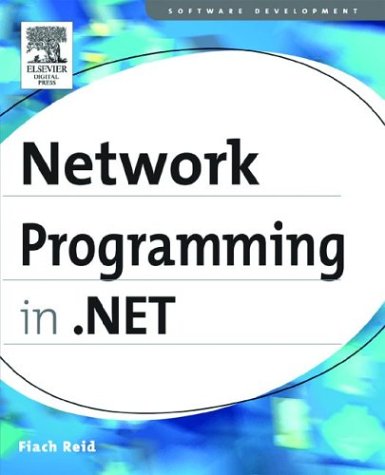
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryInterceptorAttribute.cs
- _TLSstream.cs
- XamlPointCollectionSerializer.cs
- DynamicField.cs
- DependencyPropertyDescriptor.cs
- LOSFormatter.cs
- ListItemConverter.cs
- FindSimilarActivitiesVerb.cs
- ContentType.cs
- Trace.cs
- WindowsFormsLinkLabel.cs
- TransactionFlowOption.cs
- VerificationAttribute.cs
- WaitHandleCannotBeOpenedException.cs
- UrlAuthorizationModule.cs
- XamlNamespaceHelper.cs
- ClientConfigPaths.cs
- ResolveMatchesApril2005.cs
- DirectoryObjectSecurity.cs
- IndexedGlyphRun.cs
- DataObjectAttribute.cs
- SelectionHighlightInfo.cs
- TypeHelper.cs
- EntityDataSourceWrapper.cs
- BamlResourceDeserializer.cs
- FilterElement.cs
- FaultPropagationRecord.cs
- FieldNameLookup.cs
- InkCanvasSelection.cs
- DNS.cs
- SourceElementsCollection.cs
- CompositeDesignerAccessibleObject.cs
- Latin1Encoding.cs
- XmlConverter.cs
- WbemProvider.cs
- XmlSerializerSection.cs
- QilName.cs
- PermissionToken.cs
- CodeGenerator.cs
- InvalidDataException.cs
- SqlDataSourceEnumerator.cs
- XmlCharCheckingReader.cs
- WebPartMinimizeVerb.cs
- SoapSchemaExporter.cs
- BufferedReceiveManager.cs
- EntityDataSource.cs
- RelativeSource.cs
- SpecialFolderEnumConverter.cs
- ProgressChangedEventArgs.cs
- ProcessThreadCollection.cs
- SqlTransaction.cs
- PeerChannelListener.cs
- GeneralTransform3DGroup.cs
- XmlSchemaDocumentation.cs
- TypeUtil.cs
- CommandValueSerializer.cs
- SiteMapHierarchicalDataSourceView.cs
- SafeCryptHandles.cs
- AncestorChangedEventArgs.cs
- SourceExpressionException.cs
- ExpressionEditorSheet.cs
- CodeDOMProvider.cs
- RotateTransform.cs
- DropDownList.cs
- CornerRadiusConverter.cs
- IISMapPath.cs
- GridViewActionList.cs
- ContactManager.cs
- NativeMethods.cs
- MessageBuilder.cs
- InputLanguageProfileNotifySink.cs
- NetTcpSection.cs
- StaticFileHandler.cs
- SuppressIldasmAttribute.cs
- SqlMethodCallConverter.cs
- GeneralTransform3DTo2DTo3D.cs
- ProfessionalColors.cs
- IndependentAnimationStorage.cs
- FixedSOMPageConstructor.cs
- Delegate.cs
- DbProviderConfigurationHandler.cs
- CopyAttributesAction.cs
- IsolatedStoragePermission.cs
- DefaultBindingPropertyAttribute.cs
- WorkflowOperationErrorHandler.cs
- ObfuscateAssemblyAttribute.cs
- DigestComparer.cs
- _OverlappedAsyncResult.cs
- StringAttributeCollection.cs
- GridPatternIdentifiers.cs
- StateRuntime.cs
- ObjectFullSpanRewriter.cs
- SqlNode.cs
- BasicHttpMessageSecurityElement.cs
- ResourceCategoryAttribute.cs
- RijndaelManaged.cs
- TimeSpan.cs
- ConstNode.cs
- CacheDependency.cs
- FunctionDetailsReader.cs