Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Services / Monitoring / system / Diagnosticts / Design / StringDictionaryCodeDomSerializer.cs / 1 / StringDictionaryCodeDomSerializer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics.Design { using System; using System.Design; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Reflection; using System.ComponentModel.Design.Serialization; using System.Collections.Specialized; ////// /// This serializer serializes string dictionaries. /// internal class StringDictionaryCodeDomSerializer : CodeDomSerializer { ////// /// This method takes a CodeDomObject and deserializes into a real object. /// We don't do anything here. /// public override object Deserialize(IDesignerSerializationManager manager, object codeObject) { Debug.Fail("Don't expect this to be called."); return null; } ////// /// Serializes the given object into a CodeDom object. /// public override object Serialize(IDesignerSerializationManager manager, object value) { object result = null; StringDictionary dictionary = value as StringDictionary; if (dictionary != null) { object context = manager.Context.Current; ExpressionContext exp = context as ExpressionContext; if (exp != null) { if (exp.Owner == value) { context = exp.Expression; } } // we can only serialize if we have a CodePropertyReferenceExpression CodePropertyReferenceExpression propRef = context as CodePropertyReferenceExpression; if (propRef != null) { // get the object with the property we're setting object targetObject = DeserializeExpression(manager, null, propRef.TargetObject); if (targetObject != null) { // get the PropertyDescriptor of the property we're setting PropertyDescriptor prop = TypeDescriptor.GetProperties(targetObject)[propRef.PropertyName]; if (prop != null) { // okay, we have the property and we have the StringDictionary, now we generate // a line like this (c# example): // myObject.strDictProp["key"] = "value"; // for each key/value pair in the StringDictionary CodeStatementCollection statements = new CodeStatementCollection(); CodeMethodReferenceExpression methodRef = new CodeMethodReferenceExpression(propRef, "Add"); foreach (DictionaryEntry entry in dictionary) { // serialize the key (in most languages this will look like "key") CodeExpression serializedKey = SerializeToExpression(manager, entry.Key); // serialize the value (in most languages this will look like "value") CodeExpression serializedValue = SerializeToExpression(manager, entry.Value); // serialize the method call (prop.Add("key", "value")) if (serializedKey != null && serializedValue != null) { CodeMethodInvokeExpression statement = new CodeMethodInvokeExpression(); statement.Method = methodRef; statement.Parameters.Add(serializedKey); statement.Parameters.Add(serializedValue); statements.Add(statement); } } result = statements; } } } } else { // 'value' is not a StringDictionary. What should we do? } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
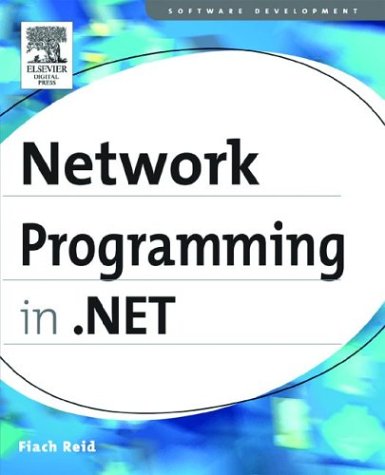
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemNetworkInterface.cs
- SharedStatics.cs
- DefaultAsyncDataDispatcher.cs
- SafeCryptoHandles.cs
- categoryentry.cs
- XPathBuilder.cs
- Funcletizer.cs
- SafeRightsManagementPubHandle.cs
- ResponseStream.cs
- SimpleHandlerBuildProvider.cs
- altserialization.cs
- Range.cs
- NetworkInformationException.cs
- ColumnResizeUndoUnit.cs
- VisualStyleTypesAndProperties.cs
- TopClause.cs
- TextServicesProperty.cs
- LinqDataSource.cs
- ControlSerializer.cs
- CodeTypeDeclarationCollection.cs
- EdmProperty.cs
- CursorInteropHelper.cs
- GcSettings.cs
- ImmutablePropertyDescriptorGridEntry.cs
- Vector3DConverter.cs
- IISMapPath.cs
- ProjectionCamera.cs
- PersonalizationStateInfoCollection.cs
- WebPartCloseVerb.cs
- SchemaInfo.cs
- ControlValuePropertyAttribute.cs
- Misc.cs
- ClientBase.cs
- DrawTreeNodeEventArgs.cs
- EditingCommands.cs
- WebScriptServiceHost.cs
- documentsequencetextcontainer.cs
- SizeF.cs
- ToolTip.cs
- CommandID.cs
- Listbox.cs
- MaterialGroup.cs
- EmptyImpersonationContext.cs
- MatchingStyle.cs
- ConversionValidationRule.cs
- SqlRowUpdatedEvent.cs
- CheckBoxRenderer.cs
- AnonymousIdentificationModule.cs
- CommandID.cs
- TextRangeAdaptor.cs
- DataGridViewRowPrePaintEventArgs.cs
- TextBreakpoint.cs
- Permission.cs
- ExternalFile.cs
- validation.cs
- BinaryFormatterWriter.cs
- BindingWorker.cs
- AnimatedTypeHelpers.cs
- PolicyManager.cs
- StateMachineHelpers.cs
- CodeMethodInvokeExpression.cs
- DataRecordInternal.cs
- DictionaryKeyPropertyAttribute.cs
- OleCmdHelper.cs
- CommandDevice.cs
- CalendarButton.cs
- CodeDelegateCreateExpression.cs
- XmlRawWriter.cs
- WorkItem.cs
- XamlDesignerSerializationManager.cs
- SqlEnums.cs
- SerializationInfo.cs
- DataGridViewImageCell.cs
- DoubleUtil.cs
- AppDomainFactory.cs
- TailCallAnalyzer.cs
- configsystem.cs
- SByteConverter.cs
- __ConsoleStream.cs
- GeneralTransform3DGroup.cs
- MsmqIntegrationBindingCollectionElement.cs
- FixedSOMContainer.cs
- ControlUtil.cs
- ExpandoObject.cs
- XpsS0ValidatingLoader.cs
- Preprocessor.cs
- IndexerNameAttribute.cs
- Trace.cs
- WinFormsSecurity.cs
- OleStrCAMarshaler.cs
- PhoneCallDesigner.cs
- DataMemberAttribute.cs
- ImageAutomationPeer.cs
- CommandLibraryHelper.cs
- SQLRoleProvider.cs
- ImageBrush.cs
- SqlCharStream.cs
- BitmapFrameDecode.cs
- Utils.cs
- Quaternion.cs