Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / TabPageDesigner.cs / 1 / TabPageDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Diagnostics; using System.Collections; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.ComponentModel.Design; using System.Windows.Forms.Design.Behavior; using System.ComponentModel; ////// /// This is the designer for tap page controls. It inherits /// from the base control designer and adds live hit testing /// capabilites for the tree view control. /// internal class TabPageDesigner : PanelDesigner { ////// /// Determines if the this designer can be parented to the specified desinger -- /// generally this means if the control for this designer can be parented into the /// given ParentControlDesigner's designer. /// public override bool CanBeParentedTo(IDesigner parentDesigner) { return (parentDesigner != null && parentDesigner.Component is TabControl); } ////// /// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = base.SelectionRules; Control ctl = Control; if (ctl.Parent is TabControl) { rules &= ~SelectionRules.AllSizeable; } return rules; } } internal void OnDragDropInternal(DragEventArgs de) { OnDragDrop(de); } internal void OnDragEnterInternal(DragEventArgs de) { OnDragEnter(de); } internal void OnDragLeaveInternal(EventArgs e) { OnDragLeave(e); } internal void OnDragOverInternal(DragEventArgs e) { OnDragOver(e); } internal void OnGiveFeedbackInternal(GiveFeedbackEventArgs e) { OnGiveFeedback(e); } protected override ControlBodyGlyph GetControlGlyph(GlyphSelectionType selectionType) { // create a new body glyph with empty bounds. // this will keep incorrect tab pages from stealing drag/drop messages // which are now handled by the TabControlDesigner //get the right cursor for this component OnSetCursor(); Rectangle translatedBounds = Rectangle.Empty; //create our glyph, and set its cursor appropriately ControlBodyGlyph g = new ControlBodyGlyph(translatedBounds, Cursor.Current, Control, this); return g; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
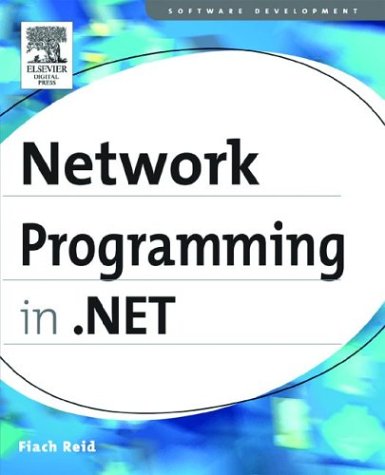
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BoundingRectTracker.cs
- Span.cs
- TextPointer.cs
- DataGridViewColumnDesigner.cs
- RegexCapture.cs
- Int32RectValueSerializer.cs
- AsyncResult.cs
- CultureTable.cs
- SecurityContext.cs
- NamespaceInfo.cs
- DispatcherTimer.cs
- PerformanceCounterManager.cs
- CachedBitmap.cs
- BindUriHelper.cs
- FontStyleConverter.cs
- Point4D.cs
- WindowPatternIdentifiers.cs
- RandomNumberGenerator.cs
- ExpressionEditorAttribute.cs
- RefreshPropertiesAttribute.cs
- PartialCachingControl.cs
- Rotation3DAnimation.cs
- XmlNode.cs
- XmlAttributeProperties.cs
- ConditionalAttribute.cs
- AutomationIdentifierGuids.cs
- SafeProcessHandle.cs
- DrawingGroup.cs
- CompositeActivityCodeGenerator.cs
- ToolStripDropDownButton.cs
- Token.cs
- LineBreakRecord.cs
- SystemResourceKey.cs
- ReflectionUtil.cs
- SerializationObjectManager.cs
- StylusTip.cs
- BCLDebug.cs
- Bold.cs
- ToolStripDropDownButton.cs
- SafeNativeMethods.cs
- SignedXml.cs
- TargetParameterCountException.cs
- NetDataContractSerializer.cs
- ProtocolsConfigurationHandler.cs
- NativeMethods.cs
- SmtpLoginAuthenticationModule.cs
- EntityDesignerDataSourceView.cs
- VariableAction.cs
- Panel.cs
- MimeTextImporter.cs
- ResponseStream.cs
- WebPartCloseVerb.cs
- CharAnimationBase.cs
- Pointer.cs
- IPEndPoint.cs
- DataObjectPastingEventArgs.cs
- GridViewCellAutomationPeer.cs
- IMembershipProvider.cs
- RectangleConverter.cs
- OdbcConnection.cs
- WebRequestModulesSection.cs
- OnOperation.cs
- DataReceivedEventArgs.cs
- FileLoadException.cs
- Misc.cs
- QilBinary.cs
- TemplateBamlTreeBuilder.cs
- TableItemStyle.cs
- RowTypePropertyElement.cs
- HtmlElementCollection.cs
- CompilationLock.cs
- DataGridViewCellParsingEventArgs.cs
- CounterCreationData.cs
- XmlReflectionImporter.cs
- AnnotationObservableCollection.cs
- PropertyNames.cs
- ImageIndexConverter.cs
- EntityDataSourceSelectedEventArgs.cs
- BaseResourcesBuildProvider.cs
- GridViewHeaderRowPresenter.cs
- LocatorPart.cs
- InitializerFacet.cs
- SharedStream.cs
- WindowsListViewItem.cs
- DataGridComponentEditor.cs
- DataGridViewImageCell.cs
- Msmq.cs
- BoundPropertyEntry.cs
- XamlHostingSectionGroup.cs
- TemplateApplicationHelper.cs
- VirtualPath.cs
- BinaryWriter.cs
- QueryCacheKey.cs
- DSASignatureDeformatter.cs
- CommandEventArgs.cs
- AuthenticatedStream.cs
- Utility.cs
- MobileUserControlDesigner.cs
- Lease.cs
- FactoryGenerator.cs