Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / DataSourceIDConverter.cs / 1 / DataSourceIDConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Runtime.InteropServices; using System.Globalization; using System.Collections.Generic; ///public class DataSourceIDConverter : TypeConverter { /// public DataSourceIDConverter() { } /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { string[] idsArray = null; if (context != null) { WebFormsRootDesigner rootDesigner = null; IDesignerHost designerHost = (IDesignerHost)(context.GetService(typeof(IDesignerHost))); Debug.Assert(designerHost != null, "Did not get DesignerHost service."); if (designerHost != null) { IComponent rootComponent = designerHost.RootComponent; if (rootComponent != null) { rootDesigner = designerHost.GetDesigner(rootComponent) as WebFormsRootDesigner; } } if (rootDesigner != null && !rootDesigner.IsDesignerViewLocked) { // Walk up the list of naming containers to get all accessible data sources IComponent component = context.Instance as IComponent; if (component == null) { // In case we are hosted in a DesignerActionList we need // to find out the component that the action list belongs to DesignerActionList actionList = context.Instance as DesignerActionList; if (actionList != null) { component = actionList.Component; } } IList allComponents = ControlHelper.GetAllComponents(component, new ControlHelper.IsValidComponentDelegate(IsValidDataSource)); List uniqueControlIDs = new List (); foreach (IComponent c in allComponents) { Control control = c as Control; if (control != null && !String.IsNullOrEmpty(control.ID)) { if (!uniqueControlIDs.Contains(control.ID)) { uniqueControlIDs.Add(control.ID); } } } uniqueControlIDs.Sort(StringComparer.OrdinalIgnoreCase); uniqueControlIDs.Insert(0, SR.GetString(SR.DataSourceIDChromeConverter_NoDataSource)); uniqueControlIDs.Add(SR.GetString(SR.DataSourceIDChromeConverter_NewDataSource)); idsArray = uniqueControlIDs.ToArray(); } } return new StandardValuesCollection(idsArray); } /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } /// protected virtual bool IsValidDataSource(IComponent component) { Control control = component as Control; if (control == null) { return false; } if (String.IsNullOrEmpty(control.ID)) { return false; } return (component is IDataSource); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
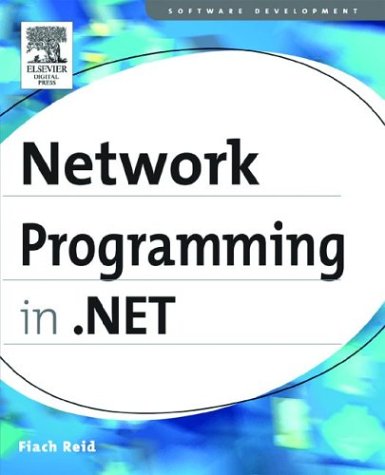
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidatingPropertiesEventArgs.cs
- VirtualPathUtility.cs
- ImageIndexConverter.cs
- DataGridViewCellParsingEventArgs.cs
- BaseTemplateParser.cs
- DropShadowEffect.cs
- StorageAssociationSetMapping.cs
- CatalogZoneDesigner.cs
- RelationshipEndMember.cs
- InternalConfigRoot.cs
- BasicHttpMessageSecurityElement.cs
- Speller.cs
- ReadOnlyDataSourceView.cs
- OpenFileDialog.cs
- MemoryStream.cs
- FunctionDetailsReader.cs
- TextViewSelectionProcessor.cs
- _UriSyntax.cs
- EpmSyndicationContentSerializer.cs
- PrinterUnitConvert.cs
- RequestBringIntoViewEventArgs.cs
- HttpPostProtocolImporter.cs
- BooleanAnimationBase.cs
- Operators.cs
- FilterException.cs
- ListViewItem.cs
- ListInitExpression.cs
- Msec.cs
- EntityDataSourceView.cs
- CodeTypeOfExpression.cs
- BitmapPalette.cs
- SqlDependency.cs
- WorkflowInvoker.cs
- ColorBlend.cs
- ContextMenu.cs
- NamedServiceModelExtensionCollectionElement.cs
- PaintEvent.cs
- PropertyDescriptorComparer.cs
- FunctionUpdateCommand.cs
- NetworkStream.cs
- ApplyTemplatesAction.cs
- Bidi.cs
- CategoryNameCollection.cs
- SystemBrushes.cs
- DynamicDataRoute.cs
- FontWeight.cs
- ISFClipboardData.cs
- FixedFindEngine.cs
- WebPartAuthorizationEventArgs.cs
- RuleEngine.cs
- TabItemAutomationPeer.cs
- WebPartDisplayModeEventArgs.cs
- DataControlField.cs
- ResourcePool.cs
- MouseActionValueSerializer.cs
- SessionEndingEventArgs.cs
- CornerRadius.cs
- KerberosReceiverSecurityToken.cs
- SafeFileHandle.cs
- ProfileService.cs
- StructuralObject.cs
- DeobfuscatingStream.cs
- ConfigsHelper.cs
- HostProtectionPermission.cs
- DurationConverter.cs
- DrawListViewColumnHeaderEventArgs.cs
- BindingUtils.cs
- LinqDataSourceUpdateEventArgs.cs
- NullableDoubleMinMaxAggregationOperator.cs
- HttpModuleAction.cs
- CharUnicodeInfo.cs
- SoapInteropTypes.cs
- SqlDependencyListener.cs
- FixedElement.cs
- UnauthorizedWebPart.cs
- DiscoveryVersionConverter.cs
- TableLayoutPanelBehavior.cs
- FontWeightConverter.cs
- ScrollProperties.cs
- FocusTracker.cs
- ColorBlend.cs
- ObjectDataSource.cs
- KnownTypesProvider.cs
- X509Chain.cs
- ResolveDuplex11AsyncResult.cs
- PreProcessInputEventArgs.cs
- QueryCacheEntry.cs
- TreeViewDataItemAutomationPeer.cs
- Padding.cs
- DataPagerField.cs
- StylusPointDescription.cs
- DbConnectionPool.cs
- ImportContext.cs
- TemplateBamlRecordReader.cs
- Funcletizer.cs
- StateBag.cs
- ContractMapping.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- FileDataSourceCache.cs