Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / HtmlControlDesigner.cs / 1 / HtmlControlDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System.Design; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Web.UI; using System.Web.UI.WebControls; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms; using WebUIControl = System.Web.UI.Control; using PropertyDescriptor = System.ComponentModel.PropertyDescriptor; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class HtmlControlDesigner : ComponentDesigner { #pragma warning disable 618 private IHtmlControlDesignerBehavior behavior = null; // the DHTML/Attached Behavior associated to this designer #pragma warning restore 618 private bool shouldCodeSerialize; ///Provides a base designer class for all server/ASP controls. ////// /// public HtmlControlDesigner() { shouldCodeSerialize = true; } ////// Initiailizes a new instance of ///. /// /// /// [Obsolete("Error: This property can no longer be referenced, and is included to support existing compiled applications. The design-time element may not always provide access to the element in the markup. There are alternate methods on WebFormsRootDesigner for handling client script and controls. http://go.microsoft.com/fwlink/?linkid=14202", true)] protected object DesignTimeElement { get { return DesignTimeElementInternal; } } internal object DesignTimeElementInternal { get { return behavior != null ? behavior.DesignTimeElement : null; } } ///The design-time object representing the control associated with this designer on the design surface. ////// /// [Obsolete("The recommended alternative is ControlDesigner.Tag. http://go.microsoft.com/fwlink/?linkid=14202")] public IHtmlControlDesignerBehavior Behavior { get { return BehaviorInternal; } set { BehaviorInternal = value; } } #pragma warning disable 618 internal virtual IHtmlControlDesignerBehavior BehaviorInternal { get { return behavior; } set { if (behavior != value) { if (behavior != null) { OnBehaviorDetaching(); // A different behavior might get attached in some cases. So, make sure to // reset the back pointer from the currently associated behavior to this designer. behavior.Designer = null; behavior = null; } if (value != null) { behavior = value; OnBehaviorAttached(); } } } } #pragma warning restore 618 ////// Points to the DHTML Behavior that is associated to this designer instance. /// ////// /// public DataBindingCollection DataBindings { get { return ((IDataBindingsAccessor)Component).DataBindings; } } ////// /// public ExpressionBindingCollection Expressions { get { return ((IExpressionsAccessor)Component).Expressions; } } ////// /// [Obsolete("Use of this property is not recommended because code serialization is not supported. http://go.microsoft.com/fwlink/?linkid=14202")] public virtual bool ShouldCodeSerialize { get { return ShouldCodeSerializeInternal; } set { ShouldCodeSerializeInternal = value; } } internal virtual bool ShouldCodeSerializeInternal { get { return shouldCodeSerialize; } set { shouldCodeSerialize = value; } } ////// /// protected override void Dispose(bool disposing) { if (disposing) { if (BehaviorInternal != null) { BehaviorInternal.Designer = null; BehaviorInternal = null; } } base.Dispose(disposing); } ////// Disposes of the resources (other than memory) used by /// the ///. /// /// /// public override void Initialize(IComponent component) { ControlDesigner.VerifyInitializeArgument(component, typeof(WebUIControl)); base.Initialize(component); } ///Initializes /// the designer and sets the component for design. ////// /// [Obsolete("The recommended alternative is ControlDesigner.Tag. http://go.microsoft.com/fwlink/?linkid=14202")] protected virtual void OnBehaviorAttached() { } ////// Notification that is called when the designer is attached to the behavior. /// ////// /// [Obsolete("The recommended alternative is ControlDesigner.Tag. http://go.microsoft.com/fwlink/?linkid=14202")] protected virtual void OnBehaviorDetaching() { } ////// Notification that is called when the designer is detached from the behavior. /// ////// /// public virtual void OnSetParent() { } ////// Notification that is called when the associated control is parented. /// ///protected override void PreFilterEvents(IDictionary events) { base.PreFilterEvents(events); if (ShouldCodeSerializeInternal == false) { // hide all the events, if this control isn't going to be serialized to code behind, ICollection eventCollection = events.Values; if ((eventCollection != null) && (eventCollection.Count != 0)) { object[] eventDescriptors = new object[eventCollection.Count]; eventCollection.CopyTo(eventDescriptors, 0); for (int i = 0; i < eventDescriptors.Length; i++) { EventDescriptor eventDesc = (EventDescriptor)eventDescriptors[i]; eventDesc = TypeDescriptor.CreateEvent(eventDesc.ComponentType, eventDesc, BrowsableAttribute.No); events[eventDesc.Name] = eventDesc; } } } } /// /// /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop = (PropertyDescriptor)properties["Modifiers"]; if (prop != null) { properties["Modifiers"] = TypeDescriptor.CreateProperty(prop.ComponentType, prop, BrowsableAttribute.No); } properties["Expressions"] = TypeDescriptor.CreateProperty(this.GetType(), "Expressions", typeof(ExpressionBindingCollection), new Attribute[] { DesignerSerializationVisibilityAttribute.Hidden, CategoryAttribute.Data, new EditorAttribute(typeof(ExpressionsCollectionEditor), typeof(UITypeEditor)), new TypeConverterAttribute(typeof(ExpressionsCollectionConverter)), new ParenthesizePropertyNameAttribute(true), MergablePropertyAttribute.No, new DescriptionAttribute(SR.GetString(SR.Control_Expressions)) }); } ////// Allows a designer to filter the set of member attributes /// that the component it is designing will expose through the ////// object. /// /// /// [Obsolete("The recommended alternative is to handle the Changed event on the DataBindings collection. The DataBindings collection allows more control of the databindings associated with the control. http://go.microsoft.com/fwlink/?linkid=14202")] protected virtual void OnBindingsCollectionChanged(string propName) { } internal void OnBindingsCollectionChangedInternal(string propName) { #pragma warning disable 618 OnBindingsCollectionChanged(propName); #pragma warning restore 618 } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Delegate to handle bindings collection changed event. /// ///
Link Menu
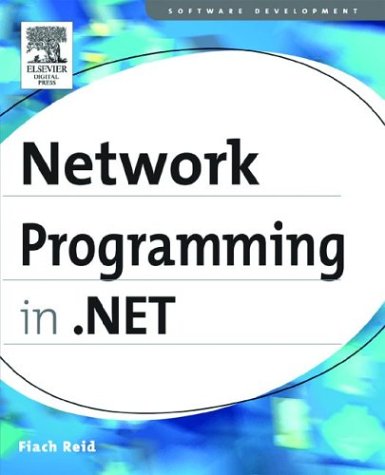
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSDualHttpBinding.cs
- CanonicalXml.cs
- PreservationFileReader.cs
- PublisherMembershipCondition.cs
- _AuthenticationState.cs
- MobileTemplatedControlDesigner.cs
- DataGridViewLayoutData.cs
- NamespaceTable.cs
- WorkflowApplicationAbortedEventArgs.cs
- QueryOutputWriterV1.cs
- infer.cs
- InvokeMethod.cs
- UserControl.cs
- HttpConfigurationSystem.cs
- ToggleButtonAutomationPeer.cs
- PrimitiveXmlSerializers.cs
- CqlLexerHelpers.cs
- WebPartZoneAutoFormat.cs
- DataPagerFieldCollection.cs
- TreeViewTemplateSelector.cs
- ComponentCommands.cs
- DeleteHelper.cs
- RequestSecurityTokenResponse.cs
- DateTimeFormatInfo.cs
- NullReferenceException.cs
- AnnouncementDispatcherAsyncResult.cs
- SqlCommandSet.cs
- CacheVirtualItemsEvent.cs
- Documentation.cs
- Control.cs
- PrintDocument.cs
- PopupRootAutomationPeer.cs
- InvalidCommandTreeException.cs
- CircleHotSpot.cs
- GridItemPattern.cs
- PopOutPanel.cs
- StringValueSerializer.cs
- Deflater.cs
- Oid.cs
- NativeConfigurationLoader.cs
- XmlDataDocument.cs
- RuleSet.cs
- WindowsSysHeader.cs
- ActivityContext.cs
- ColumnBinding.cs
- XmlQuerySequence.cs
- WebBrowserNavigatedEventHandler.cs
- EntityDescriptor.cs
- ReturnValue.cs
- Mutex.cs
- ContentPlaceHolderDesigner.cs
- ProgressChangedEventArgs.cs
- TextRunTypographyProperties.cs
- CustomCategoryAttribute.cs
- ConstructorNeedsTagAttribute.cs
- TagMapCollection.cs
- TextParagraph.cs
- BitmapFrame.cs
- VisualBrush.cs
- WrapperEqualityComparer.cs
- KnownColorTable.cs
- PropertyIDSet.cs
- SafeEventHandle.cs
- ServerIdentity.cs
- Privilege.cs
- SourceFileBuildProvider.cs
- PassportAuthenticationEventArgs.cs
- mactripleDES.cs
- GeneralTransform3DCollection.cs
- SelectionWordBreaker.cs
- ValidationError.cs
- FilteredAttributeCollection.cs
- TextHidden.cs
- ConnectionManagementSection.cs
- HtmlTableRowCollection.cs
- XmlConvert.cs
- reliableinputsessionchannel.cs
- MenuItemBindingCollection.cs
- GenericRootAutomationPeer.cs
- RedistVersionInfo.cs
- RawStylusActions.cs
- GlyphElement.cs
- SemanticResolver.cs
- QueryExpr.cs
- ActivationProxy.cs
- ScrollChangedEventArgs.cs
- MessageDecoder.cs
- DoubleAnimation.cs
- DesignerValidatorAdapter.cs
- AttributeUsageAttribute.cs
- DebugInfoGenerator.cs
- HandlerFactoryWrapper.cs
- Utils.cs
- UseLicense.cs
- OutputCacheSettings.cs
- DefaultClaimSet.cs
- DataServiceHost.cs
- ValidateNames.cs
- ClientBuildManager.cs
- NominalTypeEliminator.cs