Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / DataFieldConverter.cs / 1 / DataFieldConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Runtime.InteropServices; using System.Globalization; using System.Web.UI.Design.WebControls; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class DataFieldConverter : TypeConverter { ////// Provides design-time support for a component's data field properties. /// ////// /// public DataFieldConverter() { } ////// Initializes a new instance of ///. /// /// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. /// ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } ////// Converts the given object to the converter's native type. /// ////// private DesignerDataSourceView GetView(IDesigner dataBoundControlDesigner) { DataBoundControlDesigner dbcDesigner = dataBoundControlDesigner as DataBoundControlDesigner; if (dbcDesigner != null) { return dbcDesigner.DesignerView; } else { BaseDataListDesigner baseDataListDesigner = dataBoundControlDesigner as BaseDataListDesigner; if (baseDataListDesigner != null) { return baseDataListDesigner.DesignerView; } else { RepeaterDesigner repeaterDesigner = dataBoundControlDesigner as RepeaterDesigner; if (repeaterDesigner != null) { return repeaterDesigner.DesignerView; } } } return null; } ////// Returns the DesignerDataSourceView of the given data bound control designer. /// ////// /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { object[] names = null; if (context != null) { // // This converter shouldn't be used in a multi-select scenario. If it is, it simply // returns no standard values. IComponent component = context.Instance as IComponent; if (component != null) { ISite componentSite = component.Site; if (componentSite != null) { IDesignerHost designerHost = (IDesignerHost)componentSite.GetService(typeof(IDesignerHost)); if (designerHost != null) { IDesigner dataBoundControlDesigner = designerHost.GetDesigner(component); DesignerDataSourceView view = GetView(dataBoundControlDesigner); if (view != null) { IDataSourceViewSchema schema = null; try { schema = view.Schema; } catch (Exception ex) { IComponentDesignerDebugService debugService = (IComponentDesignerDebugService)componentSite.GetService(typeof(IComponentDesignerDebugService)); if (debugService != null) { debugService.Fail(SR.GetString(SR.DataSource_DebugService_FailedCall, "DesignerDataSourceView.Schema", ex.Message)); } } if (schema != null) { IDataSourceFieldSchema[] fieldSchemas = schema.GetFields(); if (fieldSchemas != null) { names = new object[fieldSchemas.Length]; for (int i = 0; i < fieldSchemas.Length; i++) { names[i] = fieldSchemas[i].Name; } } } } if (names == null && dataBoundControlDesigner != null && dataBoundControlDesigner is IDataSourceProvider) { IDataSourceProvider dataSourceProvider = dataBoundControlDesigner as IDataSourceProvider; IEnumerable dataSource = null; if (dataSourceProvider != null) { dataSource = dataSourceProvider.GetResolvedSelectedDataSource(); } if (dataSource != null) { PropertyDescriptorCollection props = DesignTimeData.GetDataFields(dataSource); if (props != null) { ArrayList list = new ArrayList(); foreach (PropertyDescriptor propDesc in props) { list.Add(propDesc.Name); } names = list.ToArray(); } } } } } } } return new StandardValuesCollection(names); } ////// Gets the fields present within the selected data source if information about them is available. /// ////// /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// /// /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (context != null && context.Instance is IComponent) { // We only support the dropdown in single-select mode. return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ///
Link Menu
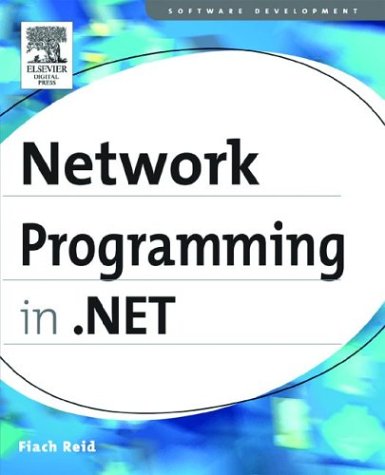
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputReportEventArgs.cs
- PropertyAccessVisitor.cs
- Native.cs
- OdbcConnectionOpen.cs
- InteropTrackingRecord.cs
- DBAsyncResult.cs
- HttpContext.cs
- ReflectTypeDescriptionProvider.cs
- ButtonColumn.cs
- PaintValueEventArgs.cs
- ConfigPathUtility.cs
- PrimitiveSchema.cs
- TargetConverter.cs
- DataTable.cs
- Group.cs
- IPAddressCollection.cs
- ParameterToken.cs
- ServiceManager.cs
- SqlClientFactory.cs
- DayRenderEvent.cs
- XmlValueConverter.cs
- DataGridViewToolTip.cs
- DbDataReader.cs
- BmpBitmapEncoder.cs
- MenuItem.cs
- InstanceKeyView.cs
- RNGCryptoServiceProvider.cs
- Delegate.cs
- PointLightBase.cs
- WindowsScroll.cs
- NativeActivityContext.cs
- VisualCollection.cs
- GridViewDeleteEventArgs.cs
- MachineKeySection.cs
- XamlPointCollectionSerializer.cs
- SchemaElementDecl.cs
- SpellerStatusTable.cs
- Transform.cs
- MultiView.cs
- Attributes.cs
- HandlerElement.cs
- SecurityAppliedMessage.cs
- Panel.cs
- Error.cs
- ApplicationId.cs
- DataGridViewRowsAddedEventArgs.cs
- EditorAttribute.cs
- ControlParameter.cs
- LassoHelper.cs
- PropertyTabChangedEvent.cs
- PrivacyNoticeBindingElement.cs
- XmlChoiceIdentifierAttribute.cs
- InheritanceContextHelper.cs
- DropShadowEffect.cs
- ExtendedPropertyCollection.cs
- IndexedGlyphRun.cs
- ImageMap.cs
- DataSvcMapFileSerializer.cs
- TreeViewDesigner.cs
- PeerConnector.cs
- SqlComparer.cs
- CompatibleComparer.cs
- InstanceLockedException.cs
- DataIdProcessor.cs
- InlineUIContainer.cs
- NonBatchDirectoryCompiler.cs
- EdmToObjectNamespaceMap.cs
- AssemblyName.cs
- FileSecurity.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- DataSourceControlBuilder.cs
- RowToFieldTransformer.cs
- EventPrivateKey.cs
- CurrentChangingEventArgs.cs
- DiagnosticTrace.cs
- MergablePropertyAttribute.cs
- Rules.cs
- WorkerRequest.cs
- UnsafeNativeMethods.cs
- HostingEnvironment.cs
- SQLUtility.cs
- Metafile.cs
- CFStream.cs
- RemoteWebConfigurationHostServer.cs
- WindowAutomationPeer.cs
- CriticalExceptions.cs
- GridViewCommandEventArgs.cs
- HelloOperation11AsyncResult.cs
- Codec.cs
- MenuItem.cs
- _ProxyChain.cs
- TimelineClockCollection.cs
- ItemChangedEventArgs.cs
- Pair.cs
- ArrayItemReference.cs
- CompoundFileReference.cs
- regiisutil.cs
- FixedPageStructure.cs
- UniqueCodeIdentifierScope.cs
- OdbcDataAdapter.cs