Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Navigation / NavigationEventArgs.cs / 1 / NavigationEventArgs.cs
//-------------------------------------------------------------------------------------------- // File: NavigationEventArgs.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // This event is fired when a navigation is completed. // This event is fired on INavigator and refired on the Application // // History: // 08/10/04: [....] Moved out of Application.cs to its own separate file. // //------------------------------------------------------------------------------------------- using System.Net; namespace System.Windows.Navigation { ////// Event args for non-cancelable navigation events - Navigated, LoadCompleted, NavigationStopped /// The NavigationEventArgs contain the uri or root element of the content being navigated to, /// and a IsNavigationInitiator property that indicates whether this is a new navigation initiated /// by this navigator, or whether this navigation is being propagated down from a higher level navigation /// taking place in a containing window or frame. /// The developer should check the IsNavigationInitiator property on the NavigationEventArgs to /// determine whether to spin the globe. /// public class NavigationEventArgs : EventArgs { // Internal constructor // URI of the content navigated to. // Root of the element tree being navigated to. // Indicates whether this navigator is // initiating the navigation or whether a parent internal NavigationEventArgs(Uri uri, Object content, Object extraData, WebResponse response, object Navigator, bool isNavigationInitiator) { _uri = uri; _content = content; _extraData = extraData; _webResponse = response; _isNavigationInitiator = isNavigationInitiator; _navigator = Navigator; } ////// URI of the markup page navigated to. /// public Uri Uri { get { return _uri; } } ////// Root of the element tree navigated to. /// Note: Only one of the Content or Uri property will be set, depending on whether the /// navigation was to a Uri or an existing element tree. /// public Object Content { get { return _content; } } ////// Indicates whether this navigator is initiating the navigation or whether a parent /// navigator is being navigated (e.g., the current navigator is a frame /// inside a page thats being navigated to inside a parent navigator). A developer /// can use this property to determine whether to spin the globe on a Navigating event or /// to stop spinning the globe on a LoadCompleted event. /// If this property is False, the navigators parent navigator is also navigating and /// the globe is already spinning. /// If this property is True, the navigation was initiated inside the current frame and /// the developer should spin the globe (or stop spinning the globe, depending on /// which event is being handled.) /// public bool IsNavigationInitiator { get { return _isNavigationInitiator; } } ////// Exposes extra data object which was optionally passed as a parameter to Navigate. /// public Object ExtraData { get { return _extraData; } } ////// Exposes the web response to allow access to HTTP headers and other properties. /// public WebResponse WebResponse { get { return _webResponse; } } ////// The navigator that raised this event /// public object Navigator { get { return _navigator; } } private Uri _uri; private Object _content; private Object _extraData; private WebResponse _webResponse; private bool _isNavigationInitiator; object _navigator; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
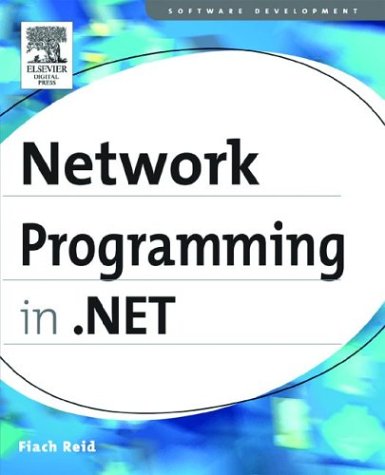
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaInclude.cs
- SocketAddress.cs
- ObjectTypeMapping.cs
- COAUTHINFO.cs
- Size3D.cs
- DecimalKeyFrameCollection.cs
- PerfService.cs
- UnsafeNativeMethodsPenimc.cs
- FileDetails.cs
- AttachmentService.cs
- System.Data.OracleClient_BID.cs
- WindowsStreamSecurityUpgradeProvider.cs
- TextTreeTextElementNode.cs
- securitymgrsite.cs
- WebPartDeleteVerb.cs
- DefaultBindingPropertyAttribute.cs
- ParenthesizePropertyNameAttribute.cs
- PropertyOrder.cs
- FileIOPermission.cs
- InheritanceContextChangedEventManager.cs
- StylusButtonCollection.cs
- PassportAuthenticationEventArgs.cs
- PageContentCollection.cs
- UIElement3D.cs
- SamlDelegatingWriter.cs
- SerializationObjectManager.cs
- SqlGenericUtil.cs
- TraceSource.cs
- ValueTypePropertyReference.cs
- NullReferenceException.cs
- PrimitiveType.cs
- EditBehavior.cs
- ModelUtilities.cs
- SendMessageRecord.cs
- LogArchiveSnapshot.cs
- ParagraphResult.cs
- DBPropSet.cs
- SelectedPathEditor.cs
- MouseGestureValueSerializer.cs
- GridPattern.cs
- InheritanceContextChangedEventManager.cs
- VisualProxy.cs
- DesignRelationCollection.cs
- MailDefinitionBodyFileNameEditor.cs
- UpdateCommand.cs
- DoubleKeyFrameCollection.cs
- ExternalCalls.cs
- RoleManagerModule.cs
- cookieexception.cs
- ProxyWebPartManager.cs
- PathFigureCollectionConverter.cs
- SBCSCodePageEncoding.cs
- ShortcutKeysEditor.cs
- UpdateCompiler.cs
- DataGridViewMethods.cs
- WinEventTracker.cs
- XmlBinaryReader.cs
- ProvidersHelper.cs
- CacheChildrenQuery.cs
- ClientSettingsStore.cs
- FixedSOMImage.cs
- BinHexEncoder.cs
- OracleConnectionStringBuilder.cs
- FrameworkElement.cs
- DataServiceStreamResponse.cs
- HostedNamedPipeTransportManager.cs
- DocumentOrderQuery.cs
- SqlPersonalizationProvider.cs
- SqlReferenceCollection.cs
- DescendantBaseQuery.cs
- UIElement.cs
- CrossSiteScriptingValidation.cs
- Guid.cs
- HtmlUtf8RawTextWriter.cs
- SR.cs
- HtmlTableCell.cs
- BookmarkUndoUnit.cs
- TypeUtils.cs
- CharUnicodeInfo.cs
- CookieHandler.cs
- Semaphore.cs
- CheckBoxBaseAdapter.cs
- MasterPageBuildProvider.cs
- OdbcConnection.cs
- EdmMember.cs
- MetadataArtifactLoaderFile.cs
- PauseStoryboard.cs
- CursorInteropHelper.cs
- SqlTransaction.cs
- Geometry3D.cs
- DataListItem.cs
- X509Utils.cs
- XmlAttributes.cs
- SqlClientWrapperSmiStream.cs
- PopupRoot.cs
- TextFormatterHost.cs
- PageWrapper.cs
- RepeatButton.cs
- XmlResolver.cs
- Scanner.cs