Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / Localizer / BamlLocalizableResource.cs / 1 / BamlLocalizableResource.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizableResource.cs // // Contents: BamlLocalizableResource class, part of Baml Localization API // // Created: 3/4/2004 [....] // History: 8/3/2004 [....] Move to System.Windows namespace // 11/29/2004 [....] Move to System.Windows.Markup.Localization namespace // 03/24/2005 [....] Move to System.Windows.Markup.Localizer namespace // //----------------------------------------------------------------------- using System; using System.Windows; using MS.Internal; using System.Diagnostics; namespace System.Windows.Markup.Localizer { ////// Localization resource in Baml /// public class BamlLocalizableResource { //-------------------------------- // constructor //-------------------------------- ////// Constructor of LocalizableResource /// public BamlLocalizableResource() : this ( null, null, LocalizationCategory.None, true, true ) { } ////// Constructor of LocalizableResource /// public BamlLocalizableResource( string content, string comments, LocalizationCategory category, bool modifiable, bool readable ) { _content = content; _comments = comments; _category = category; Modifiable = modifiable; Readable = readable; } ////// constructor that creates a deep copy of the other localizable resource /// /// the other localizale resource internal BamlLocalizableResource(BamlLocalizableResource other) { Debug.Assert(other != null); _content = other._content; _comments = other._comments; _flags = other._flags; _category = other._category; } //--------------------------------- // public properties //--------------------------------- ////// The localizable value /// public string Content { get { return _content; } set { _content = value; } } ////// The localization comments /// public string Comments { get { return _comments; } set { _comments = value; } } ////// Localization Lock by developer /// public bool Modifiable { get { return (_flags & LocalizationFlags.Modifiable) > 0; } set { if (value) { _flags |= LocalizationFlags.Modifiable; } else { _flags &= (~LocalizationFlags.Modifiable); } } } ////// Visibility of the resource for translation /// public bool Readable { get { return (_flags & LocalizationFlags.Readable) > 0; } set { if (value) { _flags |= LocalizationFlags.Readable; } else { _flags &= (~LocalizationFlags.Readable); } } } ////// String category of the resource /// public LocalizationCategory Category { get { return _category; } set { _category = value; } } ////// compare equality /// public override bool Equals(object other) { BamlLocalizableResource otherResource = other as BamlLocalizableResource; if (otherResource == null) return false; return (_content == otherResource._content && _comments == otherResource._comments && _flags == otherResource._flags && _category == otherResource._category); } //////Return the hashcode. /// public override int GetHashCode() { return (_content == null ? 0 : _content.GetHashCode()) ^(_comments == null ? 0 : _comments.GetHashCode()) ^ (int) _flags ^ (int) _category; } //--------------------------------- // private members //--------------------------------- private string _content; private string _comments; private LocalizationFlags _flags; private LocalizationCategory _category; //--------------------------------- // Private type //--------------------------------- [Flags] private enum LocalizationFlags : byte { Readable = 1, Modifiable = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
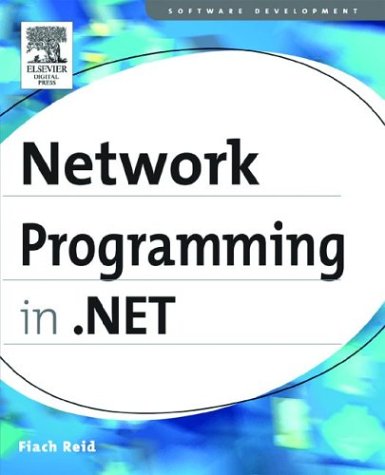
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MonitorWrapper.cs
- OneToOneMappingSerializer.cs
- CalendarTable.cs
- RoutedEventConverter.cs
- CfgArc.cs
- CleanUpVirtualizedItemEventArgs.cs
- FixedPageStructure.cs
- ParameterModifier.cs
- Selector.cs
- ValidatedMobileControlConverter.cs
- Camera.cs
- KeyToListMap.cs
- ClientScriptManager.cs
- DataGrid.cs
- ContentPlaceHolderDesigner.cs
- TemplateControl.cs
- InvalidProgramException.cs
- CodeIndexerExpression.cs
- DirectoryInfo.cs
- SafeThreadHandle.cs
- DataGridAddNewRow.cs
- LabelLiteral.cs
- activationcontext.cs
- HttpCookiesSection.cs
- VirtualDirectoryMappingCollection.cs
- WebPartDescriptionCollection.cs
- CngProvider.cs
- ReflectionTypeLoadException.cs
- EntityContainerAssociationSet.cs
- DataStorage.cs
- ObjRef.cs
- MimeMultiPart.cs
- ToolStripRenderer.cs
- RelatedEnd.cs
- RequiredFieldValidator.cs
- EntityDataSourceEntitySetNameItem.cs
- BuildProvidersCompiler.cs
- XmlSortKey.cs
- keycontainerpermission.cs
- PageParser.cs
- RoleGroup.cs
- GlyphRunDrawing.cs
- EntityProxyTypeInfo.cs
- StaticTextPointer.cs
- VectorAnimationBase.cs
- SplitterPanelDesigner.cs
- WebPartCatalogCloseVerb.cs
- CreateRefExpr.cs
- ImageBrush.cs
- XmlMembersMapping.cs
- CommunicationException.cs
- Exceptions.cs
- HtmlMeta.cs
- PingOptions.cs
- StructuredType.cs
- NativeActivityFaultContext.cs
- OptimisticConcurrencyException.cs
- NameValueCollection.cs
- CustomCredentialPolicy.cs
- XsdSchemaFileEditor.cs
- SafeNativeMethods.cs
- PackageStore.cs
- BroadcastEventHelper.cs
- DrawingImage.cs
- FontResourceCache.cs
- SqlUnionizer.cs
- HttpDictionary.cs
- ConnectionsZone.cs
- FrugalMap.cs
- TextServicesLoader.cs
- DataBindEngine.cs
- WsrmTraceRecord.cs
- TypeUtil.cs
- ScriptControl.cs
- MarshalByValueComponent.cs
- BamlBinaryReader.cs
- SuppressIldasmAttribute.cs
- GregorianCalendarHelper.cs
- TraceContext.cs
- CroppedBitmap.cs
- JsonQNameDataContract.cs
- XsdBuildProvider.cs
- ManagementEventWatcher.cs
- AppSecurityManager.cs
- DesigntimeLicenseContextSerializer.cs
- RectAnimationBase.cs
- FeatureSupport.cs
- InvalidOperationException.cs
- CodeCommentStatementCollection.cs
- TemplateEditingFrame.cs
- ContextStack.cs
- ParserStack.cs
- ReaderWriterLock.cs
- HitTestWithPointDrawingContextWalker.cs
- PropertyInformationCollection.cs
- DefaultTextStoreTextComposition.cs
- IdleTimeoutMonitor.cs
- HtmlInputText.cs
- NeutralResourcesLanguageAttribute.cs
- ExecutionEngineException.cs