Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Controls / webbrowsersite.cs / 1 / webbrowsersite.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // WebBrowserSite is a sub-class of ActiveXSite. // Used to implement IDocHostUIHandler. // // Copied from WebBrowser.cs in winforms // // History // 06/16/05 - [....] - Created // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using MS.Win32; using System.Security ; using MS.Internal.PresentationFramework; using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; namespace MS.Internal.Controls { // // WebBrowserSite class: // /// ////// /// Provides a default WebBrowserSite implementation for use in the CreateWebBrowserSite /// method in the WebBrowser class. /// /// internal class WebBrowserSite : ActiveXSite , UnsafeNativeMethods.IDocHostUIHandler { /// ////// WebBrowser implementation of ActiveXSite. Used to override GetHostInfo. /// and "turn on" our redirect notifications. /// ////// Critical - calls base class ctor which is critical. /// [ SecurityCritical ] internal WebBrowserSite(WebBrowser host) : base(host) { } // // IDocHostUIHandler Implementation // /// int UnsafeNativeMethods.IDocHostUIHandler.ShowContextMenu(int dwID, NativeMethods.POINT pt, object pcmdtReserved, object pdispReserved) { // // Returning S_FALSE will allow the native control to do default processing, // i.e., execute the shortcut key. Returning S_OK will cancel the context menu // return NativeMethods.S_FALSE; } ////// Critical - calls critical code. /// If you change this method - you could affect mitigations. /// **Needs to be critical.** /// TreatAsSafe - information returned from this method is innocous. /// lists the set of browser features/options we've enabled. /// [ SecurityCritical, SecurityTreatAsSafe ] int UnsafeNativeMethods.IDocHostUIHandler.GetHostInfo(NativeMethods.DOCHOSTUIINFO info) { WebBrowser wb = (WebBrowser)this.Host; info.dwDoubleClick = (int) NativeMethods.DOCHOSTUIDBLCLICK.DEFAULT; // // These are the current flags shdocvw uses. Assumed we want the same. // info.dwFlags = (int) ( NativeMethods.DOCHOSTUIFLAG.DISABLE_HELP_MENU | NativeMethods.DOCHOSTUIFLAG.DISABLE_SCRIPT_INACTIVE | NativeMethods.DOCHOSTUIFLAG.ENABLE_INPLACE_NAVIGATION | NativeMethods.DOCHOSTUIFLAG.IME_ENABLE_RECONVERSION | NativeMethods.DOCHOSTUIFLAG.THEME | NativeMethods.DOCHOSTUIFLAG.ENABLE_FORMS_AUTOCOMPLETE | NativeMethods.DOCHOSTUIFLAG.DISABLE_UNTRUSTEDPROTOCOL | NativeMethods.DOCHOSTUIFLAG.LOCAL_MACHINE_ACCESS_CHECK | NativeMethods.DOCHOSTUIFLAG.ENABLE_REDIRECT_NOTIFICATION ); return NativeMethods.S_OK; } int UnsafeNativeMethods.IDocHostUIHandler.EnableModeless(bool fEnable) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.ShowUI(int dwID, UnsafeNativeMethods.IOleInPlaceActiveObject activeObject, NativeMethods.IOleCommandTarget commandTarget, UnsafeNativeMethods.IOleInPlaceFrame frame, UnsafeNativeMethods.IOleInPlaceUIWindow doc) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.HideUI() { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.UpdateUI() { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.OnDocWindowActivate(bool fActivate) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.OnFrameWindowActivate(bool fActivate) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.ResizeBorder(NativeMethods.COMRECT rect, UnsafeNativeMethods.IOleInPlaceUIWindow doc, bool fFrameWindow) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.GetOptionKeyPath(string[] pbstrKey, int dw) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.GetDropTarget(UnsafeNativeMethods.IOleDropTarget pDropTarget, out UnsafeNativeMethods.IOleDropTarget ppDropTarget) { // // Set to null no matter what we return, to prevent the marshaller // from going crazy if the pointer points to random stuff. ppDropTarget = null; return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.GetExternal(out object ppDispatch) { /* WebBrowser wb = (WebBrowser)this.Host; ppDispatch = wb.ObjectForScripting; return NativeMethods.S_OK; */ ppDispatch = null; return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.TranslateAccelerator(ref System.Windows.Interop.MSG msg, ref Guid group, int nCmdID) { // // Returning S_FALSE will allow the native control to do default processing, // i.e., execute the shortcut key. Returning S_OK will cancel the shortcut key. /* WebBrowser wb = (WebBrowser)this.Host; if (!wb.WebBrowserShortcutsEnabled) { int keyCode = (int)msg.wParam | (int)Control.ModifierKeys; if (msg.message != NativeMethods.WM_CHAR && Enum.IsDefined(typeof(Shortcut), (Shortcut)keyCode)) { return NativeMethods.S_OK; } return NativeMethods.S_FALSE; } */ return NativeMethods.S_FALSE; } int UnsafeNativeMethods.IDocHostUIHandler.TranslateUrl(int dwTranslate, string strUrlIn, out string pstrUrlOut) { // // Set to null no matter what we return, to prevent the marshaller // from going crazy if the pointer points to random stuff. pstrUrlOut = null; return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IDocHostUIHandler.FilterDataObject(IComDataObject pDO, out IComDataObject ppDORet) { // // Set to null no matter what we return, to prevent the marshaller // from going crazy if the pointer points to random stuff. ppDORet = null; return NativeMethods.E_NOTIMPL; } // // Internal methods // /* internal override void OnPropertyChanged(int dispid) { if (dispid != NativeMethods.ActiveX.DISPID_READYSTATE) { base.OnPropertyChanged(dispid); } } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
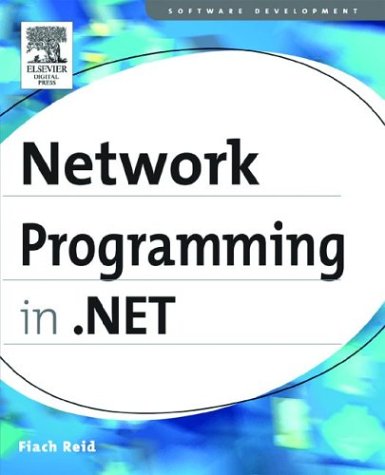
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlPointCollectionSerializer.cs
- DataSet.cs
- CalendarKeyboardHelper.cs
- SmtpAuthenticationManager.cs
- X509Certificate2.cs
- HtmlInputControl.cs
- ToggleButton.cs
- CrossSiteScriptingValidation.cs
- AsyncDataRequest.cs
- FixedBufferAttribute.cs
- DataListItemCollection.cs
- PropertyBuilder.cs
- TemplateLookupAction.cs
- SerializationSectionGroup.cs
- InteropAutomationProvider.cs
- ResourceExpressionEditor.cs
- ProtocolsConfiguration.cs
- DiagnosticsConfiguration.cs
- CodeSnippetCompileUnit.cs
- documentsequencetextview.cs
- DbModificationCommandTree.cs
- DrawingGroup.cs
- DNS.cs
- WebPartConnectionsCloseVerb.cs
- WebControlAdapter.cs
- LinqDataSourceEditData.cs
- EntityCommandDefinition.cs
- URI.cs
- SqlConnectionString.cs
- SecurityContext.cs
- StructuralComparisons.cs
- EntitySetRetriever.cs
- StaticTextPointer.cs
- PersonalizationProvider.cs
- FormatterConverter.cs
- PageWrapper.cs
- UnSafeCharBuffer.cs
- Int64AnimationUsingKeyFrames.cs
- Validator.cs
- BinaryConverter.cs
- InheritanceContextChangedEventManager.cs
- XamlBrushSerializer.cs
- Item.cs
- SimpleWorkerRequest.cs
- CounterSampleCalculator.cs
- TransformPattern.cs
- Property.cs
- UnmanagedMarshal.cs
- DeviceContext2.cs
- DateTimeConverter.cs
- COMException.cs
- cookiecollection.cs
- DataServiceHostFactory.cs
- BufferedGraphicsContext.cs
- QueueProcessor.cs
- ServiceModelSectionGroup.cs
- mediaeventshelper.cs
- Page.cs
- FileEnumerator.cs
- AndAlso.cs
- WebHttpDispatchOperationSelectorData.cs
- CodeIndexerExpression.cs
- GradientPanel.cs
- _LoggingObject.cs
- TraceLog.cs
- SafeEventLogReadHandle.cs
- RuleSettingsCollection.cs
- SymbolEqualComparer.cs
- Classification.cs
- ExpressionContext.cs
- HwndTarget.cs
- StaticExtension.cs
- RNGCryptoServiceProvider.cs
- PolicyUnit.cs
- HtmlContainerControl.cs
- ComboBox.cs
- xmlNames.cs
- CellIdBoolean.cs
- ColorTranslator.cs
- SoapAttributeAttribute.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- RijndaelManaged.cs
- SQLBinaryStorage.cs
- listitem.cs
- SafeMILHandle.cs
- WebDescriptionAttribute.cs
- PageAdapter.cs
- FragmentQuery.cs
- RightsManagementEncryptionTransform.cs
- GridViewHeaderRowPresenter.cs
- DataStreamFromComStream.cs
- TriState.cs
- AppDomainProtocolHandler.cs
- SettingsPropertyNotFoundException.cs
- ToolBar.cs
- coordinator.cs
- TableFieldsEditor.cs
- ByteStreamGeometryContext.cs
- SmtpNegotiateAuthenticationModule.cs
- Transform3D.cs