Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / MouseButtonEventArgs.cs / 1 / MouseButtonEventArgs.cs
using System; namespace System.Windows.Input { ////// The MouseButtonEventArgs describes the state of a Mouse button. /// public class MouseButtonEventArgs : MouseEventArgs { ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The mouse button whose state is being described. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button) : base(mouse, timestamp) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The Mouse button whose state is being described. /// /// /// The stylus device that was involved with this event. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button, StylusDevice stylusDevice) : base(mouse, timestamp, stylusDevice) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Read-only access to the button being described. /// public MouseButton ChangedButton { get {return _button;} } ////// Read-only access to the button state. /// public MouseButtonState ButtonState { get { MouseButtonState state = MouseButtonState.Released; switch(_button) { case MouseButton.Left: state = this.MouseDevice.LeftButton; break; case MouseButton.Right: state = this.MouseDevice.RightButton; break; case MouseButton.Middle: state = this.MouseDevice.MiddleButton; break; case MouseButton.XButton1: state = this.MouseDevice.XButton1; break; case MouseButton.XButton2: state = this.MouseDevice.XButton2; break; } return state; } } ////// Read access to the button click count. /// public int ClickCount { get {return _count;} internal set { _count = value;} } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseButtonEventHandler handler = (MouseButtonEventHandler) genericHandler; handler(genericTarget, this); } private MouseButton _button; private int _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
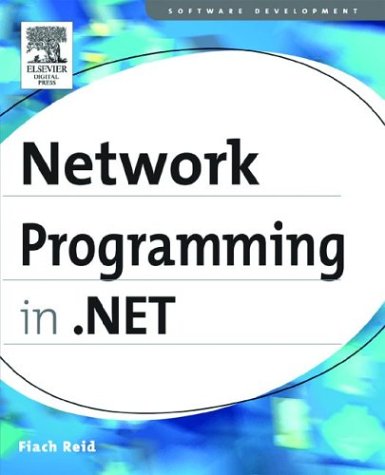
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutPanelCellPosition.cs
- CodeEntryPointMethod.cs
- MultiAsyncResult.cs
- OleDbConnection.cs
- C14NUtil.cs
- ExpressionWriter.cs
- CollectionViewGroupInternal.cs
- AsyncCodeActivity.cs
- OpenFileDialog.cs
- FrameworkContentElementAutomationPeer.cs
- TypeUnloadedException.cs
- ClientEndpointLoader.cs
- SqlBulkCopy.cs
- LZCodec.cs
- Thread.cs
- ObjectStateEntry.cs
- ContractsBCL.cs
- Part.cs
- CompositeKey.cs
- ThreadStateException.cs
- UpdatePanelTrigger.cs
- Transform.cs
- BufferedGraphics.cs
- MimeBasePart.cs
- ListViewEditEventArgs.cs
- IndexedGlyphRun.cs
- DefaultMemberAttribute.cs
- TypeElementCollection.cs
- LabelAutomationPeer.cs
- PageAsyncTask.cs
- WebPartsPersonalizationAuthorization.cs
- SqlBulkCopyColumnMapping.cs
- NameValueFileSectionHandler.cs
- SafeHandle.cs
- ResourcePart.cs
- SqlWriter.cs
- Sql8ConformanceChecker.cs
- ListViewUpdateEventArgs.cs
- CalendarBlackoutDatesCollection.cs
- XmlReaderSettings.cs
- FlowchartDesigner.xaml.cs
- ResourceDescriptionAttribute.cs
- BrowsableAttribute.cs
- SwitchAttribute.cs
- TraceUtility.cs
- ButtonBaseAdapter.cs
- SequenceFullException.cs
- GeneralTransform.cs
- WindowHideOrCloseTracker.cs
- SafeArrayRankMismatchException.cs
- XhtmlConformanceSection.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ExpressionLexer.cs
- LockedHandleGlyph.cs
- SecuritySessionServerSettings.cs
- DataGridViewRow.cs
- documentation.cs
- GridViewSortEventArgs.cs
- PeerApplicationLaunchInfo.cs
- TreeNode.cs
- SpeakInfo.cs
- DataServiceQuery.cs
- PeerResolverElement.cs
- RegistrationServices.cs
- SchemaElement.cs
- DbConnectionFactory.cs
- GlyphCollection.cs
- XslAst.cs
- IxmlLineInfo.cs
- ChineseLunisolarCalendar.cs
- WebPartTransformerCollection.cs
- SqlDataReaderSmi.cs
- WebColorConverter.cs
- SafeArrayTypeMismatchException.cs
- AnnotationResourceCollection.cs
- FusionWrap.cs
- SerialStream.cs
- PropertyRef.cs
- CodeTypeMember.cs
- WeakReference.cs
- AuthenticateEventArgs.cs
- FixUp.cs
- Rule.cs
- RegexRunnerFactory.cs
- Update.cs
- DataGridItemAttachedStorage.cs
- PeerNameRecord.cs
- SchemaHelper.cs
- FlowDocumentPaginator.cs
- RequestCacheEntry.cs
- EncoderParameters.cs
- MsmqInputChannel.cs
- Calendar.cs
- WSHttpBinding.cs
- WindowsIPAddress.cs
- PartitionResolver.cs
- SQLInt16Storage.cs
- regiisutil.cs
- WebPartDeleteVerb.cs
- PromptEventArgs.cs