Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Control.cs / 1305600 / Control.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Controls.Primitives; using System.Windows.Media; using System.Windows; using System.Windows.Input; using System.Windows.Documents; using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.PresentationFramework; using MS.Utility; namespace System.Windows.Controls { ////// The base class for all controls. /// public class Control : FrameworkElement { #region Constructors static Control() { FocusableProperty.OverrideMetadata(typeof(Control), new FrameworkPropertyMetadata(BooleanBoxes.TrueBox)); EventManager.RegisterClassHandler(typeof(Control), UIElement.PreviewMouseLeftButtonDownEvent, new MouseButtonEventHandler(HandleDoubleClick), true); EventManager.RegisterClassHandler(typeof(Control), UIElement.MouseLeftButtonDownEvent, new MouseButtonEventHandler(HandleDoubleClick), true); EventManager.RegisterClassHandler(typeof(Control), UIElement.PreviewMouseRightButtonDownEvent, new MouseButtonEventHandler(HandleDoubleClick), true); EventManager.RegisterClassHandler(typeof(Control), UIElement.MouseRightButtonDownEvent, new MouseButtonEventHandler(HandleDoubleClick), true); // change handlers to update validation visual state IsKeyboardFocusedPropertyKey.OverrideMetadata(typeof(Control), new PropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } ////// Default Control constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Control() : base() { // Initialize the _templateCache to the default value for TemplateProperty. // If the default value is non-null then wire it to the current instance. PropertyMetadata metadata = TemplateProperty.GetMetadata(DependencyObjectType); ControlTemplate defaultValue = (ControlTemplate) metadata.DefaultValue; if (defaultValue != null) { OnTemplateChanged(this, new DependencyPropertyChangedEventArgs(TemplateProperty, metadata, null, defaultValue)); } } #endregion #region Properties ////// The DependencyProperty for the BorderBrush property. /// [CommonDependencyProperty] public static readonly DependencyProperty BorderBrushProperty = Border.BorderBrushProperty.AddOwner(typeof(Control), new FrameworkPropertyMetadata( Border.BorderBrushProperty.DefaultMetadata.DefaultValue, FrameworkPropertyMetadataOptions.None)); ////// An object that describes the border background. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public Brush BorderBrush { get { return (Brush) GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// The DependencyProperty for the BorderThickness property. /// [CommonDependencyProperty] public static readonly DependencyProperty BorderThicknessProperty = Border.BorderThicknessProperty.AddOwner(typeof(Control), new FrameworkPropertyMetadata( Border.BorderThicknessProperty.DefaultMetadata.DefaultValue, FrameworkPropertyMetadataOptions.None)); ////// An object that describes the border thickness. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public Thickness BorderThickness { get { return (Thickness) GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// The DependencyProperty for the Background property. /// [CommonDependencyProperty] public static readonly DependencyProperty BackgroundProperty = Panel.BackgroundProperty.AddOwner(typeof(Control), new FrameworkPropertyMetadata( Panel.BackgroundProperty.DefaultMetadata.DefaultValue, FrameworkPropertyMetadataOptions.None)); ////// An object that describes the background. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public Brush Background { get { return (Brush) GetValue(BackgroundProperty); } set { SetValue(BackgroundProperty, value); } } ////// The DependencyProperty for the Foreground property. /// Flags: Can be used in style rules /// Default Value: System Font Color /// [CommonDependencyProperty] public static readonly DependencyProperty ForegroundProperty = TextElement.ForegroundProperty.AddOwner( typeof(Control), new FrameworkPropertyMetadata(SystemColors.ControlTextBrush, FrameworkPropertyMetadataOptions.Inherits)); ////// An brush that describes the foreground color. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public Brush Foreground { get { return (Brush) GetValue(ForegroundProperty); } set { SetValue(ForegroundProperty, value); } } ////// The DependencyProperty for the FontFamily property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font /// [CommonDependencyProperty] public static readonly DependencyProperty FontFamilyProperty = TextElement.FontFamilyProperty.AddOwner( typeof(Control), new FrameworkPropertyMetadata(SystemFonts.MessageFontFamily, FrameworkPropertyMetadataOptions.Inherits)); ////// The font family of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] [Localizability(LocalizationCategory.Font)] public FontFamily FontFamily { get { return (FontFamily) GetValue(FontFamilyProperty); } set { SetValue(FontFamilyProperty, value); } } ////// The DependencyProperty for the FontSize property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Size /// [CommonDependencyProperty] public static readonly DependencyProperty FontSizeProperty = TextElement.FontSizeProperty.AddOwner( typeof(Control), new FrameworkPropertyMetadata(SystemFonts.MessageFontSize, FrameworkPropertyMetadataOptions.Inherits)); ////// The size of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [TypeConverter(typeof(FontSizeConverter))] [Bindable(true), Category("Appearance")] [Localizability(LocalizationCategory.None)] public double FontSize { get { return (double) GetValue(FontSizeProperty); } set { SetValue(FontSizeProperty, value); } } ////// The DependencyProperty for the FontStretch property. /// Flags: Can be used in style rules /// Default Value: FontStretches.Normal /// [CommonDependencyProperty] public static readonly DependencyProperty FontStretchProperty = TextElement.FontStretchProperty.AddOwner(typeof(Control), new FrameworkPropertyMetadata(TextElement.FontStretchProperty.DefaultMetadata.DefaultValue, FrameworkPropertyMetadataOptions.Inherits)); ////// The stretch of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public FontStretch FontStretch { get { return (FontStretch) GetValue(FontStretchProperty); } set { SetValue(FontStretchProperty, value); } } ////// The DependencyProperty for the FontStyle property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Style /// [CommonDependencyProperty] public static readonly DependencyProperty FontStyleProperty = TextElement.FontStyleProperty.AddOwner( typeof(Control), new FrameworkPropertyMetadata(SystemFonts.MessageFontStyle, FrameworkPropertyMetadataOptions.Inherits)); ////// The style of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public FontStyle FontStyle { get { return (FontStyle) GetValue(FontStyleProperty); } set { SetValue(FontStyleProperty, value); } } ////// The DependencyProperty for the FontWeight property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Weight /// [CommonDependencyProperty] public static readonly DependencyProperty FontWeightProperty = TextElement.FontWeightProperty.AddOwner( typeof(Control), new FrameworkPropertyMetadata(SystemFonts.MessageFontWeight, FrameworkPropertyMetadataOptions.Inherits)); ////// The weight or thickness of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Appearance")] public FontWeight FontWeight { get { return (FontWeight) GetValue(FontWeightProperty); } set { SetValue(FontWeightProperty, value); } } ////// HorizontalContentAlignment Dependency Property. /// Flags: Can be used in style rules /// Default Value: HorizontalAlignment.Left /// [CommonDependencyProperty] public static readonly DependencyProperty HorizontalContentAlignmentProperty = DependencyProperty.Register( "HorizontalContentAlignment", typeof(HorizontalAlignment), typeof(Control), new FrameworkPropertyMetadata(HorizontalAlignment.Left), new ValidateValueCallback(FrameworkElement.ValidateHorizontalAlignmentValue)); ////// The horizontal alignment of the control. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Layout")] public HorizontalAlignment HorizontalContentAlignment { get { return (HorizontalAlignment) GetValue(HorizontalContentAlignmentProperty); } set { SetValue(HorizontalContentAlignmentProperty, value); } } ////// VerticalContentAlignment Dependency Property. /// Flags: Can be used in style rules /// Default Value: VerticalAlignment.Top /// [CommonDependencyProperty] public static readonly DependencyProperty VerticalContentAlignmentProperty = DependencyProperty.Register( "VerticalContentAlignment", typeof(VerticalAlignment), typeof(Control), new FrameworkPropertyMetadata(VerticalAlignment.Top), new ValidateValueCallback(FrameworkElement.ValidateVerticalAlignmentValue)); ////// The vertical alignment of the control. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [Bindable(true), Category("Layout")] public VerticalAlignment VerticalContentAlignment { get { return (VerticalAlignment) GetValue(VerticalContentAlignmentProperty); } set { SetValue(VerticalContentAlignmentProperty, value); } } ////// The DependencyProperty for the TabIndex property. /// [CommonDependencyProperty] public static readonly DependencyProperty TabIndexProperty = KeyboardNavigation.TabIndexProperty.AddOwner(typeof(Control)); ////// TabIndex property change the order of Tab navigation between Controls. /// Control with lower TabIndex will get focus before the Control with higher index /// [Bindable(true), Category("Behavior")] public int TabIndex { get { return (int) GetValue(TabIndexProperty); } set { SetValue(TabIndexProperty, value); } } ////// The DependencyProperty for the IsTabStop property. /// [CommonDependencyProperty] public static readonly DependencyProperty IsTabStopProperty = KeyboardNavigation.IsTabStopProperty.AddOwner(typeof(Control)); ////// Determine is the Control should be considered during Tab navigation. /// If IsTabStop is false then it is excluded from Tab navigation /// [Bindable(true), Category("Behavior")] public bool IsTabStop { get { return (bool) GetValue(IsTabStopProperty); } set { SetValue(IsTabStopProperty, BooleanBoxes.Box(value)); } } ////// PaddingProperty /// [CommonDependencyProperty] public static readonly DependencyProperty PaddingProperty = DependencyProperty.Register( "Padding", typeof(Thickness), typeof(Control), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsParentMeasure)); private static bool IsMarginValid(object value) { Thickness t = (Thickness)value; return (t.Left >= 0.0d && t.Right >= 0.0d && t.Top >= 0.0d && t.Bottom >= 0.0d); } ////// Padding Property /// [Bindable(true), Category("Layout")] public Thickness Padding { get { return (Thickness) GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// TemplateProperty /// [CommonDependencyProperty] public static readonly DependencyProperty TemplateProperty = DependencyProperty.Register( "Template", typeof(ControlTemplate), typeof(Control), new FrameworkPropertyMetadata( (ControlTemplate) null, // default value FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnTemplateChanged))); ////// Template Property /// public ControlTemplate Template { get { return _templateCache; } set { SetValue(TemplateProperty, value); } } // Internal Helper so the FrameworkElement could see this property internal override FrameworkTemplate TemplateInternal { get { return Template; } } // Internal Helper so the FrameworkElement could see the template cache internal override FrameworkTemplate TemplateCache { get { return _templateCache; } set { _templateCache = (ControlTemplate) value; } } // Internal helper so FrameworkElement could see call the template changed virtual internal override void OnTemplateChangedInternal(FrameworkTemplate oldTemplate, FrameworkTemplate newTemplate) { OnTemplateChanged((ControlTemplate)oldTemplate, (ControlTemplate)newTemplate); } // Property invalidation callback invoked when TemplateProperty is invalidated private static void OnTemplateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Control c = (Control) d; StyleHelper.UpdateTemplateCache(c, (FrameworkTemplate) e.OldValue, (FrameworkTemplate) e.NewValue, TemplateProperty); } ////// Template has changed /// ////// When a Template changes, the VisualTree is removed. The new Template's /// VisualTree will be created when ApplyTemplate is called /// /// The old Template /// The new Template protected virtual void OnTemplateChanged(ControlTemplate oldTemplate, ControlTemplate newTemplate) { } ////// If control has a scrollviewer in its style and has a custom keyboard scrolling behavior when HandlesScrolling should return true. /// Then ScrollViewer will not handle keyboard input and leave it up to the control. /// protected internal virtual bool HandlesScrolling { get { return false; } } internal bool VisualStateChangeSuspended { get { return ReadControlFlag(ControlBoolFlags.VisualStateChangeSuspended); } set { WriteControlFlag(ControlBoolFlags.VisualStateChangeSuspended, value); } } #endregion #region Public Methods ////// Returns a string representation of this object. /// ///public override string ToString() { string plainText = null; // GetPlainText overrides may try to access thread critical data if (CheckAccess()) { plainText = GetPlainText(); } else { //Not on dispatcher, try posting to the dispatcher with 20ms timeout plainText = (string)Dispatcher.Invoke(DispatcherPriority.Send, new TimeSpan(0, 0, 0, 0, 20), new DispatcherOperationCallback(delegate(object o) { return GetPlainText(); }), null); } // If there is plain text associated with this control, show it too. if (!String.IsNullOrEmpty(plainText)) { return SR.Get(SRID.ToStringFormatString_Control, base.ToString(), plainText); } return base.ToString(); } #endregion #region Events /// /// PreviewMouseDoubleClick event /// public static readonly RoutedEvent PreviewMouseDoubleClickEvent = EventManager.RegisterRoutedEvent("PreviewMouseDoubleClick", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), typeof(Control)); ////// An event reporting a mouse button was pressed twice in a row. /// public event MouseButtonEventHandler PreviewMouseDoubleClick { add { AddHandler(PreviewMouseDoubleClickEvent, value); } remove { RemoveHandler(PreviewMouseDoubleClickEvent, value); } } ////// An event reporting a mouse button was pressed twice in a row. /// /// Event arguments protected virtual void OnPreviewMouseDoubleClick(MouseButtonEventArgs e) { RaiseEvent(e); } ////// MouseDoubleClick event /// public static readonly RoutedEvent MouseDoubleClickEvent = EventManager.RegisterRoutedEvent("MouseDoubleClick", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), typeof(Control)); ////// An event reporting a mouse button was pressed twice in a row. /// public event MouseButtonEventHandler MouseDoubleClick { add { AddHandler(MouseDoubleClickEvent, value); } remove { RemoveHandler(MouseDoubleClickEvent, value); } } ////// An event reporting a mouse button was pressed twice in a row. /// /// Event arguments protected virtual void OnMouseDoubleClick(MouseButtonEventArgs e) { RaiseEvent(e); } private static void HandleDoubleClick(object sender, MouseButtonEventArgs e) { if (e.ClickCount == 2) { Control ctrl = (Control)sender; MouseButtonEventArgs doubleClick = new MouseButtonEventArgs(e.MouseDevice, e.Timestamp, e.ChangedButton, e.StylusDevice); if ((e.RoutedEvent == UIElement.PreviewMouseLeftButtonDownEvent) || (e.RoutedEvent == UIElement.PreviewMouseRightButtonDownEvent)) { doubleClick.RoutedEvent = PreviewMouseDoubleClickEvent; doubleClick.Source = e.OriginalSource; // Set OriginalSource because initially is null doubleClick.OverrideSource(e.Source); ctrl.OnPreviewMouseDoubleClick(doubleClick); } else { doubleClick.RoutedEvent = MouseDoubleClickEvent; doubleClick.Source = e.OriginalSource; // Set OriginalSource because initially is null doubleClick.OverrideSource(e.Source); ctrl.OnMouseDoubleClick(doubleClick); } // If MouseDoubleClick event is handled - we delegate the state to original MouseButtonEventArgs if (doubleClick.Handled) e.Handled = true; } } #endregion #region Methods ////// Suspends visual state changes. /// internal override void OnPreApplyTemplate() { VisualStateChangeSuspended = true; base.OnPreApplyTemplate(); } ////// Restores visual state changes & updates the visual state without transitions. /// internal override void OnPostApplyTemplate() { base.OnPostApplyTemplate(); VisualStateChangeSuspended = false; UpdateVisualState(false); } ////// Update the current visual state of the control using transitions /// internal void UpdateVisualState() { UpdateVisualState(true); } ////// Update the current visual state of the control /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal void UpdateVisualState(bool useTransitions) { EventTrace.EasyTraceEvent(EventTrace.Keyword.KeywordGeneral | EventTrace.Keyword.KeywordPerf, EventTrace.Level.Info, EventTrace.Event.UpdateVisualStateStart); if (!VisualStateChangeSuspended) { ChangeVisualState(useTransitions); } EventTrace.EasyTraceEvent(EventTrace.Keyword.KeywordGeneral | EventTrace.Keyword.KeywordPerf, EventTrace.Level.Info, EventTrace.Event.UpdateVisualStateEnd); } ////// Change to the correct visual state for the Control. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal virtual void ChangeVisualState(bool useTransitions) { ChangeValidationVisualState(useTransitions); } ////// Common code for putting a control in the validation state. Controls that use the should register /// for change notification of Validation.HasError. /// /// internal void ChangeValidationVisualState(bool useTransitions) { if (Validation.GetHasError(this)) { if (IsKeyboardFocused) { VisualStateManager.GoToState(this, VisualStates.StateInvalidFocused, useTransitions); } else { VisualStateManager.GoToState(this, VisualStates.StateInvalidUnfocused, useTransitions); } } else { VisualStateManager.GoToState(this, VisualStates.StateValid, useTransitions); } } internal static void OnVisualStatePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // Due to inherited properties, its safer not to cast to control because this might get fired for // non-controls. var control = d as Control; if (control != null) { control.UpdateVisualState(); } } ////// Default control measurement is to measure only the first visual child. /// This child would have been created by the inflation of the /// visual tree from the control's style. /// /// Derived controls may want to override this behavior. /// /// The measurement constraints. ///The desired size of the control. protected override Size MeasureOverride(Size constraint) { int count = this.VisualChildrenCount; if (count > 0) { UIElement child = (UIElement)(this.GetVisualChild(0)); if (child != null) { Helper.SetMeasureDataOnChild(this, child, constraint); // pass along MeasureData so it continues down the tree. child.Measure(constraint); return child.DesiredSize; } } return new Size(0.0, 0.0); } ////// Default control arrangement is to only arrange /// the first visual child. No transforms will be applied. /// /// The computed size. protected override Size ArrangeOverride(Size arrangeBounds) { int count = this.VisualChildrenCount; if (count>0) { UIElement child = (UIElement)(this.GetVisualChild(0)); if (child != null) { child.Arrange(new Rect(arrangeBounds)); } } return arrangeBounds; } internal bool ReadControlFlag(ControlBoolFlags reqFlag) { return (_controlBoolField & reqFlag) != 0; } internal void WriteControlFlag(ControlBoolFlags reqFlag, bool set) { if (set) { _controlBoolField |= reqFlag; } else { _controlBoolField &= (~reqFlag); } } #endregion Methods #region Data internal enum ControlBoolFlags : ushort { ContentIsNotLogical = 0x0001, // used in contentcontrol.cs IsSpaceKeyDown = 0x0002, // used in ButtonBase.cs HeaderIsNotLogical = 0x0004, // used in HeaderedContentControl.cs, HeaderedItemsControl.cs CommandDisabled = 0x0008, // used in ButtonBase.cs, MenuItem.cs ContentIsItem = 0x0010, // used in contentcontrol.cs HeaderIsItem = 0x0020, // used in HeaderedContentControl.cs, HeaderedItemsControl.cs ScrollHostValid = 0x0040, // used in ItemsControl.cs ContainsSelection = 0x0080, // used in TreeViewItem.cs VisualStateChangeSuspended = 0x0100, // used in Control.cs } // Property caches private ControlTemplate _templateCache; internal ControlBoolFlags _controlBoolField; // Cache valid bits #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
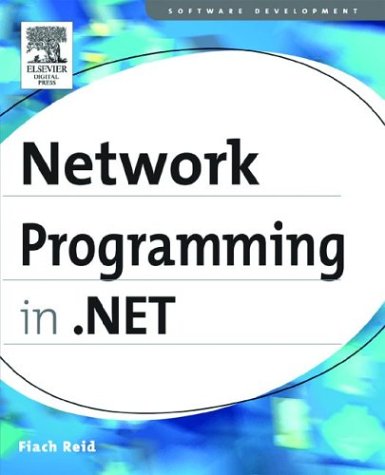
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StreamInfo.cs
- ApplicationSettingsBase.cs
- ConfigurationPermission.cs
- bidPrivateBase.cs
- HtmlElementEventArgs.cs
- SqlStream.cs
- Hex.cs
- Calendar.cs
- DeclarativeCatalogPart.cs
- SolidColorBrush.cs
- UDPClient.cs
- ContextItemManager.cs
- TypefaceCollection.cs
- HttpRuntimeSection.cs
- NumberFunctions.cs
- WindowsTab.cs
- _FtpControlStream.cs
- OleDbInfoMessageEvent.cs
- DBCSCodePageEncoding.cs
- XmlDataCollection.cs
- Transform3DGroup.cs
- InternalBufferOverflowException.cs
- TdsEnums.cs
- EncodingDataItem.cs
- PageRequestManager.cs
- BoundingRectTracker.cs
- MetadataAssemblyHelper.cs
- SmtpNetworkElement.cs
- WebPartConnectionsConfigureVerb.cs
- HttpRequestMessageProperty.cs
- XmlTextEncoder.cs
- XmlChildEnumerator.cs
- TreeNode.cs
- ControlUtil.cs
- XmlAttributeProperties.cs
- XmlCharCheckingReader.cs
- EmptyReadOnlyDictionaryInternal.cs
- MenuAutomationPeer.cs
- _Rfc2616CacheValidators.cs
- FieldBuilder.cs
- Parallel.cs
- FlowDocumentPaginator.cs
- Timer.cs
- ExpressionEditorAttribute.cs
- ResourcePool.cs
- CompilerInfo.cs
- Boolean.cs
- QuotedPairReader.cs
- CompositeCollection.cs
- ToolStripItem.cs
- RenderData.cs
- AutoResetEvent.cs
- FrameworkEventSource.cs
- XmlValueConverter.cs
- ConnectionProviderAttribute.cs
- Overlapped.cs
- BitmapEffectInput.cs
- UseManagedPresentationElement.cs
- XmlSchemaSubstitutionGroup.cs
- BamlTreeNode.cs
- XmlSchemaSimpleContentExtension.cs
- DataServicePagingProviderWrapper.cs
- PolicyVersionConverter.cs
- CodeDOMUtility.cs
- Expander.cs
- hebrewshape.cs
- Propagator.Evaluator.cs
- sortedlist.cs
- NgenServicingAttributes.cs
- Triangle.cs
- ArgumentOutOfRangeException.cs
- TypeSystemHelpers.cs
- MaterialCollection.cs
- SplitContainer.cs
- ToolStripDropDownItem.cs
- InsufficientExecutionStackException.cs
- XamlToRtfWriter.cs
- LambdaCompiler.Binary.cs
- COM2FontConverter.cs
- SystemEvents.cs
- DataGridComboBoxColumn.cs
- SaveFileDialog.cs
- Rotation3DAnimationBase.cs
- ExecutionContext.cs
- BackEase.cs
- ProtectedConfigurationSection.cs
- WindowsEditBox.cs
- VisualBasic.cs
- EncryptedReference.cs
- ExpressionEditorAttribute.cs
- BamlBinaryWriter.cs
- SqlDataSourceFilteringEventArgs.cs
- Themes.cs
- SpeechRecognizer.cs
- RoleGroupCollection.cs
- ImageAttributes.cs
- MSG.cs
- AuthStoreRoleProvider.cs
- IncrementalHitTester.cs
- SafeEventLogWriteHandle.cs