Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / LocalizabilityAttribute.cs / 1305600 / LocalizabilityAttribute.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: LocalizabiltyAttribute.cs // // Contents: Localizability attributes // // Created: 3/17/2004 Garyyang // 7/19/2004 garyyang, Update according to new spec. // // //----------------------------------------------------------------------- using System; using System.ComponentModel; namespace System.Windows { ////// Specifies the localization preferences for a class or property in Baml /// The attribute can be specified on Class, Property and Method /// [AttributeUsage( AttributeTargets.Class | AttributeTargets.Property | AttributeTargets.Field | AttributeTargets.Enum | AttributeTargets.Struct, AllowMultiple = false, Inherited = true) ] public sealed class LocalizabilityAttribute : Attribute { ////// Construct a LocalizabilityAttribute to describe the localizability of a property. /// Modifiability property default to Modifiability.Modifiable, and Readability property /// default to Readability.Readable. /// /// the string category given to the item public LocalizabilityAttribute(LocalizationCategory category) { if ( category < LocalizationCategory.None || category > LocalizationCategory.NeverLocalize) { throw new InvalidEnumArgumentException( "category", (int)category, typeof(LocalizationCategory) ); } _category = category; _readability = Readability.Readable; _modifiability = Modifiability.Modifiable; } ////// String category /// ///gets or sets the string category for the item public LocalizationCategory Category { // should have only getter, because it is a required parameter to the constructor get { return _category; } } ////// Get or set the readability of the attribute's targeted value /// ///Readability public Readability Readability { get { return _readability; } set { if ( value != Readability.Unreadable && value != Readability.Readable && value != Readability.Inherit) { throw new InvalidEnumArgumentException("Readability", (int) value, typeof(Readability)); } _readability = value; } } ////// Get or set the modifiability of the attribute's targeted value /// ///Modifiability public Modifiability Modifiability { get { return _modifiability; } set { if ( value != Modifiability.Unmodifiable && value != Modifiability.Modifiable && value != Modifiability.Inherit) { throw new InvalidEnumArgumentException("Modifiability", (int) value, typeof(Modifiability)); } _modifiability = value; } } //-------------------------------------------- // Private members //-------------------------------------------- private LocalizationCategory _category; private Readability _readability; private Modifiability _modifiability; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
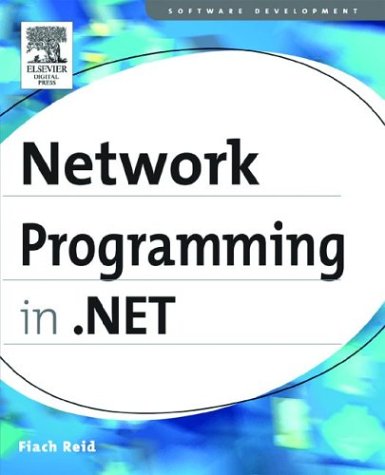
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollContentPresenter.cs
- InputDevice.cs
- MatrixTransform.cs
- HashCryptoHandle.cs
- EventQueueState.cs
- dbenumerator.cs
- TableLayoutRowStyleCollection.cs
- XmlIncludeAttribute.cs
- RubberbandSelector.cs
- NotifyInputEventArgs.cs
- FormatException.cs
- SaveFileDialog.cs
- BooleanAnimationUsingKeyFrames.cs
- WorkflowApplicationEventArgs.cs
- Adorner.cs
- GroupByQueryOperator.cs
- PropertyKey.cs
- Logging.cs
- MediaTimeline.cs
- UserControlAutomationPeer.cs
- RijndaelManaged.cs
- NullRuntimeConfig.cs
- GeometryHitTestResult.cs
- TypeReference.cs
- OracleConnectionStringBuilder.cs
- FixedTextSelectionProcessor.cs
- InfocardExtendedInformationCollection.cs
- ColorConvertedBitmap.cs
- EventTrigger.cs
- WeakReference.cs
- _NestedMultipleAsyncResult.cs
- DeferredSelectedIndexReference.cs
- XamlTreeBuilderBamlRecordWriter.cs
- ObjectListSelectEventArgs.cs
- Error.cs
- AlphaSortedEnumConverter.cs
- oledbmetadatacolumnnames.cs
- GridViewHeaderRowPresenter.cs
- LZCodec.cs
- DataMemberConverter.cs
- followingquery.cs
- SqlLiftIndependentRowExpressions.cs
- DeferredSelectedIndexReference.cs
- XamlBuildProvider.cs
- StylusPointProperties.cs
- ComEventsHelper.cs
- NetTcpSecurityElement.cs
- DBConnection.cs
- AssemblyNameEqualityComparer.cs
- SrgsElement.cs
- HebrewCalendar.cs
- DoubleAnimationClockResource.cs
- ObjectComplexPropertyMapping.cs
- XmlLanguage.cs
- MarshalByRefObject.cs
- TableItemStyle.cs
- ListItemParagraph.cs
- ExceptionDetail.cs
- ProcessHostServerConfig.cs
- WebCategoryAttribute.cs
- AddingNewEventArgs.cs
- ThreadAttributes.cs
- TraceHandlerErrorFormatter.cs
- XmlCharCheckingWriter.cs
- ToolStripProgressBar.cs
- AuthenticationException.cs
- InvokePattern.cs
- RijndaelManaged.cs
- QueryableDataSource.cs
- Timer.cs
- LayoutTable.cs
- MultiSelectRootGridEntry.cs
- WMICapabilities.cs
- FixedDocument.cs
- TaskExceptionHolder.cs
- JpegBitmapEncoder.cs
- Utils.cs
- DataControlReference.cs
- Bidi.cs
- StringKeyFrameCollection.cs
- Errors.cs
- DependencyObjectCodeDomSerializer.cs
- BufferedWebEventProvider.cs
- RSAOAEPKeyExchangeDeformatter.cs
- WindowsFormsHelpers.cs
- InputBuffer.cs
- ClaimSet.cs
- PrimitiveType.cs
- StylusPlugInCollection.cs
- QilReference.cs
- CannotUnloadAppDomainException.cs
- DSASignatureFormatter.cs
- LocalizedNameDescriptionPair.cs
- UshortList2.cs
- DeviceContext.cs
- DocumentGridContextMenu.cs
- ContentControl.cs
- PageStatePersister.cs
- TimeManager.cs
- ReadOnlyDataSource.cs