Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Security / MachineKey.cs / 1305376 / MachineKey.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * MachineKey * * Copyright (c) 2009 Microsoft Corporation */ namespace System.Web.Security { using System.Web.Configuration; ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// public enum MachineKeyProtection { All, Encryption, Validation } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// public static class MachineKey { ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// public static string Encode(byte[] data, MachineKeyProtection protectionOption) { if (data == null) throw new ArgumentNullException("data"); ////////////////////////////////////////////////////////////////////// // Step 1: Get the MAC and add to the blob if (protectionOption == MachineKeyProtection.All || protectionOption == MachineKeyProtection.Validation) { byte [] bHash = MachineKeySection.HashData(data, null, 0, data.Length); byte [] bAll = new byte[bHash.Length + data.Length]; Buffer.BlockCopy(data, 0, bAll, 0, data.Length); Buffer.BlockCopy(bHash, 0, bAll, data.Length, bHash.Length); data = bAll; } if (protectionOption == MachineKeyProtection.All || protectionOption == MachineKeyProtection.Encryption) { ////////////////////////////////////////////////////////////////////// // Step 2: Encryption data = MachineKeySection.EncryptOrDecryptData(true, data, null, 0, data.Length, false, false, IVType.Random); } ////////////////////////////////////////////////////////////////////// // Step 3: Covert the buffer to HEX string and return it return MachineKeySection.ByteArrayToHexString(data, 0); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// public static byte [] Decode(string encodedData, MachineKeyProtection protectionOption) { if (encodedData == null) throw new ArgumentNullException("encodedData"); if ((encodedData.Length % 2) != 0) throw new ArgumentException(null, "encodedData"); byte [] data = null; try { ////////////////////////////////////////////////////////////////////// // Step 1: Covert the HEX string to byte array data = MachineKeySection.HexStringToByteArray(encodedData); } catch { throw new ArgumentException(null, "encodedData"); } if (data == null || data.Length < 1) throw new ArgumentException(null, "encodedData"); if (protectionOption == MachineKeyProtection.All || protectionOption == MachineKeyProtection.Encryption) { ////////////////////////////////////////////////////////////////// // Step 2: Decrypt the data data = MachineKeySection.EncryptOrDecryptData(false, data, null, 0, data.Length, false, false, IVType.Random); if (data == null) return null; } if (protectionOption == MachineKeyProtection.All || protectionOption == MachineKeyProtection.Validation) { ////////////////////////////////////////////////////////////////// // Step 3a: Remove the hash from the end of the data if (data.Length < MachineKeySection.HashSize) return null; byte [] originalData = data; data = new byte[originalData.Length - MachineKeySection.HashSize]; Buffer.BlockCopy(originalData, 0, data, 0, data.Length); ////////////////////////////////////////////////////////////////// // Step 3b: Calculate the hash and make sure it matches byte [] bHash = MachineKeySection.HashData(data, null, 0, data.Length); if (bHash == null || bHash.Length != MachineKeySection.HashSize) return null; // Sizes don't match for(int iter=0; iter
Link Menu
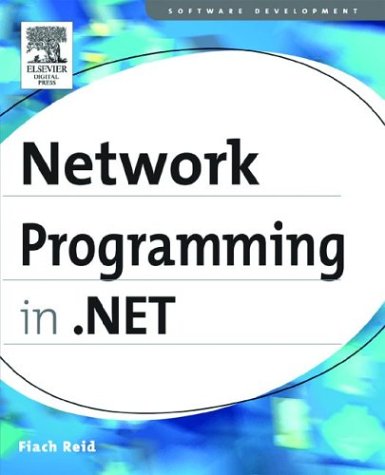
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnmanagedBitmapWrapper.cs
- CodeIterationStatement.cs
- KerberosSecurityTokenProvider.cs
- PDBReader.cs
- Console.cs
- HitTestFilterBehavior.cs
- EditingCommands.cs
- BreakRecordTable.cs
- DataError.cs
- ReachFixedPageSerializerAsync.cs
- OutputScopeManager.cs
- GlyphingCache.cs
- ValidatorCompatibilityHelper.cs
- ToolStripOverflow.cs
- AppDomainFactory.cs
- ToolTipService.cs
- VisualBasic.cs
- RetrieveVirtualItemEventArgs.cs
- ListChangedEventArgs.cs
- CodeDOMProvider.cs
- ExtensionQuery.cs
- CodeTypeMember.cs
- SymLanguageVendor.cs
- Margins.cs
- UTF8Encoding.cs
- XmlSchemaParticle.cs
- ExtensionSurface.cs
- ParenthesizePropertyNameAttribute.cs
- ObjectConverter.cs
- EdmProperty.cs
- HttpCookieCollection.cs
- BitmapEffectRenderDataResource.cs
- SqlNodeTypeOperators.cs
- XPathScanner.cs
- QueryOperatorEnumerator.cs
- Win32Exception.cs
- FullTextLine.cs
- InvalidCommandTreeException.cs
- DateTimeFormatInfo.cs
- ProfileEventArgs.cs
- ResourceIDHelper.cs
- DbConnectionPoolGroupProviderInfo.cs
- SelectionListDesigner.cs
- WebPart.cs
- WinInet.cs
- DynamicControl.cs
- NamespaceQuery.cs
- WindowsAuthenticationModule.cs
- DataTableReaderListener.cs
- Environment.cs
- KeyManager.cs
- WebHttpEndpointElement.cs
- HashSetEqualityComparer.cs
- XmlBoundElement.cs
- TextRange.cs
- TreeIterator.cs
- Composition.cs
- ObjectDataSource.cs
- ValueOfAction.cs
- KernelTypeValidation.cs
- ManifestResourceInfo.cs
- smtppermission.cs
- PeerReferralPolicy.cs
- MemberAccessException.cs
- MetadataCache.cs
- DropSourceBehavior.cs
- SignatureDescription.cs
- BaseProcessor.cs
- BasicBrowserDialog.designer.cs
- WorkflowApplicationException.cs
- BufferedReadStream.cs
- CodeCastExpression.cs
- LoadedEvent.cs
- ConfigXmlAttribute.cs
- HandlerBase.cs
- MapPathBasedVirtualPathProvider.cs
- NonParentingControl.cs
- MethodAccessException.cs
- List.cs
- StyleBamlTreeBuilder.cs
- Rotation3D.cs
- SingleResultAttribute.cs
- MarshalDirectiveException.cs
- TrustLevelCollection.cs
- DefaultClaimSet.cs
- TransformGroup.cs
- EntityTransaction.cs
- ScriptIgnoreAttribute.cs
- DataBindEngine.cs
- BaseCodePageEncoding.cs
- ConnectionConsumerAttribute.cs
- ConfigurationLockCollection.cs
- IssuanceLicense.cs
- Serializer.cs
- WindowsContainer.cs
- NameValueConfigurationCollection.cs
- WindowsListBox.cs
- InputLanguageManager.cs
- BindingCollection.cs
- Queue.cs