Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / DataAccess / ADConnectionHelper.cs / 1305376 / ADConnectionHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.DataAccess { using System.Net; using System.Diagnostics; using System.Web.Hosting; using System.Web.Security; using System.DirectoryServices; using System.DirectoryServices.Protocols; internal static class ActiveDirectoryConnectionHelper { internal static DirectoryEntryHolder GetDirectoryEntry(DirectoryInformation directoryInfo, string objectDN, bool revertImpersonation) { Debug.Assert ((objectDN != null) && (objectDN.Length != 0)); // // Get the adspath and create a directory entry holder // DirectoryEntryHolder holder = new DirectoryEntryHolder(new DirectoryEntry ( directoryInfo.GetADsPath(objectDN), directoryInfo.GetUsername(), directoryInfo.GetPassword(), directoryInfo.AuthenticationTypes)); // // If revertImpersonation is true, we need to revert // holder.Open(null, revertImpersonation); return holder; } } internal sealed class DirectoryEntryHolder { private ImpersonationContext ctx = null; private bool opened; private DirectoryEntry entry; internal DirectoryEntryHolder (DirectoryEntry entry) { Debug.Assert (entry != null); this.entry = entry; } internal void Open (HttpContext context, bool revertImpersonate) { if (opened) return; // Already opened // // Revert client impersonation if required // if (revertImpersonate) { ctx = new ApplicationImpersonationContext(); } else { ctx = null; } opened = true; // Open worked! } internal void Close () { if (!opened) // Not open! return; entry.Dispose(); RestoreImpersonation(); opened = false; } internal void RestoreImpersonation() { // Restore impersonation if (ctx != null) { ctx.Undo(); ctx = null; } } internal DirectoryEntry DirectoryEntry { get { return entry; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
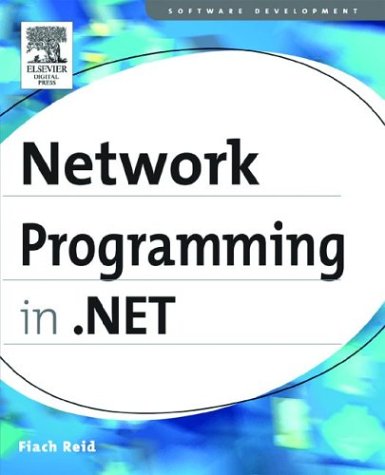
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Menu.cs
- Grid.cs
- DataBindingHandlerAttribute.cs
- BindingList.cs
- HScrollBar.cs
- BatchParser.cs
- ChannelDispatcher.cs
- SettingsAttributeDictionary.cs
- DBConnectionString.cs
- AnyAllSearchOperator.cs
- WebContext.cs
- InvalidProgramException.cs
- GetParentChain.cs
- ParseNumbers.cs
- EDesignUtil.cs
- TiffBitmapEncoder.cs
- MobileUserControl.cs
- StdRegProviderWrapper.cs
- OutputCacheSection.cs
- ToolStripItemEventArgs.cs
- DocumentAutomationPeer.cs
- mediaeventargs.cs
- _BaseOverlappedAsyncResult.cs
- DateRangeEvent.cs
- EntityType.cs
- ExtendedPropertyCollection.cs
- UnmanagedBitmapWrapper.cs
- ImageSourceConverter.cs
- TextEditorThreadLocalStore.cs
- SafeFileMapViewHandle.cs
- XamlFilter.cs
- WindowsRegion.cs
- DataSourceListEditor.cs
- SizeChangedEventArgs.cs
- FloaterParaClient.cs
- ProtocolsConfigurationHandler.cs
- PermissionAttributes.cs
- XmlWriterDelegator.cs
- OdbcPermission.cs
- DbProviderConfigurationHandler.cs
- SoapCodeExporter.cs
- ByteConverter.cs
- _DomainName.cs
- DBCommand.cs
- ThreadAttributes.cs
- TemplateField.cs
- IOException.cs
- PageAdapter.cs
- RotateTransform.cs
- LinkTarget.cs
- StrokeNode.cs
- AppDomainManager.cs
- UDPClient.cs
- SortedList.cs
- SendDesigner.xaml.cs
- ColumnBinding.cs
- WebPartZoneCollection.cs
- XXXInfos.cs
- loginstatus.cs
- ColorAnimationUsingKeyFrames.cs
- RTLAwareMessageBox.cs
- RegexParser.cs
- ToolZone.cs
- ListItemCollection.cs
- InternalResources.cs
- ArrayList.cs
- SQLByteStorage.cs
- PenThreadPool.cs
- Size3D.cs
- ConnectionPoint.cs
- ConnectionStringsExpressionEditor.cs
- TimeoutException.cs
- DecoderNLS.cs
- VolatileEnlistmentMultiplexing.cs
- StdValidatorsAndConverters.cs
- SqlBulkCopyColumnMappingCollection.cs
- StickyNote.cs
- WebDisplayNameAttribute.cs
- ListView.cs
- _FtpDataStream.cs
- Facet.cs
- PathNode.cs
- XmlSerializationReader.cs
- FixedSOMTextRun.cs
- ExtractCollection.cs
- InkCanvasInnerCanvas.cs
- Wizard.cs
- CodeArgumentReferenceExpression.cs
- GenerateTemporaryTargetAssembly.cs
- EntityViewGenerationConstants.cs
- DataGridSortingEventArgs.cs
- LineMetrics.cs
- X509SubjectKeyIdentifierClause.cs
- XDeferredAxisSource.cs
- XmlILTrace.cs
- InvokeHandlers.cs
- StylusLogic.cs
- WebConfigurationFileMap.cs
- DiffuseMaterial.cs
- ArraySegment.cs