Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / ScriptResourceDefinition.cs / 1305376 / ScriptResourceDefinition.cs
namespace System.Web.UI { using System; using System.Reflection; public class ScriptResourceDefinition : IScriptResourceDefinition { private string _path; private string _debugPath; private string _resourceName; private Assembly _resourceAssembly; private string _cdnPath; private string _cdnDebugPath; private string _cdnPathSecureConnection; private string _cdnDebugPathSecureConnection; private bool _cdnSupportsSecureConnection; public string CdnDebugPath { get { return _cdnDebugPath ?? String.Empty; } set { _cdnDebugPath = value; } } public string CdnPath { get { return _cdnPath ?? String.Empty; } set { _cdnPath = value; } } internal string CdnDebugPathSecureConnection { get { if (_cdnDebugPathSecureConnection == null) { _cdnDebugPathSecureConnection = GetSecureCdnPath(CdnDebugPath); } return _cdnDebugPathSecureConnection; } } internal string CdnPathSecureConnection { get { if (_cdnPathSecureConnection == null) { _cdnPathSecureConnection = GetSecureCdnPath(CdnPath); } return _cdnPathSecureConnection; } } public bool CdnSupportsSecureConnection { get { return _cdnSupportsSecureConnection; } set { _cdnSupportsSecureConnection = value; } } public string DebugPath { get { return _debugPath ?? String.Empty; } set { _debugPath = value; } } public string Path { get { return _path ?? String.Empty; } set { _path = value; } } public Assembly ResourceAssembly { get { return _resourceAssembly; } set { _resourceAssembly = value; } } public string ResourceName { get { return _resourceName ?? String.Empty; } set { _resourceName = value; } } private string GetSecureCdnPath(string unsecurePath) { string cdnPath = String.Empty; if (!String.IsNullOrEmpty(unsecurePath)) { if (_cdnSupportsSecureConnection) { // convert 'http' to 'https' if (unsecurePath.StartsWith("http://", StringComparison.OrdinalIgnoreCase)) { cdnPath = "https" + unsecurePath.Substring(4); } else { // cdnPath is not 'http' so we cannot determine the secure path cdnPath = String.Empty; } } else { cdnPath = String.Empty; } } return cdnPath; } string IScriptResourceDefinition.CdnPathSecureConnection { get { return CdnPathSecureConnection; } } string IScriptResourceDefinition.CdnDebugPathSecureConnection { get { return CdnDebugPathSecureConnection; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
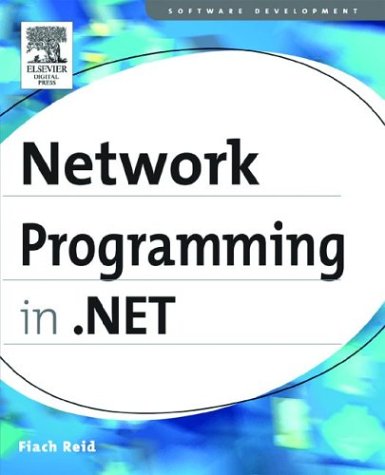
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MaskedTextBoxDesigner.cs
- SafeProcessHandle.cs
- SqlConnectionStringBuilder.cs
- WindowsAuthenticationModule.cs
- PersonalizableTypeEntry.cs
- RemotingConfigParser.cs
- DeploymentExceptionMapper.cs
- ToolStripRenderer.cs
- RepeatInfo.cs
- TextComposition.cs
- ReaderWriterLock.cs
- templategroup.cs
- OciEnlistContext.cs
- UInt32.cs
- relpropertyhelper.cs
- WebEventCodes.cs
- ClassImporter.cs
- LinqDataView.cs
- BitmapMetadataEnumerator.cs
- TemplateControl.cs
- GetBrowserTokenRequest.cs
- DbCommandTree.cs
- DetailsViewDeletedEventArgs.cs
- UnsafeNativeMethodsCLR.cs
- SqlConnectionString.cs
- AuthenticationService.cs
- Rotation3D.cs
- TypeConverter.cs
- QueryOperator.cs
- DataColumnPropertyDescriptor.cs
- QuaternionConverter.cs
- Slider.cs
- ProxyRpc.cs
- BypassElement.cs
- ClientRuntimeConfig.cs
- TransformPatternIdentifiers.cs
- FixedDocument.cs
- BrushMappingModeValidation.cs
- ComboBoxItem.cs
- EditorZone.cs
- Positioning.cs
- SqlSupersetValidator.cs
- EdmComplexTypeAttribute.cs
- TextStore.cs
- HwndStylusInputProvider.cs
- ServiceX509SecurityTokenProvider.cs
- RadioButton.cs
- ADRoleFactory.cs
- SystemColorTracker.cs
- CryptoStream.cs
- Int32AnimationUsingKeyFrames.cs
- CachedFontFace.cs
- ColumnResizeUndoUnit.cs
- MemberInfoSerializationHolder.cs
- ToolboxSnapDragDropEventArgs.cs
- UserPreferenceChangedEventArgs.cs
- ManifestResourceInfo.cs
- SettingsBindableAttribute.cs
- DataBoundControlAdapter.cs
- SQLDateTimeStorage.cs
- AppDomainProtocolHandler.cs
- XmlBoundElement.cs
- TreeIterator.cs
- HybridDictionary.cs
- LinearKeyFrames.cs
- TaskFileService.cs
- TargetException.cs
- WebPartCancelEventArgs.cs
- DummyDataSource.cs
- Expression.DebuggerProxy.cs
- DesignTableCollection.cs
- xml.cs
- ApplicationSettingsBase.cs
- TypeNameHelper.cs
- BufferedReadStream.cs
- ServiceDescriptions.cs
- AnonymousIdentificationSection.cs
- XmlNamespaceMappingCollection.cs
- Visual.cs
- BoundingRectTracker.cs
- Queue.cs
- AttributeCollection.cs
- GetKeyedHashRequest.cs
- propertytag.cs
- Compiler.cs
- ServicePointManagerElement.cs
- XmlEncodedRawTextWriter.cs
- PageParserFilter.cs
- Line.cs
- DependencyObjectProvider.cs
- DataControlFieldHeaderCell.cs
- InputLanguageCollection.cs
- ComplusEndpointConfigContainer.cs
- MatchingStyle.cs
- HtmlDocument.cs
- MessageQueueCriteria.cs
- RotateTransform.cs
- VisualBasicExpressionConverter.cs
- OperationInvokerTrace.cs
- CodeVariableDeclarationStatement.cs