Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / XPathArrayIterator.cs / 1305376 / XPathArrayIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Text; using System.Xml; using System.Xml.XPath; namespace MS.Internal.Xml.XPath { [DebuggerDisplay("Position={CurrentPosition}, Current={debuggerDisplayProxy, nq}")] internal class XPathArrayIterator : ResetableIterator { protected IList list; protected int index; public XPathArrayIterator(IList list) { this.list = list; } public XPathArrayIterator(XPathArrayIterator it) { this.list = it.list; this.index = it.index; } public XPathArrayIterator(XPathNodeIterator nodeIterator) { this.list = new ArrayList(); while (nodeIterator.MoveNext()) { this.list.Add(nodeIterator.Current.Clone()); } } public IList AsList { get { return this.list; } } public override XPathNodeIterator Clone() { return new XPathArrayIterator(this); } public override XPathNavigator Current { get { Debug.Assert(index <= list.Count); if (index < 1) { throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); } return (XPathNavigator) list[index - 1]; } } public override int CurrentPosition { get { return index; } } public override int Count { get { return list.Count; } } public override bool MoveNext() { Debug.Assert(index <= list.Count); if (index == list.Count) { return false; } index++; return true; } public override void Reset() { index = 0; } public override IEnumerator GetEnumerator() { return list.GetEnumerator(); } private object debuggerDisplayProxy { get { return index < 1 ? null : (object)new XPathNavigator.DebuggerDisplayProxy(Current); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
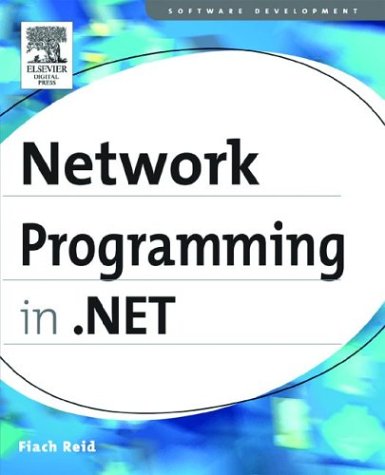
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BamlTreeNode.cs
- XDeferredAxisSource.cs
- MappedMetaModel.cs
- XmlException.cs
- SpeechSynthesizer.cs
- DataObjectMethodAttribute.cs
- CodeTypeDeclaration.cs
- HeaderedContentControl.cs
- FixedSchema.cs
- DeleteStoreRequest.cs
- DesignConnectionCollection.cs
- WindowHideOrCloseTracker.cs
- ListSourceHelper.cs
- TextElementEnumerator.cs
- LayoutInformation.cs
- ConstraintCollection.cs
- ExpressionBinding.cs
- hresults.cs
- WaitHandleCannotBeOpenedException.cs
- DesignerObjectListAdapter.cs
- MailSettingsSection.cs
- HtmlInputImage.cs
- EventSinkActivityDesigner.cs
- TreeBuilderXamlTranslator.cs
- GridViewUpdatedEventArgs.cs
- SmiTypedGetterSetter.cs
- TextDocumentView.cs
- StopStoryboard.cs
- DigestTraceRecordHelper.cs
- SqlProfileProvider.cs
- BrowserDefinition.cs
- BehaviorEditorPart.cs
- Int64.cs
- FormViewUpdatedEventArgs.cs
- EnumMemberAttribute.cs
- StopRoutingHandler.cs
- ListViewInsertionMark.cs
- ObjectAssociationEndMapping.cs
- RowToParametersTransformer.cs
- GridViewCommandEventArgs.cs
- BitmapVisualManager.cs
- SocketInformation.cs
- WorkflowInstanceTerminatedRecord.cs
- BindingsCollection.cs
- TypefaceCollection.cs
- QueryReaderSettings.cs
- TextEffectResolver.cs
- Mouse.cs
- VirtualDirectoryMappingCollection.cs
- SmiGettersStream.cs
- AnalyzedTree.cs
- ExtendedProtectionPolicyTypeConverter.cs
- SystemUdpStatistics.cs
- GatewayDefinition.cs
- TableRowGroupCollection.cs
- SpellerHighlightLayer.cs
- CompositeControl.cs
- ObjectManager.cs
- ProxyGenerator.cs
- FixedLineResult.cs
- DbTransaction.cs
- InstanceDescriptor.cs
- PageRouteHandler.cs
- ModelUIElement3D.cs
- BrowserCapabilitiesFactory.cs
- MarshalByValueComponent.cs
- CharConverter.cs
- WasEndpointConfigContainer.cs
- FilterableAttribute.cs
- TableCellCollection.cs
- KnownBoxes.cs
- TreePrinter.cs
- SpeechEvent.cs
- EncoderParameter.cs
- AppDomainGrammarProxy.cs
- RectangleGeometry.cs
- DoubleAnimationUsingKeyFrames.cs
- ExpressionQuoter.cs
- DataGridBoolColumn.cs
- ScrollChrome.cs
- DbgUtil.cs
- Baml2006ReaderFrame.cs
- OneOfTypeConst.cs
- ZeroOpNode.cs
- CollectionViewProxy.cs
- UserControl.cs
- NodeFunctions.cs
- GeometryModel3D.cs
- ISAPIApplicationHost.cs
- SubpageParaClient.cs
- MediaEntryAttribute.cs
- SecurityTokenResolver.cs
- ExtentCqlBlock.cs
- SelectionItemPattern.cs
- WorkflowWebService.cs
- TdsValueSetter.cs
- SystemIPGlobalStatistics.cs
- WSSecurityPolicy11.cs
- SiteMapNodeItem.cs
- XDRSchema.cs