Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Bits.cs / 1305376 / Bits.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Diagnostics; ////// Contains static utility methods used to manipulate bits in a word. /// internal static class Bits { private static readonly uint MASK_0101010101010101 = 0x55555555; private static readonly uint MASK_0011001100110011 = 0x33333333; private static readonly uint MASK_0000111100001111 = 0x0f0f0f0f; private static readonly uint MASK_0000000011111111 = 0x00ff00ff; private static readonly uint MASK_1111111111111111 = 0x0000ffff; ////// Returns the number of 1 bits in an unsigned integer. Counts bits by divide-and-conquer method, /// first computing 16 2-bit counts, then 8 4-bit counts, then 4 8-bit counts, then 2 16-bit counts, /// and finally 1 32-bit count. /// public static int Count(uint num) { num = (num & MASK_0101010101010101) + ((num >> 1) & MASK_0101010101010101); num = (num & MASK_0011001100110011) + ((num >> 2) & MASK_0011001100110011); num = (num & MASK_0000111100001111) + ((num >> 4) & MASK_0000111100001111); num = (num & MASK_0000000011111111) + ((num >> 8) & MASK_0000000011111111); num = (num & MASK_1111111111111111) + (num >> 16); return (int) num; } ////// Returns true if the unsigned integer has exactly one bit set. /// public static bool ExactlyOne(uint num) { return num != 0 && (num & (num - 1)) == 0; } #if !SILVERLIGHT // These methods are not used in Silverlight ////// Returns true if the unsigned integer has more than one bit set. /// public static bool MoreThanOne(uint num) { return (num & (num - 1)) != 0; } ////// Clear the least significant bit that is set and return the result. /// public static uint ClearLeast(uint num) { return num & (num - 1); } #endif ////// Compute the 1-based position of the least sigificant bit that is set, and return it (return 0 if no bits are set). /// (e.g. 0x1001100 will return 3, since the 3rd bit is set). /// public static int LeastPosition(uint num) { if (num == 0) return 0; return Count(num ^ (num - 1)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
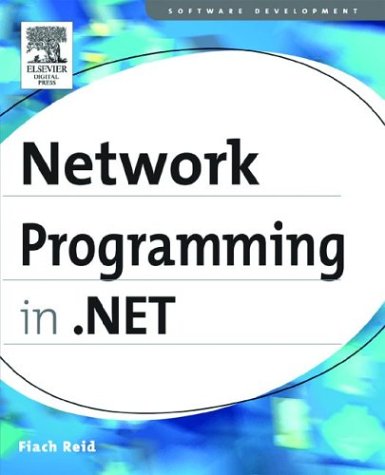
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextBreakpoint.cs
- OracleConnectionString.cs
- TransactedReceiveData.cs
- ActivityDefaults.cs
- AddInPipelineAttributes.cs
- Soap12FormatExtensions.cs
- TimeZone.cs
- smtpconnection.cs
- MDIControlStrip.cs
- StringBuilder.cs
- XmlNodeList.cs
- NetworkCredential.cs
- StatusBarPanelClickEvent.cs
- DataGridViewColumn.cs
- SyndicationLink.cs
- SharedPerformanceCounter.cs
- RadioButtonRenderer.cs
- TextSegment.cs
- DefaultPropertyAttribute.cs
- TabRenderer.cs
- Figure.cs
- SystemNetHelpers.cs
- SqlRowUpdatedEvent.cs
- IncomingWebRequestContext.cs
- BitmapEffectrendercontext.cs
- XmlSchemaCompilationSettings.cs
- IconBitmapDecoder.cs
- CodeDirectiveCollection.cs
- ToolStripPanelSelectionGlyph.cs
- OleDbPropertySetGuid.cs
- WizardStepBase.cs
- WebPartVerbCollection.cs
- BatchWriter.cs
- QilInvokeEarlyBound.cs
- CodePageEncoding.cs
- ProfilePropertyNameValidator.cs
- CodeSpit.cs
- SafeRegistryKey.cs
- NonSerializedAttribute.cs
- OracleDataAdapter.cs
- NonVisualControlAttribute.cs
- StorageScalarPropertyMapping.cs
- KerberosSecurityTokenProvider.cs
- XhtmlBasicTextViewAdapter.cs
- TextServicesCompartment.cs
- SqlTopReducer.cs
- MarginCollapsingState.cs
- ConditionalAttribute.cs
- ModelPropertyCollectionImpl.cs
- ExpandSegmentCollection.cs
- ProcessInfo.cs
- RelationshipDetailsCollection.cs
- TitleStyle.cs
- FullTextBreakpoint.cs
- WindowsRichEditRange.cs
- ParameterEditorUserControl.cs
- Parameter.cs
- OutArgumentConverter.cs
- SoapCodeExporter.cs
- NavigationExpr.cs
- Random.cs
- TextModifier.cs
- SkewTransform.cs
- ParsedRoute.cs
- ElementAction.cs
- XPathSelectionIterator.cs
- ContextMenu.cs
- PlaceHolder.cs
- TableParagraph.cs
- DataGridPreparingCellForEditEventArgs.cs
- FormViewPagerRow.cs
- OrderPreservingPipeliningSpoolingTask.cs
- XmlQueryOutput.cs
- IisTraceWebEventProvider.cs
- WhitespaceRuleReader.cs
- BuildResult.cs
- clipboard.cs
- CodeIdentifier.cs
- PerfCounters.cs
- SqlFacetAttribute.cs
- ScrollBarRenderer.cs
- PartBasedPackageProperties.cs
- TextTreeTextElementNode.cs
- ChangeNode.cs
- NestedContainer.cs
- ColorTranslator.cs
- WebControlToolBoxItem.cs
- ServiceDescriptionContext.cs
- CharUnicodeInfo.cs
- EncryptedPackage.cs
- GcHandle.cs
- MediaScriptCommandRoutedEventArgs.cs
- XmlSchemaInfo.cs
- SerialPort.cs
- Storyboard.cs
- DataTableNewRowEvent.cs
- TypeDescriptor.cs
- JavaScriptSerializer.cs
- NGCSerializationManagerAsync.cs
- Page.cs