Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripOverflowButton.cs / 1305376 / ToolStripOverflowButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Windows.Forms.Design; ////// /// ToolStripOverflowButton /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.None)] public class ToolStripOverflowButton : ToolStripDropDownButton { // we need to cache this away as the Parent property gets reset a lot. private ToolStrip parentToolStrip; ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] internal ToolStripOverflowButton(ToolStrip parentToolStrip) { SupportsItemClick = false; this.parentToolStrip = parentToolStrip; } /// protected internal override Padding DefaultMargin { get { return Padding.Empty; } } /// public override bool HasDropDownItems { get { return this.ParentInternal.OverflowItems.Count > 0; } } internal override bool OppositeDropDownAlign { get { return true; } } internal ToolStrip ParentToolStrip { get { return parentToolStrip; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool RightToLeftAutoMirrorImage { get { return base.RightToLeftAutoMirrorImage; } set { base.RightToLeftAutoMirrorImage = value; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripOverflowButtonAccessibleObject(this); } /// protected override ToolStripDropDown CreateDefaultDropDown() { // AutoGenerate a Winbar DropDown - set the property so we hook events return new ToolStripOverflow(this); } /// public override Size GetPreferredSize(Size constrainingSize) { Size preferredSize = constrainingSize; if (this.ParentInternal != null) { if (this.ParentInternal.Orientation == Orientation.Horizontal) { preferredSize.Width = Math.Min(constrainingSize.Width, 16); } else { preferredSize.Height = Math.Min(constrainingSize.Height, 16); } } return preferredSize + this.Padding.Size; } // make sure the Overflow button extends from edge-edge. (Ignore Padding/Margin). internal protected override void SetBounds(Rectangle bounds) { if (ParentInternal != null && ParentInternal.LayoutEngine is ToolStripSplitStackLayout) { if (ParentInternal.Orientation == Orientation.Horizontal) { bounds.Height = ParentInternal.Height; bounds.Y = 0; } else { bounds.Width = ParentInternal.Width; bounds.X = 0; } } base.SetBounds(bounds); } /// protected override void OnPaint(PaintEventArgs e) { if (this.ParentInternal != null) { ToolStripRenderer renderer = this.ParentInternal.Renderer; renderer.DrawOverflowButtonBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); } } internal class ToolStripOverflowButtonAccessibleObject : ToolStripDropDownItemAccessibleObject { private string stockName; public ToolStripOverflowButtonAccessibleObject(ToolStripOverflowButton owner) : base(owner){ } public override string Name { get { string name = Owner.AccessibleName; if (name != null) { return name; } if (string.IsNullOrEmpty(stockName)) { stockName = SR.GetString(SR.ToolStripOptions); } return stockName; } set { base.Name = value; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
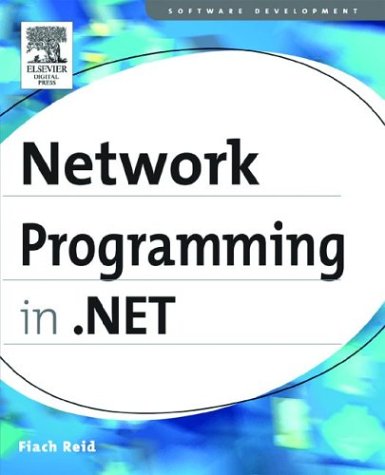
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionEditorAttribute.cs
- Model3DCollection.cs
- ContextQuery.cs
- EtwTrace.cs
- securestring.cs
- SessionPageStatePersister.cs
- TextDpi.cs
- Roles.cs
- FunctionDetailsReader.cs
- StagingAreaInputItem.cs
- _StreamFramer.cs
- HostingEnvironmentSection.cs
- MemberRelationshipService.cs
- IisTraceWebEventProvider.cs
- ChildTable.cs
- ListControlDesigner.cs
- DataTableMapping.cs
- CodeTypeReferenceExpression.cs
- M3DUtil.cs
- ManipulationLogic.cs
- HuffmanTree.cs
- WebServiceResponseDesigner.cs
- ConnectionStringSettings.cs
- UnmanagedMarshal.cs
- InvokeAction.cs
- FixedSOMTableCell.cs
- HitTestDrawingContextWalker.cs
- Stackframe.cs
- SocketInformation.cs
- SignedPkcs7.cs
- AllowedAudienceUriElement.cs
- DelayedRegex.cs
- LayoutTable.cs
- EntityContainerAssociationSetEnd.cs
- WebPartPersonalization.cs
- NullableLongSumAggregationOperator.cs
- StandardCommands.cs
- SynchronizationScope.cs
- SingleBodyParameterMessageFormatter.cs
- ByeOperationAsyncResult.cs
- HighContrastHelper.cs
- ResourceDisplayNameAttribute.cs
- BaseParser.cs
- EncodingInfo.cs
- LocationEnvironment.cs
- validationstate.cs
- HttpCookiesSection.cs
- formatter.cs
- DateTimePicker.cs
- DataSysAttribute.cs
- UnlockCardRequest.cs
- WorkerRequest.cs
- WebPartCloseVerb.cs
- ServiceDebugElement.cs
- CryptoApi.cs
- XamlHostingSection.cs
- ModuleElement.cs
- XPathBinder.cs
- XmlMapping.cs
- CellPartitioner.cs
- SiteMapProvider.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- MessageOperationFormatter.cs
- OdbcConnectionHandle.cs
- TypedTableBaseExtensions.cs
- WeakReference.cs
- WebSysDisplayNameAttribute.cs
- DependencyObject.cs
- InternalConfigSettingsFactory.cs
- MarginsConverter.cs
- ActivityInstanceReference.cs
- Effect.cs
- SerializationException.cs
- XmlSchemaCollection.cs
- ArrayList.cs
- CounterSetInstance.cs
- JournalNavigationScope.cs
- XmlSchemaSimpleTypeList.cs
- RSACryptoServiceProvider.cs
- InternalConfigHost.cs
- ValidationHelpers.cs
- Attributes.cs
- XmlDataSourceDesigner.cs
- ComUdtElementCollection.cs
- AssemblyNameProxy.cs
- CollectionContainer.cs
- VirtualDirectoryMapping.cs
- PrintControllerWithStatusDialog.cs
- MessageQueueConverter.cs
- SqlStream.cs
- BindingExpression.cs
- WebPartVerb.cs
- ResXBuildProvider.cs
- WinInet.cs
- TargetPerspective.cs
- PagePropertiesChangingEventArgs.cs
- DataSourceControlBuilder.cs
- DataServiceRequest.cs
- DataGridViewDataConnection.cs
- DoubleCollectionValueSerializer.cs