Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / OutputBuffer.cs / 1305376 / OutputBuffer.cs
namespace System.IO.Compression { using System.Diagnostics; internal class OutputBuffer { private byte[] byteBuffer; // buffer for storing bytes private int pos; // position private uint bitBuf; // store uncomplete bits private int bitCount; // number of bits in bitBuffer // set the output buffer we will be using internal void UpdateBuffer(byte[] output) { byteBuffer = output; pos = 0; } internal int BytesWritten { get { return pos; } } internal int FreeBytes { get { return byteBuffer.Length - pos; } } internal void WriteUInt16(ushort value) { Debug.Assert(FreeBytes >= 2, "No enough space in output buffer!"); byteBuffer[pos++] = (byte)value; byteBuffer[pos++] = (byte)(value >> 8); } internal void WriteBits(int n, uint bits) { Debug.Assert(n <= 16, "length must be larger than 16!"); bitBuf |= bits << bitCount; bitCount += n; if (bitCount >= 16) { Debug.Assert(byteBuffer.Length - pos >= 2, "No enough space in output buffer!"); byteBuffer[pos++] = unchecked((byte)bitBuf); byteBuffer[pos++] = unchecked((byte)(bitBuf >> 8)); bitCount -= 16; bitBuf >>= 16; } } // write the bits left in the output as bytes. internal void FlushBits() { // flush bits from bit buffer to output buffer while (bitCount >= 8) { byteBuffer[pos++] = unchecked((byte)bitBuf); bitCount -= 8; bitBuf >>= 8; } if (bitCount > 0) { byteBuffer[pos++] = unchecked((byte)bitBuf); bitBuf = 0; bitCount = 0; } } internal void WriteBytes(byte[] byteArray, int offset, int count) { Debug.Assert(FreeBytes >= count, "Not enough space in output buffer!"); // faster if (bitCount == 0) { Array.Copy(byteArray, offset, byteBuffer, pos, count); pos += count; } else { WriteBytesUnaligned(byteArray, offset, count); } } private void WriteBytesUnaligned(byte[] byteArray, int offset, int count) { for (int i = 0; i < count; i++) { byte b = byteArray[offset + i]; WriteByteUnaligned(b); } } private void WriteByteUnaligned(byte b) { WriteBits(8, b); } internal int BitsInBuffer { get { return (bitCount / 8) + 1; } } internal OutputBuffer.BufferState DumpState() { OutputBuffer.BufferState savedState; savedState.pos = pos; savedState.bitBuf = bitBuf; savedState.bitCount = bitCount; return savedState; } internal void RestoreState(OutputBuffer.BufferState state) { pos = state.pos; bitBuf = state.bitBuf; bitCount = state.bitCount; } internal struct BufferState { internal int pos; // position internal uint bitBuf; // store uncomplete bits internal int bitCount; // number of bits in bitBuffer } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
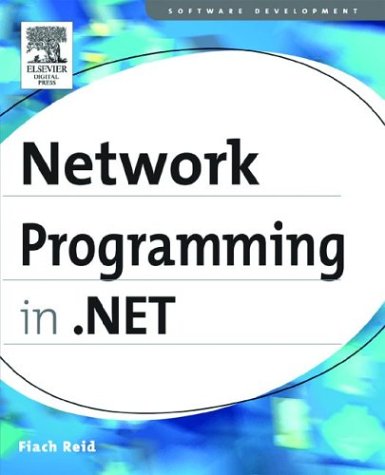
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeForwardedToAttribute.cs
- DataTransferEventArgs.cs
- CursorConverter.cs
- CodeRemoveEventStatement.cs
- RSAPKCS1SignatureFormatter.cs
- Command.cs
- ExpressionBuilderContext.cs
- Bits.cs
- SchemaCreator.cs
- ServerIdentity.cs
- FileSystemInfo.cs
- GridItemProviderWrapper.cs
- HttpRuntime.cs
- ExpanderAutomationPeer.cs
- ApplicationCommands.cs
- CodeTypeDeclarationCollection.cs
- WindowsTitleBar.cs
- MessageQueuePermissionEntryCollection.cs
- RuntimeCompatibilityAttribute.cs
- IdentityReference.cs
- CursorInteropHelper.cs
- FontSource.cs
- RuleSettingsCollection.cs
- SystemPens.cs
- SchemaTypeEmitter.cs
- ConnectionPointCookie.cs
- HighlightComponent.cs
- SqlParameterCollection.cs
- XmlQueryRuntime.cs
- SchemaCollectionCompiler.cs
- AccessibleObject.cs
- RawUIStateInputReport.cs
- TreeNodeStyle.cs
- Region.cs
- SpeechRecognitionEngine.cs
- ConfigurationManagerInternalFactory.cs
- HTMLTagNameToTypeMapper.cs
- DesignDataSource.cs
- PasswordRecovery.cs
- COM2IProvidePropertyBuilderHandler.cs
- PagerSettings.cs
- Encoder.cs
- DataGridSortCommandEventArgs.cs
- ApplicationCommands.cs
- WebPartManager.cs
- WebPartTransformerAttribute.cs
- HwndMouseInputProvider.cs
- CultureMapper.cs
- CodeCatchClause.cs
- ContentType.cs
- PaginationProgressEventArgs.cs
- SettingsContext.cs
- TraceContextRecord.cs
- HttpListener.cs
- PackageRelationshipSelector.cs
- TableAdapterManagerNameHandler.cs
- ViewCellRelation.cs
- WorkflowQueueInfo.cs
- BuilderPropertyEntry.cs
- Size.cs
- NumberFormatter.cs
- VirtualDirectoryMapping.cs
- RightsManagementInformation.cs
- SelfIssuedAuthProofToken.cs
- ISFTagAndGuidCache.cs
- FontUnitConverter.cs
- Baml2006SchemaContext.cs
- CharConverter.cs
- WindowsNonControl.cs
- LinkLabelLinkClickedEvent.cs
- OledbConnectionStringbuilder.cs
- DataGridViewSelectedColumnCollection.cs
- TreeBuilder.cs
- Label.cs
- sitestring.cs
- ColorKeyFrameCollection.cs
- DependencyPropertyValueSerializer.cs
- ConnectionsZone.cs
- RuleSetCollection.cs
- BaseParagraph.cs
- CompoundFileStreamReference.cs
- RbTree.cs
- XslVisitor.cs
- FormsAuthenticationModule.cs
- CodeExporter.cs
- ControlPaint.cs
- MsmqProcessProtocolHandler.cs
- SafeIUnknown.cs
- SQLResource.cs
- EndpointDiscoveryMetadataCD1.cs
- UnsafeNativeMethods.cs
- AuthenticatingEventArgs.cs
- DataGridViewColumnEventArgs.cs
- CardSpaceException.cs
- DBNull.cs
- PolicyUnit.cs
- ColorTransformHelper.cs
- MachineKeySection.cs
- RelatedImageListAttribute.cs
- ArgIterator.cs