Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / DomainLiteralReader.cs / 1305376 / DomainLiteralReader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mail{ using System.Net.Mime; using System.Diagnostics; using System.Text; // // RFC 2822 Section 3.4.1 - Addr-Spec, Domain-Literals // A domain literal is a domain identifier that does not conform to the dot-atom format (Section 3.2.4) and must be // enclosed in brackets '[' ']'. Domain literals may contain quoted-pairs. // internal static class DomainLiteralReader { // // Reads a domain literal in reverse // // Preconditions: // - Index must be within the bounds of the data string. // - The char at the given index is the initial bracket. (data[index] == EndSquareBracket) // // Return value: // - The next index past the terminating bracket (data[index + 1] == StartSquareBracket). // e.g. In (user@[domain]), starting at index=12 (]) returns index=4 (@). // // A FormatException will be thrown if: // - A non-escaped character is encountered that is not valid in a domain literal, including Unicode. // - The final bracket is not found. // internal static int ReadReverse(string data, int index) { Debug.Assert(0 <= index && index < data.Length, "index was outside the bounds of the string: " + index); Debug.Assert(data[index] == MailBnfHelper.EndSquareBracket, "data did not end with a square bracket"); // Skip the end bracket index--; do { // Check for valid whitespace index = WhitespaceReader.ReadFwsReverse(data, index); if (index < 0) { break; } // Check for escaped characters int quotedCharCount = QuotedPairReader.CountQuotedChars(data, index, false); if (quotedCharCount > 0) { // Skip quoted pairs index = index - quotedCharCount; } // Check for the terminating bracket else if (data[index] == MailBnfHelper.StartSquareBracket) { // We're done parsing return index - 1; } // Check for invalid characters else if (data[index] > MailBnfHelper.Ascii7bitMaxValue || !MailBnfHelper.Dtext[data[index]]) { throw new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter, data[index])); } // Valid char else { index--; } } while (index >= 0); // We didn't find a matching '[', throw. throw new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter, MailBnfHelper.EndSquareBracket)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
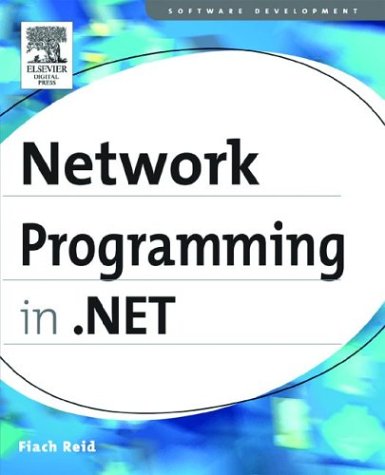
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectQueryProvider.cs
- TextWriterTraceListener.cs
- FileSystemEnumerable.cs
- Identifier.cs
- sortedlist.cs
- RepeatButtonAutomationPeer.cs
- Int32Rect.cs
- ContentDisposition.cs
- RuntimeDelegateArgument.cs
- SemanticValue.cs
- CompModSwitches.cs
- BindingCollection.cs
- TextEditorSpelling.cs
- WorkflowWebHostingModule.cs
- DataGridViewRowConverter.cs
- FlowDocumentScrollViewer.cs
- WebBrowserContainer.cs
- EndpointAddressMessageFilter.cs
- SubtreeProcessor.cs
- ControlBindingsCollection.cs
- XslTransform.cs
- ImmutableClientRuntime.cs
- InstanceKeyCompleteException.cs
- ProtocolsSection.cs
- UserControl.cs
- FileClassifier.cs
- Context.cs
- SqlDataSourceTableQuery.cs
- AdPostCacheSubstitution.cs
- BidPrivateBase.cs
- IdleTimeoutMonitor.cs
- PreProcessInputEventArgs.cs
- XmlObjectSerializerContext.cs
- TextContainerHelper.cs
- PolicyManager.cs
- SqlDataSourceQuery.cs
- UITypeEditor.cs
- BinaryFormatter.cs
- WindowsServiceElement.cs
- MasterPageParser.cs
- DPTypeDescriptorContext.cs
- SynchronizingStream.cs
- InputBuffer.cs
- LogRecordSequence.cs
- AppSettingsReader.cs
- GenericUriParser.cs
- PropagationProtocolsTracing.cs
- NullableDoubleMinMaxAggregationOperator.cs
- HashHelper.cs
- BindStream.cs
- AbandonedMutexException.cs
- MultiByteCodec.cs
- BasicKeyConstraint.cs
- ColorConverter.cs
- GridItemProviderWrapper.cs
- TableAutomationPeer.cs
- PtsHelper.cs
- RenderData.cs
- ListViewUpdateEventArgs.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- CharAnimationBase.cs
- TextOptions.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- WCFBuildProvider.cs
- ClientType.cs
- WebPartZoneAutoFormat.cs
- ReachIDocumentPaginatorSerializer.cs
- AspNetCompatibilityRequirementsMode.cs
- RequestCachingSection.cs
- XmlDataSource.cs
- DocumentApplication.cs
- ExistsInCollection.cs
- Psha1DerivedKeyGenerator.cs
- CategoryEditor.cs
- ADMembershipProvider.cs
- Comparer.cs
- ButtonStandardAdapter.cs
- OperationExecutionFault.cs
- TransformerTypeCollection.cs
- SignedInfo.cs
- DrawListViewSubItemEventArgs.cs
- OptimalTextSource.cs
- BaseCollection.cs
- LogicalExpr.cs
- SequenceDesigner.xaml.cs
- TreeNodeEventArgs.cs
- ExeConfigurationFileMap.cs
- MonthChangedEventArgs.cs
- CredentialCache.cs
- ConsumerConnectionPoint.cs
- ClientSettingsSection.cs
- TypeNameHelper.cs
- RequestSecurityTokenForGetBrowserToken.cs
- HttpListener.cs
- ObjectViewListener.cs
- MessageQueuePermission.cs
- Cursor.cs
- MgmtConfigurationRecord.cs
- NativeMethods.cs
- SecUtil.cs