Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / Converters / DataFieldConverter.cs / 1305376 / DataFieldConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls.Converters { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Diagnostics; using System.Runtime.InteropServices; using System.Globalization; using System.Web.UI.Design; using System.Web.UI.MobileControls; using System.Security.Permissions; ////// /// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class DataFieldConverter : TypeConverter { private const String _dataMemberPropertyName = "DataMember"; private const String _dataSourcePropertyName = "DataSource"; ////// Provides design-time support for a component's data field properties. /// ////// /// public DataFieldConverter() { } ////// Initializes a new instance of ///. /// /// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. /// ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } ////// Converts the given object to the converter's native type. /// ////// /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { object[] names = null; String dataMember = null; bool autoGenerateFields = false; bool autoGenerateFieldsSet = false; ObjectList objectList = null; if (context != null) { ArrayList list = new ArrayList(); PropertyDescriptorCollection props = null; IComponent component = context.Instance as IComponent; if (component is IDeviceSpecificChoiceDesigner) { Object owner = ((ChoicePropertyFilter)component).Owner; PropertyDescriptor pd = ((ICustomTypeDescriptor)component).GetProperties()[_dataMemberPropertyName]; Debug.Assert(pd != null, "Cannot get DataMember"); if (owner is ObjectList) { autoGenerateFields = ((ObjectList)owner).AutoGenerateFields; autoGenerateFieldsSet = true; } component = ((IDeviceSpecificChoiceDesigner)component).UnderlyingControl; // See if owner already has a DataMember dataMember = (String)pd.GetValue(owner); Debug.Assert(dataMember != null); if (dataMember != null && dataMember.Length == 0) { // Get it from underlying object. dataMember = (String)pd.GetValue(component); Debug.Assert(dataMember != null); } } if (component != null) { objectList = component as ObjectList; if (objectList != null) { foreach(ObjectListField field in objectList.Fields) { list.Add(field.Name); } if (!autoGenerateFieldsSet) { autoGenerateFields = objectList.AutoGenerateFields; } } if (objectList == null || autoGenerateFields) { ISite componentSite = component.Site; if (componentSite != null) { IDesignerHost designerHost = (IDesignerHost)componentSite.GetService(typeof(IDesignerHost)); if (designerHost != null) { IDesigner designer = designerHost.GetDesigner(component); if (designer is IDataSourceProvider) { IEnumerable dataSource = null; if (!String.IsNullOrEmpty(dataMember)) { DataBindingCollection dataBindings = ((HtmlControlDesigner)designer).DataBindings; DataBinding binding = dataBindings[_dataSourcePropertyName]; if (binding != null) { dataSource = DesignTimeData.GetSelectedDataSource( component, binding.Expression, dataMember); } } else { dataSource = ((IDataSourceProvider)designer).GetResolvedSelectedDataSource(); } if (dataSource != null) { props = DesignTimeData.GetDataFields(dataSource); } } } } } } if (props != null) { foreach (PropertyDescriptor propDesc in props) { list.Add(propDesc.Name); } } names = list.ToArray(); Array.Sort(names); } return new StandardValuesCollection(names); } ////// Gets the fields present within the selected data source if information about them is available. /// ////// /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// /// /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (context.Instance is IComponent) { // We only support the dropdown in single-select mode. return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ///
Link Menu
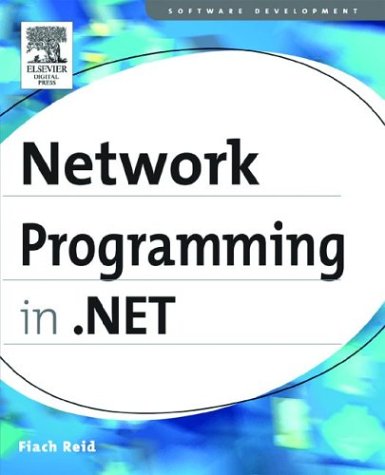
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CultureTableRecord.cs
- MessageQueueConverter.cs
- XmlDocument.cs
- IconHelper.cs
- Sql8ConformanceChecker.cs
- datacache.cs
- DrawItemEvent.cs
- DropShadowEffect.cs
- AtomParser.cs
- ZoneIdentityPermission.cs
- TemplatedWizardStep.cs
- ErrorLog.cs
- ReflectionUtil.cs
- SingleKeyFrameCollection.cs
- ViewPort3D.cs
- WindowsRegion.cs
- ThreadAbortException.cs
- GridEntryCollection.cs
- WebEventCodes.cs
- KnownBoxes.cs
- DictionaryGlobals.cs
- JsonReaderWriterFactory.cs
- Win32SafeHandles.cs
- GridErrorDlg.cs
- RecipientInfo.cs
- PriorityBindingExpression.cs
- StatusCommandUI.cs
- WebPartZoneCollection.cs
- LookupBindingPropertiesAttribute.cs
- TrailingSpaceComparer.cs
- PrimitiveType.cs
- EventLogPermissionEntry.cs
- AspNetHostingPermission.cs
- Light.cs
- PermissionListSet.cs
- BaseProcessor.cs
- FontStretchConverter.cs
- coordinatorscratchpad.cs
- UpDownBase.cs
- TextDecoration.cs
- securitycriticaldataClass.cs
- StringDictionary.cs
- ErrorReporting.cs
- Wizard.cs
- PasswordBox.cs
- EFAssociationProvider.cs
- ManipulationStartingEventArgs.cs
- EntityTemplateFactory.cs
- XamlTreeBuilderBamlRecordWriter.cs
- NominalTypeEliminator.cs
- UiaCoreProviderApi.cs
- GeneralTransform2DTo3DTo2D.cs
- CanonicalFontFamilyReference.cs
- FileAuthorizationModule.cs
- OleDbConnectionFactory.cs
- EmptyStringExpandableObjectConverter.cs
- InternalMappingException.cs
- ObjectQuery.cs
- XXXInfos.cs
- PhysicalOps.cs
- BaseTemplateBuildProvider.cs
- EntityCommandDefinition.cs
- WizardForm.cs
- SmiMetaDataProperty.cs
- ListSortDescription.cs
- AuthorizationRuleCollection.cs
- ClientBuildManager.cs
- GridViewCancelEditEventArgs.cs
- AuthenticationModuleElement.cs
- _Rfc2616CacheValidators.cs
- TreeViewItemAutomationPeer.cs
- ObjectConverter.cs
- BuilderPropertyEntry.cs
- GroupItem.cs
- ISAPIRuntime.cs
- Queue.cs
- BaseResourcesBuildProvider.cs
- DataGridViewTextBoxEditingControl.cs
- DrawTreeNodeEventArgs.cs
- StyleTypedPropertyAttribute.cs
- TransactedBatchingBehavior.cs
- GiveFeedbackEventArgs.cs
- MethodBody.cs
- XPathMultyIterator.cs
- Pens.cs
- LogExtent.cs
- controlskin.cs
- Variable.cs
- CodeTypeDeclarationCollection.cs
- ProfessionalColorTable.cs
- GridItemProviderWrapper.cs
- Composition.cs
- UserNamePasswordServiceCredential.cs
- Documentation.cs
- ColumnPropertiesGroup.cs
- ParameterCollection.cs
- PtsPage.cs
- BrowserCapabilitiesFactoryBase.cs
- StaticFileHandler.cs
- CellParagraph.cs