Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ResourceProperty.cs / 1305376 / ResourceProperty.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to describe properties on resources. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Diagnostics; using System.Linq; using System.Reflection; ///Use this class to describe a property on a resource. [DebuggerDisplay("{kind}: {name}")] public class ResourceProperty { #region Private fields. ///The name of this property. private readonly string name; ///The kind of resource Type that this property refers to. /// For e.g. for collection properties, this would return the resource type, /// and not the collection type that this property refers to. private readonly ResourceType propertyResourceType; ///The kind of property this is in relation to the resource. private ResourcePropertyKind kind; ///Is true, if this property is a actual clr property on the property type. In this case, /// astoria service will do reflection over the property type and get/set value for this property. /// False means that astoria service needs to go through the IDataServiceQueryProvider contract to get/set value for this provider. private bool canReflectOnInstanceTypeProperty; ///Is true, if the resource property is set to readonly i.e. fully initialized and validated. No more changes can be made, /// once the resource property is set to readonly. private bool isReadOnly; ///MIME type for the property, if it's a primitive value. private string mimeType; #endregion Private fields. ////// Initializes a new ResourceProperty instance for an open property. /// /// Property name for the property. /// Property kind. /// The type of the resource that this property refers to public ResourceProperty( string name, ResourcePropertyKind kind, ResourceType propertyResourceType) { WebUtil.CheckStringArgumentNull(name, "name"); WebUtil.CheckArgumentNull(propertyResourceType, "propertyResourceType"); ValidatePropertyParameters(kind, propertyResourceType); this.kind = kind; this.name = name; this.propertyResourceType = propertyResourceType; this.canReflectOnInstanceTypeProperty = true; } #region Properties ///Indicates whether this property can be accessed through reflection on the declaring resource instance type. ///A 'true' value here typically indicates astoria service will use reflection to get the property info on the declaring ResourceType.InstanceType. /// 'false' means that astoria service will go through IDataServiceQueryProvider interface to get/set this property's value. public bool CanReflectOnInstanceTypeProperty { get { return this.canReflectOnInstanceTypeProperty; } set { this.ThrowIfSealed(); this.canReflectOnInstanceTypeProperty = value; } } ////// The resource type that is property refers to [For collection, /// this will return the element of the collection, and not the /// collection]. /// public ResourceType ResourceType { [DebuggerStepThrough] get { return this.propertyResourceType; } } ///The property name. public string Name { [DebuggerStepThrough] get { return this.name; } } ///MIME type for the property, if it's a primitive value; null if none specified. public string MimeType { [DebuggerStepThrough] get { return this.mimeType; } set { this.ThrowIfSealed(); if (String.IsNullOrEmpty(value)) { throw new InvalidOperationException(Strings.ResourceProperty_MimeTypeAttributeEmpty(this.Name)); } if (this.ResourceType.ResourceTypeKind != ResourceTypeKind.Primitive) { throw new InvalidOperationException(Strings.ResourceProperty_MimeTypeAttributeOnNonPrimitive(this.Name, this.ResourceType.FullName)); } if (!WebUtil.IsValidMimeType(value)) { throw new InvalidOperationException(Strings.ResourceProperty_MimeTypeNotValid(value, this.Name)); } this.mimeType = value; } } ///The kind of property this is in relation to the resource. public ResourcePropertyKind Kind { [DebuggerStepThrough] get { return this.kind; } [DebuggerStepThrough] internal set { Debug.Assert(!this.isReadOnly, "Kind - the resource property cannot be readonly"); this.kind = value; } } ////// PlaceHolder to hold custom state information about resource property. /// public object CustomState { get; set; } ////// Returns true, if this resource property has been set to read only. Otherwise returns false. /// public bool IsReadOnly { get { return this.isReadOnly; } } ///The kind of type this property has in relation to the data service. internal ResourceTypeKind TypeKind { get { return this.ResourceType.ResourceTypeKind; } } ///The type of the property. internal Type Type { get { if (this.Kind == ResourcePropertyKind.ResourceSetReference) { return typeof(System.Collections.Generic.IEnumerable<>).MakeGenericType(this.propertyResourceType.InstanceType); } else { return this.propertyResourceType.InstanceType; } } } #endregion Properties #region Methods ////// Sets the resource property to readonly. Once this method is called, no more changes can be made to resource property. /// public void SetReadOnly() { // If its already set to readonly, do no-op if (this.isReadOnly) { return; } this.ResourceType.SetReadOnly(); this.isReadOnly = true; } ////// return true if this property is of the given kind /// /// flag which needs to be checked on the current property kind ///true if the current property is of the given kind internal bool IsOfKind(ResourcePropertyKind checkKind) { return ResourceProperty.IsOfKind(this.kind, checkKind); } ////// return true if the given property kind is of the given kind /// /// kind of the property /// flag which needs to be checked on property kind ///true if the kind flag is set on the given property kind private static bool IsOfKind(ResourcePropertyKind propertyKind, ResourcePropertyKind kind) { return ((propertyKind & kind) == kind); } ////// Validates that the given property kind is valid /// /// property kind to check /// name of the parameter private static void CheckResourcePropertyKind(ResourcePropertyKind kind, string parameterName) { // For open properties, resource property instance is created only for nav properties. if (kind != ResourcePropertyKind.ResourceReference && kind != ResourcePropertyKind.ResourceSetReference && kind != ResourcePropertyKind.ComplexType && kind != ResourcePropertyKind.Primitive && kind != (ResourcePropertyKind.Primitive | ResourcePropertyKind.Key) && kind != (ResourcePropertyKind.Primitive | ResourcePropertyKind.ETag)) { throw new ArgumentException(Strings.InvalidEnumValue(kind.GetType().Name), parameterName); } } ////// Validate the parameters of the resource property constructor. /// /// kind of the resource property. /// resource type that this property refers to. private static void ValidatePropertyParameters(ResourcePropertyKind kind, ResourceType propertyResourceType) { CheckResourcePropertyKind(kind, "kind"); if (IsOfKind(kind, ResourcePropertyKind.ResourceReference) || IsOfKind(kind, ResourcePropertyKind.ResourceSetReference)) { if (propertyResourceType.ResourceTypeKind != ResourceTypeKind.EntityType) { throw new ArgumentException(Strings.ResourceProperty_PropertyKindAndResourceTypeKindMismatch("kind", "propertyResourceType")); } } if (IsOfKind(kind, ResourcePropertyKind.Primitive)) { if (propertyResourceType.ResourceTypeKind != ResourceTypeKind.Primitive) { throw new ArgumentException(Strings.ResourceProperty_PropertyKindAndResourceTypeKindMismatch("kind", "propertyResourceType")); } } if (IsOfKind(kind, ResourcePropertyKind.ComplexType)) { if (propertyResourceType.ResourceTypeKind != ResourceTypeKind.ComplexType) { throw new ArgumentException(Strings.ResourceProperty_PropertyKindAndResourceTypeKindMismatch("kind", "propertyResourceType")); } } if (IsOfKind(kind, ResourcePropertyKind.Key) && Nullable.GetUnderlyingType(propertyResourceType.InstanceType) != null) { throw new ArgumentException(Strings.ResourceProperty_KeyPropertiesCannotBeNullable); } } ////// Checks if the resource type is sealed. If not, it throws an InvalidOperationException. /// private void ThrowIfSealed() { if (this.isReadOnly) { throw new InvalidOperationException(Strings.ResourceProperty_Sealed(this.Name)); } } #endregion Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
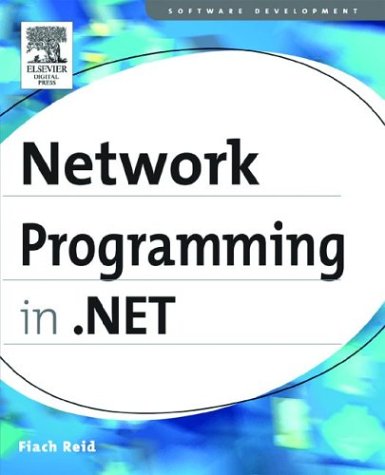
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsIdentity.cs
- Setter.cs
- AssemblyBuilderData.cs
- CompilerScopeManager.cs
- DataReaderContainer.cs
- XPathNodeInfoAtom.cs
- SoapBinding.cs
- SafeRightsManagementQueryHandle.cs
- InkCanvasInnerCanvas.cs
- SendKeys.cs
- TraceSource.cs
- HGlobalSafeHandle.cs
- SetStateDesigner.cs
- CommittableTransaction.cs
- DataObjectEventArgs.cs
- NamespaceInfo.cs
- ZipIOLocalFileDataDescriptor.cs
- DriveNotFoundException.cs
- UserControl.cs
- HtmlGenericControl.cs
- externdll.cs
- FormViewUpdatedEventArgs.cs
- BinarySecretSecurityToken.cs
- LookupNode.cs
- InvalidEnumArgumentException.cs
- Internal.cs
- ObjectConverter.cs
- WebExceptionStatus.cs
- NullRuntimeConfig.cs
- BamlVersionHeader.cs
- RotateTransform.cs
- SafeEventLogReadHandle.cs
- DispatcherFrame.cs
- DataGridViewCellValueEventArgs.cs
- ComplexBindingPropertiesAttribute.cs
- DockingAttribute.cs
- TypeResolver.cs
- NumberSubstitution.cs
- HiddenFieldPageStatePersister.cs
- CaseCqlBlock.cs
- xml.cs
- StyleCollection.cs
- DesignerPerfEventProvider.cs
- DataServiceKeyAttribute.cs
- EventLogPermission.cs
- NamespaceList.cs
- entitydatasourceentitysetnameconverter.cs
- ReadonlyMessageFilter.cs
- WebHttpBinding.cs
- _RequestCacheProtocol.cs
- DynamicPropertyHolder.cs
- ResizeGrip.cs
- PngBitmapEncoder.cs
- UnionCqlBlock.cs
- SelectionProviderWrapper.cs
- TypeConstant.cs
- Item.cs
- XPathSingletonIterator.cs
- SizeAnimationUsingKeyFrames.cs
- StrongNameUtility.cs
- PointAnimation.cs
- CodeTypeParameter.cs
- Int32CollectionConverter.cs
- ProcessHostFactoryHelper.cs
- ProgressChangedEventArgs.cs
- WindowInteractionStateTracker.cs
- HasCopySemanticsAttribute.cs
- GeneratedView.cs
- Int32Animation.cs
- StandardTransformFactory.cs
- TableLayoutColumnStyleCollection.cs
- XmlSignatureManifest.cs
- _AcceptOverlappedAsyncResult.cs
- JsonByteArrayDataContract.cs
- CodeCastExpression.cs
- AudienceUriMode.cs
- ConnectionsZoneAutoFormat.cs
- SqlMethodCallConverter.cs
- Int16.cs
- ResponseStream.cs
- DbConnectionPoolGroupProviderInfo.cs
- DbParameterCollectionHelper.cs
- HttpWebResponse.cs
- TypeDependencyAttribute.cs
- CommonGetThemePartSize.cs
- LinearQuaternionKeyFrame.cs
- MouseButton.cs
- CompositionTarget.cs
- DataGridViewRow.cs
- SafeTimerHandle.cs
- CodeDomSerializerException.cs
- ExpandCollapseProviderWrapper.cs
- GetRecipientListRequest.cs
- PerformanceCounterManager.cs
- FontCacheUtil.cs
- TextRunCacheImp.cs
- DataGridViewToolTip.cs
- WebServiceFaultDesigner.cs
- AssemblyResourceLoader.cs
- HashSetEqualityComparer.cs