Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceRequestArgs.cs / 1305376 / DataServiceRequestArgs.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// This class represents additional metadata to be applied to a request // sent from the client to a data service. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; ////// This class represent additional metadata to be applied to a request /// sent from the client to a data service. /// public class DataServiceRequestArgs { ////// The headers collection for the request. /// This is just a storage, no validation is done on this. /// private readonly Dictionaryheaders; /// /// Constructs a new DataServiceRequestArgs instance /// public DataServiceRequestArgs() { this.headers = new Dictionary(EqualityComparer .Default); } /// /// Sets the mime type (ex. image/png) to be used when retrieving the stream. /// Note that no validation is done on the contents of this property. /// It is the responsibility of the user to format it correctly to be used /// as the value of an HTTP Accept header. /// public string AcceptContentType { get { return this.GetHeaderValue(XmlConstants.HttpRequestAccept); } set { this.SetHeaderValue(XmlConstants.HttpRequestAccept, value); } } ////// Sets the Content-Type header to be used when sending the stream to the server. /// Note that no validation is done on the contents of this property. /// It is the responsibility of the user to format it correctly to be used /// as the value of an HTTP Content-Type header. /// public string ContentType { get { return this.GetHeaderValue(XmlConstants.HttpContentType); } set { this.SetHeaderValue(XmlConstants.HttpContentType, value); } } ////// Sets the Slug header to be used when sending the stream to the server. /// Note that no validation is done on the contents of this property. /// It is the responsibility of the user to format it correctly to be used /// as the value of an HTTP Slug header. /// public string Slug { get { return this.GetHeaderValue(XmlConstants.HttpSlug); } set { this.SetHeaderValue(XmlConstants.HttpSlug, value); } } ////// Dictionary containing all the request headers to be used when retrieving the stream. /// The user should take care so as to not alter an HTTP header which will change /// the meaning of the request. /// No validation is performed on the header names or values. /// This class will not attempt to fix up any of the headers specified and /// will try to use them "as is". /// public DictionaryHeaders { get { return this.headers; } } /// /// Helper to return a value of the header. /// /// The name of the header to get. ///The value of the header or null if the header is not set. private string GetHeaderValue(string header) { string value; if (!this.headers.TryGetValue(header, out value)) { return null; } return value; } ////// Helper to set a value of the header /// /// The name of the header to set. /// The value to set for the header. If this is null the header will be removed. private void SetHeaderValue(string header, string value) { if (value == null) { if (this.headers.ContainsKey(header)) { this.headers.Remove(header); } } else { this.headers[header] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
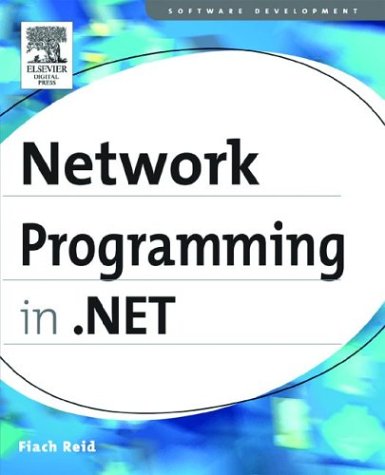
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaSimpleTypeRestriction.cs
- ElementProxy.cs
- ErrorsHelper.cs
- GeometryHitTestResult.cs
- TagNameToTypeMapper.cs
- DispatcherObject.cs
- MatrixTransform3D.cs
- DigitalSignatureProvider.cs
- WebPartCancelEventArgs.cs
- GradientBrush.cs
- ManipulationDevice.cs
- TemplateComponentConnector.cs
- Psha1DerivedKeyGeneratorHelper.cs
- SqlAliasesReferenced.cs
- Pair.cs
- RuleSettingsCollection.cs
- PathData.cs
- ToolStripManager.cs
- ExpandCollapsePattern.cs
- PageAction.cs
- ScrollBarRenderer.cs
- httpserverutility.cs
- ResourceType.cs
- CompiledQuery.cs
- Message.cs
- FrameworkObject.cs
- ProcessHostMapPath.cs
- MsmqIntegrationSecurityElement.cs
- DataGridViewColumnCollectionEditor.cs
- AssemblyInfo.cs
- SoapElementAttribute.cs
- Button.cs
- unsafenativemethodsother.cs
- Pair.cs
- MatrixIndependentAnimationStorage.cs
- WCFBuildProvider.cs
- AllowedAudienceUriElementCollection.cs
- TextModifier.cs
- SpeakCompletedEventArgs.cs
- OleStrCAMarshaler.cs
- TextServicesDisplayAttribute.cs
- TraceContext.cs
- ApplicationFileParser.cs
- XmlException.cs
- WorkflowRuntimeServiceElement.cs
- FormViewModeEventArgs.cs
- Condition.cs
- SqlRowUpdatedEvent.cs
- PathGeometry.cs
- MenuEventArgs.cs
- SQLRoleProvider.cs
- SimpleTextLine.cs
- QilBinary.cs
- RecognizedAudio.cs
- KeyNotFoundException.cs
- StrongTypingException.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- Axis.cs
- BinaryObjectInfo.cs
- StylusEventArgs.cs
- ShaderRenderModeValidation.cs
- mil_commands.cs
- ByteStorage.cs
- Base64Decoder.cs
- ToolBar.cs
- Symbol.cs
- UDPClient.cs
- IBuiltInEvidence.cs
- Utilities.cs
- NamespaceEmitter.cs
- Deflater.cs
- DBSqlParserColumnCollection.cs
- TraceRecords.cs
- TriggerBase.cs
- RoleService.cs
- PKCS1MaskGenerationMethod.cs
- ToolZone.cs
- COM2PropertyDescriptor.cs
- XmlStreamNodeWriter.cs
- TextElementAutomationPeer.cs
- TemplateComponentConnector.cs
- DataGridBoundColumn.cs
- FloaterParaClient.cs
- Wrapper.cs
- DataControlCommands.cs
- UnsafeNativeMethods.cs
- IdentityReference.cs
- FontFamily.cs
- ImmutableObjectAttribute.cs
- AutomationPatternInfo.cs
- RelOps.cs
- VirtualizedContainerService.cs
- TransformedBitmap.cs
- PassportPrincipal.cs
- SqlMethods.cs
- DebuggerService.cs
- DataFieldCollectionEditor.cs
- TextProperties.cs
- NotifyCollectionChangedEventArgs.cs
- TextParaClient.cs